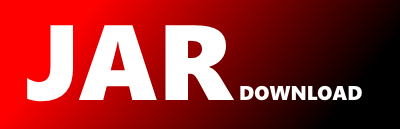
io.firebus.adapters.http.MasterHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of firebus-http Show documentation
Show all versions of firebus-http Show documentation
Firebus http gateway adapter
package io.firebus.adapters.http;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class MasterHandler extends HttpServlet
{
private static final long serialVersionUID = 1L;
protected List handlerMap;
protected HttpHandler defaultHandler;
protected String rootForward;
public MasterHandler()
{
handlerMap = new ArrayList();
}
public void addHttpHandler(String path, String method, HttpHandler handler)
{
handlerMap.add(new HttpHandlerEntry(path, method, handler));
}
public void setDefaultHander(HttpHandler dh)
{
defaultHandler = dh;
}
public void setRootForward(String path)
{
rootForward = path;
}
protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException
{
String path = req.getRequestURI();
String method = req.getMethod();
if(path.equals("/"))
{
resp.setStatus(HttpServletResponse.SC_TEMPORARY_REDIRECT);
resp.setHeader("location", rootForward);
PrintWriter writer = resp.getWriter();
writer.println("Redirect redirecting");
}
else
{
HttpHandler selected = null;
for(int i = 0; i < handlerMap.size() && selected == null; i++)
{
HttpHandlerEntry entry = handlerMap.get(i);
boolean match = true;
if(entry.method.equalsIgnoreCase(method))
{
if(entry.path.endsWith("/*"))
{
String shortEntryPath = entry.path.substring(0, entry.path.length() - 2);
if(path.startsWith(shortEntryPath + "/") || path.equals(shortEntryPath))
match = true;
else
match = false;
}
else
{
if(entry.path.equals(path))
match = true;
else
match = false;
}
}
else
{
match = false;
}
if(match)
selected = entry.handler;
}
if(selected != null)
{
selected.service(req, resp);
}
else if(defaultHandler != null)
{
defaultHandler.service(req, resp);
}
else
{
resp.setStatus(HttpServletResponse.SC_NOT_FOUND);
PrintWriter writer = resp.getWriter();
writer.println("No mapping, fool.");
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy