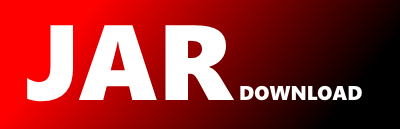
io.fabric8.openshift.api.model.DeploymentStrategyFluentImpl Maven / Gradle / Ivy
package io.fabric8.openshift.api.model;
import io.fabric8.kubernetes.api.model.ResourceRequirements;
import java.util.Map;
import java.util.Map;
import java.util.HashMap;
import java.io.Serializable;
import java.util.Map;
import java.util.AbstractMap;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.fabric8.kubernetes.api.model.ResourceRequirementsFluentImpl;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.Fluent;
import io.fabric8.kubernetes.api.model.ResourceRequirementsFluent;
import io.fabric8.kubernetes.api.builder.Nested;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.Builder;
import io.fabric8.kubernetes.api.model.ResourceRequirementsBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
public class DeploymentStrategyFluentImpl> extends BaseFluent implements DeploymentStrategyFluent{
Map annotations = new HashMap(); VisitableBuilder customParams; Map labels = new HashMap(); VisitableBuilder recreateParams; VisitableBuilder resources; VisitableBuilder rollingParams; String type; Map additionalProperties = new HashMap();
public DeploymentStrategyFluentImpl(){
}
public DeploymentStrategyFluentImpl( DeploymentStrategy instance ){
this.withAnnotations(instance.getAnnotations()); this.withCustomParams(instance.getCustomParams()); this.withLabels(instance.getLabels()); this.withRecreateParams(instance.getRecreateParams()); this.withResources(instance.getResources()); this.withRollingParams(instance.getRollingParams()); this.withType(instance.getType());
}
public T addToAnnotations( String key, String value){
if(key != null && value != null) {this.annotations.put(key, value);} return (T)this;
}
public T addToAnnotations( Map map){
if(map != null) { this.annotations.putAll(map);} return (T)this;
}
public T removeFromAnnotations( String key){
if(key != null) {this.annotations.remove(key);} return (T)this;
}
public T removeFromAnnotations( Map map){
if(map != null) { for(Object key : map.keySet()) {this.annotations.remove(key);}} return (T)this;
}
public Map getAnnotations(){
return this.annotations;
}
public T withAnnotations( Map annotations){
this.annotations.clear();if (annotations != null) {this.annotations.putAll(annotations);} return (T) this;
}
public CustomDeploymentStrategyParams getCustomParams(){
return this.customParams!=null?this.customParams.build():null;
}
public T withCustomParams( CustomDeploymentStrategyParams customParams){
if (customParams!=null){ this.customParams= new CustomDeploymentStrategyParamsBuilder(customParams); _visitables.add(this.customParams);} return (T) this;
}
public CustomParamsNested withNewCustomParams(){
return new CustomParamsNestedImpl();
}
public CustomParamsNested withNewCustomParamsLike( CustomDeploymentStrategyParams item){
return new CustomParamsNestedImpl(item);
}
public CustomParamsNested editCustomParams(){
return withNewCustomParamsLike(getCustomParams());
}
public T addToLabels( String key, String value){
if(key != null && value != null) {this.labels.put(key, value);} return (T)this;
}
public T addToLabels( Map map){
if(map != null) { this.labels.putAll(map);} return (T)this;
}
public T removeFromLabels( String key){
if(key != null) {this.labels.remove(key);} return (T)this;
}
public T removeFromLabels( Map map){
if(map != null) { for(Object key : map.keySet()) {this.labels.remove(key);}} return (T)this;
}
public Map getLabels(){
return this.labels;
}
public T withLabels( Map labels){
this.labels.clear();if (labels != null) {this.labels.putAll(labels);} return (T) this;
}
public RecreateDeploymentStrategyParams getRecreateParams(){
return this.recreateParams!=null?this.recreateParams.build():null;
}
public T withRecreateParams( RecreateDeploymentStrategyParams recreateParams){
if (recreateParams!=null){ this.recreateParams= new RecreateDeploymentStrategyParamsBuilder(recreateParams); _visitables.add(this.recreateParams);} return (T) this;
}
public RecreateParamsNested withNewRecreateParams(){
return new RecreateParamsNestedImpl();
}
public RecreateParamsNested withNewRecreateParamsLike( RecreateDeploymentStrategyParams item){
return new RecreateParamsNestedImpl(item);
}
public RecreateParamsNested editRecreateParams(){
return withNewRecreateParamsLike(getRecreateParams());
}
public ResourceRequirements getResources(){
return this.resources!=null?this.resources.build():null;
}
public T withResources( ResourceRequirements resources){
if (resources!=null){ this.resources= new ResourceRequirementsBuilder(resources); _visitables.add(this.resources);} return (T) this;
}
public ResourcesNested withNewResources(){
return new ResourcesNestedImpl();
}
public ResourcesNested withNewResourcesLike( ResourceRequirements item){
return new ResourcesNestedImpl(item);
}
public ResourcesNested editResources(){
return withNewResourcesLike(getResources());
}
public RollingDeploymentStrategyParams getRollingParams(){
return this.rollingParams!=null?this.rollingParams.build():null;
}
public T withRollingParams( RollingDeploymentStrategyParams rollingParams){
if (rollingParams!=null){ this.rollingParams= new RollingDeploymentStrategyParamsBuilder(rollingParams); _visitables.add(this.rollingParams);} return (T) this;
}
public RollingParamsNested withNewRollingParams(){
return new RollingParamsNestedImpl();
}
public RollingParamsNested withNewRollingParamsLike( RollingDeploymentStrategyParams item){
return new RollingParamsNestedImpl(item);
}
public RollingParamsNested editRollingParams(){
return withNewRollingParamsLike(getRollingParams());
}
public String getType(){
return this.type;
}
public T withType( String type){
this.type=type; return (T) this;
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public T addToAdditionalProperties( Map map){
if(map != null) { this.additionalProperties.putAll(map);} return (T)this;
}
public T removeFromAdditionalProperties( String key){
if(key != null) {this.additionalProperties.remove(key);} return (T)this;
}
public T removeFromAdditionalProperties( Map map){
if(map != null) { for(Object key : map.keySet()) {this.additionalProperties.remove(key);}} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
public boolean equals( Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
DeploymentStrategyFluentImpl that = (DeploymentStrategyFluentImpl) o;
if (annotations != null ? !annotations.equals(that.annotations) :that.annotations != null) return false;
if (customParams != null ? !customParams.equals(that.customParams) :that.customParams != null) return false;
if (labels != null ? !labels.equals(that.labels) :that.labels != null) return false;
if (recreateParams != null ? !recreateParams.equals(that.recreateParams) :that.recreateParams != null) return false;
if (resources != null ? !resources.equals(that.resources) :that.resources != null) return false;
if (rollingParams != null ? !rollingParams.equals(that.rollingParams) :that.rollingParams != null) return false;
if (type != null ? !type.equals(that.type) :that.type != null) return false;
if (additionalProperties != null ? !additionalProperties.equals(that.additionalProperties) :that.additionalProperties != null) return false;
return true;
}
public class CustomParamsNestedImpl extends CustomDeploymentStrategyParamsFluentImpl> implements CustomParamsNested{
private final CustomDeploymentStrategyParamsBuilder builder;
CustomParamsNestedImpl (){
this.builder = new CustomDeploymentStrategyParamsBuilder(this);
}
CustomParamsNestedImpl ( CustomDeploymentStrategyParams item){
this.builder = new CustomDeploymentStrategyParamsBuilder(this, item);
}
public N endCustomParams(){
return and();
}
public N and(){
return (N) DeploymentStrategyFluentImpl.this.withCustomParams(builder.build());
}
}
public class RecreateParamsNestedImpl extends RecreateDeploymentStrategyParamsFluentImpl> implements RecreateParamsNested{
private final RecreateDeploymentStrategyParamsBuilder builder;
RecreateParamsNestedImpl (){
this.builder = new RecreateDeploymentStrategyParamsBuilder(this);
}
RecreateParamsNestedImpl ( RecreateDeploymentStrategyParams item){
this.builder = new RecreateDeploymentStrategyParamsBuilder(this, item);
}
public N and(){
return (N) DeploymentStrategyFluentImpl.this.withRecreateParams(builder.build());
}
public N endRecreateParams(){
return and();
}
}
public class ResourcesNestedImpl extends ResourceRequirementsFluentImpl> implements ResourcesNested{
private final ResourceRequirementsBuilder builder;
ResourcesNestedImpl (){
this.builder = new ResourceRequirementsBuilder(this);
}
ResourcesNestedImpl ( ResourceRequirements item){
this.builder = new ResourceRequirementsBuilder(this, item);
}
public N and(){
return (N) DeploymentStrategyFluentImpl.this.withResources(builder.build());
}
public N endResources(){
return and();
}
}
public class RollingParamsNestedImpl extends RollingDeploymentStrategyParamsFluentImpl> implements RollingParamsNested{
private final RollingDeploymentStrategyParamsBuilder builder;
RollingParamsNestedImpl (){
this.builder = new RollingDeploymentStrategyParamsBuilder(this);
}
RollingParamsNestedImpl ( RollingDeploymentStrategyParams item){
this.builder = new RollingDeploymentStrategyParamsBuilder(this, item);
}
public N and(){
return (N) DeploymentStrategyFluentImpl.this.withRollingParams(builder.build());
}
public N endRollingParams(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy