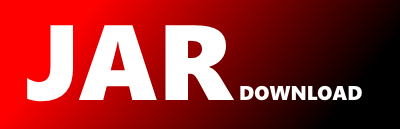
io.fabric8.openshift.api.model.machine.v1alpha1.NetworkParamFluent Maven / Gradle / Ivy
package io.fabric8.openshift.api.model.machine.v1alpha1;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.Nested;
import java.util.ArrayList;
import java.lang.String;
import java.util.LinkedHashMap;
import java.util.function.Predicate;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.Iterator;
import java.util.List;
import java.lang.Boolean;
import java.util.Collection;
import java.lang.Object;
import java.util.Map;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class NetworkParamFluent> extends BaseFluent{
public NetworkParamFluent() {
}
public NetworkParamFluent(NetworkParam instance) {
this.copyInstance(instance);
}
private FilterBuilder filter;
private String fixedIp;
private Boolean noAllowedAddressPairs;
private Boolean portSecurity;
private List portTags = new ArrayList();
private Map profile;
private ArrayList subnets = new ArrayList();
private String uuid;
private String vnicType;
private Map additionalProperties;
protected void copyInstance(NetworkParam instance) {
instance = (instance != null ? instance : new NetworkParam());
if (instance != null) {
this.withFilter(instance.getFilter());
this.withFixedIp(instance.getFixedIp());
this.withNoAllowedAddressPairs(instance.getNoAllowedAddressPairs());
this.withPortSecurity(instance.getPortSecurity());
this.withPortTags(instance.getPortTags());
this.withProfile(instance.getProfile());
this.withSubnets(instance.getSubnets());
this.withUuid(instance.getUuid());
this.withVnicType(instance.getVnicType());
this.withFilter(instance.getFilter());
this.withFixedIp(instance.getFixedIp());
this.withNoAllowedAddressPairs(instance.getNoAllowedAddressPairs());
this.withPortSecurity(instance.getPortSecurity());
this.withPortTags(instance.getPortTags());
this.withProfile(instance.getProfile());
this.withSubnets(instance.getSubnets());
this.withUuid(instance.getUuid());
this.withVnicType(instance.getVnicType());
this.withAdditionalProperties(instance.getAdditionalProperties());
}
}
public Filter buildFilter() {
return this.filter!=null ?this.filter.build():null;
}
public A withFilter(Filter filter) {
_visitables.get("filter").remove(this.filter);
if (filter!=null){ this.filter= new FilterBuilder(filter); _visitables.get("filter").add(this.filter);} else { this.filter = null; _visitables.get("filter").remove(this.filter); } return (A) this;
}
public boolean hasFilter() {
return this.filter != null;
}
public FilterNested withNewFilter() {
return new FilterNested(null);
}
public FilterNested withNewFilterLike(Filter item) {
return new FilterNested(item);
}
public FilterNested editFilter() {
return withNewFilterLike(java.util.Optional.ofNullable(buildFilter()).orElse(null));
}
public FilterNested editOrNewFilter() {
return withNewFilterLike(java.util.Optional.ofNullable(buildFilter()).orElse(new FilterBuilder().build()));
}
public FilterNested editOrNewFilterLike(Filter item) {
return withNewFilterLike(java.util.Optional.ofNullable(buildFilter()).orElse(item));
}
public String getFixedIp() {
return this.fixedIp;
}
public A withFixedIp(String fixedIp) {
this.fixedIp=fixedIp; return (A) this;
}
public boolean hasFixedIp() {
return this.fixedIp != null;
}
public Boolean getNoAllowedAddressPairs() {
return this.noAllowedAddressPairs;
}
public A withNoAllowedAddressPairs(Boolean noAllowedAddressPairs) {
this.noAllowedAddressPairs=noAllowedAddressPairs; return (A) this;
}
public boolean hasNoAllowedAddressPairs() {
return this.noAllowedAddressPairs != null;
}
public Boolean getPortSecurity() {
return this.portSecurity;
}
public A withPortSecurity(Boolean portSecurity) {
this.portSecurity=portSecurity; return (A) this;
}
public boolean hasPortSecurity() {
return this.portSecurity != null;
}
public A addToPortTags(int index,String item) {
if (this.portTags == null) {this.portTags = new ArrayList();}
this.portTags.add(index, item);
return (A)this;
}
public A setToPortTags(int index,String item) {
if (this.portTags == null) {this.portTags = new ArrayList();}
this.portTags.set(index, item); return (A)this;
}
public A addToPortTags(java.lang.String... items) {
if (this.portTags == null) {this.portTags = new ArrayList();}
for (String item : items) {this.portTags.add(item);} return (A)this;
}
public A addAllToPortTags(Collection items) {
if (this.portTags == null) {this.portTags = new ArrayList();}
for (String item : items) {this.portTags.add(item);} return (A)this;
}
public A removeFromPortTags(java.lang.String... items) {
if (this.portTags == null) return (A)this;
for (String item : items) { this.portTags.remove(item);} return (A)this;
}
public A removeAllFromPortTags(Collection items) {
if (this.portTags == null) return (A)this;
for (String item : items) { this.portTags.remove(item);} return (A)this;
}
public List getPortTags() {
return this.portTags;
}
public String getPortTag(int index) {
return this.portTags.get(index);
}
public String getFirstPortTag() {
return this.portTags.get(0);
}
public String getLastPortTag() {
return this.portTags.get(portTags.size() - 1);
}
public String getMatchingPortTag(Predicate predicate) {
for (String item: portTags) { if(predicate.test(item)){ return item;} } return null;
}
public boolean hasMatchingPortTag(Predicate predicate) {
for (String item: portTags) { if(predicate.test(item)){ return true;} } return false;
}
public A withPortTags(List portTags) {
if (portTags != null) {this.portTags = new ArrayList(); for (String item : portTags){this.addToPortTags(item);}} else { this.portTags = null;} return (A) this;
}
public A withPortTags(java.lang.String... portTags) {
if (this.portTags != null) {this.portTags.clear(); _visitables.remove("portTags"); }
if (portTags != null) {for (String item :portTags){ this.addToPortTags(item);}} return (A) this;
}
public boolean hasPortTags() {
return portTags != null && !portTags.isEmpty();
}
public A addToProfile(String key,String value) {
if(this.profile == null && key != null && value != null) { this.profile = new LinkedHashMap(); }
if(key != null && value != null) {this.profile.put(key, value);} return (A)this;
}
public A addToProfile(Map map) {
if(this.profile == null && map != null) { this.profile = new LinkedHashMap(); }
if(map != null) { this.profile.putAll(map);} return (A)this;
}
public A removeFromProfile(String key) {
if(this.profile == null) { return (A) this; }
if(key != null && this.profile != null) {this.profile.remove(key);} return (A)this;
}
public A removeFromProfile(Map map) {
if(this.profile == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.profile != null){this.profile.remove(key);}}} return (A)this;
}
public Map getProfile() {
return this.profile;
}
public A withProfile(Map profile) {
if (profile == null) { this.profile = null;} else {this.profile = new LinkedHashMap(profile);} return (A) this;
}
public boolean hasProfile() {
return this.profile != null;
}
public A addToSubnets(int index,SubnetParam item) {
if (this.subnets == null) {this.subnets = new ArrayList();}
SubnetParamBuilder builder = new SubnetParamBuilder(item);
if (index < 0 || index >= subnets.size()) { _visitables.get("subnets").add(builder); subnets.add(builder); } else { _visitables.get("subnets").add(index, builder); subnets.add(index, builder);}
return (A)this;
}
public A setToSubnets(int index,SubnetParam item) {
if (this.subnets == null) {this.subnets = new ArrayList();}
SubnetParamBuilder builder = new SubnetParamBuilder(item);
if (index < 0 || index >= subnets.size()) { _visitables.get("subnets").add(builder); subnets.add(builder); } else { _visitables.get("subnets").set(index, builder); subnets.set(index, builder);}
return (A)this;
}
public A addToSubnets(io.fabric8.openshift.api.model.machine.v1alpha1.SubnetParam... items) {
if (this.subnets == null) {this.subnets = new ArrayList();}
for (SubnetParam item : items) {SubnetParamBuilder builder = new SubnetParamBuilder(item);_visitables.get("subnets").add(builder);this.subnets.add(builder);} return (A)this;
}
public A addAllToSubnets(Collection items) {
if (this.subnets == null) {this.subnets = new ArrayList();}
for (SubnetParam item : items) {SubnetParamBuilder builder = new SubnetParamBuilder(item);_visitables.get("subnets").add(builder);this.subnets.add(builder);} return (A)this;
}
public A removeFromSubnets(io.fabric8.openshift.api.model.machine.v1alpha1.SubnetParam... items) {
if (this.subnets == null) return (A)this;
for (SubnetParam item : items) {SubnetParamBuilder builder = new SubnetParamBuilder(item);_visitables.get("subnets").remove(builder); this.subnets.remove(builder);} return (A)this;
}
public A removeAllFromSubnets(Collection items) {
if (this.subnets == null) return (A)this;
for (SubnetParam item : items) {SubnetParamBuilder builder = new SubnetParamBuilder(item);_visitables.get("subnets").remove(builder); this.subnets.remove(builder);} return (A)this;
}
public A removeMatchingFromSubnets(Predicate predicate) {
if (subnets == null) return (A) this;
final Iterator each = subnets.iterator();
final List visitables = _visitables.get("subnets");
while (each.hasNext()) {
SubnetParamBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildSubnets() {
return subnets != null ? build(subnets) : null;
}
public SubnetParam buildSubnet(int index) {
return this.subnets.get(index).build();
}
public SubnetParam buildFirstSubnet() {
return this.subnets.get(0).build();
}
public SubnetParam buildLastSubnet() {
return this.subnets.get(subnets.size() - 1).build();
}
public SubnetParam buildMatchingSubnet(Predicate predicate) {
for (SubnetParamBuilder item: subnets) { if(predicate.test(item)){ return item.build();} } return null;
}
public boolean hasMatchingSubnet(Predicate predicate) {
for (SubnetParamBuilder item: subnets) { if(predicate.test(item)){ return true;} } return false;
}
public A withSubnets(List subnets) {
if (this.subnets != null) { _visitables.get("subnets").clear();}
if (subnets != null) {this.subnets = new ArrayList(); for (SubnetParam item : subnets){this.addToSubnets(item);}} else { this.subnets = null;} return (A) this;
}
public A withSubnets(io.fabric8.openshift.api.model.machine.v1alpha1.SubnetParam... subnets) {
if (this.subnets != null) {this.subnets.clear(); _visitables.remove("subnets"); }
if (subnets != null) {for (SubnetParam item :subnets){ this.addToSubnets(item);}} return (A) this;
}
public boolean hasSubnets() {
return subnets != null && !subnets.isEmpty();
}
public SubnetsNested addNewSubnet() {
return new SubnetsNested(-1, null);
}
public SubnetsNested addNewSubnetLike(SubnetParam item) {
return new SubnetsNested(-1, item);
}
public SubnetsNested setNewSubnetLike(int index,SubnetParam item) {
return new SubnetsNested(index, item);
}
public SubnetsNested editSubnet(int index) {
if (subnets.size() <= index) throw new RuntimeException("Can't edit subnets. Index exceeds size.");
return setNewSubnetLike(index, buildSubnet(index));
}
public SubnetsNested editFirstSubnet() {
if (subnets.size() == 0) throw new RuntimeException("Can't edit first subnets. The list is empty.");
return setNewSubnetLike(0, buildSubnet(0));
}
public SubnetsNested editLastSubnet() {
int index = subnets.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last subnets. The list is empty.");
return setNewSubnetLike(index, buildSubnet(index));
}
public SubnetsNested editMatchingSubnet(Predicate predicate) {
int index = -1;
for (int i=0;i map) {
if(this.additionalProperties == null && map != null) { this.additionalProperties = new LinkedHashMap(); }
if(map != null) { this.additionalProperties.putAll(map);} return (A)this;
}
public A removeFromAdditionalProperties(String key) {
if(this.additionalProperties == null) { return (A) this; }
if(key != null && this.additionalProperties != null) {this.additionalProperties.remove(key);} return (A)this;
}
public A removeFromAdditionalProperties(Map map) {
if(this.additionalProperties == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.additionalProperties != null){this.additionalProperties.remove(key);}}} return (A)this;
}
public Map getAdditionalProperties() {
return this.additionalProperties;
}
public A withAdditionalProperties(Map additionalProperties) {
if (additionalProperties == null) { this.additionalProperties = null;} else {this.additionalProperties = new LinkedHashMap(additionalProperties);} return (A) this;
}
public boolean hasAdditionalProperties() {
return this.additionalProperties != null;
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
NetworkParamFluent that = (NetworkParamFluent) o;
if (!java.util.Objects.equals(filter, that.filter)) return false;
if (!java.util.Objects.equals(fixedIp, that.fixedIp)) return false;
if (!java.util.Objects.equals(noAllowedAddressPairs, that.noAllowedAddressPairs)) return false;
if (!java.util.Objects.equals(portSecurity, that.portSecurity)) return false;
if (!java.util.Objects.equals(portTags, that.portTags)) return false;
if (!java.util.Objects.equals(profile, that.profile)) return false;
if (!java.util.Objects.equals(subnets, that.subnets)) return false;
if (!java.util.Objects.equals(uuid, that.uuid)) return false;
if (!java.util.Objects.equals(vnicType, that.vnicType)) return false;
if (!java.util.Objects.equals(additionalProperties, that.additionalProperties)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(filter, fixedIp, noAllowedAddressPairs, portSecurity, portTags, profile, subnets, uuid, vnicType, additionalProperties, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (filter != null) { sb.append("filter:"); sb.append(filter + ","); }
if (fixedIp != null) { sb.append("fixedIp:"); sb.append(fixedIp + ","); }
if (noAllowedAddressPairs != null) { sb.append("noAllowedAddressPairs:"); sb.append(noAllowedAddressPairs + ","); }
if (portSecurity != null) { sb.append("portSecurity:"); sb.append(portSecurity + ","); }
if (portTags != null && !portTags.isEmpty()) { sb.append("portTags:"); sb.append(portTags + ","); }
if (profile != null && !profile.isEmpty()) { sb.append("profile:"); sb.append(profile + ","); }
if (subnets != null && !subnets.isEmpty()) { sb.append("subnets:"); sb.append(subnets + ","); }
if (uuid != null) { sb.append("uuid:"); sb.append(uuid + ","); }
if (vnicType != null) { sb.append("vnicType:"); sb.append(vnicType + ","); }
if (additionalProperties != null && !additionalProperties.isEmpty()) { sb.append("additionalProperties:"); sb.append(additionalProperties); }
sb.append("}");
return sb.toString();
}
public A withNoAllowedAddressPairs() {
return withNoAllowedAddressPairs(true);
}
public A withPortSecurity() {
return withPortSecurity(true);
}
public class FilterNested extends FilterFluent> implements Nested{
FilterNested(Filter item) {
this.builder = new FilterBuilder(this, item);
}
FilterBuilder builder;
public N and() {
return (N) NetworkParamFluent.this.withFilter(builder.build());
}
public N endFilter() {
return and();
}
}
public class SubnetsNested extends SubnetParamFluent> implements Nested{
SubnetsNested(int index,SubnetParam item) {
this.index = index;
this.builder = new SubnetParamBuilder(this, item);
}
SubnetParamBuilder builder;
int index;
public N and() {
return (N) NetworkParamFluent.this.setToSubnets(index,builder.build());
}
public N endSubnet() {
return and();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy