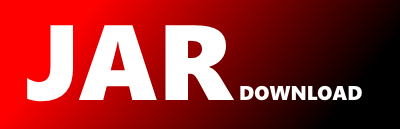
io.fixprotocol.md.antlr.MarkdownBaseVisitor Maven / Gradle / Ivy
// Generated from io\fixprotocol\md\antlr\Markdown.g4 by ANTLR 4.9.2
package io.fixprotocol.md.antlr;
import org.antlr.v4.runtime.tree.AbstractParseTreeVisitor;
/**
* This class provides an empty implementation of {@link MarkdownVisitor},
* which can be extended to create a visitor which only needs to handle a subset
* of the available methods.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public class MarkdownBaseVisitor extends AbstractParseTreeVisitor implements MarkdownVisitor {
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitDocument(MarkdownParser.DocumentContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitBlock(MarkdownParser.BlockContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitHeading(MarkdownParser.HeadingContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitParagraph(MarkdownParser.ParagraphContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitParagraphline(MarkdownParser.ParagraphlineContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitList(MarkdownParser.ListContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitListline(MarkdownParser.ListlineContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitBlockquote(MarkdownParser.BlockquoteContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitQuoteline(MarkdownParser.QuotelineContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitFencedcodeblock(MarkdownParser.FencedcodeblockContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitInfostring(MarkdownParser.InfostringContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitTable(MarkdownParser.TableContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitTableheading(MarkdownParser.TableheadingContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitTablerow(MarkdownParser.TablerowContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitCell(MarkdownParser.CellContext ctx) { return visitChildren(ctx); }
/**
* {@inheritDoc}
*
* The default implementation returns the result of calling
* {@link #visitChildren} on {@code ctx}.
*/
@Override public T visitTabledelimiterrow(MarkdownParser.TabledelimiterrowContext ctx) { return visitChildren(ctx); }
}