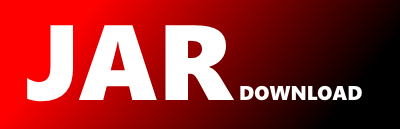
io.fabric8.kubernetes.api.model.ObjectMetaFluentImpl Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import java.util.HashMap;
import java.io.Serializable;
import java.util.Map;
import java.util.Map;
import java.util.Map;
import java.util.AbstractMap;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.fabric8.kubernetes.api.builder.Fluent;
public class ObjectMetaFluentImpl> extends BaseFluent implements ObjectMetaFluent{
Map annotations = new HashMap(); String creationTimestamp; Long deletionGracePeriodSeconds; String deletionTimestamp; String generateName; Long generation; Map labels = new HashMap(); String name; String namespace; String resourceVersion; String selfLink; String uid; Map additionalProperties = new HashMap();
public ObjectMetaFluentImpl(){
}
public ObjectMetaFluentImpl( ObjectMeta instance ){
this.withAnnotations(instance.getAnnotations()); this.withCreationTimestamp(instance.getCreationTimestamp()); this.withDeletionGracePeriodSeconds(instance.getDeletionGracePeriodSeconds()); this.withDeletionTimestamp(instance.getDeletionTimestamp()); this.withGenerateName(instance.getGenerateName()); this.withGeneration(instance.getGeneration()); this.withLabels(instance.getLabels()); this.withName(instance.getName()); this.withNamespace(instance.getNamespace()); this.withResourceVersion(instance.getResourceVersion()); this.withSelfLink(instance.getSelfLink()); this.withUid(instance.getUid());
}
public T addToAnnotations( String key, String value){
if(key != null && value != null) {this.annotations.put(key, value);} return (T)this;
}
public T addToAnnotations( Map map){
if(map != null) { this.annotations.putAll(map);} return (T)this;
}
public T removeFromAnnotations( String key){
if(key != null) {this.annotations.remove(key);} return (T)this;
}
public T removeFromAnnotations( Map map){
if(map != null) { for(Object key : map.keySet()) {this.annotations.remove(key);}} return (T)this;
}
public Map getAnnotations(){
return this.annotations;
}
public T withAnnotations( Map annotations){
this.annotations.clear();if (annotations != null) {this.annotations.putAll(annotations);} return (T) this;
}
public String getCreationTimestamp(){
return this.creationTimestamp;
}
public T withCreationTimestamp( String creationTimestamp){
this.creationTimestamp=creationTimestamp; return (T) this;
}
public Long getDeletionGracePeriodSeconds(){
return this.deletionGracePeriodSeconds;
}
public T withDeletionGracePeriodSeconds( Long deletionGracePeriodSeconds){
this.deletionGracePeriodSeconds=deletionGracePeriodSeconds; return (T) this;
}
public String getDeletionTimestamp(){
return this.deletionTimestamp;
}
public T withDeletionTimestamp( String deletionTimestamp){
this.deletionTimestamp=deletionTimestamp; return (T) this;
}
public String getGenerateName(){
return this.generateName;
}
public T withGenerateName( String generateName){
this.generateName=generateName; return (T) this;
}
public Long getGeneration(){
return this.generation;
}
public T withGeneration( Long generation){
this.generation=generation; return (T) this;
}
public T addToLabels( String key, String value){
if(key != null && value != null) {this.labels.put(key, value);} return (T)this;
}
public T addToLabels( Map map){
if(map != null) { this.labels.putAll(map);} return (T)this;
}
public T removeFromLabels( String key){
if(key != null) {this.labels.remove(key);} return (T)this;
}
public T removeFromLabels( Map map){
if(map != null) { for(Object key : map.keySet()) {this.labels.remove(key);}} return (T)this;
}
public Map getLabels(){
return this.labels;
}
public T withLabels( Map labels){
this.labels.clear();if (labels != null) {this.labels.putAll(labels);} return (T) this;
}
public String getName(){
return this.name;
}
public T withName( String name){
this.name=name; return (T) this;
}
public String getNamespace(){
return this.namespace;
}
public T withNamespace( String namespace){
this.namespace=namespace; return (T) this;
}
public String getResourceVersion(){
return this.resourceVersion;
}
public T withResourceVersion( String resourceVersion){
this.resourceVersion=resourceVersion; return (T) this;
}
public String getSelfLink(){
return this.selfLink;
}
public T withSelfLink( String selfLink){
this.selfLink=selfLink; return (T) this;
}
public String getUid(){
return this.uid;
}
public T withUid( String uid){
this.uid=uid; return (T) this;
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public T addToAdditionalProperties( Map map){
if(map != null) { this.additionalProperties.putAll(map);} return (T)this;
}
public T removeFromAdditionalProperties( String key){
if(key != null) {this.additionalProperties.remove(key);} return (T)this;
}
public T removeFromAdditionalProperties( Map map){
if(map != null) { for(Object key : map.keySet()) {this.additionalProperties.remove(key);}} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
public boolean equals( Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ObjectMetaFluentImpl that = (ObjectMetaFluentImpl) o;
if (annotations != null ? !annotations.equals(that.annotations) :that.annotations != null) return false;
if (creationTimestamp != null ? !creationTimestamp.equals(that.creationTimestamp) :that.creationTimestamp != null) return false;
if (deletionGracePeriodSeconds != null ? !deletionGracePeriodSeconds.equals(that.deletionGracePeriodSeconds) :that.deletionGracePeriodSeconds != null) return false;
if (deletionTimestamp != null ? !deletionTimestamp.equals(that.deletionTimestamp) :that.deletionTimestamp != null) return false;
if (generateName != null ? !generateName.equals(that.generateName) :that.generateName != null) return false;
if (generation != null ? !generation.equals(that.generation) :that.generation != null) return false;
if (labels != null ? !labels.equals(that.labels) :that.labels != null) return false;
if (name != null ? !name.equals(that.name) :that.name != null) return false;
if (namespace != null ? !namespace.equals(that.namespace) :that.namespace != null) return false;
if (resourceVersion != null ? !resourceVersion.equals(that.resourceVersion) :that.resourceVersion != null) return false;
if (selfLink != null ? !selfLink.equals(that.selfLink) :that.selfLink != null) return false;
if (uid != null ? !uid.equals(that.uid) :that.uid != null) return false;
if (additionalProperties != null ? !additionalProperties.equals(that.additionalProperties) :that.additionalProperties != null) return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy