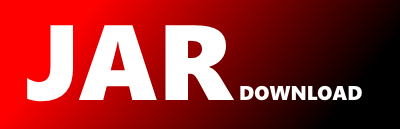
io.fabric8.swagger.model.ApiDeclaration Maven / Gradle / Ivy
package io.fabric8.swagger.model;
import java.net.URI;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.annotation.Generated;
import javax.validation.Valid;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Pattern;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import lombok.EqualsAndHashCode;
import lombok.ToString;
@JsonInclude(JsonInclude.Include.NON_NULL)
@Generated("org.jsonschema2pojo")
@JsonPropertyOrder({
"swaggerVersion",
"apiVersion",
"basePath",
"resourcePath",
"apis",
"models",
"produces",
"consumes",
"authorizations"
})
@ToString
@EqualsAndHashCode
public class ApiDeclaration {
/**
*
* (Required)
*
*/
@JsonProperty("swaggerVersion")
@NotNull
private ApiDeclaration.SwaggerVersion swaggerVersion;
@JsonProperty("apiVersion")
private String apiVersion;
/**
*
* (Required)
*
*/
@JsonProperty("basePath")
@Pattern(regexp = "^https?://")
@NotNull
private URI basePath;
@JsonProperty("resourcePath")
@Pattern(regexp = "^/")
private String resourcePath;
/**
*
* (Required)
*
*/
@JsonProperty("apis")
@Valid
@NotNull
private List apis = new ArrayList();
@JsonProperty("models")
@Valid
private Models models;
@JsonProperty("produces")
@JsonDeserialize(as = java.util.LinkedHashSet.class)
@Valid
private Set produces = new LinkedHashSet();
@JsonProperty("consumes")
@JsonDeserialize(as = java.util.LinkedHashSet.class)
@Valid
private Set consumes = new LinkedHashSet();
@JsonProperty("authorizations")
@Valid
private Authorizations_ authorizations;
/**
*
* (Required)
*
* @return
* The swaggerVersion
*/
@JsonProperty("swaggerVersion")
public ApiDeclaration.SwaggerVersion getSwaggerVersion() {
return swaggerVersion;
}
/**
*
* (Required)
*
* @param swaggerVersion
* The swaggerVersion
*/
@JsonProperty("swaggerVersion")
public void setSwaggerVersion(ApiDeclaration.SwaggerVersion swaggerVersion) {
this.swaggerVersion = swaggerVersion;
}
/**
*
* @return
* The apiVersion
*/
@JsonProperty("apiVersion")
public String getApiVersion() {
return apiVersion;
}
/**
*
* @param apiVersion
* The apiVersion
*/
@JsonProperty("apiVersion")
public void setApiVersion(String apiVersion) {
this.apiVersion = apiVersion;
}
/**
*
* (Required)
*
* @return
* The basePath
*/
@JsonProperty("basePath")
public URI getBasePath() {
return basePath;
}
/**
*
* (Required)
*
* @param basePath
* The basePath
*/
@JsonProperty("basePath")
public void setBasePath(URI basePath) {
this.basePath = basePath;
}
/**
*
* @return
* The resourcePath
*/
@JsonProperty("resourcePath")
public String getResourcePath() {
return resourcePath;
}
/**
*
* @param resourcePath
* The resourcePath
*/
@JsonProperty("resourcePath")
public void setResourcePath(String resourcePath) {
this.resourcePath = resourcePath;
}
/**
*
* (Required)
*
* @return
* The apis
*/
@JsonProperty("apis")
public List getApis() {
return apis;
}
/**
*
* (Required)
*
* @param apis
* The apis
*/
@JsonProperty("apis")
public void setApis(List apis) {
this.apis = apis;
}
/**
*
* @return
* The models
*/
@JsonProperty("models")
public Models getModels() {
return models;
}
/**
*
* @param models
* The models
*/
@JsonProperty("models")
public void setModels(Models models) {
this.models = models;
}
/**
*
* @return
* The produces
*/
@JsonProperty("produces")
public Set getProduces() {
return produces;
}
/**
*
* @param produces
* The produces
*/
@JsonProperty("produces")
public void setProduces(Set produces) {
this.produces = produces;
}
/**
*
* @return
* The consumes
*/
@JsonProperty("consumes")
public Set getConsumes() {
return consumes;
}
/**
*
* @param consumes
* The consumes
*/
@JsonProperty("consumes")
public void setConsumes(Set consumes) {
this.consumes = consumes;
}
/**
*
* @return
* The authorizations
*/
@JsonProperty("authorizations")
public Authorizations_ getAuthorizations() {
return authorizations;
}
/**
*
* @param authorizations
* The authorizations
*/
@JsonProperty("authorizations")
public void setAuthorizations(Authorizations_ authorizations) {
this.authorizations = authorizations;
}
@Generated("org.jsonschema2pojo")
public enum SwaggerVersion {
_1_2("1.2");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (ApiDeclaration.SwaggerVersion c: values()) {
CONSTANTS.put(c.value, c);
}
}
private SwaggerVersion(String value) {
this.value = value;
}
@JsonValue
@Override
public String toString() {
return this.value;
}
@JsonCreator
public static ApiDeclaration.SwaggerVersion fromValue(String value) {
ApiDeclaration.SwaggerVersion constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy