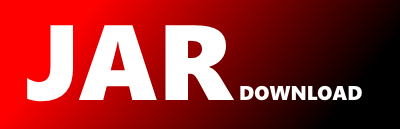
annotations.io.fabric8.kubernetes.api.model.VolumeFluentImpl Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import java.util.Map;
import java.util.Map;
import java.util.HashMap;
import java.io.Serializable;
import java.util.AbstractMap;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.Fluent;
import io.fabric8.kubernetes.api.builder.Builder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.Nested;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
public class VolumeFluentImpl> extends BaseFluent implements VolumeFluent{
VisitableBuilder awsElasticBlockStore; VisitableBuilder azureFile; VisitableBuilder cephfs; VisitableBuilder cinder; VisitableBuilder configMap; VisitableBuilder downwardAPI; VisitableBuilder emptyDir; VisitableBuilder fc; VisitableBuilder flexVolume; VisitableBuilder flocker; VisitableBuilder gcePersistentDisk; VisitableBuilder gitRepo; VisitableBuilder glusterfs; VisitableBuilder hostPath; VisitableBuilder iscsi; VisitableBuilder metadata; String name; VisitableBuilder nfs; VisitableBuilder persistentVolumeClaim; VisitableBuilder rbd; VisitableBuilder secret; Map additionalProperties = new HashMap();
public VolumeFluentImpl(){
}
public VolumeFluentImpl( Volume instance ){
this.withAwsElasticBlockStore(instance.getAwsElasticBlockStore()); this.withAzureFile(instance.getAzureFile()); this.withCephfs(instance.getCephfs()); this.withCinder(instance.getCinder()); this.withConfigMap(instance.getConfigMap()); this.withDownwardAPI(instance.getDownwardAPI()); this.withEmptyDir(instance.getEmptyDir()); this.withFc(instance.getFc()); this.withFlexVolume(instance.getFlexVolume()); this.withFlocker(instance.getFlocker()); this.withGcePersistentDisk(instance.getGcePersistentDisk()); this.withGitRepo(instance.getGitRepo()); this.withGlusterfs(instance.getGlusterfs()); this.withHostPath(instance.getHostPath()); this.withIscsi(instance.getIscsi()); this.withMetadata(instance.getMetadata()); this.withName(instance.getName()); this.withNfs(instance.getNfs()); this.withPersistentVolumeClaim(instance.getPersistentVolumeClaim()); this.withRbd(instance.getRbd()); this.withSecret(instance.getSecret());
}
public AWSElasticBlockStoreVolumeSource getAwsElasticBlockStore(){
return this.awsElasticBlockStore!=null?this.awsElasticBlockStore.build():null;
}
public T withAwsElasticBlockStore( AWSElasticBlockStoreVolumeSource awsElasticBlockStore){
if (awsElasticBlockStore!=null){ this.awsElasticBlockStore= new AWSElasticBlockStoreVolumeSourceBuilder(awsElasticBlockStore); _visitables.add(this.awsElasticBlockStore);} return (T) this;
}
public AwsElasticBlockStoreNested withNewAwsElasticBlockStore(){
return new AwsElasticBlockStoreNestedImpl();
}
public AwsElasticBlockStoreNested withNewAwsElasticBlockStoreLike( AWSElasticBlockStoreVolumeSource item){
return new AwsElasticBlockStoreNestedImpl(item);
}
public AwsElasticBlockStoreNested editAwsElasticBlockStore(){
return withNewAwsElasticBlockStoreLike(getAwsElasticBlockStore());
}
public T withNewAwsElasticBlockStore( String fsType, Integer partition, Boolean readOnly, String volumeID){
return withAwsElasticBlockStore(new AWSElasticBlockStoreVolumeSource(fsType, partition, readOnly, volumeID));
}
public AzureFileVolumeSource getAzureFile(){
return this.azureFile!=null?this.azureFile.build():null;
}
public T withAzureFile( AzureFileVolumeSource azureFile){
if (azureFile!=null){ this.azureFile= new AzureFileVolumeSourceBuilder(azureFile); _visitables.add(this.azureFile);} return (T) this;
}
public AzureFileNested withNewAzureFile(){
return new AzureFileNestedImpl();
}
public AzureFileNested withNewAzureFileLike( AzureFileVolumeSource item){
return new AzureFileNestedImpl(item);
}
public AzureFileNested editAzureFile(){
return withNewAzureFileLike(getAzureFile());
}
public T withNewAzureFile( Boolean readOnly, String secretName, String shareName){
return withAzureFile(new AzureFileVolumeSource(readOnly, secretName, shareName));
}
public CephFSVolumeSource getCephfs(){
return this.cephfs!=null?this.cephfs.build():null;
}
public T withCephfs( CephFSVolumeSource cephfs){
if (cephfs!=null){ this.cephfs= new CephFSVolumeSourceBuilder(cephfs); _visitables.add(this.cephfs);} return (T) this;
}
public CephfsNested withNewCephfs(){
return new CephfsNestedImpl();
}
public CephfsNested withNewCephfsLike( CephFSVolumeSource item){
return new CephfsNestedImpl(item);
}
public CephfsNested editCephfs(){
return withNewCephfsLike(getCephfs());
}
public CinderVolumeSource getCinder(){
return this.cinder!=null?this.cinder.build():null;
}
public T withCinder( CinderVolumeSource cinder){
if (cinder!=null){ this.cinder= new CinderVolumeSourceBuilder(cinder); _visitables.add(this.cinder);} return (T) this;
}
public CinderNested withNewCinder(){
return new CinderNestedImpl();
}
public CinderNested withNewCinderLike( CinderVolumeSource item){
return new CinderNestedImpl(item);
}
public CinderNested editCinder(){
return withNewCinderLike(getCinder());
}
public T withNewCinder( String fsType, Boolean readOnly, String volumeID){
return withCinder(new CinderVolumeSource(fsType, readOnly, volumeID));
}
public ConfigMapVolumeSource getConfigMap(){
return this.configMap!=null?this.configMap.build():null;
}
public T withConfigMap( ConfigMapVolumeSource configMap){
if (configMap!=null){ this.configMap= new ConfigMapVolumeSourceBuilder(configMap); _visitables.add(this.configMap);} return (T) this;
}
public ConfigMapNested withNewConfigMap(){
return new ConfigMapNestedImpl();
}
public ConfigMapNested withNewConfigMapLike( ConfigMapVolumeSource item){
return new ConfigMapNestedImpl(item);
}
public ConfigMapNested editConfigMap(){
return withNewConfigMapLike(getConfigMap());
}
public DownwardAPIVolumeSource getDownwardAPI(){
return this.downwardAPI!=null?this.downwardAPI.build():null;
}
public T withDownwardAPI( DownwardAPIVolumeSource downwardAPI){
if (downwardAPI!=null){ this.downwardAPI= new DownwardAPIVolumeSourceBuilder(downwardAPI); _visitables.add(this.downwardAPI);} return (T) this;
}
public DownwardAPINested withNewDownwardAPI(){
return new DownwardAPINestedImpl();
}
public DownwardAPINested withNewDownwardAPILike( DownwardAPIVolumeSource item){
return new DownwardAPINestedImpl(item);
}
public DownwardAPINested editDownwardAPI(){
return withNewDownwardAPILike(getDownwardAPI());
}
public EmptyDirVolumeSource getEmptyDir(){
return this.emptyDir!=null?this.emptyDir.build():null;
}
public T withEmptyDir( EmptyDirVolumeSource emptyDir){
if (emptyDir!=null){ this.emptyDir= new EmptyDirVolumeSourceBuilder(emptyDir); _visitables.add(this.emptyDir);} return (T) this;
}
public EmptyDirNested withNewEmptyDir(){
return new EmptyDirNestedImpl();
}
public EmptyDirNested withNewEmptyDirLike( EmptyDirVolumeSource item){
return new EmptyDirNestedImpl(item);
}
public EmptyDirNested editEmptyDir(){
return withNewEmptyDirLike(getEmptyDir());
}
public T withNewEmptyDir( String medium){
return withEmptyDir(new EmptyDirVolumeSource(medium));
}
public FCVolumeSource getFc(){
return this.fc!=null?this.fc.build():null;
}
public T withFc( FCVolumeSource fc){
if (fc!=null){ this.fc= new FCVolumeSourceBuilder(fc); _visitables.add(this.fc);} return (T) this;
}
public FcNested withNewFc(){
return new FcNestedImpl();
}
public FcNested withNewFcLike( FCVolumeSource item){
return new FcNestedImpl(item);
}
public FcNested editFc(){
return withNewFcLike(getFc());
}
public FlexVolumeSource getFlexVolume(){
return this.flexVolume!=null?this.flexVolume.build():null;
}
public T withFlexVolume( FlexVolumeSource flexVolume){
if (flexVolume!=null){ this.flexVolume= new FlexVolumeSourceBuilder(flexVolume); _visitables.add(this.flexVolume);} return (T) this;
}
public FlexVolumeNested withNewFlexVolume(){
return new FlexVolumeNestedImpl();
}
public FlexVolumeNested withNewFlexVolumeLike( FlexVolumeSource item){
return new FlexVolumeNestedImpl(item);
}
public FlexVolumeNested editFlexVolume(){
return withNewFlexVolumeLike(getFlexVolume());
}
public FlockerVolumeSource getFlocker(){
return this.flocker!=null?this.flocker.build():null;
}
public T withFlocker( FlockerVolumeSource flocker){
if (flocker!=null){ this.flocker= new FlockerVolumeSourceBuilder(flocker); _visitables.add(this.flocker);} return (T) this;
}
public FlockerNested withNewFlocker(){
return new FlockerNestedImpl();
}
public FlockerNested withNewFlockerLike( FlockerVolumeSource item){
return new FlockerNestedImpl(item);
}
public FlockerNested editFlocker(){
return withNewFlockerLike(getFlocker());
}
public T withNewFlocker( String datasetName){
return withFlocker(new FlockerVolumeSource(datasetName));
}
public GCEPersistentDiskVolumeSource getGcePersistentDisk(){
return this.gcePersistentDisk!=null?this.gcePersistentDisk.build():null;
}
public T withGcePersistentDisk( GCEPersistentDiskVolumeSource gcePersistentDisk){
if (gcePersistentDisk!=null){ this.gcePersistentDisk= new GCEPersistentDiskVolumeSourceBuilder(gcePersistentDisk); _visitables.add(this.gcePersistentDisk);} return (T) this;
}
public GcePersistentDiskNested withNewGcePersistentDisk(){
return new GcePersistentDiskNestedImpl();
}
public GcePersistentDiskNested withNewGcePersistentDiskLike( GCEPersistentDiskVolumeSource item){
return new GcePersistentDiskNestedImpl(item);
}
public GcePersistentDiskNested editGcePersistentDisk(){
return withNewGcePersistentDiskLike(getGcePersistentDisk());
}
public T withNewGcePersistentDisk( String fsType, Integer partition, String pdName, Boolean readOnly){
return withGcePersistentDisk(new GCEPersistentDiskVolumeSource(fsType, partition, pdName, readOnly));
}
public GitRepoVolumeSource getGitRepo(){
return this.gitRepo!=null?this.gitRepo.build():null;
}
public T withGitRepo( GitRepoVolumeSource gitRepo){
if (gitRepo!=null){ this.gitRepo= new GitRepoVolumeSourceBuilder(gitRepo); _visitables.add(this.gitRepo);} return (T) this;
}
public GitRepoNested withNewGitRepo(){
return new GitRepoNestedImpl();
}
public GitRepoNested withNewGitRepoLike( GitRepoVolumeSource item){
return new GitRepoNestedImpl(item);
}
public GitRepoNested editGitRepo(){
return withNewGitRepoLike(getGitRepo());
}
public T withNewGitRepo( String directory, String repository, String revision){
return withGitRepo(new GitRepoVolumeSource(directory, repository, revision));
}
public GlusterfsVolumeSource getGlusterfs(){
return this.glusterfs!=null?this.glusterfs.build():null;
}
public T withGlusterfs( GlusterfsVolumeSource glusterfs){
if (glusterfs!=null){ this.glusterfs= new GlusterfsVolumeSourceBuilder(glusterfs); _visitables.add(this.glusterfs);} return (T) this;
}
public GlusterfsNested withNewGlusterfs(){
return new GlusterfsNestedImpl();
}
public GlusterfsNested withNewGlusterfsLike( GlusterfsVolumeSource item){
return new GlusterfsNestedImpl(item);
}
public GlusterfsNested editGlusterfs(){
return withNewGlusterfsLike(getGlusterfs());
}
public T withNewGlusterfs( String endpoints, String path, Boolean readOnly){
return withGlusterfs(new GlusterfsVolumeSource(endpoints, path, readOnly));
}
public HostPathVolumeSource getHostPath(){
return this.hostPath!=null?this.hostPath.build():null;
}
public T withHostPath( HostPathVolumeSource hostPath){
if (hostPath!=null){ this.hostPath= new HostPathVolumeSourceBuilder(hostPath); _visitables.add(this.hostPath);} return (T) this;
}
public HostPathNested withNewHostPath(){
return new HostPathNestedImpl();
}
public HostPathNested withNewHostPathLike( HostPathVolumeSource item){
return new HostPathNestedImpl(item);
}
public HostPathNested editHostPath(){
return withNewHostPathLike(getHostPath());
}
public T withNewHostPath( String path){
return withHostPath(new HostPathVolumeSource(path));
}
public ISCSIVolumeSource getIscsi(){
return this.iscsi!=null?this.iscsi.build():null;
}
public T withIscsi( ISCSIVolumeSource iscsi){
if (iscsi!=null){ this.iscsi= new ISCSIVolumeSourceBuilder(iscsi); _visitables.add(this.iscsi);} return (T) this;
}
public IscsiNested withNewIscsi(){
return new IscsiNestedImpl();
}
public IscsiNested withNewIscsiLike( ISCSIVolumeSource item){
return new IscsiNestedImpl(item);
}
public IscsiNested editIscsi(){
return withNewIscsiLike(getIscsi());
}
public MetadataVolumeSource getMetadata(){
return this.metadata!=null?this.metadata.build():null;
}
public T withMetadata( MetadataVolumeSource metadata){
if (metadata!=null){ this.metadata= new MetadataVolumeSourceBuilder(metadata); _visitables.add(this.metadata);} return (T) this;
}
public MetadataNested withNewMetadata(){
return new MetadataNestedImpl();
}
public MetadataNested withNewMetadataLike( MetadataVolumeSource item){
return new MetadataNestedImpl(item);
}
public MetadataNested editMetadata(){
return withNewMetadataLike(getMetadata());
}
public String getName(){
return this.name;
}
public T withName( String name){
this.name=name; return (T) this;
}
public NFSVolumeSource getNfs(){
return this.nfs!=null?this.nfs.build():null;
}
public T withNfs( NFSVolumeSource nfs){
if (nfs!=null){ this.nfs= new NFSVolumeSourceBuilder(nfs); _visitables.add(this.nfs);} return (T) this;
}
public NfsNested withNewNfs(){
return new NfsNestedImpl();
}
public NfsNested withNewNfsLike( NFSVolumeSource item){
return new NfsNestedImpl(item);
}
public NfsNested editNfs(){
return withNewNfsLike(getNfs());
}
public T withNewNfs( String path, Boolean readOnly, String server){
return withNfs(new NFSVolumeSource(path, readOnly, server));
}
public PersistentVolumeClaimVolumeSource getPersistentVolumeClaim(){
return this.persistentVolumeClaim!=null?this.persistentVolumeClaim.build():null;
}
public T withPersistentVolumeClaim( PersistentVolumeClaimVolumeSource persistentVolumeClaim){
if (persistentVolumeClaim!=null){ this.persistentVolumeClaim= new PersistentVolumeClaimVolumeSourceBuilder(persistentVolumeClaim); _visitables.add(this.persistentVolumeClaim);} return (T) this;
}
public PersistentVolumeClaimNested withNewPersistentVolumeClaim(){
return new PersistentVolumeClaimNestedImpl();
}
public PersistentVolumeClaimNested withNewPersistentVolumeClaimLike( PersistentVolumeClaimVolumeSource item){
return new PersistentVolumeClaimNestedImpl(item);
}
public PersistentVolumeClaimNested editPersistentVolumeClaim(){
return withNewPersistentVolumeClaimLike(getPersistentVolumeClaim());
}
public T withNewPersistentVolumeClaim( String claimName, Boolean readOnly){
return withPersistentVolumeClaim(new PersistentVolumeClaimVolumeSource(claimName, readOnly));
}
public RBDVolumeSource getRbd(){
return this.rbd!=null?this.rbd.build():null;
}
public T withRbd( RBDVolumeSource rbd){
if (rbd!=null){ this.rbd= new RBDVolumeSourceBuilder(rbd); _visitables.add(this.rbd);} return (T) this;
}
public RbdNested withNewRbd(){
return new RbdNestedImpl();
}
public RbdNested withNewRbdLike( RBDVolumeSource item){
return new RbdNestedImpl(item);
}
public RbdNested editRbd(){
return withNewRbdLike(getRbd());
}
public SecretVolumeSource getSecret(){
return this.secret!=null?this.secret.build():null;
}
public T withSecret( SecretVolumeSource secret){
if (secret!=null){ this.secret= new SecretVolumeSourceBuilder(secret); _visitables.add(this.secret);} return (T) this;
}
public SecretNested withNewSecret(){
return new SecretNestedImpl();
}
public SecretNested withNewSecretLike( SecretVolumeSource item){
return new SecretNestedImpl(item);
}
public SecretNested editSecret(){
return withNewSecretLike(getSecret());
}
public T withNewSecret( String secretName){
return withSecret(new SecretVolumeSource(secretName));
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public T addToAdditionalProperties( Map map){
if(map != null) { this.additionalProperties.putAll(map);} return (T)this;
}
public T removeFromAdditionalProperties( String key){
if(key != null) {this.additionalProperties.remove(key);} return (T)this;
}
public T removeFromAdditionalProperties( Map map){
if(map != null) { for(Object key : map.keySet()) {this.additionalProperties.remove(key);}} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
public boolean equals( Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
VolumeFluentImpl that = (VolumeFluentImpl) o;
if (awsElasticBlockStore != null ? !awsElasticBlockStore.equals(that.awsElasticBlockStore) :that.awsElasticBlockStore != null) return false;
if (azureFile != null ? !azureFile.equals(that.azureFile) :that.azureFile != null) return false;
if (cephfs != null ? !cephfs.equals(that.cephfs) :that.cephfs != null) return false;
if (cinder != null ? !cinder.equals(that.cinder) :that.cinder != null) return false;
if (configMap != null ? !configMap.equals(that.configMap) :that.configMap != null) return false;
if (downwardAPI != null ? !downwardAPI.equals(that.downwardAPI) :that.downwardAPI != null) return false;
if (emptyDir != null ? !emptyDir.equals(that.emptyDir) :that.emptyDir != null) return false;
if (fc != null ? !fc.equals(that.fc) :that.fc != null) return false;
if (flexVolume != null ? !flexVolume.equals(that.flexVolume) :that.flexVolume != null) return false;
if (flocker != null ? !flocker.equals(that.flocker) :that.flocker != null) return false;
if (gcePersistentDisk != null ? !gcePersistentDisk.equals(that.gcePersistentDisk) :that.gcePersistentDisk != null) return false;
if (gitRepo != null ? !gitRepo.equals(that.gitRepo) :that.gitRepo != null) return false;
if (glusterfs != null ? !glusterfs.equals(that.glusterfs) :that.glusterfs != null) return false;
if (hostPath != null ? !hostPath.equals(that.hostPath) :that.hostPath != null) return false;
if (iscsi != null ? !iscsi.equals(that.iscsi) :that.iscsi != null) return false;
if (metadata != null ? !metadata.equals(that.metadata) :that.metadata != null) return false;
if (name != null ? !name.equals(that.name) :that.name != null) return false;
if (nfs != null ? !nfs.equals(that.nfs) :that.nfs != null) return false;
if (persistentVolumeClaim != null ? !persistentVolumeClaim.equals(that.persistentVolumeClaim) :that.persistentVolumeClaim != null) return false;
if (rbd != null ? !rbd.equals(that.rbd) :that.rbd != null) return false;
if (secret != null ? !secret.equals(that.secret) :that.secret != null) return false;
if (additionalProperties != null ? !additionalProperties.equals(that.additionalProperties) :that.additionalProperties != null) return false;
return true;
}
public class AwsElasticBlockStoreNestedImpl extends AWSElasticBlockStoreVolumeSourceFluentImpl> implements AwsElasticBlockStoreNested{
private final AWSElasticBlockStoreVolumeSourceBuilder builder;
AwsElasticBlockStoreNestedImpl (){
this.builder = new AWSElasticBlockStoreVolumeSourceBuilder(this);
}
AwsElasticBlockStoreNestedImpl ( AWSElasticBlockStoreVolumeSource item){
this.builder = new AWSElasticBlockStoreVolumeSourceBuilder(this, item);
}
public N endAwsElasticBlockStore(){
return and();
}
public N and(){
return (N) VolumeFluentImpl.this.withAwsElasticBlockStore(builder.build());
}
}
public class AzureFileNestedImpl extends AzureFileVolumeSourceFluentImpl> implements AzureFileNested{
private final AzureFileVolumeSourceBuilder builder;
AzureFileNestedImpl ( AzureFileVolumeSource item){
this.builder = new AzureFileVolumeSourceBuilder(this, item);
}
AzureFileNestedImpl (){
this.builder = new AzureFileVolumeSourceBuilder(this);
}
public N endAzureFile(){
return and();
}
public N and(){
return (N) VolumeFluentImpl.this.withAzureFile(builder.build());
}
}
public class CephfsNestedImpl extends CephFSVolumeSourceFluentImpl> implements CephfsNested{
private final CephFSVolumeSourceBuilder builder;
CephfsNestedImpl (){
this.builder = new CephFSVolumeSourceBuilder(this);
}
CephfsNestedImpl ( CephFSVolumeSource item){
this.builder = new CephFSVolumeSourceBuilder(this, item);
}
public N endCephfs(){
return and();
}
public N and(){
return (N) VolumeFluentImpl.this.withCephfs(builder.build());
}
}
public class CinderNestedImpl extends CinderVolumeSourceFluentImpl> implements CinderNested{
private final CinderVolumeSourceBuilder builder;
CinderNestedImpl (){
this.builder = new CinderVolumeSourceBuilder(this);
}
CinderNestedImpl ( CinderVolumeSource item){
this.builder = new CinderVolumeSourceBuilder(this, item);
}
public N and(){
return (N) VolumeFluentImpl.this.withCinder(builder.build());
}
public N endCinder(){
return and();
}
}
public class ConfigMapNestedImpl extends ConfigMapVolumeSourceFluentImpl> implements ConfigMapNested{
private final ConfigMapVolumeSourceBuilder builder;
ConfigMapNestedImpl (){
this.builder = new ConfigMapVolumeSourceBuilder(this);
}
ConfigMapNestedImpl ( ConfigMapVolumeSource item){
this.builder = new ConfigMapVolumeSourceBuilder(this, item);
}
public N and(){
return (N) VolumeFluentImpl.this.withConfigMap(builder.build());
}
public N endConfigMap(){
return and();
}
}
public class DownwardAPINestedImpl extends DownwardAPIVolumeSourceFluentImpl> implements DownwardAPINested{
private final DownwardAPIVolumeSourceBuilder builder;
DownwardAPINestedImpl (){
this.builder = new DownwardAPIVolumeSourceBuilder(this);
}
DownwardAPINestedImpl ( DownwardAPIVolumeSource item){
this.builder = new DownwardAPIVolumeSourceBuilder(this, item);
}
public N endDownwardAPI(){
return and();
}
public N and(){
return (N) VolumeFluentImpl.this.withDownwardAPI(builder.build());
}
}
public class EmptyDirNestedImpl extends EmptyDirVolumeSourceFluentImpl> implements EmptyDirNested{
private final EmptyDirVolumeSourceBuilder builder;
EmptyDirNestedImpl (){
this.builder = new EmptyDirVolumeSourceBuilder(this);
}
EmptyDirNestedImpl ( EmptyDirVolumeSource item){
this.builder = new EmptyDirVolumeSourceBuilder(this, item);
}
public N endEmptyDir(){
return and();
}
public N and(){
return (N) VolumeFluentImpl.this.withEmptyDir(builder.build());
}
}
public class FcNestedImpl extends FCVolumeSourceFluentImpl> implements FcNested{
private final FCVolumeSourceBuilder builder;
FcNestedImpl (){
this.builder = new FCVolumeSourceBuilder(this);
}
FcNestedImpl ( FCVolumeSource item){
this.builder = new FCVolumeSourceBuilder(this, item);
}
public N and(){
return (N) VolumeFluentImpl.this.withFc(builder.build());
}
public N endFc(){
return and();
}
}
public class FlexVolumeNestedImpl extends FlexVolumeSourceFluentImpl> implements FlexVolumeNested{
private final FlexVolumeSourceBuilder builder;
FlexVolumeNestedImpl (){
this.builder = new FlexVolumeSourceBuilder(this);
}
FlexVolumeNestedImpl ( FlexVolumeSource item){
this.builder = new FlexVolumeSourceBuilder(this, item);
}
public N and(){
return (N) VolumeFluentImpl.this.withFlexVolume(builder.build());
}
public N endFlexVolume(){
return and();
}
}
public class FlockerNestedImpl extends FlockerVolumeSourceFluentImpl> implements FlockerNested{
private final FlockerVolumeSourceBuilder builder;
FlockerNestedImpl (){
this.builder = new FlockerVolumeSourceBuilder(this);
}
FlockerNestedImpl ( FlockerVolumeSource item){
this.builder = new FlockerVolumeSourceBuilder(this, item);
}
public N and(){
return (N) VolumeFluentImpl.this.withFlocker(builder.build());
}
public N endFlocker(){
return and();
}
}
public class GcePersistentDiskNestedImpl extends GCEPersistentDiskVolumeSourceFluentImpl> implements GcePersistentDiskNested{
private final GCEPersistentDiskVolumeSourceBuilder builder;
GcePersistentDiskNestedImpl (){
this.builder = new GCEPersistentDiskVolumeSourceBuilder(this);
}
GcePersistentDiskNestedImpl ( GCEPersistentDiskVolumeSource item){
this.builder = new GCEPersistentDiskVolumeSourceBuilder(this, item);
}
public N endGcePersistentDisk(){
return and();
}
public N and(){
return (N) VolumeFluentImpl.this.withGcePersistentDisk(builder.build());
}
}
public class GitRepoNestedImpl extends GitRepoVolumeSourceFluentImpl> implements GitRepoNested{
private final GitRepoVolumeSourceBuilder builder;
GitRepoNestedImpl (){
this.builder = new GitRepoVolumeSourceBuilder(this);
}
GitRepoNestedImpl ( GitRepoVolumeSource item){
this.builder = new GitRepoVolumeSourceBuilder(this, item);
}
public N endGitRepo(){
return and();
}
public N and(){
return (N) VolumeFluentImpl.this.withGitRepo(builder.build());
}
}
public class GlusterfsNestedImpl extends GlusterfsVolumeSourceFluentImpl> implements GlusterfsNested{
private final GlusterfsVolumeSourceBuilder builder;
GlusterfsNestedImpl (){
this.builder = new GlusterfsVolumeSourceBuilder(this);
}
GlusterfsNestedImpl ( GlusterfsVolumeSource item){
this.builder = new GlusterfsVolumeSourceBuilder(this, item);
}
public N and(){
return (N) VolumeFluentImpl.this.withGlusterfs(builder.build());
}
public N endGlusterfs(){
return and();
}
}
public class HostPathNestedImpl extends HostPathVolumeSourceFluentImpl> implements HostPathNested{
private final HostPathVolumeSourceBuilder builder;
HostPathNestedImpl (){
this.builder = new HostPathVolumeSourceBuilder(this);
}
HostPathNestedImpl ( HostPathVolumeSource item){
this.builder = new HostPathVolumeSourceBuilder(this, item);
}
public N and(){
return (N) VolumeFluentImpl.this.withHostPath(builder.build());
}
public N endHostPath(){
return and();
}
}
public class IscsiNestedImpl extends ISCSIVolumeSourceFluentImpl> implements IscsiNested{
private final ISCSIVolumeSourceBuilder builder;
IscsiNestedImpl ( ISCSIVolumeSource item){
this.builder = new ISCSIVolumeSourceBuilder(this, item);
}
IscsiNestedImpl (){
this.builder = new ISCSIVolumeSourceBuilder(this);
}
public N and(){
return (N) VolumeFluentImpl.this.withIscsi(builder.build());
}
public N endIscsi(){
return and();
}
}
public class MetadataNestedImpl extends MetadataVolumeSourceFluentImpl> implements MetadataNested{
private final MetadataVolumeSourceBuilder builder;
MetadataNestedImpl (){
this.builder = new MetadataVolumeSourceBuilder(this);
}
MetadataNestedImpl ( MetadataVolumeSource item){
this.builder = new MetadataVolumeSourceBuilder(this, item);
}
public N and(){
return (N) VolumeFluentImpl.this.withMetadata(builder.build());
}
public N endMetadata(){
return and();
}
}
public class NfsNestedImpl extends NFSVolumeSourceFluentImpl> implements NfsNested{
private final NFSVolumeSourceBuilder builder;
NfsNestedImpl ( NFSVolumeSource item){
this.builder = new NFSVolumeSourceBuilder(this, item);
}
NfsNestedImpl (){
this.builder = new NFSVolumeSourceBuilder(this);
}
public N endNfs(){
return and();
}
public N and(){
return (N) VolumeFluentImpl.this.withNfs(builder.build());
}
}
public class PersistentVolumeClaimNestedImpl extends PersistentVolumeClaimVolumeSourceFluentImpl> implements PersistentVolumeClaimNested{
private final PersistentVolumeClaimVolumeSourceBuilder builder;
PersistentVolumeClaimNestedImpl (){
this.builder = new PersistentVolumeClaimVolumeSourceBuilder(this);
}
PersistentVolumeClaimNestedImpl ( PersistentVolumeClaimVolumeSource item){
this.builder = new PersistentVolumeClaimVolumeSourceBuilder(this, item);
}
public N endPersistentVolumeClaim(){
return and();
}
public N and(){
return (N) VolumeFluentImpl.this.withPersistentVolumeClaim(builder.build());
}
}
public class RbdNestedImpl extends RBDVolumeSourceFluentImpl> implements RbdNested{
private final RBDVolumeSourceBuilder builder;
RbdNestedImpl (){
this.builder = new RBDVolumeSourceBuilder(this);
}
RbdNestedImpl ( RBDVolumeSource item){
this.builder = new RBDVolumeSourceBuilder(this, item);
}
public N and(){
return (N) VolumeFluentImpl.this.withRbd(builder.build());
}
public N endRbd(){
return and();
}
}
public class SecretNestedImpl extends SecretVolumeSourceFluentImpl> implements SecretNested{
private final SecretVolumeSourceBuilder builder;
SecretNestedImpl (){
this.builder = new SecretVolumeSourceBuilder(this);
}
SecretNestedImpl ( SecretVolumeSource item){
this.builder = new SecretVolumeSourceBuilder(this, item);
}
public N and(){
return (N) VolumeFluentImpl.this.withSecret(builder.build());
}
public N endSecret(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy