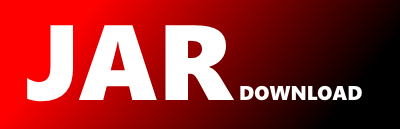
io.fabric8.kubernetes.api.model.NodeStatusFluentImpl Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import java.util.ArrayList;
import java.util.RandomAccess;
import java.util.Map;
import java.util.Map;
import java.util.List;
import java.util.List;
import java.util.AbstractCollection;
import java.util.List;
import java.util.AbstractList;
import java.util.Map;
import java.util.HashMap;
import java.io.Serializable;
import java.util.List;
import java.util.Collection;
import java.util.AbstractMap;
import java.util.List;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.Fluent;
import io.fabric8.kubernetes.api.builder.Nested;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.Builder;
import java.util.List;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.util.List;
public class NodeStatusFluentImpl> extends BaseFluent implements NodeStatusFluent{
List> addresses = new ArrayList(); Map allocatable = new HashMap(); Map capacity = new HashMap(); List> conditions = new ArrayList(); VisitableBuilder daemonEndpoints; List> images = new ArrayList(); VisitableBuilder nodeInfo; String phase; Map additionalProperties = new HashMap();
public NodeStatusFluentImpl(){
}
public NodeStatusFluentImpl( NodeStatus instance ){
this.withAddresses(instance.getAddresses()); this.withAllocatable(instance.getAllocatable()); this.withCapacity(instance.getCapacity()); this.withConditions(instance.getConditions()); this.withDaemonEndpoints(instance.getDaemonEndpoints()); this.withImages(instance.getImages()); this.withNodeInfo(instance.getNodeInfo()); this.withPhase(instance.getPhase());
}
public T addToAddresses( NodeAddress ...items){
for (NodeAddress item : items) {NodeAddressBuilder builder = new NodeAddressBuilder(item);_visitables.add(builder);this.addresses.add(builder);} return (T)this;
}
public T removeFromAddresses( NodeAddress ...items){
for (NodeAddress item : items) {NodeAddressBuilder builder = new NodeAddressBuilder(item);_visitables.remove(builder);this.addresses.remove(builder);} return (T)this;
}
public List getAddresses(){
return build(addresses);
}
public T withAddresses( List addresses){
this.addresses.clear();if (addresses != null) {for (NodeAddress item : addresses){this.addToAddresses(item);}} return (T) this;
}
public T withAddresses( NodeAddress ...addresses){
this.addresses.clear(); if (addresses != null) {for (NodeAddress item :addresses){ this.addToAddresses(item);}} return (T) this;
}
public AddressesNested addNewAddresse(){
return new AddressesNestedImpl();
}
public AddressesNested addNewAddresseLike( NodeAddress item){
return new AddressesNestedImpl(item);
}
public T addNewAddresse( String address, String type){
return addToAddresses(new NodeAddress(address, type));
}
public T addToAllocatable( String key, Quantity value){
if(key != null && value != null) {this.allocatable.put(key, value);} return (T)this;
}
public T addToAllocatable( Map map){
if(map != null) { this.allocatable.putAll(map);} return (T)this;
}
public T removeFromAllocatable( String key){
if(key != null) {this.allocatable.remove(key);} return (T)this;
}
public T removeFromAllocatable( Map map){
if(map != null) { for(Object key : map.keySet()) {this.allocatable.remove(key);}} return (T)this;
}
public Map getAllocatable(){
return this.allocatable;
}
public T withAllocatable( Map allocatable){
this.allocatable.clear();if (allocatable != null) {this.allocatable.putAll(allocatable);} return (T) this;
}
public T addToCapacity( String key, Quantity value){
if(key != null && value != null) {this.capacity.put(key, value);} return (T)this;
}
public T addToCapacity( Map map){
if(map != null) { this.capacity.putAll(map);} return (T)this;
}
public T removeFromCapacity( String key){
if(key != null) {this.capacity.remove(key);} return (T)this;
}
public T removeFromCapacity( Map map){
if(map != null) { for(Object key : map.keySet()) {this.capacity.remove(key);}} return (T)this;
}
public Map getCapacity(){
return this.capacity;
}
public T withCapacity( Map capacity){
this.capacity.clear();if (capacity != null) {this.capacity.putAll(capacity);} return (T) this;
}
public T addToConditions( NodeCondition ...items){
for (NodeCondition item : items) {NodeConditionBuilder builder = new NodeConditionBuilder(item);_visitables.add(builder);this.conditions.add(builder);} return (T)this;
}
public T removeFromConditions( NodeCondition ...items){
for (NodeCondition item : items) {NodeConditionBuilder builder = new NodeConditionBuilder(item);_visitables.remove(builder);this.conditions.remove(builder);} return (T)this;
}
public List getConditions(){
return build(conditions);
}
public T withConditions( List conditions){
this.conditions.clear();if (conditions != null) {for (NodeCondition item : conditions){this.addToConditions(item);}} return (T) this;
}
public T withConditions( NodeCondition ...conditions){
this.conditions.clear(); if (conditions != null) {for (NodeCondition item :conditions){ this.addToConditions(item);}} return (T) this;
}
public ConditionsNested addNewCondition(){
return new ConditionsNestedImpl();
}
public ConditionsNested addNewConditionLike( NodeCondition item){
return new ConditionsNestedImpl(item);
}
public NodeDaemonEndpoints getDaemonEndpoints(){
return this.daemonEndpoints!=null?this.daemonEndpoints.build():null;
}
public T withDaemonEndpoints( NodeDaemonEndpoints daemonEndpoints){
if (daemonEndpoints!=null){ this.daemonEndpoints= new NodeDaemonEndpointsBuilder(daemonEndpoints); _visitables.add(this.daemonEndpoints);} return (T) this;
}
public DaemonEndpointsNested withNewDaemonEndpoints(){
return new DaemonEndpointsNestedImpl();
}
public DaemonEndpointsNested withNewDaemonEndpointsLike( NodeDaemonEndpoints item){
return new DaemonEndpointsNestedImpl(item);
}
public DaemonEndpointsNested editDaemonEndpoints(){
return withNewDaemonEndpointsLike(getDaemonEndpoints());
}
public T addToImages( ContainerImage ...items){
for (ContainerImage item : items) {ContainerImageBuilder builder = new ContainerImageBuilder(item);_visitables.add(builder);this.images.add(builder);} return (T)this;
}
public T removeFromImages( ContainerImage ...items){
for (ContainerImage item : items) {ContainerImageBuilder builder = new ContainerImageBuilder(item);_visitables.remove(builder);this.images.remove(builder);} return (T)this;
}
public List getImages(){
return build(images);
}
public T withImages( List images){
this.images.clear();if (images != null) {for (ContainerImage item : images){this.addToImages(item);}} return (T) this;
}
public T withImages( ContainerImage ...images){
this.images.clear(); if (images != null) {for (ContainerImage item :images){ this.addToImages(item);}} return (T) this;
}
public ImagesNested addNewImage(){
return new ImagesNestedImpl();
}
public ImagesNested addNewImageLike( ContainerImage item){
return new ImagesNestedImpl(item);
}
public NodeSystemInfo getNodeInfo(){
return this.nodeInfo!=null?this.nodeInfo.build():null;
}
public T withNodeInfo( NodeSystemInfo nodeInfo){
if (nodeInfo!=null){ this.nodeInfo= new NodeSystemInfoBuilder(nodeInfo); _visitables.add(this.nodeInfo);} return (T) this;
}
public NodeInfoNested withNewNodeInfo(){
return new NodeInfoNestedImpl();
}
public NodeInfoNested withNewNodeInfoLike( NodeSystemInfo item){
return new NodeInfoNestedImpl(item);
}
public NodeInfoNested editNodeInfo(){
return withNewNodeInfoLike(getNodeInfo());
}
public String getPhase(){
return this.phase;
}
public T withPhase( String phase){
this.phase=phase; return (T) this;
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public T addToAdditionalProperties( Map map){
if(map != null) { this.additionalProperties.putAll(map);} return (T)this;
}
public T removeFromAdditionalProperties( String key){
if(key != null) {this.additionalProperties.remove(key);} return (T)this;
}
public T removeFromAdditionalProperties( Map map){
if(map != null) { for(Object key : map.keySet()) {this.additionalProperties.remove(key);}} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
public boolean equals( Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
NodeStatusFluentImpl that = (NodeStatusFluentImpl) o;
if (addresses != null ? !addresses.equals(that.addresses) :that.addresses != null) return false;
if (allocatable != null ? !allocatable.equals(that.allocatable) :that.allocatable != null) return false;
if (capacity != null ? !capacity.equals(that.capacity) :that.capacity != null) return false;
if (conditions != null ? !conditions.equals(that.conditions) :that.conditions != null) return false;
if (daemonEndpoints != null ? !daemonEndpoints.equals(that.daemonEndpoints) :that.daemonEndpoints != null) return false;
if (images != null ? !images.equals(that.images) :that.images != null) return false;
if (nodeInfo != null ? !nodeInfo.equals(that.nodeInfo) :that.nodeInfo != null) return false;
if (phase != null ? !phase.equals(that.phase) :that.phase != null) return false;
if (additionalProperties != null ? !additionalProperties.equals(that.additionalProperties) :that.additionalProperties != null) return false;
return true;
}
public class AddressesNestedImpl extends NodeAddressFluentImpl> implements AddressesNested{
private final NodeAddressBuilder builder;
AddressesNestedImpl (){
this.builder = new NodeAddressBuilder(this);
}
AddressesNestedImpl ( NodeAddress item){
this.builder = new NodeAddressBuilder(this, item);
}
public N and(){
return (N) NodeStatusFluentImpl.this.addToAddresses(builder.build());
}
public N endAddresse(){
return and();
}
}
public class ConditionsNestedImpl extends NodeConditionFluentImpl> implements ConditionsNested{
private final NodeConditionBuilder builder;
ConditionsNestedImpl (){
this.builder = new NodeConditionBuilder(this);
}
ConditionsNestedImpl ( NodeCondition item){
this.builder = new NodeConditionBuilder(this, item);
}
public N endCondition(){
return and();
}
public N and(){
return (N) NodeStatusFluentImpl.this.addToConditions(builder.build());
}
}
public class DaemonEndpointsNestedImpl extends NodeDaemonEndpointsFluentImpl> implements DaemonEndpointsNested{
private final NodeDaemonEndpointsBuilder builder;
DaemonEndpointsNestedImpl (){
this.builder = new NodeDaemonEndpointsBuilder(this);
}
DaemonEndpointsNestedImpl ( NodeDaemonEndpoints item){
this.builder = new NodeDaemonEndpointsBuilder(this, item);
}
public N endDaemonEndpoints(){
return and();
}
public N and(){
return (N) NodeStatusFluentImpl.this.withDaemonEndpoints(builder.build());
}
}
public class ImagesNestedImpl extends ContainerImageFluentImpl> implements ImagesNested{
private final ContainerImageBuilder builder;
ImagesNestedImpl (){
this.builder = new ContainerImageBuilder(this);
}
ImagesNestedImpl ( ContainerImage item){
this.builder = new ContainerImageBuilder(this, item);
}
public N and(){
return (N) NodeStatusFluentImpl.this.addToImages(builder.build());
}
public N endImage(){
return and();
}
}
public class NodeInfoNestedImpl extends NodeSystemInfoFluentImpl> implements NodeInfoNested{
private final NodeSystemInfoBuilder builder;
NodeInfoNestedImpl ( NodeSystemInfo item){
this.builder = new NodeSystemInfoBuilder(this, item);
}
NodeInfoNestedImpl (){
this.builder = new NodeSystemInfoBuilder(this);
}
public N and(){
return (N) NodeStatusFluentImpl.this.withNodeInfo(builder.build());
}
public N endNodeInfo(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy