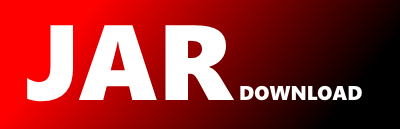
io.fabric8.kubernetes.api.model.extensions.IngressSpecFluent Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model.extensions;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.Nested;
import java.util.ArrayList;
import java.lang.String;
import java.util.LinkedHashMap;
import java.util.function.Predicate;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.Iterator;
import java.util.List;
import java.util.Collection;
import java.lang.Object;
import java.util.Map;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class IngressSpecFluent> extends BaseFluent{
public IngressSpecFluent() {
}
public IngressSpecFluent(IngressSpec instance) {
this.copyInstance(instance);
}
private IngressBackendBuilder backend;
private String ingressClassName;
private ArrayList rules = new ArrayList();
private ArrayList tls = new ArrayList();
private Map additionalProperties;
protected void copyInstance(IngressSpec instance) {
instance = (instance != null ? instance : new IngressSpec());
if (instance != null) {
this.withBackend(instance.getBackend());
this.withIngressClassName(instance.getIngressClassName());
this.withRules(instance.getRules());
this.withTls(instance.getTls());
this.withAdditionalProperties(instance.getAdditionalProperties());
}
}
public IngressBackend buildBackend() {
return this.backend != null ? this.backend.build() : null;
}
public A withBackend(IngressBackend backend) {
this._visitables.remove("backend");
if (backend != null) {
this.backend = new IngressBackendBuilder(backend);
this._visitables.get("backend").add(this.backend);
} else {
this.backend = null;
this._visitables.get("backend").remove(this.backend);
}
return (A) this;
}
public boolean hasBackend() {
return this.backend != null;
}
public BackendNested withNewBackend() {
return new BackendNested(null);
}
public BackendNested withNewBackendLike(IngressBackend item) {
return new BackendNested(item);
}
public BackendNested editBackend() {
return withNewBackendLike(java.util.Optional.ofNullable(buildBackend()).orElse(null));
}
public BackendNested editOrNewBackend() {
return withNewBackendLike(java.util.Optional.ofNullable(buildBackend()).orElse(new IngressBackendBuilder().build()));
}
public BackendNested editOrNewBackendLike(IngressBackend item) {
return withNewBackendLike(java.util.Optional.ofNullable(buildBackend()).orElse(item));
}
public String getIngressClassName() {
return this.ingressClassName;
}
public A withIngressClassName(String ingressClassName) {
this.ingressClassName = ingressClassName;
return (A) this;
}
public boolean hasIngressClassName() {
return this.ingressClassName != null;
}
public A addToRules(int index,IngressRule item) {
if (this.rules == null) {this.rules = new ArrayList();}
IngressRuleBuilder builder = new IngressRuleBuilder(item);
if (index < 0 || index >= rules.size()) { _visitables.get("rules").add(builder); rules.add(builder); } else { _visitables.get("rules").add(index, builder); rules.add(index, builder);}
return (A)this;
}
public A setToRules(int index,IngressRule item) {
if (this.rules == null) {this.rules = new ArrayList();}
IngressRuleBuilder builder = new IngressRuleBuilder(item);
if (index < 0 || index >= rules.size()) { _visitables.get("rules").add(builder); rules.add(builder); } else { _visitables.get("rules").set(index, builder); rules.set(index, builder);}
return (A)this;
}
public A addToRules(io.fabric8.kubernetes.api.model.extensions.IngressRule... items) {
if (this.rules == null) {this.rules = new ArrayList();}
for (IngressRule item : items) {IngressRuleBuilder builder = new IngressRuleBuilder(item);_visitables.get("rules").add(builder);this.rules.add(builder);} return (A)this;
}
public A addAllToRules(Collection items) {
if (this.rules == null) {this.rules = new ArrayList();}
for (IngressRule item : items) {IngressRuleBuilder builder = new IngressRuleBuilder(item);_visitables.get("rules").add(builder);this.rules.add(builder);} return (A)this;
}
public A removeFromRules(io.fabric8.kubernetes.api.model.extensions.IngressRule... items) {
if (this.rules == null) return (A)this;
for (IngressRule item : items) {IngressRuleBuilder builder = new IngressRuleBuilder(item);_visitables.get("rules").remove(builder); this.rules.remove(builder);} return (A)this;
}
public A removeAllFromRules(Collection items) {
if (this.rules == null) return (A)this;
for (IngressRule item : items) {IngressRuleBuilder builder = new IngressRuleBuilder(item);_visitables.get("rules").remove(builder); this.rules.remove(builder);} return (A)this;
}
public A removeMatchingFromRules(Predicate predicate) {
if (rules == null) return (A) this;
final Iterator each = rules.iterator();
final List visitables = _visitables.get("rules");
while (each.hasNext()) {
IngressRuleBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildRules() {
return this.rules != null ? build(rules) : null;
}
public IngressRule buildRule(int index) {
return this.rules.get(index).build();
}
public IngressRule buildFirstRule() {
return this.rules.get(0).build();
}
public IngressRule buildLastRule() {
return this.rules.get(rules.size() - 1).build();
}
public IngressRule buildMatchingRule(Predicate predicate) {
for (IngressRuleBuilder item : rules) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingRule(Predicate predicate) {
for (IngressRuleBuilder item : rules) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withRules(List rules) {
if (this.rules != null) {
this._visitables.get("rules").clear();
}
if (rules != null) {
this.rules = new ArrayList();
for (IngressRule item : rules) {
this.addToRules(item);
}
} else {
this.rules = null;
}
return (A) this;
}
public A withRules(io.fabric8.kubernetes.api.model.extensions.IngressRule... rules) {
if (this.rules != null) {
this.rules.clear();
_visitables.remove("rules");
}
if (rules != null) {
for (IngressRule item : rules) {
this.addToRules(item);
}
}
return (A) this;
}
public boolean hasRules() {
return this.rules != null && !this.rules.isEmpty();
}
public RulesNested addNewRule() {
return new RulesNested(-1, null);
}
public RulesNested addNewRuleLike(IngressRule item) {
return new RulesNested(-1, item);
}
public RulesNested setNewRuleLike(int index,IngressRule item) {
return new RulesNested(index, item);
}
public RulesNested editRule(int index) {
if (rules.size() <= index) throw new RuntimeException("Can't edit rules. Index exceeds size.");
return setNewRuleLike(index, buildRule(index));
}
public RulesNested editFirstRule() {
if (rules.size() == 0) throw new RuntimeException("Can't edit first rules. The list is empty.");
return setNewRuleLike(0, buildRule(0));
}
public RulesNested editLastRule() {
int index = rules.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last rules. The list is empty.");
return setNewRuleLike(index, buildRule(index));
}
public RulesNested editMatchingRule(Predicate predicate) {
int index = -1;
for (int i=0;i();}
IngressTLSBuilder builder = new IngressTLSBuilder(item);
if (index < 0 || index >= tls.size()) { _visitables.get("tls").add(builder); tls.add(builder); } else { _visitables.get("tls").add(index, builder); tls.add(index, builder);}
return (A)this;
}
public A setToTls(int index,IngressTLS item) {
if (this.tls == null) {this.tls = new ArrayList();}
IngressTLSBuilder builder = new IngressTLSBuilder(item);
if (index < 0 || index >= tls.size()) { _visitables.get("tls").add(builder); tls.add(builder); } else { _visitables.get("tls").set(index, builder); tls.set(index, builder);}
return (A)this;
}
public A addToTls(io.fabric8.kubernetes.api.model.extensions.IngressTLS... items) {
if (this.tls == null) {this.tls = new ArrayList();}
for (IngressTLS item : items) {IngressTLSBuilder builder = new IngressTLSBuilder(item);_visitables.get("tls").add(builder);this.tls.add(builder);} return (A)this;
}
public A addAllToTls(Collection items) {
if (this.tls == null) {this.tls = new ArrayList();}
for (IngressTLS item : items) {IngressTLSBuilder builder = new IngressTLSBuilder(item);_visitables.get("tls").add(builder);this.tls.add(builder);} return (A)this;
}
public A removeFromTls(io.fabric8.kubernetes.api.model.extensions.IngressTLS... items) {
if (this.tls == null) return (A)this;
for (IngressTLS item : items) {IngressTLSBuilder builder = new IngressTLSBuilder(item);_visitables.get("tls").remove(builder); this.tls.remove(builder);} return (A)this;
}
public A removeAllFromTls(Collection items) {
if (this.tls == null) return (A)this;
for (IngressTLS item : items) {IngressTLSBuilder builder = new IngressTLSBuilder(item);_visitables.get("tls").remove(builder); this.tls.remove(builder);} return (A)this;
}
public A removeMatchingFromTls(Predicate predicate) {
if (tls == null) return (A) this;
final Iterator each = tls.iterator();
final List visitables = _visitables.get("tls");
while (each.hasNext()) {
IngressTLSBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildTls() {
return this.tls != null ? build(tls) : null;
}
public IngressTLS buildTl(int index) {
return this.tls.get(index).build();
}
public IngressTLS buildFirstTl() {
return this.tls.get(0).build();
}
public IngressTLS buildLastTl() {
return this.tls.get(tls.size() - 1).build();
}
public IngressTLS buildMatchingTl(Predicate predicate) {
for (IngressTLSBuilder item : tls) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingTl(Predicate predicate) {
for (IngressTLSBuilder item : tls) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withTls(List tls) {
if (this.tls != null) {
this._visitables.get("tls").clear();
}
if (tls != null) {
this.tls = new ArrayList();
for (IngressTLS item : tls) {
this.addToTls(item);
}
} else {
this.tls = null;
}
return (A) this;
}
public A withTls(io.fabric8.kubernetes.api.model.extensions.IngressTLS... tls) {
if (this.tls != null) {
this.tls.clear();
_visitables.remove("tls");
}
if (tls != null) {
for (IngressTLS item : tls) {
this.addToTls(item);
}
}
return (A) this;
}
public boolean hasTls() {
return this.tls != null && !this.tls.isEmpty();
}
public TlsNested addNewTl() {
return new TlsNested(-1, null);
}
public TlsNested addNewTlLike(IngressTLS item) {
return new TlsNested(-1, item);
}
public TlsNested setNewTlLike(int index,IngressTLS item) {
return new TlsNested(index, item);
}
public TlsNested editTl(int index) {
if (tls.size() <= index) throw new RuntimeException("Can't edit tls. Index exceeds size.");
return setNewTlLike(index, buildTl(index));
}
public TlsNested editFirstTl() {
if (tls.size() == 0) throw new RuntimeException("Can't edit first tls. The list is empty.");
return setNewTlLike(0, buildTl(0));
}
public TlsNested editLastTl() {
int index = tls.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last tls. The list is empty.");
return setNewTlLike(index, buildTl(index));
}
public TlsNested editMatchingTl(Predicate predicate) {
int index = -1;
for (int i=0;i map) {
if(this.additionalProperties == null && map != null) { this.additionalProperties = new LinkedHashMap(); }
if(map != null) { this.additionalProperties.putAll(map);} return (A)this;
}
public A removeFromAdditionalProperties(String key) {
if(this.additionalProperties == null) { return (A) this; }
if(key != null && this.additionalProperties != null) {this.additionalProperties.remove(key);} return (A)this;
}
public A removeFromAdditionalProperties(Map map) {
if(this.additionalProperties == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.additionalProperties != null){this.additionalProperties.remove(key);}}} return (A)this;
}
public Map getAdditionalProperties() {
return this.additionalProperties;
}
public A withAdditionalProperties(Map additionalProperties) {
if (additionalProperties == null) {
this.additionalProperties = null;
} else {
this.additionalProperties = new LinkedHashMap(additionalProperties);
}
return (A) this;
}
public boolean hasAdditionalProperties() {
return this.additionalProperties != null;
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
IngressSpecFluent that = (IngressSpecFluent) o;
if (!java.util.Objects.equals(backend, that.backend)) return false;
if (!java.util.Objects.equals(ingressClassName, that.ingressClassName)) return false;
if (!java.util.Objects.equals(rules, that.rules)) return false;
if (!java.util.Objects.equals(tls, that.tls)) return false;
if (!java.util.Objects.equals(additionalProperties, that.additionalProperties)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(backend, ingressClassName, rules, tls, additionalProperties, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (backend != null) { sb.append("backend:"); sb.append(backend + ","); }
if (ingressClassName != null) { sb.append("ingressClassName:"); sb.append(ingressClassName + ","); }
if (rules != null && !rules.isEmpty()) { sb.append("rules:"); sb.append(rules + ","); }
if (tls != null && !tls.isEmpty()) { sb.append("tls:"); sb.append(tls + ","); }
if (additionalProperties != null && !additionalProperties.isEmpty()) { sb.append("additionalProperties:"); sb.append(additionalProperties); }
sb.append("}");
return sb.toString();
}
public class BackendNested extends IngressBackendFluent> implements Nested{
BackendNested(IngressBackend item) {
this.builder = new IngressBackendBuilder(this, item);
}
IngressBackendBuilder builder;
public N and() {
return (N) IngressSpecFluent.this.withBackend(builder.build());
}
public N endBackend() {
return and();
}
}
public class RulesNested extends IngressRuleFluent> implements Nested{
RulesNested(int index,IngressRule item) {
this.index = index;
this.builder = new IngressRuleBuilder(this, item);
}
IngressRuleBuilder builder;
int index;
public N and() {
return (N) IngressSpecFluent.this.setToRules(index,builder.build());
}
public N endRule() {
return and();
}
}
public class TlsNested extends IngressTLSFluent> implements Nested{
TlsNested(int index,IngressTLS item) {
this.index = index;
this.builder = new IngressTLSBuilder(this, item);
}
IngressTLSBuilder builder;
int index;
public N and() {
return (N) IngressSpecFluent.this.setToTls(index,builder.build());
}
public N endTl() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy