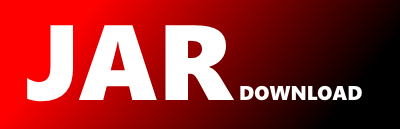
io.fabric8.kubernetes.api.model.KubeSchemaBuilder Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import javax.validation.ConstraintViolation;
import javax.validation.Validation;
import javax.validation.Validator;
import javax.validation.ValidatorFactory;
import javax.validation.ValidationException;
import java.util.Set;
import io.fabric8.openshift.api.model.OAuthAccessToken;
import io.fabric8.openshift.api.model.Identity;
import io.fabric8.openshift.api.model.ClusterRoleBinding;
import io.fabric8.openshift.api.model.Template;
import io.fabric8.kubernetes.api.model.extensions.ThirdPartyResource;
import java.io.Serializable;
import io.fabric8.kubernetes.api.model.extensions.Ingress;
import io.fabric8.openshift.api.model.OAuthAuthorizeToken;
import io.fabric8.kubernetes.api.model.extensions.Deployment;
import io.fabric8.openshift.api.model.PolicyBindingList;
import io.fabric8.kubernetes.api.model.extensions.DeploymentRollback;
import io.fabric8.kubernetes.api.model.extensions.HorizontalPodAutoscaler;
import io.fabric8.openshift.api.model.ClusterPolicyBinding;
import io.fabric8.openshift.api.model.SubjectAccessReview;
import io.fabric8.openshift.api.model.PolicyList;
import io.fabric8.openshift.api.model.ClusterPolicyList;
import io.fabric8.openshift.api.model.OAuthClient;
import io.fabric8.openshift.api.model.LocalSubjectAccessReview;
import io.fabric8.openshift.api.model.ProjectList;
import io.fabric8.kubernetes.api.model.extensions.ReplicaSetList;
import io.fabric8.openshift.api.model.ImageList;
import io.fabric8.openshift.api.model.Policy;
import io.fabric8.openshift.api.model.BuildRequest;
import io.fabric8.openshift.api.model.UserList;
import io.fabric8.openshift.api.model.ImageStreamList;
import io.fabric8.openshift.api.model.OAuthClientList;
import io.fabric8.openshift.api.model.RouteList;
import io.fabric8.kubernetes.api.model.extensions.HorizontalPodAutoscalerList;
import io.fabric8.kubernetes.api.model.extensions.Job;
import io.fabric8.openshift.api.model.TemplateList;
import io.fabric8.openshift.api.model.Project;
import io.fabric8.kubernetes.api.model.extensions.DaemonSetList;
import io.fabric8.openshift.api.model.OAuthClientAuthorizationList;
import io.fabric8.openshift.api.model.OAuthClientAuthorization;
import io.fabric8.openshift.api.model.TagEvent;
import io.fabric8.openshift.api.model.RoleList;
import io.fabric8.kubernetes.api.model.extensions.ThirdPartyResourceList;
import io.fabric8.kubernetes.api.model.extensions.JobList;
import java.util.Map;
import io.fabric8.openshift.api.model.ProjectRequest;
import io.fabric8.openshift.api.model.BuildList;
import io.fabric8.openshift.api.model.SubjectAccessReviewResponse;
import io.fabric8.openshift.api.model.GroupList;
import io.fabric8.openshift.api.model.RoleBinding;
import io.fabric8.openshift.api.model.User;
import io.fabric8.kubernetes.api.model.extensions.DeploymentList;
import io.fabric8.openshift.api.model.BuildConfigList;
import io.fabric8.kubernetes.api.model.extensions.Scale;
import io.fabric8.openshift.api.model.ClusterPolicyBindingList;
import java.util.AbstractMap;
import io.fabric8.openshift.api.model.RoleBindingList;
import io.fabric8.openshift.api.model.Role;
import java.util.Map;
import io.fabric8.kubernetes.api.model.extensions.IngressList;
import io.fabric8.openshift.api.model.OAuthAccessTokenList;
import io.fabric8.openshift.api.model.ClusterPolicy;
import io.fabric8.openshift.api.model.DeploymentConfigList;
import io.fabric8.openshift.api.model.Group;
import io.fabric8.openshift.api.model.ClusterRoleBindingList;
import java.util.HashMap;
import io.fabric8.openshift.api.model.IdentityList;
import io.fabric8.openshift.api.model.OAuthAuthorizeTokenList;
import io.fabric8.kubernetes.api.model.extensions.DaemonSet;
import io.fabric8.kubernetes.api.model.extensions.ReplicaSet;
import io.fabric8.openshift.api.model.PolicyBinding;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.Builder;
import io.fabric8.kubernetes.api.builder.Fluent;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.BaseFluent;
public class KubeSchemaBuilder extends KubeSchemaFluentImpl implements VisitableBuilder{
KubeSchemaFluent> fluent;
public KubeSchemaBuilder(){
this(new KubeSchema());
}
public KubeSchemaBuilder( KubeSchemaFluent> fluent ){
this(fluent, new KubeSchema());
}
public KubeSchemaBuilder( KubeSchemaFluent> fluent , KubeSchema instance ){
this.fluent = fluent; fluent.withBaseKubernetesList(instance.getBaseKubernetesList()); fluent.withBinding(instance.getBinding()); fluent.withBuildConfigList(instance.getBuildConfigList()); fluent.withBuildList(instance.getBuildList()); fluent.withBuildRequest(instance.getBuildRequest()); fluent.withClusterPolicy(instance.getClusterPolicy()); fluent.withClusterPolicyBinding(instance.getClusterPolicyBinding()); fluent.withClusterPolicyBindingList(instance.getClusterPolicyBindingList()); fluent.withClusterPolicyList(instance.getClusterPolicyList()); fluent.withClusterRoleBinding(instance.getClusterRoleBinding()); fluent.withClusterRoleBindingList(instance.getClusterRoleBindingList()); fluent.withComponentStatusList(instance.getComponentStatusList()); fluent.withConfig(instance.getConfig()); fluent.withConfigMap(instance.getConfigMap()); fluent.withConfigMapList(instance.getConfigMapList()); fluent.withContainerStatus(instance.getContainerStatus()); fluent.withDaemonSet(instance.getDaemonSet()); fluent.withDaemonSetList(instance.getDaemonSetList()); fluent.withDeleteOptions(instance.getDeleteOptions()); fluent.withDeployment(instance.getDeployment()); fluent.withDeploymentConfigList(instance.getDeploymentConfigList()); fluent.withDeploymentList(instance.getDeploymentList()); fluent.withDeploymentRollback(instance.getDeploymentRollback()); fluent.withEndpoints(instance.getEndpoints()); fluent.withEndpointsList(instance.getEndpointsList()); fluent.withEnvVar(instance.getEnvVar()); fluent.withEventList(instance.getEventList()); fluent.withGroup(instance.getGroup()); fluent.withGroupList(instance.getGroupList()); fluent.withHorizontalPodAutoscaler(instance.getHorizontalPodAutoscaler()); fluent.withHorizontalPodAutoscalerList(instance.getHorizontalPodAutoscalerList()); fluent.withIdentity(instance.getIdentity()); fluent.withIdentityList(instance.getIdentityList()); fluent.withImageList(instance.getImageList()); fluent.withImageStreamList(instance.getImageStreamList()); fluent.withIngress(instance.getIngress()); fluent.withIngressList(instance.getIngressList()); fluent.withJob(instance.getJob()); fluent.withJobList(instance.getJobList()); fluent.withLimitRangeList(instance.getLimitRangeList()); fluent.withListMeta(instance.getListMeta()); fluent.withLocalSubjectAccessReview(instance.getLocalSubjectAccessReview()); fluent.withNamespace(instance.getNamespace()); fluent.withNamespaceList(instance.getNamespaceList()); fluent.withNode(instance.getNode()); fluent.withNodeList(instance.getNodeList()); fluent.withOAuthAccessToken(instance.getOAuthAccessToken()); fluent.withOAuthAccessTokenList(instance.getOAuthAccessTokenList()); fluent.withOAuthAuthorizeToken(instance.getOAuthAuthorizeToken()); fluent.withOAuthAuthorizeTokenList(instance.getOAuthAuthorizeTokenList()); fluent.withOAuthClient(instance.getOAuthClient()); fluent.withOAuthClientAuthorization(instance.getOAuthClientAuthorization()); fluent.withOAuthClientAuthorizationList(instance.getOAuthClientAuthorizationList()); fluent.withOAuthClientList(instance.getOAuthClientList()); fluent.withObjectMeta(instance.getObjectMeta()); fluent.withPersistentVolume(instance.getPersistentVolume()); fluent.withPersistentVolumeClaim(instance.getPersistentVolumeClaim()); fluent.withPersistentVolumeClaimList(instance.getPersistentVolumeClaimList()); fluent.withPersistentVolumeList(instance.getPersistentVolumeList()); fluent.withPodList(instance.getPodList()); fluent.withPodTemplateList(instance.getPodTemplateList()); fluent.withPolicy(instance.getPolicy()); fluent.withPolicyBinding(instance.getPolicyBinding()); fluent.withPolicyBindingList(instance.getPolicyBindingList()); fluent.withPolicyList(instance.getPolicyList()); fluent.withProject(instance.getProject()); fluent.withProjectList(instance.getProjectList()); fluent.withProjectRequest(instance.getProjectRequest()); fluent.withQuantity(instance.getQuantity()); fluent.withReplicaSet(instance.getReplicaSet()); fluent.withReplicaSetList(instance.getReplicaSetList()); fluent.withReplicationControllerList(instance.getReplicationControllerList()); fluent.withResourceQuota(instance.getResourceQuota()); fluent.withResourceQuotaList(instance.getResourceQuotaList()); fluent.withRole(instance.getRole()); fluent.withRoleBinding(instance.getRoleBinding()); fluent.withRoleBindingList(instance.getRoleBindingList()); fluent.withRoleList(instance.getRoleList()); fluent.withRootPaths(instance.getRootPaths()); fluent.withRouteList(instance.getRouteList()); fluent.withScale(instance.getScale()); fluent.withSecret(instance.getSecret()); fluent.withSecretList(instance.getSecretList()); fluent.withSecurityContextConstraints(instance.getSecurityContextConstraints()); fluent.withSecurityContextConstraintsList(instance.getSecurityContextConstraintsList()); fluent.withServiceAccount(instance.getServiceAccount()); fluent.withServiceAccountList(instance.getServiceAccountList()); fluent.withServiceList(instance.getServiceList()); fluent.withStatus(instance.getStatus()); fluent.withSubjectAccessReview(instance.getSubjectAccessReview()); fluent.withSubjectAccessReviewResponse(instance.getSubjectAccessReviewResponse()); fluent.withTagEvent(instance.getTagEvent()); fluent.withTemplate(instance.getTemplate()); fluent.withTemplateList(instance.getTemplateList()); fluent.withThirdPartyResource(instance.getThirdPartyResource()); fluent.withThirdPartyResourceList(instance.getThirdPartyResourceList()); fluent.withUser(instance.getUser()); fluent.withUserList(instance.getUserList()); fluent.withWatchEvent(instance.getWatchEvent());
}
public KubeSchemaBuilder( KubeSchema instance ){
this.fluent = this; this.withBaseKubernetesList(instance.getBaseKubernetesList()); this.withBinding(instance.getBinding()); this.withBuildConfigList(instance.getBuildConfigList()); this.withBuildList(instance.getBuildList()); this.withBuildRequest(instance.getBuildRequest()); this.withClusterPolicy(instance.getClusterPolicy()); this.withClusterPolicyBinding(instance.getClusterPolicyBinding()); this.withClusterPolicyBindingList(instance.getClusterPolicyBindingList()); this.withClusterPolicyList(instance.getClusterPolicyList()); this.withClusterRoleBinding(instance.getClusterRoleBinding()); this.withClusterRoleBindingList(instance.getClusterRoleBindingList()); this.withComponentStatusList(instance.getComponentStatusList()); this.withConfig(instance.getConfig()); this.withConfigMap(instance.getConfigMap()); this.withConfigMapList(instance.getConfigMapList()); this.withContainerStatus(instance.getContainerStatus()); this.withDaemonSet(instance.getDaemonSet()); this.withDaemonSetList(instance.getDaemonSetList()); this.withDeleteOptions(instance.getDeleteOptions()); this.withDeployment(instance.getDeployment()); this.withDeploymentConfigList(instance.getDeploymentConfigList()); this.withDeploymentList(instance.getDeploymentList()); this.withDeploymentRollback(instance.getDeploymentRollback()); this.withEndpoints(instance.getEndpoints()); this.withEndpointsList(instance.getEndpointsList()); this.withEnvVar(instance.getEnvVar()); this.withEventList(instance.getEventList()); this.withGroup(instance.getGroup()); this.withGroupList(instance.getGroupList()); this.withHorizontalPodAutoscaler(instance.getHorizontalPodAutoscaler()); this.withHorizontalPodAutoscalerList(instance.getHorizontalPodAutoscalerList()); this.withIdentity(instance.getIdentity()); this.withIdentityList(instance.getIdentityList()); this.withImageList(instance.getImageList()); this.withImageStreamList(instance.getImageStreamList()); this.withIngress(instance.getIngress()); this.withIngressList(instance.getIngressList()); this.withJob(instance.getJob()); this.withJobList(instance.getJobList()); this.withLimitRangeList(instance.getLimitRangeList()); this.withListMeta(instance.getListMeta()); this.withLocalSubjectAccessReview(instance.getLocalSubjectAccessReview()); this.withNamespace(instance.getNamespace()); this.withNamespaceList(instance.getNamespaceList()); this.withNode(instance.getNode()); this.withNodeList(instance.getNodeList()); this.withOAuthAccessToken(instance.getOAuthAccessToken()); this.withOAuthAccessTokenList(instance.getOAuthAccessTokenList()); this.withOAuthAuthorizeToken(instance.getOAuthAuthorizeToken()); this.withOAuthAuthorizeTokenList(instance.getOAuthAuthorizeTokenList()); this.withOAuthClient(instance.getOAuthClient()); this.withOAuthClientAuthorization(instance.getOAuthClientAuthorization()); this.withOAuthClientAuthorizationList(instance.getOAuthClientAuthorizationList()); this.withOAuthClientList(instance.getOAuthClientList()); this.withObjectMeta(instance.getObjectMeta()); this.withPersistentVolume(instance.getPersistentVolume()); this.withPersistentVolumeClaim(instance.getPersistentVolumeClaim()); this.withPersistentVolumeClaimList(instance.getPersistentVolumeClaimList()); this.withPersistentVolumeList(instance.getPersistentVolumeList()); this.withPodList(instance.getPodList()); this.withPodTemplateList(instance.getPodTemplateList()); this.withPolicy(instance.getPolicy()); this.withPolicyBinding(instance.getPolicyBinding()); this.withPolicyBindingList(instance.getPolicyBindingList()); this.withPolicyList(instance.getPolicyList()); this.withProject(instance.getProject()); this.withProjectList(instance.getProjectList()); this.withProjectRequest(instance.getProjectRequest()); this.withQuantity(instance.getQuantity()); this.withReplicaSet(instance.getReplicaSet()); this.withReplicaSetList(instance.getReplicaSetList()); this.withReplicationControllerList(instance.getReplicationControllerList()); this.withResourceQuota(instance.getResourceQuota()); this.withResourceQuotaList(instance.getResourceQuotaList()); this.withRole(instance.getRole()); this.withRoleBinding(instance.getRoleBinding()); this.withRoleBindingList(instance.getRoleBindingList()); this.withRoleList(instance.getRoleList()); this.withRootPaths(instance.getRootPaths()); this.withRouteList(instance.getRouteList()); this.withScale(instance.getScale()); this.withSecret(instance.getSecret()); this.withSecretList(instance.getSecretList()); this.withSecurityContextConstraints(instance.getSecurityContextConstraints()); this.withSecurityContextConstraintsList(instance.getSecurityContextConstraintsList()); this.withServiceAccount(instance.getServiceAccount()); this.withServiceAccountList(instance.getServiceAccountList()); this.withServiceList(instance.getServiceList()); this.withStatus(instance.getStatus()); this.withSubjectAccessReview(instance.getSubjectAccessReview()); this.withSubjectAccessReviewResponse(instance.getSubjectAccessReviewResponse()); this.withTagEvent(instance.getTagEvent()); this.withTemplate(instance.getTemplate()); this.withTemplateList(instance.getTemplateList()); this.withThirdPartyResource(instance.getThirdPartyResource()); this.withThirdPartyResourceList(instance.getThirdPartyResourceList()); this.withUser(instance.getUser()); this.withUserList(instance.getUserList()); this.withWatchEvent(instance.getWatchEvent());
}
public EditableKubeSchema build(){
EditableKubeSchema buildable = new EditableKubeSchema(fluent.getBaseKubernetesList(),fluent.getBinding(),fluent.getBuildConfigList(),fluent.getBuildList(),fluent.getBuildRequest(),fluent.getClusterPolicy(),fluent.getClusterPolicyBinding(),fluent.getClusterPolicyBindingList(),fluent.getClusterPolicyList(),fluent.getClusterRoleBinding(),fluent.getClusterRoleBindingList(),fluent.getComponentStatusList(),fluent.getConfig(),fluent.getConfigMap(),fluent.getConfigMapList(),fluent.getContainerStatus(),fluent.getDaemonSet(),fluent.getDaemonSetList(),fluent.getDeleteOptions(),fluent.getDeployment(),fluent.getDeploymentConfigList(),fluent.getDeploymentList(),fluent.getDeploymentRollback(),fluent.getEndpoints(),fluent.getEndpointsList(),fluent.getEnvVar(),fluent.getEventList(),fluent.getGroup(),fluent.getGroupList(),fluent.getHorizontalPodAutoscaler(),fluent.getHorizontalPodAutoscalerList(),fluent.getIdentity(),fluent.getIdentityList(),fluent.getImageList(),fluent.getImageStreamList(),fluent.getIngress(),fluent.getIngressList(),fluent.getJob(),fluent.getJobList(),fluent.getLimitRangeList(),fluent.getListMeta(),fluent.getLocalSubjectAccessReview(),fluent.getNamespace(),fluent.getNamespaceList(),fluent.getNode(),fluent.getNodeList(),fluent.getOAuthAccessToken(),fluent.getOAuthAccessTokenList(),fluent.getOAuthAuthorizeToken(),fluent.getOAuthAuthorizeTokenList(),fluent.getOAuthClient(),fluent.getOAuthClientAuthorization(),fluent.getOAuthClientAuthorizationList(),fluent.getOAuthClientList(),fluent.getObjectMeta(),fluent.getPersistentVolume(),fluent.getPersistentVolumeClaim(),fluent.getPersistentVolumeClaimList(),fluent.getPersistentVolumeList(),fluent.getPodList(),fluent.getPodTemplateList(),fluent.getPolicy(),fluent.getPolicyBinding(),fluent.getPolicyBindingList(),fluent.getPolicyList(),fluent.getProject(),fluent.getProjectList(),fluent.getProjectRequest(),fluent.getQuantity(),fluent.getReplicaSet(),fluent.getReplicaSetList(),fluent.getReplicationControllerList(),fluent.getResourceQuota(),fluent.getResourceQuotaList(),fluent.getRole(),fluent.getRoleBinding(),fluent.getRoleBindingList(),fluent.getRoleList(),fluent.getRootPaths(),fluent.getRouteList(),fluent.getScale(),fluent.getSecret(),fluent.getSecretList(),fluent.getSecurityContextConstraints(),fluent.getSecurityContextConstraintsList(),fluent.getServiceAccount(),fluent.getServiceAccountList(),fluent.getServiceList(),fluent.getStatus(),fluent.getSubjectAccessReview(),fluent.getSubjectAccessReviewResponse(),fluent.getTagEvent(),fluent.getTemplate(),fluent.getTemplateList(),fluent.getThirdPartyResource(),fluent.getThirdPartyResourceList(),fluent.getUser(),fluent.getUserList(),fluent.getWatchEvent());
validate(buildable);
return buildable;
}
public boolean equals( Object o ){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
KubeSchemaBuilder that = (KubeSchemaBuilder) o;
if (fluent != null &&fluent != this ? !fluent.equals(that.fluent) :that.fluent != null &&fluent != this ) return false;
return true;
}
private void validate(T item) {
try {
ValidatorFactory factory = Validation.buildDefaultValidatorFactory();
Validator validator = factory.getValidator();
Set> violations = validator.validate(item);
if (!violations.isEmpty()) {
StringBuilder sb = new StringBuilder();
sb.append("Constraint Violations:\n");
for (ConstraintViolation violation : violations) {
sb.append("\t").append(violation.getRootBeanClass().getSimpleName()).append(" ").append(violation.getPropertyPath()).append(":").append(violation.getMessage()).append("\n");
}
throw new IllegalStateException(sb.toString());
}
} catch(ValidationException e) {
//ignore
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy