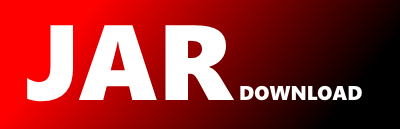
io.fabric8.swagger.model.Parameter Maven / Gradle / Ivy
package io.fabric8.swagger.model;
import java.util.HashMap;
import java.util.Map;
import javax.annotation.Generated;
import javax.validation.constraints.NotNull;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
import lombok.EqualsAndHashCode;
import lombok.ToString;
@JsonInclude(JsonInclude.Include.NON_NULL)
@Generated("org.jsonschema2pojo")
@JsonPropertyOrder({
"paramType",
"name",
"type",
"description",
"required",
"allowMultiple",
"consumes"
})
@ToString
@EqualsAndHashCode
public class Parameter {
@JsonProperty("paramType")
private Parameter.ParamType paramType;
/**
*
* (Required)
*
*/
@NotNull
@JsonProperty("name")
private String name;
@JsonProperty("type")
private String type;
@JsonProperty("description")
private String description;
@JsonProperty("required")
private Boolean required;
@JsonProperty("allowMultiple")
private Boolean allowMultiple;
@JsonProperty("consumes")
private Parameter.Consumes consumes;
@JsonIgnore
private Map additionalProperties = new HashMap();
/**
*
* @return
* The paramType
*/
@JsonProperty("paramType")
public Parameter.ParamType getParamType() {
return paramType;
}
/**
*
* @param paramType
* The paramType
*/
@JsonProperty("paramType")
public void setParamType(Parameter.ParamType paramType) {
this.paramType = paramType;
}
/**
*
* (Required)
*
* @return
* The name
*/
@JsonProperty("name")
public String getName() {
return name;
}
/**
*
* (Required)
*
* @param name
* The name
*/
@JsonProperty("name")
public void setName(String name) {
this.name = name;
}
/**
*
* @return
* The type
*/
@JsonProperty("type")
public String getType() {
return type;
}
/**
*
* @param type
* The type
*/
@JsonProperty("type")
public void setType(String type) {
this.type = type;
}
/**
*
* @return
* The description
*/
@JsonProperty("description")
public String getDescription() {
return description;
}
/**
*
* @param description
* The description
*/
@JsonProperty("description")
public void setDescription(String description) {
this.description = description;
}
/**
*
* @return
* The required
*/
@JsonProperty("required")
public Boolean getRequired() {
return required;
}
/**
*
* @param required
* The required
*/
@JsonProperty("required")
public void setRequired(Boolean required) {
this.required = required;
}
/**
*
* @return
* The allowMultiple
*/
@JsonProperty("allowMultiple")
public Boolean getAllowMultiple() {
return allowMultiple;
}
/**
*
* @param allowMultiple
* The allowMultiple
*/
@JsonProperty("allowMultiple")
public void setAllowMultiple(Boolean allowMultiple) {
this.allowMultiple = allowMultiple;
}
/**
*
* @return
* The consumes
*/
@JsonProperty("consumes")
public Parameter.Consumes getConsumes() {
return consumes;
}
/**
*
* @param consumes
* The consumes
*/
@JsonProperty("consumes")
public void setConsumes(Parameter.Consumes consumes) {
this.consumes = consumes;
}
@JsonAnyGetter
public Map getAdditionalProperties() {
return this.additionalProperties;
}
@JsonAnySetter
public void setAdditionalProperty(String name, Object value) {
this.additionalProperties.put(name, value);
}
@Generated("org.jsonschema2pojo")
public enum Consumes {
MULTIPART_FORM_DATA("multipart/form-data");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (Parameter.Consumes c: values()) {
CONSTANTS.put(c.value, c);
}
}
private Consumes(String value) {
this.value = value;
}
@JsonValue
@Override
public String toString() {
return this.value;
}
@JsonCreator
public static Parameter.Consumes fromValue(String value) {
Parameter.Consumes constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
@Generated("org.jsonschema2pojo")
public enum ParamType {
PATH("path"),
QUERY("query"),
BODY("body"),
HEADER("header"),
FORM("form");
private final String value;
private final static Map CONSTANTS = new HashMap();
static {
for (Parameter.ParamType c: values()) {
CONSTANTS.put(c.value, c);
}
}
private ParamType(String value) {
this.value = value;
}
@JsonValue
@Override
public String toString() {
return this.value;
}
@JsonCreator
public static Parameter.ParamType fromValue(String value) {
Parameter.ParamType constant = CONSTANTS.get(value);
if (constant == null) {
throw new IllegalArgumentException(value);
} else {
return constant;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy