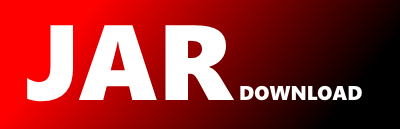
io.fabric8.openshift.api.model.machineconfiguration.v1.ControllerConfigSpecFluent Maven / Gradle / Ivy
package io.fabric8.openshift.api.model.machineconfiguration.v1;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.Nested;
import io.fabric8.openshift.api.model.config.v1.DNS;
import java.util.ArrayList;
import io.fabric8.openshift.api.model.config.v1.ProxyStatus;
import java.lang.String;
import java.util.LinkedHashMap;
import java.util.function.Predicate;
import io.fabric8.kubernetes.api.model.ObjectReference;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import io.fabric8.openshift.api.model.config.v1.Infrastructure;
import java.util.Iterator;
import java.util.List;
import io.fabric8.kubernetes.api.model.ObjectReferenceFluent;
import io.fabric8.kubernetes.api.model.ObjectReferenceBuilder;
import java.util.Collection;
import java.lang.Object;
import java.util.Map;
/**
* Generated
*/
@SuppressWarnings("unchecked")
public class ControllerConfigSpecFluent> extends BaseFluent{
public ControllerConfigSpecFluent() {
}
public ControllerConfigSpecFluent(ControllerConfigSpec instance) {
this.copyInstance(instance);
}
private String additionalTrustBundle;
private String baseOSContainerImage;
private String baseOSExtensionsContainerImage;
private String cloudProviderCAData;
private String cloudProviderConfig;
private String clusterDNSIP;
private DNS dns;
private String etcdDiscoveryDomain;
private ArrayList imageRegistryBundleData = new ArrayList();
private ArrayList imageRegistryBundleUserData = new ArrayList();
private Map images;
private Infrastructure infra;
private String internalRegistryPullSecret;
private String ipFamilies;
private String kubeAPIServerServingCAData;
private NetworkInfoBuilder network;
private String networkType;
private String osImageURL;
private String platform;
private ProxyStatus proxy;
private ObjectReferenceBuilder pullSecret;
private String releaseImage;
private String rootCAData;
private Map additionalProperties;
protected void copyInstance(ControllerConfigSpec instance) {
instance = (instance != null ? instance : new ControllerConfigSpec());
if (instance != null) {
this.withAdditionalTrustBundle(instance.getAdditionalTrustBundle());
this.withBaseOSContainerImage(instance.getBaseOSContainerImage());
this.withBaseOSExtensionsContainerImage(instance.getBaseOSExtensionsContainerImage());
this.withCloudProviderCAData(instance.getCloudProviderCAData());
this.withCloudProviderConfig(instance.getCloudProviderConfig());
this.withClusterDNSIP(instance.getClusterDNSIP());
this.withDns(instance.getDns());
this.withEtcdDiscoveryDomain(instance.getEtcdDiscoveryDomain());
this.withImageRegistryBundleData(instance.getImageRegistryBundleData());
this.withImageRegistryBundleUserData(instance.getImageRegistryBundleUserData());
this.withImages(instance.getImages());
this.withInfra(instance.getInfra());
this.withInternalRegistryPullSecret(instance.getInternalRegistryPullSecret());
this.withIpFamilies(instance.getIpFamilies());
this.withKubeAPIServerServingCAData(instance.getKubeAPIServerServingCAData());
this.withNetwork(instance.getNetwork());
this.withNetworkType(instance.getNetworkType());
this.withOsImageURL(instance.getOsImageURL());
this.withPlatform(instance.getPlatform());
this.withProxy(instance.getProxy());
this.withPullSecret(instance.getPullSecret());
this.withReleaseImage(instance.getReleaseImage());
this.withRootCAData(instance.getRootCAData());
this.withAdditionalProperties(instance.getAdditionalProperties());
}
}
public String getAdditionalTrustBundle() {
return this.additionalTrustBundle;
}
public A withAdditionalTrustBundle(String additionalTrustBundle) {
this.additionalTrustBundle = additionalTrustBundle;
return (A) this;
}
public boolean hasAdditionalTrustBundle() {
return this.additionalTrustBundle != null;
}
public String getBaseOSContainerImage() {
return this.baseOSContainerImage;
}
public A withBaseOSContainerImage(String baseOSContainerImage) {
this.baseOSContainerImage = baseOSContainerImage;
return (A) this;
}
public boolean hasBaseOSContainerImage() {
return this.baseOSContainerImage != null;
}
public String getBaseOSExtensionsContainerImage() {
return this.baseOSExtensionsContainerImage;
}
public A withBaseOSExtensionsContainerImage(String baseOSExtensionsContainerImage) {
this.baseOSExtensionsContainerImage = baseOSExtensionsContainerImage;
return (A) this;
}
public boolean hasBaseOSExtensionsContainerImage() {
return this.baseOSExtensionsContainerImage != null;
}
public String getCloudProviderCAData() {
return this.cloudProviderCAData;
}
public A withCloudProviderCAData(String cloudProviderCAData) {
this.cloudProviderCAData = cloudProviderCAData;
return (A) this;
}
public boolean hasCloudProviderCAData() {
return this.cloudProviderCAData != null;
}
public String getCloudProviderConfig() {
return this.cloudProviderConfig;
}
public A withCloudProviderConfig(String cloudProviderConfig) {
this.cloudProviderConfig = cloudProviderConfig;
return (A) this;
}
public boolean hasCloudProviderConfig() {
return this.cloudProviderConfig != null;
}
public String getClusterDNSIP() {
return this.clusterDNSIP;
}
public A withClusterDNSIP(String clusterDNSIP) {
this.clusterDNSIP = clusterDNSIP;
return (A) this;
}
public boolean hasClusterDNSIP() {
return this.clusterDNSIP != null;
}
public DNS getDns() {
return this.dns;
}
public A withDns(DNS dns) {
this.dns = dns;
return (A) this;
}
public boolean hasDns() {
return this.dns != null;
}
public String getEtcdDiscoveryDomain() {
return this.etcdDiscoveryDomain;
}
public A withEtcdDiscoveryDomain(String etcdDiscoveryDomain) {
this.etcdDiscoveryDomain = etcdDiscoveryDomain;
return (A) this;
}
public boolean hasEtcdDiscoveryDomain() {
return this.etcdDiscoveryDomain != null;
}
public A addToImageRegistryBundleData(int index,ImageRegistryBundle item) {
if (this.imageRegistryBundleData == null) {this.imageRegistryBundleData = new ArrayList();}
ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);
if (index < 0 || index >= imageRegistryBundleData.size()) { _visitables.get("imageRegistryBundleData").add(builder); imageRegistryBundleData.add(builder); } else { _visitables.get("imageRegistryBundleData").add(index, builder); imageRegistryBundleData.add(index, builder);}
return (A)this;
}
public A setToImageRegistryBundleData(int index,ImageRegistryBundle item) {
if (this.imageRegistryBundleData == null) {this.imageRegistryBundleData = new ArrayList();}
ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);
if (index < 0 || index >= imageRegistryBundleData.size()) { _visitables.get("imageRegistryBundleData").add(builder); imageRegistryBundleData.add(builder); } else { _visitables.get("imageRegistryBundleData").set(index, builder); imageRegistryBundleData.set(index, builder);}
return (A)this;
}
public A addToImageRegistryBundleData(io.fabric8.openshift.api.model.machineconfiguration.v1.ImageRegistryBundle... items) {
if (this.imageRegistryBundleData == null) {this.imageRegistryBundleData = new ArrayList();}
for (ImageRegistryBundle item : items) {ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);_visitables.get("imageRegistryBundleData").add(builder);this.imageRegistryBundleData.add(builder);} return (A)this;
}
public A addAllToImageRegistryBundleData(Collection items) {
if (this.imageRegistryBundleData == null) {this.imageRegistryBundleData = new ArrayList();}
for (ImageRegistryBundle item : items) {ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);_visitables.get("imageRegistryBundleData").add(builder);this.imageRegistryBundleData.add(builder);} return (A)this;
}
public A removeFromImageRegistryBundleData(io.fabric8.openshift.api.model.machineconfiguration.v1.ImageRegistryBundle... items) {
if (this.imageRegistryBundleData == null) return (A)this;
for (ImageRegistryBundle item : items) {ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);_visitables.get("imageRegistryBundleData").remove(builder); this.imageRegistryBundleData.remove(builder);} return (A)this;
}
public A removeAllFromImageRegistryBundleData(Collection items) {
if (this.imageRegistryBundleData == null) return (A)this;
for (ImageRegistryBundle item : items) {ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);_visitables.get("imageRegistryBundleData").remove(builder); this.imageRegistryBundleData.remove(builder);} return (A)this;
}
public A removeMatchingFromImageRegistryBundleData(Predicate predicate) {
if (imageRegistryBundleData == null) return (A) this;
final Iterator each = imageRegistryBundleData.iterator();
final List visitables = _visitables.get("imageRegistryBundleData");
while (each.hasNext()) {
ImageRegistryBundleBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildImageRegistryBundleData() {
return this.imageRegistryBundleData != null ? build(imageRegistryBundleData) : null;
}
public ImageRegistryBundle buildImageRegistryBundleDatum(int index) {
return this.imageRegistryBundleData.get(index).build();
}
public ImageRegistryBundle buildFirstImageRegistryBundleDatum() {
return this.imageRegistryBundleData.get(0).build();
}
public ImageRegistryBundle buildLastImageRegistryBundleDatum() {
return this.imageRegistryBundleData.get(imageRegistryBundleData.size() - 1).build();
}
public ImageRegistryBundle buildMatchingImageRegistryBundleDatum(Predicate predicate) {
for (ImageRegistryBundleBuilder item : imageRegistryBundleData) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingImageRegistryBundleDatum(Predicate predicate) {
for (ImageRegistryBundleBuilder item : imageRegistryBundleData) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withImageRegistryBundleData(List imageRegistryBundleData) {
if (this.imageRegistryBundleData != null) {
this._visitables.get("imageRegistryBundleData").clear();
}
if (imageRegistryBundleData != null) {
this.imageRegistryBundleData = new ArrayList();
for (ImageRegistryBundle item : imageRegistryBundleData) {
this.addToImageRegistryBundleData(item);
}
} else {
this.imageRegistryBundleData = null;
}
return (A) this;
}
public A withImageRegistryBundleData(io.fabric8.openshift.api.model.machineconfiguration.v1.ImageRegistryBundle... imageRegistryBundleData) {
if (this.imageRegistryBundleData != null) {
this.imageRegistryBundleData.clear();
_visitables.remove("imageRegistryBundleData");
}
if (imageRegistryBundleData != null) {
for (ImageRegistryBundle item : imageRegistryBundleData) {
this.addToImageRegistryBundleData(item);
}
}
return (A) this;
}
public boolean hasImageRegistryBundleData() {
return this.imageRegistryBundleData != null && !this.imageRegistryBundleData.isEmpty();
}
public A addNewImageRegistryBundleDatum(String data,String file) {
return (A)addToImageRegistryBundleData(new ImageRegistryBundle(data, file));
}
public ImageRegistryBundleDataNested addNewImageRegistryBundleDatum() {
return new ImageRegistryBundleDataNested(-1, null);
}
public ImageRegistryBundleDataNested addNewImageRegistryBundleDatumLike(ImageRegistryBundle item) {
return new ImageRegistryBundleDataNested(-1, item);
}
public ImageRegistryBundleDataNested setNewImageRegistryBundleDatumLike(int index,ImageRegistryBundle item) {
return new ImageRegistryBundleDataNested(index, item);
}
public ImageRegistryBundleDataNested editImageRegistryBundleDatum(int index) {
if (imageRegistryBundleData.size() <= index) throw new RuntimeException("Can't edit imageRegistryBundleData. Index exceeds size.");
return setNewImageRegistryBundleDatumLike(index, buildImageRegistryBundleDatum(index));
}
public ImageRegistryBundleDataNested editFirstImageRegistryBundleDatum() {
if (imageRegistryBundleData.size() == 0) throw new RuntimeException("Can't edit first imageRegistryBundleData. The list is empty.");
return setNewImageRegistryBundleDatumLike(0, buildImageRegistryBundleDatum(0));
}
public ImageRegistryBundleDataNested editLastImageRegistryBundleDatum() {
int index = imageRegistryBundleData.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last imageRegistryBundleData. The list is empty.");
return setNewImageRegistryBundleDatumLike(index, buildImageRegistryBundleDatum(index));
}
public ImageRegistryBundleDataNested editMatchingImageRegistryBundleDatum(Predicate predicate) {
int index = -1;
for (int i=0;i();}
ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);
if (index < 0 || index >= imageRegistryBundleUserData.size()) { _visitables.get("imageRegistryBundleUserData").add(builder); imageRegistryBundleUserData.add(builder); } else { _visitables.get("imageRegistryBundleUserData").add(index, builder); imageRegistryBundleUserData.add(index, builder);}
return (A)this;
}
public A setToImageRegistryBundleUserData(int index,ImageRegistryBundle item) {
if (this.imageRegistryBundleUserData == null) {this.imageRegistryBundleUserData = new ArrayList();}
ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);
if (index < 0 || index >= imageRegistryBundleUserData.size()) { _visitables.get("imageRegistryBundleUserData").add(builder); imageRegistryBundleUserData.add(builder); } else { _visitables.get("imageRegistryBundleUserData").set(index, builder); imageRegistryBundleUserData.set(index, builder);}
return (A)this;
}
public A addToImageRegistryBundleUserData(io.fabric8.openshift.api.model.machineconfiguration.v1.ImageRegistryBundle... items) {
if (this.imageRegistryBundleUserData == null) {this.imageRegistryBundleUserData = new ArrayList();}
for (ImageRegistryBundle item : items) {ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);_visitables.get("imageRegistryBundleUserData").add(builder);this.imageRegistryBundleUserData.add(builder);} return (A)this;
}
public A addAllToImageRegistryBundleUserData(Collection items) {
if (this.imageRegistryBundleUserData == null) {this.imageRegistryBundleUserData = new ArrayList();}
for (ImageRegistryBundle item : items) {ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);_visitables.get("imageRegistryBundleUserData").add(builder);this.imageRegistryBundleUserData.add(builder);} return (A)this;
}
public A removeFromImageRegistryBundleUserData(io.fabric8.openshift.api.model.machineconfiguration.v1.ImageRegistryBundle... items) {
if (this.imageRegistryBundleUserData == null) return (A)this;
for (ImageRegistryBundle item : items) {ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);_visitables.get("imageRegistryBundleUserData").remove(builder); this.imageRegistryBundleUserData.remove(builder);} return (A)this;
}
public A removeAllFromImageRegistryBundleUserData(Collection items) {
if (this.imageRegistryBundleUserData == null) return (A)this;
for (ImageRegistryBundle item : items) {ImageRegistryBundleBuilder builder = new ImageRegistryBundleBuilder(item);_visitables.get("imageRegistryBundleUserData").remove(builder); this.imageRegistryBundleUserData.remove(builder);} return (A)this;
}
public A removeMatchingFromImageRegistryBundleUserData(Predicate predicate) {
if (imageRegistryBundleUserData == null) return (A) this;
final Iterator each = imageRegistryBundleUserData.iterator();
final List visitables = _visitables.get("imageRegistryBundleUserData");
while (each.hasNext()) {
ImageRegistryBundleBuilder builder = each.next();
if (predicate.test(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
public List buildImageRegistryBundleUserData() {
return this.imageRegistryBundleUserData != null ? build(imageRegistryBundleUserData) : null;
}
public ImageRegistryBundle buildImageRegistryBundleUserDatum(int index) {
return this.imageRegistryBundleUserData.get(index).build();
}
public ImageRegistryBundle buildFirstImageRegistryBundleUserDatum() {
return this.imageRegistryBundleUserData.get(0).build();
}
public ImageRegistryBundle buildLastImageRegistryBundleUserDatum() {
return this.imageRegistryBundleUserData.get(imageRegistryBundleUserData.size() - 1).build();
}
public ImageRegistryBundle buildMatchingImageRegistryBundleUserDatum(Predicate predicate) {
for (ImageRegistryBundleBuilder item : imageRegistryBundleUserData) {
if (predicate.test(item)) {
return item.build();
}
}
return null;
}
public boolean hasMatchingImageRegistryBundleUserDatum(Predicate predicate) {
for (ImageRegistryBundleBuilder item : imageRegistryBundleUserData) {
if (predicate.test(item)) {
return true;
}
}
return false;
}
public A withImageRegistryBundleUserData(List imageRegistryBundleUserData) {
if (this.imageRegistryBundleUserData != null) {
this._visitables.get("imageRegistryBundleUserData").clear();
}
if (imageRegistryBundleUserData != null) {
this.imageRegistryBundleUserData = new ArrayList();
for (ImageRegistryBundle item : imageRegistryBundleUserData) {
this.addToImageRegistryBundleUserData(item);
}
} else {
this.imageRegistryBundleUserData = null;
}
return (A) this;
}
public A withImageRegistryBundleUserData(io.fabric8.openshift.api.model.machineconfiguration.v1.ImageRegistryBundle... imageRegistryBundleUserData) {
if (this.imageRegistryBundleUserData != null) {
this.imageRegistryBundleUserData.clear();
_visitables.remove("imageRegistryBundleUserData");
}
if (imageRegistryBundleUserData != null) {
for (ImageRegistryBundle item : imageRegistryBundleUserData) {
this.addToImageRegistryBundleUserData(item);
}
}
return (A) this;
}
public boolean hasImageRegistryBundleUserData() {
return this.imageRegistryBundleUserData != null && !this.imageRegistryBundleUserData.isEmpty();
}
public A addNewImageRegistryBundleUserDatum(String data,String file) {
return (A)addToImageRegistryBundleUserData(new ImageRegistryBundle(data, file));
}
public ImageRegistryBundleUserDataNested addNewImageRegistryBundleUserDatum() {
return new ImageRegistryBundleUserDataNested(-1, null);
}
public ImageRegistryBundleUserDataNested addNewImageRegistryBundleUserDatumLike(ImageRegistryBundle item) {
return new ImageRegistryBundleUserDataNested(-1, item);
}
public ImageRegistryBundleUserDataNested setNewImageRegistryBundleUserDatumLike(int index,ImageRegistryBundle item) {
return new ImageRegistryBundleUserDataNested(index, item);
}
public ImageRegistryBundleUserDataNested editImageRegistryBundleUserDatum(int index) {
if (imageRegistryBundleUserData.size() <= index) throw new RuntimeException("Can't edit imageRegistryBundleUserData. Index exceeds size.");
return setNewImageRegistryBundleUserDatumLike(index, buildImageRegistryBundleUserDatum(index));
}
public ImageRegistryBundleUserDataNested editFirstImageRegistryBundleUserDatum() {
if (imageRegistryBundleUserData.size() == 0) throw new RuntimeException("Can't edit first imageRegistryBundleUserData. The list is empty.");
return setNewImageRegistryBundleUserDatumLike(0, buildImageRegistryBundleUserDatum(0));
}
public ImageRegistryBundleUserDataNested editLastImageRegistryBundleUserDatum() {
int index = imageRegistryBundleUserData.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last imageRegistryBundleUserData. The list is empty.");
return setNewImageRegistryBundleUserDatumLike(index, buildImageRegistryBundleUserDatum(index));
}
public ImageRegistryBundleUserDataNested editMatchingImageRegistryBundleUserDatum(Predicate predicate) {
int index = -1;
for (int i=0;i map) {
if(this.images == null && map != null) { this.images = new LinkedHashMap(); }
if(map != null) { this.images.putAll(map);} return (A)this;
}
public A removeFromImages(String key) {
if(this.images == null) { return (A) this; }
if(key != null && this.images != null) {this.images.remove(key);} return (A)this;
}
public A removeFromImages(Map map) {
if(this.images == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.images != null){this.images.remove(key);}}} return (A)this;
}
public Map getImages() {
return this.images;
}
public A withImages(Map images) {
if (images == null) {
this.images = null;
} else {
this.images = new LinkedHashMap(images);
}
return (A) this;
}
public boolean hasImages() {
return this.images != null;
}
public Infrastructure getInfra() {
return this.infra;
}
public A withInfra(Infrastructure infra) {
this.infra = infra;
return (A) this;
}
public boolean hasInfra() {
return this.infra != null;
}
public String getInternalRegistryPullSecret() {
return this.internalRegistryPullSecret;
}
public A withInternalRegistryPullSecret(String internalRegistryPullSecret) {
this.internalRegistryPullSecret = internalRegistryPullSecret;
return (A) this;
}
public boolean hasInternalRegistryPullSecret() {
return this.internalRegistryPullSecret != null;
}
public String getIpFamilies() {
return this.ipFamilies;
}
public A withIpFamilies(String ipFamilies) {
this.ipFamilies = ipFamilies;
return (A) this;
}
public boolean hasIpFamilies() {
return this.ipFamilies != null;
}
public String getKubeAPIServerServingCAData() {
return this.kubeAPIServerServingCAData;
}
public A withKubeAPIServerServingCAData(String kubeAPIServerServingCAData) {
this.kubeAPIServerServingCAData = kubeAPIServerServingCAData;
return (A) this;
}
public boolean hasKubeAPIServerServingCAData() {
return this.kubeAPIServerServingCAData != null;
}
public NetworkInfo buildNetwork() {
return this.network != null ? this.network.build() : null;
}
public A withNetwork(NetworkInfo network) {
this._visitables.remove("network");
if (network != null) {
this.network = new NetworkInfoBuilder(network);
this._visitables.get("network").add(this.network);
} else {
this.network = null;
this._visitables.get("network").remove(this.network);
}
return (A) this;
}
public boolean hasNetwork() {
return this.network != null;
}
public NetworkNested withNewNetwork() {
return new NetworkNested(null);
}
public NetworkNested withNewNetworkLike(NetworkInfo item) {
return new NetworkNested(item);
}
public NetworkNested editNetwork() {
return withNewNetworkLike(java.util.Optional.ofNullable(buildNetwork()).orElse(null));
}
public NetworkNested editOrNewNetwork() {
return withNewNetworkLike(java.util.Optional.ofNullable(buildNetwork()).orElse(new NetworkInfoBuilder().build()));
}
public NetworkNested editOrNewNetworkLike(NetworkInfo item) {
return withNewNetworkLike(java.util.Optional.ofNullable(buildNetwork()).orElse(item));
}
public String getNetworkType() {
return this.networkType;
}
public A withNetworkType(String networkType) {
this.networkType = networkType;
return (A) this;
}
public boolean hasNetworkType() {
return this.networkType != null;
}
public String getOsImageURL() {
return this.osImageURL;
}
public A withOsImageURL(String osImageURL) {
this.osImageURL = osImageURL;
return (A) this;
}
public boolean hasOsImageURL() {
return this.osImageURL != null;
}
public String getPlatform() {
return this.platform;
}
public A withPlatform(String platform) {
this.platform = platform;
return (A) this;
}
public boolean hasPlatform() {
return this.platform != null;
}
public ProxyStatus getProxy() {
return this.proxy;
}
public A withProxy(ProxyStatus proxy) {
this.proxy = proxy;
return (A) this;
}
public boolean hasProxy() {
return this.proxy != null;
}
public A withNewProxy(String httpProxy,String httpsProxy,String noProxy) {
return (A)withProxy(new ProxyStatus(httpProxy, httpsProxy, noProxy));
}
public ObjectReference buildPullSecret() {
return this.pullSecret != null ? this.pullSecret.build() : null;
}
public A withPullSecret(ObjectReference pullSecret) {
this._visitables.remove("pullSecret");
if (pullSecret != null) {
this.pullSecret = new ObjectReferenceBuilder(pullSecret);
this._visitables.get("pullSecret").add(this.pullSecret);
} else {
this.pullSecret = null;
this._visitables.get("pullSecret").remove(this.pullSecret);
}
return (A) this;
}
public boolean hasPullSecret() {
return this.pullSecret != null;
}
public PullSecretNested withNewPullSecret() {
return new PullSecretNested(null);
}
public PullSecretNested withNewPullSecretLike(ObjectReference item) {
return new PullSecretNested(item);
}
public PullSecretNested editPullSecret() {
return withNewPullSecretLike(java.util.Optional.ofNullable(buildPullSecret()).orElse(null));
}
public PullSecretNested editOrNewPullSecret() {
return withNewPullSecretLike(java.util.Optional.ofNullable(buildPullSecret()).orElse(new ObjectReferenceBuilder().build()));
}
public PullSecretNested editOrNewPullSecretLike(ObjectReference item) {
return withNewPullSecretLike(java.util.Optional.ofNullable(buildPullSecret()).orElse(item));
}
public String getReleaseImage() {
return this.releaseImage;
}
public A withReleaseImage(String releaseImage) {
this.releaseImage = releaseImage;
return (A) this;
}
public boolean hasReleaseImage() {
return this.releaseImage != null;
}
public String getRootCAData() {
return this.rootCAData;
}
public A withRootCAData(String rootCAData) {
this.rootCAData = rootCAData;
return (A) this;
}
public boolean hasRootCAData() {
return this.rootCAData != null;
}
public A addToAdditionalProperties(String key,Object value) {
if(this.additionalProperties == null && key != null && value != null) { this.additionalProperties = new LinkedHashMap(); }
if(key != null && value != null) {this.additionalProperties.put(key, value);} return (A)this;
}
public A addToAdditionalProperties(Map map) {
if(this.additionalProperties == null && map != null) { this.additionalProperties = new LinkedHashMap(); }
if(map != null) { this.additionalProperties.putAll(map);} return (A)this;
}
public A removeFromAdditionalProperties(String key) {
if(this.additionalProperties == null) { return (A) this; }
if(key != null && this.additionalProperties != null) {this.additionalProperties.remove(key);} return (A)this;
}
public A removeFromAdditionalProperties(Map map) {
if(this.additionalProperties == null) { return (A) this; }
if(map != null) { for(Object key : map.keySet()) {if (this.additionalProperties != null){this.additionalProperties.remove(key);}}} return (A)this;
}
public Map getAdditionalProperties() {
return this.additionalProperties;
}
public A withAdditionalProperties(Map additionalProperties) {
if (additionalProperties == null) {
this.additionalProperties = null;
} else {
this.additionalProperties = new LinkedHashMap(additionalProperties);
}
return (A) this;
}
public boolean hasAdditionalProperties() {
return this.additionalProperties != null;
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
ControllerConfigSpecFluent that = (ControllerConfigSpecFluent) o;
if (!java.util.Objects.equals(additionalTrustBundle, that.additionalTrustBundle)) return false;
if (!java.util.Objects.equals(baseOSContainerImage, that.baseOSContainerImage)) return false;
if (!java.util.Objects.equals(baseOSExtensionsContainerImage, that.baseOSExtensionsContainerImage)) return false;
if (!java.util.Objects.equals(cloudProviderCAData, that.cloudProviderCAData)) return false;
if (!java.util.Objects.equals(cloudProviderConfig, that.cloudProviderConfig)) return false;
if (!java.util.Objects.equals(clusterDNSIP, that.clusterDNSIP)) return false;
if (!java.util.Objects.equals(dns, that.dns)) return false;
if (!java.util.Objects.equals(etcdDiscoveryDomain, that.etcdDiscoveryDomain)) return false;
if (!java.util.Objects.equals(imageRegistryBundleData, that.imageRegistryBundleData)) return false;
if (!java.util.Objects.equals(imageRegistryBundleUserData, that.imageRegistryBundleUserData)) return false;
if (!java.util.Objects.equals(images, that.images)) return false;
if (!java.util.Objects.equals(infra, that.infra)) return false;
if (!java.util.Objects.equals(internalRegistryPullSecret, that.internalRegistryPullSecret)) return false;
if (!java.util.Objects.equals(ipFamilies, that.ipFamilies)) return false;
if (!java.util.Objects.equals(kubeAPIServerServingCAData, that.kubeAPIServerServingCAData)) return false;
if (!java.util.Objects.equals(network, that.network)) return false;
if (!java.util.Objects.equals(networkType, that.networkType)) return false;
if (!java.util.Objects.equals(osImageURL, that.osImageURL)) return false;
if (!java.util.Objects.equals(platform, that.platform)) return false;
if (!java.util.Objects.equals(proxy, that.proxy)) return false;
if (!java.util.Objects.equals(pullSecret, that.pullSecret)) return false;
if (!java.util.Objects.equals(releaseImage, that.releaseImage)) return false;
if (!java.util.Objects.equals(rootCAData, that.rootCAData)) return false;
if (!java.util.Objects.equals(additionalProperties, that.additionalProperties)) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(additionalTrustBundle, baseOSContainerImage, baseOSExtensionsContainerImage, cloudProviderCAData, cloudProviderConfig, clusterDNSIP, dns, etcdDiscoveryDomain, imageRegistryBundleData, imageRegistryBundleUserData, images, infra, internalRegistryPullSecret, ipFamilies, kubeAPIServerServingCAData, network, networkType, osImageURL, platform, proxy, pullSecret, releaseImage, rootCAData, additionalProperties, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (additionalTrustBundle != null) { sb.append("additionalTrustBundle:"); sb.append(additionalTrustBundle + ","); }
if (baseOSContainerImage != null) { sb.append("baseOSContainerImage:"); sb.append(baseOSContainerImage + ","); }
if (baseOSExtensionsContainerImage != null) { sb.append("baseOSExtensionsContainerImage:"); sb.append(baseOSExtensionsContainerImage + ","); }
if (cloudProviderCAData != null) { sb.append("cloudProviderCAData:"); sb.append(cloudProviderCAData + ","); }
if (cloudProviderConfig != null) { sb.append("cloudProviderConfig:"); sb.append(cloudProviderConfig + ","); }
if (clusterDNSIP != null) { sb.append("clusterDNSIP:"); sb.append(clusterDNSIP + ","); }
if (dns != null) { sb.append("dns:"); sb.append(dns + ","); }
if (etcdDiscoveryDomain != null) { sb.append("etcdDiscoveryDomain:"); sb.append(etcdDiscoveryDomain + ","); }
if (imageRegistryBundleData != null && !imageRegistryBundleData.isEmpty()) { sb.append("imageRegistryBundleData:"); sb.append(imageRegistryBundleData + ","); }
if (imageRegistryBundleUserData != null && !imageRegistryBundleUserData.isEmpty()) { sb.append("imageRegistryBundleUserData:"); sb.append(imageRegistryBundleUserData + ","); }
if (images != null && !images.isEmpty()) { sb.append("images:"); sb.append(images + ","); }
if (infra != null) { sb.append("infra:"); sb.append(infra + ","); }
if (internalRegistryPullSecret != null) { sb.append("internalRegistryPullSecret:"); sb.append(internalRegistryPullSecret + ","); }
if (ipFamilies != null) { sb.append("ipFamilies:"); sb.append(ipFamilies + ","); }
if (kubeAPIServerServingCAData != null) { sb.append("kubeAPIServerServingCAData:"); sb.append(kubeAPIServerServingCAData + ","); }
if (network != null) { sb.append("network:"); sb.append(network + ","); }
if (networkType != null) { sb.append("networkType:"); sb.append(networkType + ","); }
if (osImageURL != null) { sb.append("osImageURL:"); sb.append(osImageURL + ","); }
if (platform != null) { sb.append("platform:"); sb.append(platform + ","); }
if (proxy != null) { sb.append("proxy:"); sb.append(proxy + ","); }
if (pullSecret != null) { sb.append("pullSecret:"); sb.append(pullSecret + ","); }
if (releaseImage != null) { sb.append("releaseImage:"); sb.append(releaseImage + ","); }
if (rootCAData != null) { sb.append("rootCAData:"); sb.append(rootCAData + ","); }
if (additionalProperties != null && !additionalProperties.isEmpty()) { sb.append("additionalProperties:"); sb.append(additionalProperties); }
sb.append("}");
return sb.toString();
}
public class ImageRegistryBundleDataNested extends ImageRegistryBundleFluent> implements Nested{
ImageRegistryBundleDataNested(int index,ImageRegistryBundle item) {
this.index = index;
this.builder = new ImageRegistryBundleBuilder(this, item);
}
ImageRegistryBundleBuilder builder;
int index;
public N and() {
return (N) ControllerConfigSpecFluent.this.setToImageRegistryBundleData(index,builder.build());
}
public N endImageRegistryBundleDatum() {
return and();
}
}
public class ImageRegistryBundleUserDataNested extends ImageRegistryBundleFluent> implements Nested{
ImageRegistryBundleUserDataNested(int index,ImageRegistryBundle item) {
this.index = index;
this.builder = new ImageRegistryBundleBuilder(this, item);
}
ImageRegistryBundleBuilder builder;
int index;
public N and() {
return (N) ControllerConfigSpecFluent.this.setToImageRegistryBundleUserData(index,builder.build());
}
public N endImageRegistryBundleUserDatum() {
return and();
}
}
public class NetworkNested extends NetworkInfoFluent> implements Nested{
NetworkNested(NetworkInfo item) {
this.builder = new NetworkInfoBuilder(this, item);
}
NetworkInfoBuilder builder;
public N and() {
return (N) ControllerConfigSpecFluent.this.withNetwork(builder.build());
}
public N endNetwork() {
return and();
}
}
public class PullSecretNested extends ObjectReferenceFluent> implements Nested{
PullSecretNested(ObjectReference item) {
this.builder = new ObjectReferenceBuilder(this, item);
}
ObjectReferenceBuilder builder;
public N and() {
return (N) ControllerConfigSpecFluent.this.withPullSecret(builder.build());
}
public N endPullSecret() {
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy