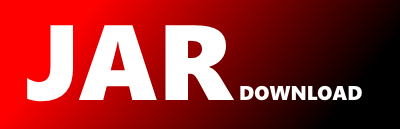
io.fabric8.openshift.api.model.machine.v1beta1.MachineSetStatusFluent Maven / Gradle / Ivy
package io.fabric8.openshift.api.model.machine.v1beta1;
import java.lang.Integer;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.lang.Long;
import java.lang.Object;
import java.lang.String;
import java.util.Map;
import java.util.LinkedHashMap;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class MachineSetStatusFluent> extends BaseFluent{
public MachineSetStatusFluent() {
}
public MachineSetStatusFluent(MachineSetStatus instance) {
this.copyInstance(instance);
}
private Integer availableReplicas;
private String errorMessage;
private String errorReason;
private Integer fullyLabeledReplicas;
private Long observedGeneration;
private Integer readyReplicas;
private Integer replicas;
private Map additionalProperties;
protected void copyInstance(MachineSetStatus instance) {
instance = (instance != null ? instance : new MachineSetStatus());
if (instance != null) {
this.withAvailableReplicas(instance.getAvailableReplicas());
this.withErrorMessage(instance.getErrorMessage());
this.withErrorReason(instance.getErrorReason());
this.withFullyLabeledReplicas(instance.getFullyLabeledReplicas());
this.withObservedGeneration(instance.getObservedGeneration());
this.withReadyReplicas(instance.getReadyReplicas());
this.withReplicas(instance.getReplicas());
this.withAvailableReplicas(instance.getAvailableReplicas());
this.withErrorMessage(instance.getErrorMessage());
this.withErrorReason(instance.getErrorReason());
this.withFullyLabeledReplicas(instance.getFullyLabeledReplicas());
this.withObservedGeneration(instance.getObservedGeneration());
this.withReadyReplicas(instance.getReadyReplicas());
this.withReplicas(instance.getReplicas());
this.withAdditionalProperties(instance.getAdditionalProperties());
}
}
public Integer getAvailableReplicas() {
return this.availableReplicas;
}
public A withAvailableReplicas(Integer availableReplicas) {
this.availableReplicas=availableReplicas; return (A) this;
}
public boolean hasAvailableReplicas() {
return this.availableReplicas != null;
}
public String getErrorMessage() {
return this.errorMessage;
}
public A withErrorMessage(String errorMessage) {
this.errorMessage=errorMessage; return (A) this;
}
public boolean hasErrorMessage() {
return this.errorMessage != null;
}
public String getErrorReason() {
return this.errorReason;
}
public A withErrorReason(String errorReason) {
this.errorReason=errorReason; return (A) this;
}
public boolean hasErrorReason() {
return this.errorReason != null;
}
public Integer getFullyLabeledReplicas() {
return this.fullyLabeledReplicas;
}
public A withFullyLabeledReplicas(Integer fullyLabeledReplicas) {
this.fullyLabeledReplicas=fullyLabeledReplicas; return (A) this;
}
public boolean hasFullyLabeledReplicas() {
return this.fullyLabeledReplicas != null;
}
public Long getObservedGeneration() {
return this.observedGeneration;
}
public A withObservedGeneration(Long observedGeneration) {
this.observedGeneration=observedGeneration; return (A) this;
}
public boolean hasObservedGeneration() {
return this.observedGeneration != null;
}
public Integer getReadyReplicas() {
return this.readyReplicas;
}
public A withReadyReplicas(Integer readyReplicas) {
this.readyReplicas=readyReplicas; return (A) this;
}
public boolean hasReadyReplicas() {
return this.readyReplicas != null;
}
public Integer getReplicas() {
return this.replicas;
}
public A withReplicas(Integer replicas) {
this.replicas=replicas; return (A) this;
}
public boolean hasReplicas() {
return this.replicas != null;
}
public A addToAdditionalProperties(String key,Object value) {
if(this.additionalProperties == null && key != null && value != null) { this.additionalProperties = new LinkedHashMap(); }
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (A)this;
}
public A addToAdditionalProperties(Map map) {
if(this.additionalProperties == null && map != null) { this.additionalProperties = new LinkedHashMap(); }
if(map != null) { this.additionalProperties.putAll(map);} return (A)this;
}
public A removeFromAdditionalProperties(String key) {
if(this.additionalProperties == null) { return (A) this; }
if(key != null && this.additionalProperties != null) {this.additionalProperties.remove(key);} return (A)this;
}
public A removeFromAdditionalProperties(Map map) {
if(this.additionalProperties == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.additionalProperties != null){this.additionalProperties.remove(key);}}} return (A)this;
}
public Map getAdditionalProperties() {
return this.additionalProperties;
}
public A withAdditionalProperties(Map additionalProperties) {
if (additionalProperties == null) { this.additionalProperties = null;} else {this.additionalProperties = new LinkedHashMap(additionalProperties);} return (A) this;
}
public boolean hasAdditionalProperties() {
return this.additionalProperties != null;
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
MachineSetStatusFluent that = (MachineSetStatusFluent) o;
if (!java.util.Objects.equals(availableReplicas, that.availableReplicas)) return false;
if (!java.util.Objects.equals(errorMessage, that.errorMessage)) return false;
if (!java.util.Objects.equals(errorReason, that.errorReason)) return false;
if (!java.util.Objects.equals(fullyLabeledReplicas, that.fullyLabeledReplicas)) return false;
if (!java.util.Objects.equals(observedGeneration, that.observedGeneration)) return false;
if (!java.util.Objects.equals(readyReplicas, that.readyReplicas)) return false;
if (!java.util.Objects.equals(replicas, that.replicas)) return false;
if (!java.util.Objects.equals(additionalProperties, that.additionalProperties)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(availableReplicas, errorMessage, errorReason, fullyLabeledReplicas, observedGeneration, readyReplicas, replicas, additionalProperties, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (availableReplicas != null) { sb.append("availableReplicas:"); sb.append(availableReplicas + ","); }
if (errorMessage != null) { sb.append("errorMessage:"); sb.append(errorMessage + ","); }
if (errorReason != null) { sb.append("errorReason:"); sb.append(errorReason + ","); }
if (fullyLabeledReplicas != null) { sb.append("fullyLabeledReplicas:"); sb.append(fullyLabeledReplicas + ","); }
if (observedGeneration != null) { sb.append("observedGeneration:"); sb.append(observedGeneration + ","); }
if (readyReplicas != null) { sb.append("readyReplicas:"); sb.append(readyReplicas + ","); }
if (replicas != null) { sb.append("replicas:"); sb.append(replicas + ","); }
if (additionalProperties != null && !additionalProperties.isEmpty()) { sb.append("additionalProperties:"); sb.append(additionalProperties); }
sb.append("}");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy