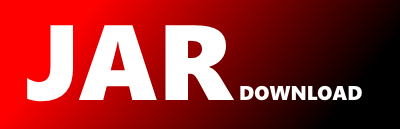
io.fabric8.openshift.api.model.PolicyRuleFluentImpl Maven / Gradle / Ivy
package io.fabric8.openshift.api.model;
import java.util.ArrayList;
import java.util.RandomAccess;
import io.fabric8.kubernetes.api.model.HasMetadata;
import java.util.Map;
import io.fabric8.kubernetes.api.model.KubernetesResource;
import java.util.Map;
import java.util.List;
import java.util.List;
import java.util.AbstractCollection;
import java.util.AbstractList;
import java.util.HashMap;
import java.io.Serializable;
import java.util.Collection;
import java.util.AbstractMap;
import io.fabric8.kubernetes.api.builder.Visitable;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.fabric8.kubernetes.api.builder.Fluent;
public class PolicyRuleFluentImpl> extends BaseFluent implements PolicyRuleFluent{
List apiGroups = new ArrayList(); HasMetadata attributeRestrictions; List nonResourceURLs = new ArrayList(); List resourceNames = new ArrayList(); List resources = new ArrayList(); List verbs = new ArrayList(); Map additionalProperties = new HashMap();
public PolicyRuleFluentImpl(){
}
public PolicyRuleFluentImpl( PolicyRule instance ){
this.withApiGroups(instance.getApiGroups()); this.withAttributeRestrictions(instance.getAttributeRestrictions()); this.withNonResourceURLs(instance.getNonResourceURLs()); this.withResourceNames(instance.getResourceNames()); this.withResources(instance.getResources()); this.withVerbs(instance.getVerbs());
}
public T addToApiGroups( String ...items){
for (String item : items) {this.apiGroups.add(item);} return (T)this;
}
public T removeFromApiGroups( String ...items){
for (String item : items) {this.apiGroups.remove(item);} return (T)this;
}
public List getApiGroups(){
return this.apiGroups;
}
public T withApiGroups( List apiGroups){
this.apiGroups.clear();if (apiGroups != null) {for (String item : apiGroups){this.addToApiGroups(item);}} return (T) this;
}
public T withApiGroups( String ...apiGroups){
this.apiGroups.clear(); if (apiGroups != null) {for (String item :apiGroups){ this.addToApiGroups(item);}} return (T) this;
}
public HasMetadata getAttributeRestrictions(){
return this.attributeRestrictions;
}
public T withAttributeRestrictions( HasMetadata attributeRestrictions){
this.attributeRestrictions=attributeRestrictions; return (T) this;
}
public T addToNonResourceURLs( String ...items){
for (String item : items) {this.nonResourceURLs.add(item);} return (T)this;
}
public T removeFromNonResourceURLs( String ...items){
for (String item : items) {this.nonResourceURLs.remove(item);} return (T)this;
}
public List getNonResourceURLs(){
return this.nonResourceURLs;
}
public T withNonResourceURLs( List nonResourceURLs){
this.nonResourceURLs.clear();if (nonResourceURLs != null) {for (String item : nonResourceURLs){this.addToNonResourceURLs(item);}} return (T) this;
}
public T withNonResourceURLs( String ...nonResourceURLs){
this.nonResourceURLs.clear(); if (nonResourceURLs != null) {for (String item :nonResourceURLs){ this.addToNonResourceURLs(item);}} return (T) this;
}
public T addToResourceNames( String ...items){
for (String item : items) {this.resourceNames.add(item);} return (T)this;
}
public T removeFromResourceNames( String ...items){
for (String item : items) {this.resourceNames.remove(item);} return (T)this;
}
public List getResourceNames(){
return this.resourceNames;
}
public T withResourceNames( List resourceNames){
this.resourceNames.clear();if (resourceNames != null) {for (String item : resourceNames){this.addToResourceNames(item);}} return (T) this;
}
public T withResourceNames( String ...resourceNames){
this.resourceNames.clear(); if (resourceNames != null) {for (String item :resourceNames){ this.addToResourceNames(item);}} return (T) this;
}
public T addToResources( String ...items){
for (String item : items) {this.resources.add(item);} return (T)this;
}
public T removeFromResources( String ...items){
for (String item : items) {this.resources.remove(item);} return (T)this;
}
public List getResources(){
return this.resources;
}
public T withResources( List resources){
this.resources.clear();if (resources != null) {for (String item : resources){this.addToResources(item);}} return (T) this;
}
public T withResources( String ...resources){
this.resources.clear(); if (resources != null) {for (String item :resources){ this.addToResources(item);}} return (T) this;
}
public T addToVerbs( String ...items){
for (String item : items) {this.verbs.add(item);} return (T)this;
}
public T removeFromVerbs( String ...items){
for (String item : items) {this.verbs.remove(item);} return (T)this;
}
public List getVerbs(){
return this.verbs;
}
public T withVerbs( List verbs){
this.verbs.clear();if (verbs != null) {for (String item : verbs){this.addToVerbs(item);}} return (T) this;
}
public T withVerbs( String ...verbs){
this.verbs.clear(); if (verbs != null) {for (String item :verbs){ this.addToVerbs(item);}} return (T) this;
}
public T addToAdditionalProperties( String key, Object value){
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (T)this;
}
public T addToAdditionalProperties( Map map){
if(map != null) { this.additionalProperties.putAll(map);} return (T)this;
}
public T removeFromAdditionalProperties( String key){
if(key != null) {this.additionalProperties.remove(key);} return (T)this;
}
public T removeFromAdditionalProperties( Map map){
if(map != null) { for(Object key : map.keySet()) {this.additionalProperties.remove(key);}} return (T)this;
}
public Map getAdditionalProperties(){
return this.additionalProperties;
}
public T withAdditionalProperties( Map additionalProperties){
this.additionalProperties.clear();if (additionalProperties != null) {this.additionalProperties.putAll(additionalProperties);} return (T) this;
}
public boolean equals( Object o){
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
PolicyRuleFluentImpl that = (PolicyRuleFluentImpl) o;
if (apiGroups != null ? !apiGroups.equals(that.apiGroups) :that.apiGroups != null) return false;
if (attributeRestrictions != null ? !attributeRestrictions.equals(that.attributeRestrictions) :that.attributeRestrictions != null) return false;
if (nonResourceURLs != null ? !nonResourceURLs.equals(that.nonResourceURLs) :that.nonResourceURLs != null) return false;
if (resourceNames != null ? !resourceNames.equals(that.resourceNames) :that.resourceNames != null) return false;
if (resources != null ? !resources.equals(that.resources) :that.resources != null) return false;
if (verbs != null ? !verbs.equals(that.verbs) :that.verbs != null) return false;
if (additionalProperties != null ? !additionalProperties.equals(that.additionalProperties) :that.additionalProperties != null) return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy