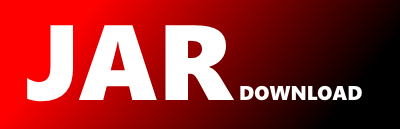
io.fabric8.kubernetes.api.model.PatchOptionsFluentImpl Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.lang.StringBuilder;
import com.fasterxml.jackson.annotation.JsonInclude;
import java.util.ArrayList;
import java.lang.String;
import io.fabric8.kubernetes.api.builder.Predicate;
import java.lang.StringBuffer;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.Collection;
import java.lang.Object;
import java.util.List;
import java.lang.Boolean;
public class PatchOptionsFluentImpl> extends io.fabric8.kubernetes.api.builder.BaseFluent implements PatchOptionsFluent{
private String apiVersion;
private List dryRun = new ArrayList();
private String fieldManager;
private Boolean force;
private String kind;
public PatchOptionsFluentImpl(){
}
public PatchOptionsFluentImpl(PatchOptions instance){
this.withApiVersion(instance.getApiVersion());
this.withDryRun(instance.getDryRun());
this.withFieldManager(instance.getFieldManager());
this.withForce(instance.getForce());
this.withKind(instance.getKind());
}
public String getApiVersion(){
return this.apiVersion;
}
public A withApiVersion(String apiVersion){
this.apiVersion=apiVersion; return (A) this;
}
public Boolean hasApiVersion(){
return this.apiVersion != null;
}
public A withNewApiVersion(String arg1){
return (A)withApiVersion(new String(arg1));
}
public A withNewApiVersion(StringBuilder arg1){
return (A)withApiVersion(new String(arg1));
}
public A withNewApiVersion(StringBuffer arg1){
return (A)withApiVersion(new String(arg1));
}
public A addToDryRun(int index,String item){
if (this.dryRun == null) {this.dryRun = new ArrayList();}
this.dryRun.add(index, item);
return (A)this;
}
public A setToDryRun(int index,String item){
if (this.dryRun == null) {this.dryRun = new ArrayList();}
this.dryRun.set(index, item); return (A)this;
}
public A addToDryRun(String... items){
if (this.dryRun == null) {this.dryRun = new ArrayList();}
for (String item : items) {this.dryRun.add(item);} return (A)this;
}
public A addAllToDryRun(Collection items){
if (this.dryRun == null) {this.dryRun = new ArrayList();}
for (String item : items) {this.dryRun.add(item);} return (A)this;
}
public A removeFromDryRun(String... items){
for (String item : items) {if (this.dryRun!= null){ this.dryRun.remove(item);}} return (A)this;
}
public A removeAllFromDryRun(Collection items){
for (String item : items) {if (this.dryRun!= null){ this.dryRun.remove(item);}} return (A)this;
}
public List getDryRun(){
return this.dryRun;
}
public String getDryRun(int index){
return this.dryRun.get(index);
}
public String getFirstDryRun(){
return this.dryRun.get(0);
}
public String getLastDryRun(){
return this.dryRun.get(dryRun.size() - 1);
}
public String getMatchingDryRun(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (String item: dryRun) { if(predicate.apply(item)){ return item;} } return null;
}
public Boolean hasMatchingDryRun(io.fabric8.kubernetes.api.builder.Predicate predicate){
for (String item: dryRun) { if(predicate.apply(item)){ return true;} } return false;
}
public A withDryRun(List dryRun){
if (this.dryRun != null) { _visitables.get("dryRun").removeAll(this.dryRun);}
if (dryRun != null) {this.dryRun = new ArrayList(); for (String item : dryRun){this.addToDryRun(item);}} else { this.dryRun = null;} return (A) this;
}
public A withDryRun(String... dryRun){
if (this.dryRun != null) {this.dryRun.clear();}
if (dryRun != null) {for (String item :dryRun){ this.addToDryRun(item);}} return (A) this;
}
public Boolean hasDryRun(){
return dryRun != null && !dryRun.isEmpty();
}
public A addNewDryRun(String arg1){
return (A)addToDryRun(new String(arg1));
}
public A addNewDryRun(StringBuilder arg1){
return (A)addToDryRun(new String(arg1));
}
public A addNewDryRun(StringBuffer arg1){
return (A)addToDryRun(new String(arg1));
}
public String getFieldManager(){
return this.fieldManager;
}
public A withFieldManager(String fieldManager){
this.fieldManager=fieldManager; return (A) this;
}
public Boolean hasFieldManager(){
return this.fieldManager != null;
}
public A withNewFieldManager(String arg1){
return (A)withFieldManager(new String(arg1));
}
public A withNewFieldManager(StringBuilder arg1){
return (A)withFieldManager(new String(arg1));
}
public A withNewFieldManager(StringBuffer arg1){
return (A)withFieldManager(new String(arg1));
}
public Boolean isForce(){
return this.force;
}
public A withForce(Boolean force){
this.force=force; return (A) this;
}
public Boolean hasForce(){
return this.force != null;
}
public A withNewForce(String arg1){
return (A)withForce(new Boolean(arg1));
}
public A withNewForce(boolean arg1){
return (A)withForce(new Boolean(arg1));
}
public String getKind(){
return this.kind;
}
public A withKind(String kind){
this.kind=kind; return (A) this;
}
public Boolean hasKind(){
return this.kind != null;
}
public A withNewKind(String arg1){
return (A)withKind(new String(arg1));
}
public A withNewKind(StringBuilder arg1){
return (A)withKind(new String(arg1));
}
public A withNewKind(StringBuffer arg1){
return (A)withKind(new String(arg1));
}
public boolean equals(Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PatchOptionsFluentImpl that = (PatchOptionsFluentImpl) o;
if (apiVersion != null ? !apiVersion.equals(that.apiVersion) :that.apiVersion != null) return false;
if (dryRun != null ? !dryRun.equals(that.dryRun) :that.dryRun != null) return false;
if (fieldManager != null ? !fieldManager.equals(that.fieldManager) :that.fieldManager != null) return false;
if (force != null ? !force.equals(that.force) :that.force != null) return false;
if (kind != null ? !kind.equals(that.kind) :that.kind != null) return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy