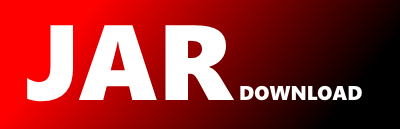
io.fabric8.openshift.api.model.machine.v1alpha1.PortOptsFluent Maven / Gradle / Ivy
package io.fabric8.openshift.api.model.machine.v1alpha1;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.Nested;
import java.util.ArrayList;
import java.lang.String;
import java.util.LinkedHashMap;
import java.util.function.Predicate;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.Iterator;
import java.util.List;
import java.lang.Boolean;
import java.util.Collection;
import java.lang.Object;
import java.util.Map;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class PortOptsFluent> extends BaseFluent{
public PortOptsFluent() {
}
public PortOptsFluent(PortOpts instance) {
this.copyInstance(instance);
}
private Boolean adminStateUp;
private ArrayList allowedAddressPairs = new ArrayList();
private String description;
private ArrayList fixedIPs = new ArrayList();
private String hostID;
private String macAddress;
private String nameSuffix;
private String networkID;
private Boolean portSecurity;
private Map profile;
private String projectID;
private List securityGroups = new ArrayList();
private List tags = new ArrayList();
private String tenantID;
private Boolean trunk;
private String vnicType;
private Map additionalProperties;
protected void copyInstance(PortOpts instance) {
instance = (instance != null ? instance : new PortOpts());
if (instance != null) {
this.withAdminStateUp(instance.getAdminStateUp());
this.withAllowedAddressPairs(instance.getAllowedAddressPairs());
this.withDescription(instance.getDescription());
this.withFixedIPs(instance.getFixedIPs());
this.withHostID(instance.getHostID());
this.withMacAddress(instance.getMacAddress());
this.withNameSuffix(instance.getNameSuffix());
this.withNetworkID(instance.getNetworkID());
this.withPortSecurity(instance.getPortSecurity());
this.withProfile(instance.getProfile());
this.withProjectID(instance.getProjectID());
this.withSecurityGroups(instance.getSecurityGroups());
this.withTags(instance.getTags());
this.withTenantID(instance.getTenantID());
this.withTrunk(instance.getTrunk());
this.withVnicType(instance.getVnicType());
this.withAdminStateUp(instance.getAdminStateUp());
this.withAllowedAddressPairs(instance.getAllowedAddressPairs());
this.withDescription(instance.getDescription());
this.withFixedIPs(instance.getFixedIPs());
this.withHostID(instance.getHostID());
this.withMacAddress(instance.getMacAddress());
this.withNameSuffix(instance.getNameSuffix());
this.withNetworkID(instance.getNetworkID());
this.withPortSecurity(instance.getPortSecurity());
this.withProfile(instance.getProfile());
this.withProjectID(instance.getProjectID());
this.withSecurityGroups(instance.getSecurityGroups());
this.withTags(instance.getTags());
this.withTenantID(instance.getTenantID());
this.withTrunk(instance.getTrunk());
this.withVnicType(instance.getVnicType());
this.withAdditionalProperties(instance.getAdditionalProperties());
}
}
public Boolean getAdminStateUp() {
return this.adminStateUp;
}
public A withAdminStateUp(Boolean adminStateUp) {
this.adminStateUp=adminStateUp; return (A) this;
}
public boolean hasAdminStateUp() {
return this.adminStateUp != null;
}
public A addToAllowedAddressPairs(int index,AddressPair item) {
if (this.allowedAddressPairs == null) {this.allowedAddressPairs = new ArrayList();}
AddressPairBuilder builder = new AddressPairBuilder(item);
if (index < 0 || index >= allowedAddressPairs.size()) { _visitables.get("allowedAddressPairs").add(builder); allowedAddressPairs.add(builder); } else { _visitables.get("allowedAddressPairs").add(index, builder); allowedAddressPairs.add(index, builder);}
return (A)this;
}
public A setToAllowedAddressPairs(int index,AddressPair item) {
if (this.allowedAddressPairs == null) {this.allowedAddressPairs = new ArrayList();}
AddressPairBuilder builder = new AddressPairBuilder(item);
if (index < 0 || index >= allowedAddressPairs.size()) { _visitables.get("allowedAddressPairs").add(builder); allowedAddressPairs.add(builder); } else { _visitables.get("allowedAddressPairs").set(index, builder); allowedAddressPairs.set(index, builder);}
return (A)this;
}
public A addToAllowedAddressPairs(io.fabric8.openshift.api.model.machine.v1alpha1.AddressPair... items) {
if (this.allowedAddressPairs == null) {this.allowedAddressPairs = new ArrayList();}
for (AddressPair item : items) {AddressPairBuilder builder = new AddressPairBuilder(item);_visitables.get("allowedAddressPairs").add(builder);this.allowedAddressPairs.add(builder);} return (A)this;
}
public A addAllToAllowedAddressPairs(Collection items) {
if (this.allowedAddressPairs == null) {this.allowedAddressPairs = new ArrayList();}
for (AddressPair item : items) {AddressPairBuilder builder = new AddressPairBuilder(item);_visitables.get("allowedAddressPairs").add(builder);this.allowedAddressPairs.add(builder);} return (A)this;
}
public A removeFromAllowedAddressPairs(io.fabric8.openshift.api.model.machine.v1alpha1.AddressPair... items) {
if (this.allowedAddressPairs == null) return (A)this;
for (AddressPair item : items) {AddressPairBuilder builder = new AddressPairBuilder(item);_visitables.get("allowedAddressPairs").remove(builder); this.allowedAddressPairs.remove(builder);} return (A)this;
}
public A removeAllFromAllowedAddressPairs(Collection items) {
if (this.allowedAddressPairs == null) return (A)this;
for (AddressPair item : items) {AddressPairBuilder builder = new AddressPairBuilder(item);_visitables.get("allowedAddressPairs").remove(builder); this.allowedAddressPairs.remove(builder);} return (A)this;
}
public A removeMatchingFromAllowedAddressPairs(Predicate predicate) {
if (allowedAddressPairs == null) return (A) this;
final Iterator each = allowedAddressPairs.iterator();
final List visitables = _visitables.get("allowedAddressPairs");
while (each.hasNext()) {
AddressPairBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildAllowedAddressPairs() {
return allowedAddressPairs != null ? build(allowedAddressPairs) : null;
}
public AddressPair buildAllowedAddressPair(int index) {
return this.allowedAddressPairs.get(index).build();
}
public AddressPair buildFirstAllowedAddressPair() {
return this.allowedAddressPairs.get(0).build();
}
public AddressPair buildLastAllowedAddressPair() {
return this.allowedAddressPairs.get(allowedAddressPairs.size() - 1).build();
}
public AddressPair buildMatchingAllowedAddressPair(Predicate predicate) {
for (AddressPairBuilder item: allowedAddressPairs) { if(predicate.test(item)){ return item.build();} } return null;
}
public boolean hasMatchingAllowedAddressPair(Predicate predicate) {
for (AddressPairBuilder item: allowedAddressPairs) { if(predicate.test(item)){ return true;} } return false;
}
public A withAllowedAddressPairs(List allowedAddressPairs) {
if (this.allowedAddressPairs != null) { _visitables.get("allowedAddressPairs").clear();}
if (allowedAddressPairs != null) {this.allowedAddressPairs = new ArrayList(); for (AddressPair item : allowedAddressPairs){this.addToAllowedAddressPairs(item);}} else { this.allowedAddressPairs = null;} return (A) this;
}
public A withAllowedAddressPairs(io.fabric8.openshift.api.model.machine.v1alpha1.AddressPair... allowedAddressPairs) {
if (this.allowedAddressPairs != null) {this.allowedAddressPairs.clear(); _visitables.remove("allowedAddressPairs"); }
if (allowedAddressPairs != null) {for (AddressPair item :allowedAddressPairs){ this.addToAllowedAddressPairs(item);}} return (A) this;
}
public boolean hasAllowedAddressPairs() {
return allowedAddressPairs != null && !allowedAddressPairs.isEmpty();
}
public A addNewAllowedAddressPair(String ipAddress,String macAddress) {
return (A)addToAllowedAddressPairs(new AddressPair(ipAddress, macAddress));
}
public AllowedAddressPairsNested addNewAllowedAddressPair() {
return new AllowedAddressPairsNested(-1, null);
}
public AllowedAddressPairsNested addNewAllowedAddressPairLike(AddressPair item) {
return new AllowedAddressPairsNested(-1, item);
}
public AllowedAddressPairsNested setNewAllowedAddressPairLike(int index,AddressPair item) {
return new AllowedAddressPairsNested(index, item);
}
public AllowedAddressPairsNested editAllowedAddressPair(int index) {
if (allowedAddressPairs.size() <= index) throw new RuntimeException("Can't edit allowedAddressPairs. Index exceeds size.");
return setNewAllowedAddressPairLike(index, buildAllowedAddressPair(index));
}
public AllowedAddressPairsNested editFirstAllowedAddressPair() {
if (allowedAddressPairs.size() == 0) throw new RuntimeException("Can't edit first allowedAddressPairs. The list is empty.");
return setNewAllowedAddressPairLike(0, buildAllowedAddressPair(0));
}
public AllowedAddressPairsNested editLastAllowedAddressPair() {
int index = allowedAddressPairs.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last allowedAddressPairs. The list is empty.");
return setNewAllowedAddressPairLike(index, buildAllowedAddressPair(index));
}
public AllowedAddressPairsNested editMatchingAllowedAddressPair(Predicate predicate) {
int index = -1;
for (int i=0;i();}
FixedIPsBuilder builder = new FixedIPsBuilder(item);
if (index < 0 || index >= fixedIPs.size()) { _visitables.get("fixedIPs").add(builder); fixedIPs.add(builder); } else { _visitables.get("fixedIPs").add(index, builder); fixedIPs.add(index, builder);}
return (A)this;
}
public A setToFixedIPs(int index,FixedIPs item) {
if (this.fixedIPs == null) {this.fixedIPs = new ArrayList();}
FixedIPsBuilder builder = new FixedIPsBuilder(item);
if (index < 0 || index >= fixedIPs.size()) { _visitables.get("fixedIPs").add(builder); fixedIPs.add(builder); } else { _visitables.get("fixedIPs").set(index, builder); fixedIPs.set(index, builder);}
return (A)this;
}
public A addToFixedIPs(io.fabric8.openshift.api.model.machine.v1alpha1.FixedIPs... items) {
if (this.fixedIPs == null) {this.fixedIPs = new ArrayList();}
for (FixedIPs item : items) {FixedIPsBuilder builder = new FixedIPsBuilder(item);_visitables.get("fixedIPs").add(builder);this.fixedIPs.add(builder);} return (A)this;
}
public A addAllToFixedIPs(Collection items) {
if (this.fixedIPs == null) {this.fixedIPs = new ArrayList();}
for (FixedIPs item : items) {FixedIPsBuilder builder = new FixedIPsBuilder(item);_visitables.get("fixedIPs").add(builder);this.fixedIPs.add(builder);} return (A)this;
}
public A removeFromFixedIPs(io.fabric8.openshift.api.model.machine.v1alpha1.FixedIPs... items) {
if (this.fixedIPs == null) return (A)this;
for (FixedIPs item : items) {FixedIPsBuilder builder = new FixedIPsBuilder(item);_visitables.get("fixedIPs").remove(builder); this.fixedIPs.remove(builder);} return (A)this;
}
public A removeAllFromFixedIPs(Collection items) {
if (this.fixedIPs == null) return (A)this;
for (FixedIPs item : items) {FixedIPsBuilder builder = new FixedIPsBuilder(item);_visitables.get("fixedIPs").remove(builder); this.fixedIPs.remove(builder);} return (A)this;
}
public A removeMatchingFromFixedIPs(Predicate predicate) {
if (fixedIPs == null) return (A) this;
final Iterator each = fixedIPs.iterator();
final List visitables = _visitables.get("fixedIPs");
while (each.hasNext()) {
FixedIPsBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildFixedIPs() {
return fixedIPs != null ? build(fixedIPs) : null;
}
public FixedIPs buildFixedIP(int index) {
return this.fixedIPs.get(index).build();
}
public FixedIPs buildFirstFixedIP() {
return this.fixedIPs.get(0).build();
}
public FixedIPs buildLastFixedIP() {
return this.fixedIPs.get(fixedIPs.size() - 1).build();
}
public FixedIPs buildMatchingFixedIP(Predicate predicate) {
for (FixedIPsBuilder item: fixedIPs) { if(predicate.test(item)){ return item.build();} } return null;
}
public boolean hasMatchingFixedIP(Predicate predicate) {
for (FixedIPsBuilder item: fixedIPs) { if(predicate.test(item)){ return true;} } return false;
}
public A withFixedIPs(List fixedIPs) {
if (this.fixedIPs != null) { _visitables.get("fixedIPs").clear();}
if (fixedIPs != null) {this.fixedIPs = new ArrayList(); for (FixedIPs item : fixedIPs){this.addToFixedIPs(item);}} else { this.fixedIPs = null;} return (A) this;
}
public A withFixedIPs(io.fabric8.openshift.api.model.machine.v1alpha1.FixedIPs... fixedIPs) {
if (this.fixedIPs != null) {this.fixedIPs.clear(); _visitables.remove("fixedIPs"); }
if (fixedIPs != null) {for (FixedIPs item :fixedIPs){ this.addToFixedIPs(item);}} return (A) this;
}
public boolean hasFixedIPs() {
return fixedIPs != null && !fixedIPs.isEmpty();
}
public A addNewFixedIP(String ipAddress,String subnetID) {
return (A)addToFixedIPs(new FixedIPs(ipAddress, subnetID));
}
public FixedIPsNested addNewFixedIP() {
return new FixedIPsNested(-1, null);
}
public FixedIPsNested addNewFixedIPLike(FixedIPs item) {
return new FixedIPsNested(-1, item);
}
public FixedIPsNested setNewFixedIPLike(int index,FixedIPs item) {
return new FixedIPsNested(index, item);
}
public FixedIPsNested editFixedIP(int index) {
if (fixedIPs.size() <= index) throw new RuntimeException("Can't edit fixedIPs. Index exceeds size.");
return setNewFixedIPLike(index, buildFixedIP(index));
}
public FixedIPsNested editFirstFixedIP() {
if (fixedIPs.size() == 0) throw new RuntimeException("Can't edit first fixedIPs. The list is empty.");
return setNewFixedIPLike(0, buildFixedIP(0));
}
public FixedIPsNested editLastFixedIP() {
int index = fixedIPs.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last fixedIPs. The list is empty.");
return setNewFixedIPLike(index, buildFixedIP(index));
}
public FixedIPsNested editMatchingFixedIP(Predicate predicate) {
int index = -1;
for (int i=0;i map) {
if(this.profile == null && map != null) { this.profile = new LinkedHashMap(); }
if(map != null) { this.profile.putAll(map);} return (A)this;
}
public A removeFromProfile(String key) {
if(this.profile == null) { return (A) this; }
if(key != null && this.profile != null) {this.profile.remove(key);} return (A)this;
}
public A removeFromProfile(Map map) {
if(this.profile == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.profile != null){this.profile.remove(key);}}} return (A)this;
}
public Map getProfile() {
return this.profile;
}
public A withProfile(Map profile) {
if (profile == null) { this.profile = null;} else {this.profile = new LinkedHashMap(profile);} return (A) this;
}
public boolean hasProfile() {
return this.profile != null;
}
public String getProjectID() {
return this.projectID;
}
public A withProjectID(String projectID) {
this.projectID=projectID; return (A) this;
}
public boolean hasProjectID() {
return this.projectID != null;
}
public A addToSecurityGroups(int index,String item) {
if (this.securityGroups == null) {this.securityGroups = new ArrayList();}
this.securityGroups.add(index, item);
return (A)this;
}
public A setToSecurityGroups(int index,String item) {
if (this.securityGroups == null) {this.securityGroups = new ArrayList();}
this.securityGroups.set(index, item); return (A)this;
}
public A addToSecurityGroups(java.lang.String... items) {
if (this.securityGroups == null) {this.securityGroups = new ArrayList();}
for (String item : items) {this.securityGroups.add(item);} return (A)this;
}
public A addAllToSecurityGroups(Collection items) {
if (this.securityGroups == null) {this.securityGroups = new ArrayList();}
for (String item : items) {this.securityGroups.add(item);} return (A)this;
}
public A removeFromSecurityGroups(java.lang.String... items) {
if (this.securityGroups == null) return (A)this;
for (String item : items) { this.securityGroups.remove(item);} return (A)this;
}
public A removeAllFromSecurityGroups(Collection items) {
if (this.securityGroups == null) return (A)this;
for (String item : items) { this.securityGroups.remove(item);} return (A)this;
}
public List getSecurityGroups() {
return this.securityGroups;
}
public String getSecurityGroup(int index) {
return this.securityGroups.get(index);
}
public String getFirstSecurityGroup() {
return this.securityGroups.get(0);
}
public String getLastSecurityGroup() {
return this.securityGroups.get(securityGroups.size() - 1);
}
public String getMatchingSecurityGroup(Predicate predicate) {
for (String item: securityGroups) { if(predicate.test(item)){ return item;} } return null;
}
public boolean hasMatchingSecurityGroup(Predicate predicate) {
for (String item: securityGroups) { if(predicate.test(item)){ return true;} } return false;
}
public A withSecurityGroups(List securityGroups) {
if (securityGroups != null) {this.securityGroups = new ArrayList(); for (String item : securityGroups){this.addToSecurityGroups(item);}} else { this.securityGroups = null;} return (A) this;
}
public A withSecurityGroups(java.lang.String... securityGroups) {
if (this.securityGroups != null) {this.securityGroups.clear(); _visitables.remove("securityGroups"); }
if (securityGroups != null) {for (String item :securityGroups){ this.addToSecurityGroups(item);}} return (A) this;
}
public boolean hasSecurityGroups() {
return securityGroups != null && !securityGroups.isEmpty();
}
public A addToTags(int index,String item) {
if (this.tags == null) {this.tags = new ArrayList();}
this.tags.add(index, item);
return (A)this;
}
public A setToTags(int index,String item) {
if (this.tags == null) {this.tags = new ArrayList();}
this.tags.set(index, item); return (A)this;
}
public A addToTags(java.lang.String... items) {
if (this.tags == null) {this.tags = new ArrayList();}
for (String item : items) {this.tags.add(item);} return (A)this;
}
public A addAllToTags(Collection items) {
if (this.tags == null) {this.tags = new ArrayList();}
for (String item : items) {this.tags.add(item);} return (A)this;
}
public A removeFromTags(java.lang.String... items) {
if (this.tags == null) return (A)this;
for (String item : items) { this.tags.remove(item);} return (A)this;
}
public A removeAllFromTags(Collection items) {
if (this.tags == null) return (A)this;
for (String item : items) { this.tags.remove(item);} return (A)this;
}
public List getTags() {
return this.tags;
}
public String getTag(int index) {
return this.tags.get(index);
}
public String getFirstTag() {
return this.tags.get(0);
}
public String getLastTag() {
return this.tags.get(tags.size() - 1);
}
public String getMatchingTag(Predicate predicate) {
for (String item: tags) { if(predicate.test(item)){ return item;} } return null;
}
public boolean hasMatchingTag(Predicate predicate) {
for (String item: tags) { if(predicate.test(item)){ return true;} } return false;
}
public A withTags(List tags) {
if (tags != null) {this.tags = new ArrayList(); for (String item : tags){this.addToTags(item);}} else { this.tags = null;} return (A) this;
}
public A withTags(java.lang.String... tags) {
if (this.tags != null) {this.tags.clear(); _visitables.remove("tags"); }
if (tags != null) {for (String item :tags){ this.addToTags(item);}} return (A) this;
}
public boolean hasTags() {
return tags != null && !tags.isEmpty();
}
public String getTenantID() {
return this.tenantID;
}
public A withTenantID(String tenantID) {
this.tenantID=tenantID; return (A) this;
}
public boolean hasTenantID() {
return this.tenantID != null;
}
public Boolean getTrunk() {
return this.trunk;
}
public A withTrunk(Boolean trunk) {
this.trunk=trunk; return (A) this;
}
public boolean hasTrunk() {
return this.trunk != null;
}
public String getVnicType() {
return this.vnicType;
}
public A withVnicType(String vnicType) {
this.vnicType=vnicType; return (A) this;
}
public boolean hasVnicType() {
return this.vnicType != null;
}
public A addToAdditionalProperties(String key,Object value) {
if(this.additionalProperties == null && key != null && value != null) { this.additionalProperties = new LinkedHashMap(); }
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (A)this;
}
public A addToAdditionalProperties(Map map) {
if(this.additionalProperties == null && map != null) { this.additionalProperties = new LinkedHashMap(); }
if(map != null) { this.additionalProperties.putAll(map);} return (A)this;
}
public A removeFromAdditionalProperties(String key) {
if(this.additionalProperties == null) { return (A) this; }
if(key != null && this.additionalProperties != null) {this.additionalProperties.remove(key);} return (A)this;
}
public A removeFromAdditionalProperties(Map map) {
if(this.additionalProperties == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.additionalProperties != null){this.additionalProperties.remove(key);}}} return (A)this;
}
public Map getAdditionalProperties() {
return this.additionalProperties;
}
public A withAdditionalProperties(Map additionalProperties) {
if (additionalProperties == null) { this.additionalProperties = null;} else {this.additionalProperties = new LinkedHashMap(additionalProperties);} return (A) this;
}
public boolean hasAdditionalProperties() {
return this.additionalProperties != null;
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
PortOptsFluent that = (PortOptsFluent) o;
if (!java.util.Objects.equals(adminStateUp, that.adminStateUp)) return false;
if (!java.util.Objects.equals(allowedAddressPairs, that.allowedAddressPairs)) return false;
if (!java.util.Objects.equals(description, that.description)) return false;
if (!java.util.Objects.equals(fixedIPs, that.fixedIPs)) return false;
if (!java.util.Objects.equals(hostID, that.hostID)) return false;
if (!java.util.Objects.equals(macAddress, that.macAddress)) return false;
if (!java.util.Objects.equals(nameSuffix, that.nameSuffix)) return false;
if (!java.util.Objects.equals(networkID, that.networkID)) return false;
if (!java.util.Objects.equals(portSecurity, that.portSecurity)) return false;
if (!java.util.Objects.equals(profile, that.profile)) return false;
if (!java.util.Objects.equals(projectID, that.projectID)) return false;
if (!java.util.Objects.equals(securityGroups, that.securityGroups)) return false;
if (!java.util.Objects.equals(tags, that.tags)) return false;
if (!java.util.Objects.equals(tenantID, that.tenantID)) return false;
if (!java.util.Objects.equals(trunk, that.trunk)) return false;
if (!java.util.Objects.equals(vnicType, that.vnicType)) return false;
if (!java.util.Objects.equals(additionalProperties, that.additionalProperties)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(adminStateUp, allowedAddressPairs, description, fixedIPs, hostID, macAddress, nameSuffix, networkID, portSecurity, profile, projectID, securityGroups, tags, tenantID, trunk, vnicType, additionalProperties, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (adminStateUp != null) { sb.append("adminStateUp:"); sb.append(adminStateUp + ","); }
if (allowedAddressPairs != null && !allowedAddressPairs.isEmpty()) { sb.append("allowedAddressPairs:"); sb.append(allowedAddressPairs + ","); }
if (description != null) { sb.append("description:"); sb.append(description + ","); }
if (fixedIPs != null && !fixedIPs.isEmpty()) { sb.append("fixedIPs:"); sb.append(fixedIPs + ","); }
if (hostID != null) { sb.append("hostID:"); sb.append(hostID + ","); }
if (macAddress != null) { sb.append("macAddress:"); sb.append(macAddress + ","); }
if (nameSuffix != null) { sb.append("nameSuffix:"); sb.append(nameSuffix + ","); }
if (networkID != null) { sb.append("networkID:"); sb.append(networkID + ","); }
if (portSecurity != null) { sb.append("portSecurity:"); sb.append(portSecurity + ","); }
if (profile != null && !profile.isEmpty()) { sb.append("profile:"); sb.append(profile + ","); }
if (projectID != null) { sb.append("projectID:"); sb.append(projectID + ","); }
if (securityGroups != null && !securityGroups.isEmpty()) { sb.append("securityGroups:"); sb.append(securityGroups + ","); }
if (tags != null && !tags.isEmpty()) { sb.append("tags:"); sb.append(tags + ","); }
if (tenantID != null) { sb.append("tenantID:"); sb.append(tenantID + ","); }
if (trunk != null) { sb.append("trunk:"); sb.append(trunk + ","); }
if (vnicType != null) { sb.append("vnicType:"); sb.append(vnicType + ","); }
if (additionalProperties != null && !additionalProperties.isEmpty()) { sb.append("additionalProperties:"); sb.append(additionalProperties); }
sb.append("}");
return sb.toString();
}
public A withAdminStateUp() {
return withAdminStateUp(true);
}
public A withPortSecurity() {
return withPortSecurity(true);
}
public A withTrunk() {
return withTrunk(true);
}
public class AllowedAddressPairsNested extends AddressPairFluent> implements Nested{
AllowedAddressPairsNested(int index,AddressPair item) {
this.index = index;
this.builder = new AddressPairBuilder(this, item);
}
AddressPairBuilder builder;
int index;
public N and() {
return (N) PortOptsFluent.this.setToAllowedAddressPairs(index,builder.build());
}
public N endAllowedAddressPair() {
return and();
}
}
public class FixedIPsNested extends FixedIPsFluent> implements Nested{
FixedIPsNested(int index,FixedIPs item) {
this.index = index;
this.builder = new FixedIPsBuilder(this, item);
}
FixedIPsBuilder builder;
int index;
public N and() {
return (N) PortOptsFluent.this.setToFixedIPs(index,builder.build());
}
public N endFixedIP() {
return and();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy