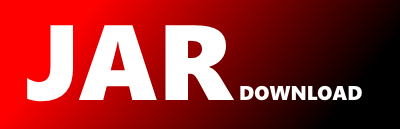
io.fabric8.kubernetes.api.model.KubeSchemaFluent Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import java.lang.String;
import com.fasterxml.jackson.databind.SerializerProvider;
import java.lang.Deprecated;
import java.lang.StringBuffer;
import java.lang.Override;
import java.lang.Long;
import java.lang.StringBuilder;
import com.fasterxml.jackson.databind.JsonSerializer;
import com.fasterxml.jackson.core.JsonParser;
import io.fabric8.kubernetes.api.model.version.Info;
import java.lang.Integer;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import io.fabric8.kubernetes.api.model.version.InfoFluent;
import com.fasterxml.jackson.databind.JsonDeserializer;
import java.lang.Boolean;
import java.lang.Object;
import java.util.Map;
import io.fabric8.kubernetes.api.model.version.InfoBuilder;
import com.fasterxml.jackson.core.JsonGenerator;
import io.fabric8.kubernetes.api.builder.Fluent;
import io.fabric8.kubernetes.api.builder.Nested;
import com.fasterxml.jackson.databind.DeserializationContext;
public interface KubeSchemaFluent> extends Fluent{
/**
* This method has been deprecated, please use method buildAPIGroup instead.
* @return The buildable object.
*/
@Deprecated public APIGroup getAPIGroup();
public APIGroup buildAPIGroup();
public A withAPIGroup(APIGroup aPIGroup);
public Boolean hasAPIGroup();
public KubeSchemaFluent.APIGroupNested withNewAPIGroup();
public KubeSchemaFluent.APIGroupNested withNewAPIGroupLike(APIGroup item);
public KubeSchemaFluent.APIGroupNested editAPIGroup();
public KubeSchemaFluent.APIGroupNested editOrNewAPIGroup();
public KubeSchemaFluent.APIGroupNested editOrNewAPIGroupLike(APIGroup item);
/**
* This method has been deprecated, please use method buildAPIGroupList instead.
* @return The buildable object.
*/
@Deprecated public APIGroupList getAPIGroupList();
public APIGroupList buildAPIGroupList();
public A withAPIGroupList(APIGroupList aPIGroupList);
public Boolean hasAPIGroupList();
public KubeSchemaFluent.APIGroupListNested withNewAPIGroupList();
public KubeSchemaFluent.APIGroupListNested withNewAPIGroupListLike(APIGroupList item);
public KubeSchemaFluent.APIGroupListNested editAPIGroupList();
public KubeSchemaFluent.APIGroupListNested editOrNewAPIGroupList();
public KubeSchemaFluent.APIGroupListNested editOrNewAPIGroupListLike(APIGroupList item);
/**
* This method has been deprecated, please use method buildBaseKubernetesList instead.
* @return The buildable object.
*/
@Deprecated public BaseKubernetesList getBaseKubernetesList();
public BaseKubernetesList buildBaseKubernetesList();
public A withBaseKubernetesList(BaseKubernetesList baseKubernetesList);
public Boolean hasBaseKubernetesList();
public KubeSchemaFluent.BaseKubernetesListNested withNewBaseKubernetesList();
public KubeSchemaFluent.BaseKubernetesListNested withNewBaseKubernetesListLike(BaseKubernetesList item);
public KubeSchemaFluent.BaseKubernetesListNested editBaseKubernetesList();
public KubeSchemaFluent.BaseKubernetesListNested editOrNewBaseKubernetesList();
public KubeSchemaFluent.BaseKubernetesListNested editOrNewBaseKubernetesListLike(BaseKubernetesList item);
/**
* This method has been deprecated, please use method buildBinding instead.
* @return The buildable object.
*/
@Deprecated public Binding getBinding();
public Binding buildBinding();
public A withBinding(Binding binding);
public Boolean hasBinding();
public KubeSchemaFluent.BindingNested withNewBinding();
public KubeSchemaFluent.BindingNested withNewBindingLike(Binding item);
public KubeSchemaFluent.BindingNested editBinding();
public KubeSchemaFluent.BindingNested editOrNewBinding();
public KubeSchemaFluent.BindingNested editOrNewBindingLike(Binding item);
/**
* This method has been deprecated, please use method buildComponentStatus instead.
* @return The buildable object.
*/
@Deprecated public ComponentStatus getComponentStatus();
public ComponentStatus buildComponentStatus();
public A withComponentStatus(ComponentStatus componentStatus);
public Boolean hasComponentStatus();
public KubeSchemaFluent.ComponentStatusNested withNewComponentStatus();
public KubeSchemaFluent.ComponentStatusNested withNewComponentStatusLike(ComponentStatus item);
public KubeSchemaFluent.ComponentStatusNested editComponentStatus();
public KubeSchemaFluent.ComponentStatusNested editOrNewComponentStatus();
public KubeSchemaFluent.ComponentStatusNested editOrNewComponentStatusLike(ComponentStatus item);
/**
* This method has been deprecated, please use method buildComponentStatusList instead.
* @return The buildable object.
*/
@Deprecated public ComponentStatusList getComponentStatusList();
public ComponentStatusList buildComponentStatusList();
public A withComponentStatusList(ComponentStatusList componentStatusList);
public Boolean hasComponentStatusList();
public KubeSchemaFluent.ComponentStatusListNested withNewComponentStatusList();
public KubeSchemaFluent.ComponentStatusListNested withNewComponentStatusListLike(ComponentStatusList item);
public KubeSchemaFluent.ComponentStatusListNested editComponentStatusList();
public KubeSchemaFluent.ComponentStatusListNested editOrNewComponentStatusList();
public KubeSchemaFluent.ComponentStatusListNested editOrNewComponentStatusListLike(ComponentStatusList item);
/**
* This method has been deprecated, please use method buildConfig instead.
* @return The buildable object.
*/
@Deprecated public Config getConfig();
public Config buildConfig();
public A withConfig(Config config);
public Boolean hasConfig();
public KubeSchemaFluent.ConfigNested withNewConfig();
public KubeSchemaFluent.ConfigNested withNewConfigLike(Config item);
public KubeSchemaFluent.ConfigNested editConfig();
public KubeSchemaFluent.ConfigNested editOrNewConfig();
public KubeSchemaFluent.ConfigNested editOrNewConfigLike(Config item);
/**
* This method has been deprecated, please use method buildConfigMap instead.
* @return The buildable object.
*/
@Deprecated public ConfigMap getConfigMap();
public ConfigMap buildConfigMap();
public A withConfigMap(ConfigMap configMap);
public Boolean hasConfigMap();
public KubeSchemaFluent.ConfigMapNested withNewConfigMap();
public KubeSchemaFluent.ConfigMapNested withNewConfigMapLike(ConfigMap item);
public KubeSchemaFluent.ConfigMapNested editConfigMap();
public KubeSchemaFluent.ConfigMapNested editOrNewConfigMap();
public KubeSchemaFluent.ConfigMapNested editOrNewConfigMapLike(ConfigMap item);
/**
* This method has been deprecated, please use method buildConfigMapList instead.
* @return The buildable object.
*/
@Deprecated public ConfigMapList getConfigMapList();
public ConfigMapList buildConfigMapList();
public A withConfigMapList(ConfigMapList configMapList);
public Boolean hasConfigMapList();
public KubeSchemaFluent.ConfigMapListNested withNewConfigMapList();
public KubeSchemaFluent.ConfigMapListNested withNewConfigMapListLike(ConfigMapList item);
public KubeSchemaFluent.ConfigMapListNested editConfigMapList();
public KubeSchemaFluent.ConfigMapListNested editOrNewConfigMapList();
public KubeSchemaFluent.ConfigMapListNested editOrNewConfigMapListLike(ConfigMapList item);
/**
* This method has been deprecated, please use method buildContainerStatus instead.
* @return The buildable object.
*/
@Deprecated public ContainerStatus getContainerStatus();
public ContainerStatus buildContainerStatus();
public A withContainerStatus(ContainerStatus containerStatus);
public Boolean hasContainerStatus();
public KubeSchemaFluent.ContainerStatusNested withNewContainerStatus();
public KubeSchemaFluent.ContainerStatusNested withNewContainerStatusLike(ContainerStatus item);
public KubeSchemaFluent.ContainerStatusNested editContainerStatus();
public KubeSchemaFluent.ContainerStatusNested editOrNewContainerStatus();
public KubeSchemaFluent.ContainerStatusNested editOrNewContainerStatusLike(ContainerStatus item);
/**
* This method has been deprecated, please use method buildCreateOptions instead.
* @return The buildable object.
*/
@Deprecated public CreateOptions getCreateOptions();
public CreateOptions buildCreateOptions();
public A withCreateOptions(CreateOptions createOptions);
public Boolean hasCreateOptions();
public KubeSchemaFluent.CreateOptionsNested withNewCreateOptions();
public KubeSchemaFluent.CreateOptionsNested withNewCreateOptionsLike(CreateOptions item);
public KubeSchemaFluent.CreateOptionsNested editCreateOptions();
public KubeSchemaFluent.CreateOptionsNested editOrNewCreateOptions();
public KubeSchemaFluent.CreateOptionsNested editOrNewCreateOptionsLike(CreateOptions item);
/**
* This method has been deprecated, please use method buildDeleteOptions instead.
* @return The buildable object.
*/
@Deprecated public DeleteOptions getDeleteOptions();
public DeleteOptions buildDeleteOptions();
public A withDeleteOptions(DeleteOptions deleteOptions);
public Boolean hasDeleteOptions();
public KubeSchemaFluent.DeleteOptionsNested withNewDeleteOptions();
public KubeSchemaFluent.DeleteOptionsNested withNewDeleteOptionsLike(DeleteOptions item);
public KubeSchemaFluent.DeleteOptionsNested editDeleteOptions();
public KubeSchemaFluent.DeleteOptionsNested editOrNewDeleteOptions();
public KubeSchemaFluent.DeleteOptionsNested editOrNewDeleteOptionsLike(DeleteOptions item);
/**
* This method has been deprecated, please use method buildEndpointPort instead.
* @return The buildable object.
*/
@Deprecated public EndpointPort getEndpointPort();
public EndpointPort buildEndpointPort();
public A withEndpointPort(EndpointPort endpointPort);
public Boolean hasEndpointPort();
public A withNewEndpointPort(String appProtocol,String name,Integer port,String protocol);
public KubeSchemaFluent.EndpointPortNested withNewEndpointPort();
public KubeSchemaFluent.EndpointPortNested withNewEndpointPortLike(EndpointPort item);
public KubeSchemaFluent.EndpointPortNested editEndpointPort();
public KubeSchemaFluent.EndpointPortNested editOrNewEndpointPort();
public KubeSchemaFluent.EndpointPortNested editOrNewEndpointPortLike(EndpointPort item);
/**
* This method has been deprecated, please use method buildEndpoints instead.
* @return The buildable object.
*/
@Deprecated public Endpoints getEndpoints();
public Endpoints buildEndpoints();
public A withEndpoints(Endpoints endpoints);
public Boolean hasEndpoints();
public KubeSchemaFluent.EndpointsNested withNewEndpoints();
public KubeSchemaFluent.EndpointsNested withNewEndpointsLike(Endpoints item);
public KubeSchemaFluent.EndpointsNested editEndpoints();
public KubeSchemaFluent.EndpointsNested editOrNewEndpoints();
public KubeSchemaFluent.EndpointsNested editOrNewEndpointsLike(Endpoints item);
/**
* This method has been deprecated, please use method buildEndpointsList instead.
* @return The buildable object.
*/
@Deprecated public EndpointsList getEndpointsList();
public EndpointsList buildEndpointsList();
public A withEndpointsList(EndpointsList endpointsList);
public Boolean hasEndpointsList();
public KubeSchemaFluent.EndpointsListNested withNewEndpointsList();
public KubeSchemaFluent.EndpointsListNested withNewEndpointsListLike(EndpointsList item);
public KubeSchemaFluent.EndpointsListNested editEndpointsList();
public KubeSchemaFluent.EndpointsListNested editOrNewEndpointsList();
public KubeSchemaFluent.EndpointsListNested editOrNewEndpointsListLike(EndpointsList item);
/**
* This method has been deprecated, please use method buildEnvVar instead.
* @return The buildable object.
*/
@Deprecated public EnvVar getEnvVar();
public EnvVar buildEnvVar();
public A withEnvVar(EnvVar envVar);
public Boolean hasEnvVar();
public KubeSchemaFluent.EnvVarNested withNewEnvVar();
public KubeSchemaFluent.EnvVarNested withNewEnvVarLike(EnvVar item);
public KubeSchemaFluent.EnvVarNested editEnvVar();
public KubeSchemaFluent.EnvVarNested editOrNewEnvVar();
public KubeSchemaFluent.EnvVarNested editOrNewEnvVarLike(EnvVar item);
/**
* This method has been deprecated, please use method buildEvent instead.
* @return The buildable object.
*/
@Deprecated public Event getEvent();
public Event buildEvent();
public A withEvent(Event event);
public Boolean hasEvent();
public KubeSchemaFluent.EventNested withNewEvent();
public KubeSchemaFluent.EventNested withNewEventLike(Event item);
public KubeSchemaFluent.EventNested editEvent();
public KubeSchemaFluent.EventNested editOrNewEvent();
public KubeSchemaFluent.EventNested editOrNewEventLike(Event item);
/**
* This method has been deprecated, please use method buildEventList instead.
* @return The buildable object.
*/
@Deprecated public EventList getEventList();
public EventList buildEventList();
public A withEventList(EventList eventList);
public Boolean hasEventList();
public KubeSchemaFluent.EventListNested withNewEventList();
public KubeSchemaFluent.EventListNested withNewEventListLike(EventList item);
public KubeSchemaFluent.EventListNested editEventList();
public KubeSchemaFluent.EventListNested editOrNewEventList();
public KubeSchemaFluent.EventListNested editOrNewEventListLike(EventList item);
/**
* This method has been deprecated, please use method buildEventSeries instead.
* @return The buildable object.
*/
@Deprecated public EventSeries getEventSeries();
public EventSeries buildEventSeries();
public A withEventSeries(EventSeries eventSeries);
public Boolean hasEventSeries();
public KubeSchemaFluent.EventSeriesNested withNewEventSeries();
public KubeSchemaFluent.EventSeriesNested withNewEventSeriesLike(EventSeries item);
public KubeSchemaFluent.EventSeriesNested editEventSeries();
public KubeSchemaFluent.EventSeriesNested editOrNewEventSeries();
public KubeSchemaFluent.EventSeriesNested editOrNewEventSeriesLike(EventSeries item);
/**
* This method has been deprecated, please use method buildEventSource instead.
* @return The buildable object.
*/
@Deprecated public EventSource getEventSource();
public EventSource buildEventSource();
public A withEventSource(EventSource eventSource);
public Boolean hasEventSource();
public A withNewEventSource(String component,String host);
public KubeSchemaFluent.EventSourceNested withNewEventSource();
public KubeSchemaFluent.EventSourceNested withNewEventSourceLike(EventSource item);
public KubeSchemaFluent.EventSourceNested editEventSource();
public KubeSchemaFluent.EventSourceNested editOrNewEventSource();
public KubeSchemaFluent.EventSourceNested editOrNewEventSourceLike(EventSource item);
/**
* This method has been deprecated, please use method buildGetOptions instead.
* @return The buildable object.
*/
@Deprecated public GetOptions getGetOptions();
public GetOptions buildGetOptions();
public A withGetOptions(GetOptions getOptions);
public Boolean hasGetOptions();
public A withNewGetOptions(String apiVersion,String kind,String resourceVersion);
public KubeSchemaFluent.GetOptionsNested withNewGetOptions();
public KubeSchemaFluent.GetOptionsNested withNewGetOptionsLike(GetOptions item);
public KubeSchemaFluent.GetOptionsNested editGetOptions();
public KubeSchemaFluent.GetOptionsNested editOrNewGetOptions();
public KubeSchemaFluent.GetOptionsNested editOrNewGetOptionsLike(GetOptions item);
/**
* This method has been deprecated, please use method buildGroupVersionKind instead.
* @return The buildable object.
*/
@Deprecated public GroupVersionKind getGroupVersionKind();
public GroupVersionKind buildGroupVersionKind();
public A withGroupVersionKind(GroupVersionKind groupVersionKind);
public Boolean hasGroupVersionKind();
public A withNewGroupVersionKind(String group,String kind,String version);
public KubeSchemaFluent.GroupVersionKindNested withNewGroupVersionKind();
public KubeSchemaFluent.GroupVersionKindNested withNewGroupVersionKindLike(GroupVersionKind item);
public KubeSchemaFluent.GroupVersionKindNested editGroupVersionKind();
public KubeSchemaFluent.GroupVersionKindNested editOrNewGroupVersionKind();
public KubeSchemaFluent.GroupVersionKindNested editOrNewGroupVersionKindLike(GroupVersionKind item);
/**
* This method has been deprecated, please use method buildGroupVersionResource instead.
* @return The buildable object.
*/
@Deprecated public GroupVersionResource getGroupVersionResource();
public GroupVersionResource buildGroupVersionResource();
public A withGroupVersionResource(GroupVersionResource groupVersionResource);
public Boolean hasGroupVersionResource();
public A withNewGroupVersionResource(String group,String resource,String version);
public KubeSchemaFluent.GroupVersionResourceNested withNewGroupVersionResource();
public KubeSchemaFluent.GroupVersionResourceNested withNewGroupVersionResourceLike(GroupVersionResource item);
public KubeSchemaFluent.GroupVersionResourceNested editGroupVersionResource();
public KubeSchemaFluent.GroupVersionResourceNested editOrNewGroupVersionResource();
public KubeSchemaFluent.GroupVersionResourceNested editOrNewGroupVersionResourceLike(GroupVersionResource item);
/**
* This method has been deprecated, please use method buildInfo instead.
* @return The buildable object.
*/
@Deprecated public Info getInfo();
public Info buildInfo();
public A withInfo(Info info);
public Boolean hasInfo();
public KubeSchemaFluent.InfoNested withNewInfo();
public KubeSchemaFluent.InfoNested withNewInfoLike(Info item);
public KubeSchemaFluent.InfoNested editInfo();
public KubeSchemaFluent.InfoNested editOrNewInfo();
public KubeSchemaFluent.InfoNested editOrNewInfoLike(Info item);
/**
* This method has been deprecated, please use method buildLimitRangeList instead.
* @return The buildable object.
*/
@Deprecated public LimitRangeList getLimitRangeList();
public LimitRangeList buildLimitRangeList();
public A withLimitRangeList(LimitRangeList limitRangeList);
public Boolean hasLimitRangeList();
public KubeSchemaFluent.LimitRangeListNested withNewLimitRangeList();
public KubeSchemaFluent.LimitRangeListNested withNewLimitRangeListLike(LimitRangeList item);
public KubeSchemaFluent.LimitRangeListNested editLimitRangeList();
public KubeSchemaFluent.LimitRangeListNested editOrNewLimitRangeList();
public KubeSchemaFluent.LimitRangeListNested editOrNewLimitRangeListLike(LimitRangeList item);
/**
* This method has been deprecated, please use method buildListOptions instead.
* @return The buildable object.
*/
@Deprecated public ListOptions getListOptions();
public ListOptions buildListOptions();
public A withListOptions(ListOptions listOptions);
public Boolean hasListOptions();
public KubeSchemaFluent.ListOptionsNested withNewListOptions();
public KubeSchemaFluent.ListOptionsNested withNewListOptionsLike(ListOptions item);
public KubeSchemaFluent.ListOptionsNested editListOptions();
public KubeSchemaFluent.ListOptionsNested editOrNewListOptions();
public KubeSchemaFluent.ListOptionsNested editOrNewListOptionsLike(ListOptions item);
/**
* This method has been deprecated, please use method buildMicroTime instead.
* @return The buildable object.
*/
@Deprecated public MicroTime getMicroTime();
public MicroTime buildMicroTime();
public A withMicroTime(MicroTime microTime);
public Boolean hasMicroTime();
public A withNewMicroTime(String time);
public KubeSchemaFluent.MicroTimeNested withNewMicroTime();
public KubeSchemaFluent.MicroTimeNested withNewMicroTimeLike(MicroTime item);
public KubeSchemaFluent.MicroTimeNested editMicroTime();
public KubeSchemaFluent.MicroTimeNested editOrNewMicroTime();
public KubeSchemaFluent.MicroTimeNested editOrNewMicroTimeLike(MicroTime item);
/**
* This method has been deprecated, please use method buildNamespace instead.
* @return The buildable object.
*/
@Deprecated public Namespace getNamespace();
public Namespace buildNamespace();
public A withNamespace(Namespace namespace);
public Boolean hasNamespace();
public KubeSchemaFluent.NamespaceNested withNewNamespace();
public KubeSchemaFluent.NamespaceNested withNewNamespaceLike(Namespace item);
public KubeSchemaFluent.NamespaceNested editNamespace();
public KubeSchemaFluent.NamespaceNested editOrNewNamespace();
public KubeSchemaFluent.NamespaceNested editOrNewNamespaceLike(Namespace item);
/**
* This method has been deprecated, please use method buildNamespaceList instead.
* @return The buildable object.
*/
@Deprecated public NamespaceList getNamespaceList();
public NamespaceList buildNamespaceList();
public A withNamespaceList(NamespaceList namespaceList);
public Boolean hasNamespaceList();
public KubeSchemaFluent.NamespaceListNested withNewNamespaceList();
public KubeSchemaFluent.NamespaceListNested withNewNamespaceListLike(NamespaceList item);
public KubeSchemaFluent.NamespaceListNested editNamespaceList();
public KubeSchemaFluent.NamespaceListNested editOrNewNamespaceList();
public KubeSchemaFluent.NamespaceListNested editOrNewNamespaceListLike(NamespaceList item);
/**
* This method has been deprecated, please use method buildNode instead.
* @return The buildable object.
*/
@Deprecated public Node getNode();
public Node buildNode();
public A withNode(Node node);
public Boolean hasNode();
public KubeSchemaFluent.NodeNested withNewNode();
public KubeSchemaFluent.NodeNested withNewNodeLike(Node item);
public KubeSchemaFluent.NodeNested editNode();
public KubeSchemaFluent.NodeNested editOrNewNode();
public KubeSchemaFluent.NodeNested editOrNewNodeLike(Node item);
/**
* This method has been deprecated, please use method buildNodeList instead.
* @return The buildable object.
*/
@Deprecated public NodeList getNodeList();
public NodeList buildNodeList();
public A withNodeList(NodeList nodeList);
public Boolean hasNodeList();
public KubeSchemaFluent.NodeListNested withNewNodeList();
public KubeSchemaFluent.NodeListNested withNewNodeListLike(NodeList item);
public KubeSchemaFluent.NodeListNested editNodeList();
public KubeSchemaFluent.NodeListNested editOrNewNodeList();
public KubeSchemaFluent.NodeListNested editOrNewNodeListLike(NodeList item);
/**
* This method has been deprecated, please use method buildObjectMeta instead.
* @return The buildable object.
*/
@Deprecated public ObjectMeta getObjectMeta();
public ObjectMeta buildObjectMeta();
public A withObjectMeta(ObjectMeta objectMeta);
public Boolean hasObjectMeta();
public KubeSchemaFluent.ObjectMetaNested withNewObjectMeta();
public KubeSchemaFluent.ObjectMetaNested withNewObjectMetaLike(ObjectMeta item);
public KubeSchemaFluent.ObjectMetaNested editObjectMeta();
public KubeSchemaFluent.ObjectMetaNested editOrNewObjectMeta();
public KubeSchemaFluent.ObjectMetaNested editOrNewObjectMetaLike(ObjectMeta item);
/**
* This method has been deprecated, please use method buildPatch instead.
* @return The buildable object.
*/
@Deprecated public Patch getPatch();
public Patch buildPatch();
public A withPatch(Patch patch);
public Boolean hasPatch();
public KubeSchemaFluent.PatchNested withNewPatch();
public KubeSchemaFluent.PatchNested withNewPatchLike(Patch item);
public KubeSchemaFluent.PatchNested editPatch();
public KubeSchemaFluent.PatchNested editOrNewPatch();
public KubeSchemaFluent.PatchNested editOrNewPatchLike(Patch item);
/**
* This method has been deprecated, please use method buildPatchOptions instead.
* @return The buildable object.
*/
@Deprecated public PatchOptions getPatchOptions();
public PatchOptions buildPatchOptions();
public A withPatchOptions(PatchOptions patchOptions);
public Boolean hasPatchOptions();
public KubeSchemaFluent.PatchOptionsNested withNewPatchOptions();
public KubeSchemaFluent.PatchOptionsNested withNewPatchOptionsLike(PatchOptions item);
public KubeSchemaFluent.PatchOptionsNested editPatchOptions();
public KubeSchemaFluent.PatchOptionsNested editOrNewPatchOptions();
public KubeSchemaFluent.PatchOptionsNested editOrNewPatchOptionsLike(PatchOptions item);
/**
* This method has been deprecated, please use method buildPersistentVolume instead.
* @return The buildable object.
*/
@Deprecated public PersistentVolume getPersistentVolume();
public PersistentVolume buildPersistentVolume();
public A withPersistentVolume(PersistentVolume persistentVolume);
public Boolean hasPersistentVolume();
public KubeSchemaFluent.PersistentVolumeNested withNewPersistentVolume();
public KubeSchemaFluent.PersistentVolumeNested withNewPersistentVolumeLike(PersistentVolume item);
public KubeSchemaFluent.PersistentVolumeNested editPersistentVolume();
public KubeSchemaFluent.PersistentVolumeNested editOrNewPersistentVolume();
public KubeSchemaFluent.PersistentVolumeNested editOrNewPersistentVolumeLike(PersistentVolume item);
/**
* This method has been deprecated, please use method buildPersistentVolumeClaim instead.
* @return The buildable object.
*/
@Deprecated public PersistentVolumeClaim getPersistentVolumeClaim();
public PersistentVolumeClaim buildPersistentVolumeClaim();
public A withPersistentVolumeClaim(PersistentVolumeClaim persistentVolumeClaim);
public Boolean hasPersistentVolumeClaim();
public KubeSchemaFluent.PersistentVolumeClaimNested withNewPersistentVolumeClaim();
public KubeSchemaFluent.PersistentVolumeClaimNested withNewPersistentVolumeClaimLike(PersistentVolumeClaim item);
public KubeSchemaFluent.PersistentVolumeClaimNested editPersistentVolumeClaim();
public KubeSchemaFluent.PersistentVolumeClaimNested editOrNewPersistentVolumeClaim();
public KubeSchemaFluent.PersistentVolumeClaimNested editOrNewPersistentVolumeClaimLike(PersistentVolumeClaim item);
/**
* This method has been deprecated, please use method buildPersistentVolumeClaimList instead.
* @return The buildable object.
*/
@Deprecated public PersistentVolumeClaimList getPersistentVolumeClaimList();
public PersistentVolumeClaimList buildPersistentVolumeClaimList();
public A withPersistentVolumeClaimList(PersistentVolumeClaimList persistentVolumeClaimList);
public Boolean hasPersistentVolumeClaimList();
public KubeSchemaFluent.PersistentVolumeClaimListNested withNewPersistentVolumeClaimList();
public KubeSchemaFluent.PersistentVolumeClaimListNested withNewPersistentVolumeClaimListLike(PersistentVolumeClaimList item);
public KubeSchemaFluent.PersistentVolumeClaimListNested editPersistentVolumeClaimList();
public KubeSchemaFluent.PersistentVolumeClaimListNested editOrNewPersistentVolumeClaimList();
public KubeSchemaFluent.PersistentVolumeClaimListNested editOrNewPersistentVolumeClaimListLike(PersistentVolumeClaimList item);
/**
* This method has been deprecated, please use method buildPersistentVolumeList instead.
* @return The buildable object.
*/
@Deprecated public PersistentVolumeList getPersistentVolumeList();
public PersistentVolumeList buildPersistentVolumeList();
public A withPersistentVolumeList(PersistentVolumeList persistentVolumeList);
public Boolean hasPersistentVolumeList();
public KubeSchemaFluent.PersistentVolumeListNested withNewPersistentVolumeList();
public KubeSchemaFluent.PersistentVolumeListNested withNewPersistentVolumeListLike(PersistentVolumeList item);
public KubeSchemaFluent.PersistentVolumeListNested editPersistentVolumeList();
public KubeSchemaFluent.PersistentVolumeListNested editOrNewPersistentVolumeList();
public KubeSchemaFluent.PersistentVolumeListNested editOrNewPersistentVolumeListLike(PersistentVolumeList item);
/**
* This method has been deprecated, please use method buildPodList instead.
* @return The buildable object.
*/
@Deprecated public PodList getPodList();
public PodList buildPodList();
public A withPodList(PodList podList);
public Boolean hasPodList();
public KubeSchemaFluent.PodListNested withNewPodList();
public KubeSchemaFluent.PodListNested withNewPodListLike(PodList item);
public KubeSchemaFluent.PodListNested editPodList();
public KubeSchemaFluent.PodListNested editOrNewPodList();
public KubeSchemaFluent.PodListNested editOrNewPodListLike(PodList item);
/**
* This method has been deprecated, please use method buildPodTemplateList instead.
* @return The buildable object.
*/
@Deprecated public PodTemplateList getPodTemplateList();
public PodTemplateList buildPodTemplateList();
public A withPodTemplateList(PodTemplateList podTemplateList);
public Boolean hasPodTemplateList();
public KubeSchemaFluent.PodTemplateListNested withNewPodTemplateList();
public KubeSchemaFluent.PodTemplateListNested withNewPodTemplateListLike(PodTemplateList item);
public KubeSchemaFluent.PodTemplateListNested editPodTemplateList();
public KubeSchemaFluent.PodTemplateListNested editOrNewPodTemplateList();
public KubeSchemaFluent.PodTemplateListNested editOrNewPodTemplateListLike(PodTemplateList item);
/**
* This method has been deprecated, please use method buildQuantity instead.
* @return The buildable object.
*/
@Deprecated public Quantity getQuantity();
public Quantity buildQuantity();
public A withQuantity(Quantity quantity);
public Boolean hasQuantity();
public A withNewQuantity(String amount,String format);
public A withNewQuantity(String amount);
public KubeSchemaFluent.QuantityNested withNewQuantity();
public KubeSchemaFluent.QuantityNested withNewQuantityLike(Quantity item);
public KubeSchemaFluent.QuantityNested editQuantity();
public KubeSchemaFluent.QuantityNested editOrNewQuantity();
public KubeSchemaFluent.QuantityNested editOrNewQuantityLike(Quantity item);
/**
* This method has been deprecated, please use method buildReplicationControllerList instead.
* @return The buildable object.
*/
@Deprecated public ReplicationControllerList getReplicationControllerList();
public ReplicationControllerList buildReplicationControllerList();
public A withReplicationControllerList(ReplicationControllerList replicationControllerList);
public Boolean hasReplicationControllerList();
public KubeSchemaFluent.ReplicationControllerListNested withNewReplicationControllerList();
public KubeSchemaFluent.ReplicationControllerListNested withNewReplicationControllerListLike(ReplicationControllerList item);
public KubeSchemaFluent.ReplicationControllerListNested editReplicationControllerList();
public KubeSchemaFluent.ReplicationControllerListNested editOrNewReplicationControllerList();
public KubeSchemaFluent.ReplicationControllerListNested editOrNewReplicationControllerListLike(ReplicationControllerList item);
/**
* This method has been deprecated, please use method buildResourceQuota instead.
* @return The buildable object.
*/
@Deprecated public ResourceQuota getResourceQuota();
public ResourceQuota buildResourceQuota();
public A withResourceQuota(ResourceQuota resourceQuota);
public Boolean hasResourceQuota();
public KubeSchemaFluent.ResourceQuotaNested withNewResourceQuota();
public KubeSchemaFluent.ResourceQuotaNested withNewResourceQuotaLike(ResourceQuota item);
public KubeSchemaFluent.ResourceQuotaNested editResourceQuota();
public KubeSchemaFluent.ResourceQuotaNested editOrNewResourceQuota();
public KubeSchemaFluent.ResourceQuotaNested editOrNewResourceQuotaLike(ResourceQuota item);
/**
* This method has been deprecated, please use method buildResourceQuotaList instead.
* @return The buildable object.
*/
@Deprecated public ResourceQuotaList getResourceQuotaList();
public ResourceQuotaList buildResourceQuotaList();
public A withResourceQuotaList(ResourceQuotaList resourceQuotaList);
public Boolean hasResourceQuotaList();
public KubeSchemaFluent.ResourceQuotaListNested withNewResourceQuotaList();
public KubeSchemaFluent.ResourceQuotaListNested withNewResourceQuotaListLike(ResourceQuotaList item);
public KubeSchemaFluent.ResourceQuotaListNested editResourceQuotaList();
public KubeSchemaFluent.ResourceQuotaListNested editOrNewResourceQuotaList();
public KubeSchemaFluent.ResourceQuotaListNested editOrNewResourceQuotaListLike(ResourceQuotaList item);
/**
* This method has been deprecated, please use method buildRootPaths instead.
* @return The buildable object.
*/
@Deprecated public RootPaths getRootPaths();
public RootPaths buildRootPaths();
public A withRootPaths(RootPaths rootPaths);
public Boolean hasRootPaths();
public KubeSchemaFluent.RootPathsNested withNewRootPaths();
public KubeSchemaFluent.RootPathsNested withNewRootPathsLike(RootPaths item);
public KubeSchemaFluent.RootPathsNested editRootPaths();
public KubeSchemaFluent.RootPathsNested editOrNewRootPaths();
public KubeSchemaFluent.RootPathsNested editOrNewRootPathsLike(RootPaths item);
/**
* This method has been deprecated, please use method buildSecret instead.
* @return The buildable object.
*/
@Deprecated public Secret getSecret();
public Secret buildSecret();
public A withSecret(Secret secret);
public Boolean hasSecret();
public KubeSchemaFluent.SecretNested withNewSecret();
public KubeSchemaFluent.SecretNested withNewSecretLike(Secret item);
public KubeSchemaFluent.SecretNested editSecret();
public KubeSchemaFluent.SecretNested editOrNewSecret();
public KubeSchemaFluent.SecretNested editOrNewSecretLike(Secret item);
/**
* This method has been deprecated, please use method buildSecretList instead.
* @return The buildable object.
*/
@Deprecated public SecretList getSecretList();
public SecretList buildSecretList();
public A withSecretList(SecretList secretList);
public Boolean hasSecretList();
public KubeSchemaFluent.SecretListNested withNewSecretList();
public KubeSchemaFluent.SecretListNested withNewSecretListLike(SecretList item);
public KubeSchemaFluent.SecretListNested editSecretList();
public KubeSchemaFluent.SecretListNested editOrNewSecretList();
public KubeSchemaFluent.SecretListNested editOrNewSecretListLike(SecretList item);
/**
* This method has been deprecated, please use method buildServiceAccount instead.
* @return The buildable object.
*/
@Deprecated public ServiceAccount getServiceAccount();
public ServiceAccount buildServiceAccount();
public A withServiceAccount(ServiceAccount serviceAccount);
public Boolean hasServiceAccount();
public KubeSchemaFluent.ServiceAccountNested withNewServiceAccount();
public KubeSchemaFluent.ServiceAccountNested withNewServiceAccountLike(ServiceAccount item);
public KubeSchemaFluent.ServiceAccountNested editServiceAccount();
public KubeSchemaFluent.ServiceAccountNested editOrNewServiceAccount();
public KubeSchemaFluent.ServiceAccountNested editOrNewServiceAccountLike(ServiceAccount item);
/**
* This method has been deprecated, please use method buildServiceAccountList instead.
* @return The buildable object.
*/
@Deprecated public ServiceAccountList getServiceAccountList();
public ServiceAccountList buildServiceAccountList();
public A withServiceAccountList(ServiceAccountList serviceAccountList);
public Boolean hasServiceAccountList();
public KubeSchemaFluent.ServiceAccountListNested withNewServiceAccountList();
public KubeSchemaFluent.ServiceAccountListNested withNewServiceAccountListLike(ServiceAccountList item);
public KubeSchemaFluent.ServiceAccountListNested editServiceAccountList();
public KubeSchemaFluent.ServiceAccountListNested editOrNewServiceAccountList();
public KubeSchemaFluent.ServiceAccountListNested editOrNewServiceAccountListLike(ServiceAccountList item);
/**
* This method has been deprecated, please use method buildServiceList instead.
* @return The buildable object.
*/
@Deprecated public ServiceList getServiceList();
public ServiceList buildServiceList();
public A withServiceList(ServiceList serviceList);
public Boolean hasServiceList();
public KubeSchemaFluent.ServiceListNested withNewServiceList();
public KubeSchemaFluent.ServiceListNested withNewServiceListLike(ServiceList item);
public KubeSchemaFluent.ServiceListNested editServiceList();
public KubeSchemaFluent.ServiceListNested editOrNewServiceList();
public KubeSchemaFluent.ServiceListNested editOrNewServiceListLike(ServiceList item);
/**
* This method has been deprecated, please use method buildStatus instead.
* @return The buildable object.
*/
@Deprecated public Status getStatus();
public Status buildStatus();
public A withStatus(Status status);
public Boolean hasStatus();
public KubeSchemaFluent.StatusNested withNewStatus();
public KubeSchemaFluent.StatusNested withNewStatusLike(Status item);
public KubeSchemaFluent.StatusNested editStatus();
public KubeSchemaFluent.StatusNested editOrNewStatus();
public KubeSchemaFluent.StatusNested editOrNewStatusLike(Status item);
public String getTime();
public A withTime(String time);
public Boolean hasTime();
public A withNewTime(String arg1);
public A withNewTime(StringBuilder arg1);
public A withNewTime(StringBuffer arg1);
/**
* This method has been deprecated, please use method buildToleration instead.
* @return The buildable object.
*/
@Deprecated public Toleration getToleration();
public Toleration buildToleration();
public A withToleration(Toleration toleration);
public Boolean hasToleration();
public A withNewToleration(String effect,String key,String operator,Long tolerationSeconds,String value);
public KubeSchemaFluent.TolerationNested withNewToleration();
public KubeSchemaFluent.TolerationNested withNewTolerationLike(Toleration item);
public KubeSchemaFluent.TolerationNested editToleration();
public KubeSchemaFluent.TolerationNested editOrNewToleration();
public KubeSchemaFluent.TolerationNested editOrNewTolerationLike(Toleration item);
/**
* This method has been deprecated, please use method buildTopologySelectorTerm instead.
* @return The buildable object.
*/
@Deprecated public TopologySelectorTerm getTopologySelectorTerm();
public TopologySelectorTerm buildTopologySelectorTerm();
public A withTopologySelectorTerm(TopologySelectorTerm topologySelectorTerm);
public Boolean hasTopologySelectorTerm();
public KubeSchemaFluent.TopologySelectorTermNested withNewTopologySelectorTerm();
public KubeSchemaFluent.TopologySelectorTermNested withNewTopologySelectorTermLike(TopologySelectorTerm item);
public KubeSchemaFluent.TopologySelectorTermNested editTopologySelectorTerm();
public KubeSchemaFluent.TopologySelectorTermNested editOrNewTopologySelectorTerm();
public KubeSchemaFluent.TopologySelectorTermNested editOrNewTopologySelectorTermLike(TopologySelectorTerm item);
/**
* This method has been deprecated, please use method buildTypeMeta instead.
* @return The buildable object.
*/
@Deprecated public TypeMeta getTypeMeta();
public TypeMeta buildTypeMeta();
public A withTypeMeta(TypeMeta typeMeta);
public Boolean hasTypeMeta();
public A withNewTypeMeta(String apiVersion,String kind);
public KubeSchemaFluent.TypeMetaNested withNewTypeMeta();
public KubeSchemaFluent.TypeMetaNested withNewTypeMetaLike(TypeMeta item);
public KubeSchemaFluent.TypeMetaNested editTypeMeta();
public KubeSchemaFluent.TypeMetaNested editOrNewTypeMeta();
public KubeSchemaFluent.TypeMetaNested editOrNewTypeMetaLike(TypeMeta item);
/**
* This method has been deprecated, please use method buildUpdateOptions instead.
* @return The buildable object.
*/
@Deprecated public UpdateOptions getUpdateOptions();
public UpdateOptions buildUpdateOptions();
public A withUpdateOptions(UpdateOptions updateOptions);
public Boolean hasUpdateOptions();
public KubeSchemaFluent.UpdateOptionsNested withNewUpdateOptions();
public KubeSchemaFluent.UpdateOptionsNested withNewUpdateOptionsLike(UpdateOptions item);
public KubeSchemaFluent.UpdateOptionsNested editUpdateOptions();
public KubeSchemaFluent.UpdateOptionsNested editOrNewUpdateOptions();
public KubeSchemaFluent.UpdateOptionsNested editOrNewUpdateOptionsLike(UpdateOptions item);
/**
* This method has been deprecated, please use method buildWatchEvent instead.
* @return The buildable object.
*/
@Deprecated public WatchEvent getWatchEvent();
public WatchEvent buildWatchEvent();
public A withWatchEvent(WatchEvent watchEvent);
public Boolean hasWatchEvent();
public KubeSchemaFluent.WatchEventNested withNewWatchEvent();
public KubeSchemaFluent.WatchEventNested withNewWatchEventLike(WatchEvent item);
public KubeSchemaFluent.WatchEventNested editWatchEvent();
public KubeSchemaFluent.WatchEventNested editOrNewWatchEvent();
public KubeSchemaFluent.WatchEventNested editOrNewWatchEventLike(WatchEvent item);
public interface APIGroupNested extends io.fabric8.kubernetes.api.builder.Nested,APIGroupFluent>{
public N and(); public N endAPIGroup();
}
public interface APIGroupListNested extends io.fabric8.kubernetes.api.builder.Nested,APIGroupListFluent>{
public N and(); public N endAPIGroupList();
}
public interface BaseKubernetesListNested extends io.fabric8.kubernetes.api.builder.Nested,BaseKubernetesListFluent>{
public N and(); public N endBaseKubernetesList();
}
public interface BindingNested extends io.fabric8.kubernetes.api.builder.Nested,BindingFluent>{
public N and(); public N endBinding();
}
public interface ComponentStatusNested extends io.fabric8.kubernetes.api.builder.Nested,ComponentStatusFluent>{
public N and(); public N endComponentStatus();
}
public interface ComponentStatusListNested extends io.fabric8.kubernetes.api.builder.Nested,ComponentStatusListFluent>{
public N and(); public N endComponentStatusList();
}
public interface ConfigNested extends io.fabric8.kubernetes.api.builder.Nested,ConfigFluent>{
public N and(); public N endConfig();
}
public interface ConfigMapNested extends io.fabric8.kubernetes.api.builder.Nested,ConfigMapFluent>{
public N and(); public N endConfigMap();
}
public interface ConfigMapListNested extends io.fabric8.kubernetes.api.builder.Nested,ConfigMapListFluent>{
public N and(); public N endConfigMapList();
}
public interface ContainerStatusNested extends io.fabric8.kubernetes.api.builder.Nested,ContainerStatusFluent>{
public N and(); public N endContainerStatus();
}
public interface CreateOptionsNested extends io.fabric8.kubernetes.api.builder.Nested,CreateOptionsFluent>{
public N and(); public N endCreateOptions();
}
public interface DeleteOptionsNested extends io.fabric8.kubernetes.api.builder.Nested,DeleteOptionsFluent>{
public N and(); public N endDeleteOptions();
}
public interface EndpointPortNested extends io.fabric8.kubernetes.api.builder.Nested,EndpointPortFluent>{
public N and(); public N endEndpointPort();
}
public interface EndpointsNested extends io.fabric8.kubernetes.api.builder.Nested,EndpointsFluent>{
public N and(); public N endEndpoints();
}
public interface EndpointsListNested extends io.fabric8.kubernetes.api.builder.Nested,EndpointsListFluent>{
public N and(); public N endEndpointsList();
}
public interface EnvVarNested extends io.fabric8.kubernetes.api.builder.Nested,EnvVarFluent>{
public N and(); public N endEnvVar();
}
public interface EventNested extends io.fabric8.kubernetes.api.builder.Nested,EventFluent>{
public N and(); public N endEvent();
}
public interface EventListNested extends io.fabric8.kubernetes.api.builder.Nested,EventListFluent>{
public N and(); public N endEventList();
}
public interface EventSeriesNested extends io.fabric8.kubernetes.api.builder.Nested,EventSeriesFluent>{
public N and(); public N endEventSeries();
}
public interface EventSourceNested extends io.fabric8.kubernetes.api.builder.Nested,EventSourceFluent>{
public N and(); public N endEventSource();
}
public interface GetOptionsNested extends io.fabric8.kubernetes.api.builder.Nested,GetOptionsFluent>{
public N and(); public N endGetOptions();
}
public interface GroupVersionKindNested extends io.fabric8.kubernetes.api.builder.Nested,GroupVersionKindFluent>{
public N and(); public N endGroupVersionKind();
}
public interface GroupVersionResourceNested extends io.fabric8.kubernetes.api.builder.Nested,GroupVersionResourceFluent>{
public N and(); public N endGroupVersionResource();
}
public interface InfoNested extends io.fabric8.kubernetes.api.builder.Nested,InfoFluent>{
public N and(); public N endInfo();
}
public interface LimitRangeListNested extends io.fabric8.kubernetes.api.builder.Nested,LimitRangeListFluent>{
public N and(); public N endLimitRangeList();
}
public interface ListOptionsNested extends io.fabric8.kubernetes.api.builder.Nested,ListOptionsFluent>{
public N and(); public N endListOptions();
}
public interface MicroTimeNested extends io.fabric8.kubernetes.api.builder.Nested,MicroTimeFluent>{
public N and(); public N endMicroTime();
}
public interface NamespaceNested extends io.fabric8.kubernetes.api.builder.Nested,NamespaceFluent>{
public N and(); public N endNamespace();
}
public interface NamespaceListNested extends io.fabric8.kubernetes.api.builder.Nested,NamespaceListFluent>{
public N and(); public N endNamespaceList();
}
public interface NodeNested extends io.fabric8.kubernetes.api.builder.Nested,NodeFluent>{
public N and(); public N endNode();
}
public interface NodeListNested extends io.fabric8.kubernetes.api.builder.Nested,NodeListFluent>{
public N and(); public N endNodeList();
}
public interface ObjectMetaNested extends io.fabric8.kubernetes.api.builder.Nested,ObjectMetaFluent>{
public N and(); public N endObjectMeta();
}
public interface PatchNested extends io.fabric8.kubernetes.api.builder.Nested,PatchFluent>{
public N and(); public N endPatch();
}
public interface PatchOptionsNested extends io.fabric8.kubernetes.api.builder.Nested,PatchOptionsFluent>{
public N and(); public N endPatchOptions();
}
public interface PersistentVolumeNested extends io.fabric8.kubernetes.api.builder.Nested,PersistentVolumeFluent>{
public N and(); public N endPersistentVolume();
}
public interface PersistentVolumeClaimNested extends io.fabric8.kubernetes.api.builder.Nested,PersistentVolumeClaimFluent>{
public N and(); public N endPersistentVolumeClaim();
}
public interface PersistentVolumeClaimListNested extends io.fabric8.kubernetes.api.builder.Nested,PersistentVolumeClaimListFluent>{
public N and(); public N endPersistentVolumeClaimList();
}
public interface PersistentVolumeListNested extends io.fabric8.kubernetes.api.builder.Nested,PersistentVolumeListFluent>{
public N and(); public N endPersistentVolumeList();
}
public interface PodListNested extends io.fabric8.kubernetes.api.builder.Nested,PodListFluent>{
public N and(); public N endPodList();
}
public interface PodTemplateListNested extends io.fabric8.kubernetes.api.builder.Nested,PodTemplateListFluent>{
public N and(); public N endPodTemplateList();
}
public interface QuantityNested extends io.fabric8.kubernetes.api.builder.Nested,QuantityFluent>{
public N and(); public N endQuantity();
}
public interface ReplicationControllerListNested extends io.fabric8.kubernetes.api.builder.Nested,ReplicationControllerListFluent>{
public N and(); public N endReplicationControllerList();
}
public interface ResourceQuotaNested extends io.fabric8.kubernetes.api.builder.Nested,ResourceQuotaFluent>{
public N and(); public N endResourceQuota();
}
public interface ResourceQuotaListNested extends io.fabric8.kubernetes.api.builder.Nested,ResourceQuotaListFluent>{
public N and(); public N endResourceQuotaList();
}
public interface RootPathsNested extends io.fabric8.kubernetes.api.builder.Nested,RootPathsFluent>{
public N and(); public N endRootPaths();
}
public interface SecretNested extends io.fabric8.kubernetes.api.builder.Nested,SecretFluent>{
public N and(); public N endSecret();
}
public interface SecretListNested extends io.fabric8.kubernetes.api.builder.Nested,SecretListFluent>{
public N and(); public N endSecretList();
}
public interface ServiceAccountNested extends io.fabric8.kubernetes.api.builder.Nested,ServiceAccountFluent>{
public N and(); public N endServiceAccount();
}
public interface ServiceAccountListNested extends io.fabric8.kubernetes.api.builder.Nested,ServiceAccountListFluent>{
public N and(); public N endServiceAccountList();
}
public interface ServiceListNested extends io.fabric8.kubernetes.api.builder.Nested,ServiceListFluent>{
public N and(); public N endServiceList();
}
public interface StatusNested extends io.fabric8.kubernetes.api.builder.Nested,StatusFluent>{
public N and(); public N endStatus();
}
public interface TolerationNested extends io.fabric8.kubernetes.api.builder.Nested,TolerationFluent>{
public N and(); public N endToleration();
}
public interface TopologySelectorTermNested extends io.fabric8.kubernetes.api.builder.Nested,TopologySelectorTermFluent>{
public N and(); public N endTopologySelectorTerm();
}
public interface TypeMetaNested extends io.fabric8.kubernetes.api.builder.Nested,TypeMetaFluent>{
public N and(); public N endTypeMeta();
}
public interface UpdateOptionsNested extends io.fabric8.kubernetes.api.builder.Nested,UpdateOptionsFluent>{
public N and(); public N endUpdateOptions();
}
public interface WatchEventNested extends io.fabric8.kubernetes.api.builder.Nested,WatchEventFluent>{
public N and(); public N endWatchEvent();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy