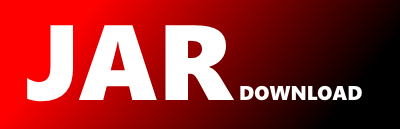
io.fabric8.kubernetes.api.model.PersistentVolumeSpecFluent Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import java.util.ArrayList;
import java.util.RandomAccess;
import java.util.Map;
import java.util.Map;
import java.util.List;
import java.util.List;
import java.util.AbstractCollection;
import java.util.AbstractList;
import java.util.Map;
import java.util.HashMap;
import java.io.Serializable;
import java.util.Collection;
import java.util.AbstractMap;
import io.fabric8.kubernetes.api.builder.Fluent;
import io.fabric8.kubernetes.api.builder.Nested;
public interface PersistentVolumeSpecFluent> extends Fluent{
public T addToAccessModes( String ...items); public T removeFromAccessModes( String ...items); public List getAccessModes(); public T withAccessModes( List accessModes); public T withAccessModes( String ...accessModes); public AWSElasticBlockStoreVolumeSource getAwsElasticBlockStore(); public T withAwsElasticBlockStore( AWSElasticBlockStoreVolumeSource awsElasticBlockStore); public AwsElasticBlockStoreNested withNewAwsElasticBlockStore(); public AwsElasticBlockStoreNested withNewAwsElasticBlockStoreLike( AWSElasticBlockStoreVolumeSource item); public AwsElasticBlockStoreNested editAwsElasticBlockStore(); public T withNewAwsElasticBlockStore( String fsType, Integer partition, Boolean readOnly, String volumeID); public AzureFileVolumeSource getAzureFile(); public T withAzureFile( AzureFileVolumeSource azureFile); public AzureFileNested withNewAzureFile(); public AzureFileNested withNewAzureFileLike( AzureFileVolumeSource item); public AzureFileNested editAzureFile(); public T withNewAzureFile( Boolean readOnly, String secretName, String shareName); public T addToCapacity( String key, Quantity value); public T addToCapacity( Map map); public T removeFromCapacity( String key); public T removeFromCapacity( Map map); public Map getCapacity(); public T withCapacity( Map capacity); public CephFSVolumeSource getCephfs(); public T withCephfs( CephFSVolumeSource cephfs); public CephfsNested withNewCephfs(); public CephfsNested withNewCephfsLike( CephFSVolumeSource item); public CephfsNested editCephfs(); public CinderVolumeSource getCinder(); public T withCinder( CinderVolumeSource cinder); public CinderNested withNewCinder(); public CinderNested withNewCinderLike( CinderVolumeSource item); public CinderNested editCinder(); public T withNewCinder( String fsType, Boolean readOnly, String volumeID); public ObjectReference getClaimRef(); public T withClaimRef( ObjectReference claimRef); public ClaimRefNested withNewClaimRef(); public ClaimRefNested withNewClaimRefLike( ObjectReference item); public ClaimRefNested editClaimRef(); public FCVolumeSource getFc(); public T withFc( FCVolumeSource fc); public FcNested withNewFc(); public FcNested withNewFcLike( FCVolumeSource item); public FcNested editFc(); public FlexVolumeSource getFlexVolume(); public T withFlexVolume( FlexVolumeSource flexVolume); public FlexVolumeNested withNewFlexVolume(); public FlexVolumeNested withNewFlexVolumeLike( FlexVolumeSource item); public FlexVolumeNested editFlexVolume(); public FlockerVolumeSource getFlocker(); public T withFlocker( FlockerVolumeSource flocker); public FlockerNested withNewFlocker(); public FlockerNested withNewFlockerLike( FlockerVolumeSource item); public FlockerNested editFlocker(); public T withNewFlocker( String datasetName); public GCEPersistentDiskVolumeSource getGcePersistentDisk(); public T withGcePersistentDisk( GCEPersistentDiskVolumeSource gcePersistentDisk); public GcePersistentDiskNested withNewGcePersistentDisk(); public GcePersistentDiskNested withNewGcePersistentDiskLike( GCEPersistentDiskVolumeSource item); public GcePersistentDiskNested editGcePersistentDisk(); public T withNewGcePersistentDisk( String fsType, Integer partition, String pdName, Boolean readOnly); public GlusterfsVolumeSource getGlusterfs(); public T withGlusterfs( GlusterfsVolumeSource glusterfs); public GlusterfsNested withNewGlusterfs(); public GlusterfsNested withNewGlusterfsLike( GlusterfsVolumeSource item); public GlusterfsNested editGlusterfs(); public T withNewGlusterfs( String endpoints, String path, Boolean readOnly); public HostPathVolumeSource getHostPath(); public T withHostPath( HostPathVolumeSource hostPath); public HostPathNested withNewHostPath(); public HostPathNested withNewHostPathLike( HostPathVolumeSource item); public HostPathNested editHostPath(); public T withNewHostPath( String path); public ISCSIVolumeSource getIscsi(); public T withIscsi( ISCSIVolumeSource iscsi); public IscsiNested withNewIscsi(); public IscsiNested withNewIscsiLike( ISCSIVolumeSource item); public IscsiNested editIscsi(); public NFSVolumeSource getNfs(); public T withNfs( NFSVolumeSource nfs); public NfsNested withNewNfs(); public NfsNested withNewNfsLike( NFSVolumeSource item); public NfsNested editNfs(); public T withNewNfs( String path, Boolean readOnly, String server); public String getPersistentVolumeReclaimPolicy(); public T withPersistentVolumeReclaimPolicy( String persistentVolumeReclaimPolicy); public RBDVolumeSource getRbd(); public T withRbd( RBDVolumeSource rbd); public RbdNested withNewRbd(); public RbdNested withNewRbdLike( RBDVolumeSource item); public RbdNested editRbd(); public T addToAdditionalProperties( String key, Object value); public T addToAdditionalProperties( Map map); public T removeFromAdditionalProperties( String key); public T removeFromAdditionalProperties( Map map); public Map getAdditionalProperties(); public T withAdditionalProperties( Map additionalProperties);
public interface AwsElasticBlockStoreNested extends Nested, AWSElasticBlockStoreVolumeSourceFluent>{
public N endAwsElasticBlockStore(); public N and();
}
public interface AzureFileNested extends Nested, AzureFileVolumeSourceFluent>{
public N endAzureFile(); public N and();
}
public interface CephfsNested extends Nested, CephFSVolumeSourceFluent>{
public N endCephfs(); public N and();
}
public interface CinderNested extends Nested, CinderVolumeSourceFluent>{
public N and(); public N endCinder();
}
public interface ClaimRefNested extends Nested, ObjectReferenceFluent>{
public N and(); public N endClaimRef();
}
public interface FcNested extends Nested, FCVolumeSourceFluent>{
public N and(); public N endFc();
}
public interface FlexVolumeNested extends Nested, FlexVolumeSourceFluent>{
public N and(); public N endFlexVolume();
}
public interface FlockerNested extends Nested, FlockerVolumeSourceFluent>{
public N and(); public N endFlocker();
}
public interface GcePersistentDiskNested extends Nested, GCEPersistentDiskVolumeSourceFluent>{
public N endGcePersistentDisk(); public N and();
}
public interface GlusterfsNested extends Nested, GlusterfsVolumeSourceFluent>{
public N and(); public N endGlusterfs();
}
public interface HostPathNested extends Nested, HostPathVolumeSourceFluent>{
public N and(); public N endHostPath();
}
public interface IscsiNested extends Nested, ISCSIVolumeSourceFluent>{
public N and(); public N endIscsi();
}
public interface NfsNested extends Nested, NFSVolumeSourceFluent>{
public N endNfs(); public N and();
}
public interface RbdNested extends Nested, RBDVolumeSourceFluent>{
public N and(); public N endRbd();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy