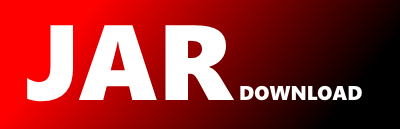
io.fabric8.kubernetes.api.model.ServiceSpecFluentImpl Maven / Gradle / Ivy
package io.fabric8.kubernetes.api.model;
import java.util.ArrayList;
import java.util.RandomAccess;
import java.util.Map;
import java.util.Map;
import java.util.List;
import java.util.List;
import java.util.AbstractCollection;
import java.util.List;
import java.util.AbstractList;
import java.util.HashMap;
import java.io.Serializable;
import java.util.Map;
import java.util.Collection;
import java.util.AbstractMap;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.Builder;
import java.util.List;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.fabric8.kubernetes.api.builder.Fluent;
import io.fabric8.kubernetes.api.builder.Nested;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
public class ServiceSpecFluentImpl> extends BaseFluent implements ServiceSpecFluent{
String clusterIP; List deprecatedPublicIPs = new ArrayList(); List externalIPs = new ArrayList(); String loadBalancerIP; String portalIP; List> ports = new ArrayList(); Map selector = new HashMap(); String sessionAffinity; String type; Map additionalProperties = new HashMap();
public ServiceSpecFluentImpl(){
}
public ServiceSpecFluentImpl( ServiceSpec instance ){
this.withClusterIP(instance.getClusterIP()); this.withDeprecatedPublicIPs(instance.getDeprecatedPublicIPs()); this.withExternalIPs(instance.getExternalIPs()); this.withLoadBalancerIP(instance.getLoadBalancerIP()); this.withPortalIP(instance.getPortalIP()); this.withPorts(instance.getPorts()); this.withSelector(instance.getSelector()); this.withSessionAffinity(instance.getSessionAffinity()); this.withType(instance.getType());
}
public String getClusterIP(){
return this.clusterIP;
}
public T withClusterIP( String clusterIP){
this.clusterIP=clusterIP; return (T) this;
}
public T addToDeprecatedPublicIPs( String ...items){
for (String item : items) {this.deprecatedPublicIPs.add(item);} return (T)this;
}
public T removeFromDeprecatedPublicIPs( String ...items){
for (String item : items) {this.deprecatedPublicIPs.remove(item);} return (T)this;
}
public List getDeprecatedPublicIPs(){
return this.deprecatedPublicIPs;
}
public T withDeprecatedPublicIPs( List deprecatedPublicIPs){
this.deprecatedPublicIPs.clear();if (deprecatedPublicIPs != null) {for (String item : deprecatedPublicIPs){this.addToDeprecatedPublicIPs(item);}} return (T) this;
}
public T withDeprecatedPublicIPs( String ...deprecatedPublicIPs){
this.deprecatedPublicIPs.clear(); if (deprecatedPublicIPs != null) {for (String item :deprecatedPublicIPs){ this.addToDeprecatedPublicIPs(item);}} return (T) this;
}
public T addToExternalIPs( String ...items){
for (String item : items) {this.externalIPs.add(item);} return (T)this;
}
public T removeFromExternalIPs( String ...items){
for (String item : items) {this.externalIPs.remove(item);} return (T)this;
}
public List getExternalIPs(){
return this.externalIPs;
}
public T withExternalIPs( List externalIPs){
this.externalIPs.clear();if (externalIPs != null) {for (String item : externalIPs){this.addToExternalIPs(item);}} return (T) this;
}
public T withExternalIPs( String ...externalIPs){
this.externalIPs.clear(); if (externalIPs != null) {for (String item :externalIPs){ this.addToExternalIPs(item);}} return (T) this;
}
public String getLoadBalancerIP(){
return this.loadBalancerIP;
}
public T withLoadBalancerIP( String loadBalancerIP){
this.loadBalancerIP=loadBalancerIP; return (T) this;
}
public String getPortalIP(){
return this.portalIP;
}
public T withPortalIP( String portalIP){
this.portalIP=portalIP; return (T) this;
}
public T addToPorts( ServicePort ...items){
for (ServicePort item : items) {ServicePortBuilder builder = new ServicePortBuilder(item);_visitables.add(builder);this.ports.add(builder);} return (T)this;
}
public T removeFromPorts( ServicePort ...items){
for (ServicePort item : items) {ServicePortBuilder builder = new ServicePortBuilder(item);_visitables.remove(builder);this.ports.remove(builder);} return (T)this;
}
public List getPorts(){
return build(ports);
}
public T withPorts( List ports){
this.ports.clear();if (ports != null) {for (ServicePort item : ports){this.addToPorts(item);}} return (T) this;
}
public T withPorts( ServicePort ...ports){
this.ports.clear(); if (ports != null) {for (ServicePort item :ports){ this.addToPorts(item);}} return (T) this;
}
public PortsNested addNewPort(){
return new PortsNestedImpl();
}
public PortsNested addNewPortLike( ServicePort item){
return new PortsNestedImpl(item);
}
public T addToSelector( String key, String value){
if(key != null && value != null) {this.selector.put(key, value);} return (T)this;
}
public T addToSelector( Map map){
if(map != null) { this.selector.putAll(map);} return (T)this;
}
public T removeFromSelector( String key){
if(key != null) {this.selector.remove(key);} return (T)this;
}
public T removeFromSelector( Map map){
if(map != null) { for(Object key : map.keySet()) {this.selector.remove(key);}} return (T)this;
}
public Map getSelector(){
return this.selector;
}
public T withSelector( Map selector){
this.selector.clear();if (selector != null) {this.selector.putAll(selector);} return (T) this;
}
public String getSessionAffinity(){
return this.sessionAffinity;
}
public T withSessionAffinity( String sessionAffinity){
this.sessionAffinity=sessionAffinity; return (T) this;
}
public String getType(){
return this.type;
}
public T withType( String type){
this.type=type; return (T) this;
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public T addToAdditionalProperties( Map map){
if(map != null) { this.additionalProperties.putAll(map);} return (T)this;
}
public T removeFromAdditionalProperties( String key){
if(key != null) {this.additionalProperties.remove(key);} return (T)this;
}
public T removeFromAdditionalProperties( Map map){
if(map != null) { for(Object key : map.keySet()) {this.additionalProperties.remove(key);}} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
public boolean equals( Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ServiceSpecFluentImpl that = (ServiceSpecFluentImpl) o;
if (clusterIP != null ? !clusterIP.equals(that.clusterIP) :that.clusterIP != null) return false;
if (deprecatedPublicIPs != null ? !deprecatedPublicIPs.equals(that.deprecatedPublicIPs) :that.deprecatedPublicIPs != null) return false;
if (externalIPs != null ? !externalIPs.equals(that.externalIPs) :that.externalIPs != null) return false;
if (loadBalancerIP != null ? !loadBalancerIP.equals(that.loadBalancerIP) :that.loadBalancerIP != null) return false;
if (portalIP != null ? !portalIP.equals(that.portalIP) :that.portalIP != null) return false;
if (ports != null ? !ports.equals(that.ports) :that.ports != null) return false;
if (selector != null ? !selector.equals(that.selector) :that.selector != null) return false;
if (sessionAffinity != null ? !sessionAffinity.equals(that.sessionAffinity) :that.sessionAffinity != null) return false;
if (type != null ? !type.equals(that.type) :that.type != null) return false;
if (additionalProperties != null ? !additionalProperties.equals(that.additionalProperties) :that.additionalProperties != null) return false;
return true;
}
public class PortsNestedImpl extends ServicePortFluentImpl> implements PortsNested{
private final ServicePortBuilder builder;
PortsNestedImpl (){
this.builder = new ServicePortBuilder(this);
}
PortsNestedImpl ( ServicePort item){
this.builder = new ServicePortBuilder(this, item);
}
public N endPort(){
return and();
}
public N and(){
return (N) ServiceSpecFluentImpl.this.addToPorts(builder.build());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy