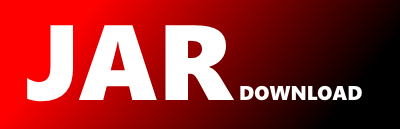
io.fixprotocol.md.antlr.MarkdownParser Maven / Gradle / Ivy
// Generated from io\fixprotocol\md\antlr\Markdown.g4 by ANTLR 4.9.2
package io.fixprotocol.md.antlr;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast"})
public class MarkdownParser extends Parser {
static { RuntimeMetaData.checkVersion("4.9.2", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
LITERAL=1, HEADINGLINE=2, QUOTELINE=3, LISTLINE=4, PARAGRAPHLINE=5, TABLEDELIMINATORCELL=6,
FENCE=7, IGNORE_WS=8, NEWLINE=9, CELLTEXT=10, BACKTICK=11, GT=12, HASH=13,
PIPE=14;
public static final int
RULE_document = 0, RULE_block = 1, RULE_heading = 2, RULE_paragraph = 3,
RULE_paragraphline = 4, RULE_list = 5, RULE_listline = 6, RULE_blockquote = 7,
RULE_quoteline = 8, RULE_fencedcodeblock = 9, RULE_infostring = 10, RULE_table = 11,
RULE_tableheading = 12, RULE_tablerow = 13, RULE_cell = 14, RULE_tabledelimiterrow = 15;
private static String[] makeRuleNames() {
return new String[] {
"document", "block", "heading", "paragraph", "paragraphline", "list",
"listline", "blockquote", "quoteline", "fencedcodeblock", "infostring",
"table", "tableheading", "tablerow", "cell", "tabledelimiterrow"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, null, null, null, null, null, null, "'```'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "LITERAL", "HEADINGLINE", "QUOTELINE", "LISTLINE", "PARAGRAPHLINE",
"TABLEDELIMINATORCELL", "FENCE", "IGNORE_WS", "NEWLINE", "CELLTEXT",
"BACKTICK", "GT", "HASH", "PIPE"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "Markdown.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public MarkdownParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
public static class DocumentContext extends ParserRuleContext {
public TerminalNode EOF() { return getToken(MarkdownParser.EOF, 0); }
public List block() {
return getRuleContexts(BlockContext.class);
}
public BlockContext block(int i) {
return getRuleContext(BlockContext.class,i);
}
public DocumentContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_document; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterDocument(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitDocument(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitDocument(this);
else return visitor.visitChildren(this);
}
}
public final DocumentContext document() throws RecognitionException {
DocumentContext _localctx = new DocumentContext(_ctx, getState());
enterRule(_localctx, 0, RULE_document);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(33);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(32);
block();
}
}
setState(35);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( (((_la) & ~0x3f) == 0 && ((1L << _la) & ((1L << HEADINGLINE) | (1L << QUOTELINE) | (1L << LISTLINE) | (1L << PARAGRAPHLINE) | (1L << FENCE) | (1L << NEWLINE) | (1L << CELLTEXT))) != 0) );
setState(37);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BlockContext extends ParserRuleContext {
public HeadingContext heading() {
return getRuleContext(HeadingContext.class,0);
}
public ParagraphContext paragraph() {
return getRuleContext(ParagraphContext.class,0);
}
public ListContext list() {
return getRuleContext(ListContext.class,0);
}
public BlockquoteContext blockquote() {
return getRuleContext(BlockquoteContext.class,0);
}
public FencedcodeblockContext fencedcodeblock() {
return getRuleContext(FencedcodeblockContext.class,0);
}
public TableContext table() {
return getRuleContext(TableContext.class,0);
}
public TerminalNode NEWLINE() { return getToken(MarkdownParser.NEWLINE, 0); }
public BlockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_block; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterBlock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitBlock(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitBlock(this);
else return visitor.visitChildren(this);
}
}
public final BlockContext block() throws RecognitionException {
BlockContext _localctx = new BlockContext(_ctx, getState());
enterRule(_localctx, 2, RULE_block);
try {
setState(46);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,1,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(39);
heading();
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(40);
paragraph();
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(41);
list();
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(42);
blockquote();
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(43);
fencedcodeblock();
}
break;
case 6:
enterOuterAlt(_localctx, 6);
{
setState(44);
table();
}
break;
case 7:
enterOuterAlt(_localctx, 7);
{
setState(45);
match(NEWLINE);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class HeadingContext extends ParserRuleContext {
public TerminalNode HEADINGLINE() { return getToken(MarkdownParser.HEADINGLINE, 0); }
public List NEWLINE() { return getTokens(MarkdownParser.NEWLINE); }
public TerminalNode NEWLINE(int i) {
return getToken(MarkdownParser.NEWLINE, i);
}
public HeadingContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_heading; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterHeading(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitHeading(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitHeading(this);
else return visitor.visitChildren(this);
}
}
public final HeadingContext heading() throws RecognitionException {
HeadingContext _localctx = new HeadingContext(_ctx, getState());
enterRule(_localctx, 4, RULE_heading);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(51);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NEWLINE) {
{
{
setState(48);
match(NEWLINE);
}
}
setState(53);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(54);
match(HEADINGLINE);
setState(55);
match(NEWLINE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ParagraphContext extends ParserRuleContext {
public List NEWLINE() { return getTokens(MarkdownParser.NEWLINE); }
public TerminalNode NEWLINE(int i) {
return getToken(MarkdownParser.NEWLINE, i);
}
public List paragraphline() {
return getRuleContexts(ParagraphlineContext.class);
}
public ParagraphlineContext paragraphline(int i) {
return getRuleContext(ParagraphlineContext.class,i);
}
public ParagraphContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_paragraph; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterParagraph(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitParagraph(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitParagraph(this);
else return visitor.visitChildren(this);
}
}
public final ParagraphContext paragraph() throws RecognitionException {
ParagraphContext _localctx = new ParagraphContext(_ctx, getState());
enterRule(_localctx, 6, RULE_paragraph);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(60);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NEWLINE) {
{
{
setState(57);
match(NEWLINE);
}
}
setState(62);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(64);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(63);
paragraphline();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(66);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,4,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ParagraphlineContext extends ParserRuleContext {
public TerminalNode PARAGRAPHLINE() { return getToken(MarkdownParser.PARAGRAPHLINE, 0); }
public TerminalNode NEWLINE() { return getToken(MarkdownParser.NEWLINE, 0); }
public TerminalNode EOF() { return getToken(MarkdownParser.EOF, 0); }
public ParagraphlineContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_paragraphline; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterParagraphline(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitParagraphline(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitParagraphline(this);
else return visitor.visitChildren(this);
}
}
public final ParagraphlineContext paragraphline() throws RecognitionException {
ParagraphlineContext _localctx = new ParagraphlineContext(_ctx, getState());
enterRule(_localctx, 8, RULE_paragraphline);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(68);
match(PARAGRAPHLINE);
setState(69);
_la = _input.LA(1);
if ( !(_la==EOF || _la==NEWLINE) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ListContext extends ParserRuleContext {
public List NEWLINE() { return getTokens(MarkdownParser.NEWLINE); }
public TerminalNode NEWLINE(int i) {
return getToken(MarkdownParser.NEWLINE, i);
}
public List listline() {
return getRuleContexts(ListlineContext.class);
}
public ListlineContext listline(int i) {
return getRuleContext(ListlineContext.class,i);
}
public ListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_list; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterList(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitList(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitList(this);
else return visitor.visitChildren(this);
}
}
public final ListContext list() throws RecognitionException {
ListContext _localctx = new ListContext(_ctx, getState());
enterRule(_localctx, 10, RULE_list);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(74);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NEWLINE) {
{
{
setState(71);
match(NEWLINE);
}
}
setState(76);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(78);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(77);
listline();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(80);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,6,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class ListlineContext extends ParserRuleContext {
public TerminalNode LISTLINE() { return getToken(MarkdownParser.LISTLINE, 0); }
public TerminalNode NEWLINE() { return getToken(MarkdownParser.NEWLINE, 0); }
public TerminalNode EOF() { return getToken(MarkdownParser.EOF, 0); }
public ListlineContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_listline; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterListline(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitListline(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitListline(this);
else return visitor.visitChildren(this);
}
}
public final ListlineContext listline() throws RecognitionException {
ListlineContext _localctx = new ListlineContext(_ctx, getState());
enterRule(_localctx, 12, RULE_listline);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(82);
match(LISTLINE);
setState(83);
_la = _input.LA(1);
if ( !(_la==EOF || _la==NEWLINE) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class BlockquoteContext extends ParserRuleContext {
public List NEWLINE() { return getTokens(MarkdownParser.NEWLINE); }
public TerminalNode NEWLINE(int i) {
return getToken(MarkdownParser.NEWLINE, i);
}
public List quoteline() {
return getRuleContexts(QuotelineContext.class);
}
public QuotelineContext quoteline(int i) {
return getRuleContext(QuotelineContext.class,i);
}
public BlockquoteContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_blockquote; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterBlockquote(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitBlockquote(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitBlockquote(this);
else return visitor.visitChildren(this);
}
}
public final BlockquoteContext blockquote() throws RecognitionException {
BlockquoteContext _localctx = new BlockquoteContext(_ctx, getState());
enterRule(_localctx, 14, RULE_blockquote);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(88);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NEWLINE) {
{
{
setState(85);
match(NEWLINE);
}
}
setState(90);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(92);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(91);
quoteline();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(94);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,8,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class QuotelineContext extends ParserRuleContext {
public TerminalNode QUOTELINE() { return getToken(MarkdownParser.QUOTELINE, 0); }
public TerminalNode NEWLINE() { return getToken(MarkdownParser.NEWLINE, 0); }
public TerminalNode EOF() { return getToken(MarkdownParser.EOF, 0); }
public QuotelineContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_quoteline; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterQuoteline(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitQuoteline(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitQuoteline(this);
else return visitor.visitChildren(this);
}
}
public final QuotelineContext quoteline() throws RecognitionException {
QuotelineContext _localctx = new QuotelineContext(_ctx, getState());
enterRule(_localctx, 16, RULE_quoteline);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(96);
match(QUOTELINE);
setState(97);
_la = _input.LA(1);
if ( !(_la==EOF || _la==NEWLINE) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class FencedcodeblockContext extends ParserRuleContext {
public List FENCE() { return getTokens(MarkdownParser.FENCE); }
public TerminalNode FENCE(int i) {
return getToken(MarkdownParser.FENCE, i);
}
public TerminalNode NEWLINE() { return getToken(MarkdownParser.NEWLINE, 0); }
public InfostringContext infostring() {
return getRuleContext(InfostringContext.class,0);
}
public List paragraphline() {
return getRuleContexts(ParagraphlineContext.class);
}
public ParagraphlineContext paragraphline(int i) {
return getRuleContext(ParagraphlineContext.class,i);
}
public FencedcodeblockContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_fencedcodeblock; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterFencedcodeblock(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitFencedcodeblock(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitFencedcodeblock(this);
else return visitor.visitChildren(this);
}
}
public final FencedcodeblockContext fencedcodeblock() throws RecognitionException {
FencedcodeblockContext _localctx = new FencedcodeblockContext(_ctx, getState());
enterRule(_localctx, 18, RULE_fencedcodeblock);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(99);
match(FENCE);
setState(101);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PARAGRAPHLINE) {
{
setState(100);
infostring();
}
}
setState(103);
match(NEWLINE);
setState(105);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(104);
paragraphline();
}
}
setState(107);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==PARAGRAPHLINE );
setState(109);
match(FENCE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class InfostringContext extends ParserRuleContext {
public TerminalNode PARAGRAPHLINE() { return getToken(MarkdownParser.PARAGRAPHLINE, 0); }
public InfostringContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_infostring; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterInfostring(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitInfostring(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitInfostring(this);
else return visitor.visitChildren(this);
}
}
public final InfostringContext infostring() throws RecognitionException {
InfostringContext _localctx = new InfostringContext(_ctx, getState());
enterRule(_localctx, 20, RULE_infostring);
try {
enterOuterAlt(_localctx, 1);
{
setState(111);
match(PARAGRAPHLINE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TableContext extends ParserRuleContext {
public TableheadingContext tableheading() {
return getRuleContext(TableheadingContext.class,0);
}
public TabledelimiterrowContext tabledelimiterrow() {
return getRuleContext(TabledelimiterrowContext.class,0);
}
public List NEWLINE() { return getTokens(MarkdownParser.NEWLINE); }
public TerminalNode NEWLINE(int i) {
return getToken(MarkdownParser.NEWLINE, i);
}
public List tablerow() {
return getRuleContexts(TablerowContext.class);
}
public TablerowContext tablerow(int i) {
return getRuleContext(TablerowContext.class,i);
}
public TableContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_table; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterTable(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitTable(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitTable(this);
else return visitor.visitChildren(this);
}
}
public final TableContext table() throws RecognitionException {
TableContext _localctx = new TableContext(_ctx, getState());
enterRule(_localctx, 22, RULE_table);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(116);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==NEWLINE) {
{
{
setState(113);
match(NEWLINE);
}
}
setState(118);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(119);
tableheading();
setState(120);
tabledelimiterrow();
setState(122);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(121);
tablerow();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(124);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,12,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TableheadingContext extends ParserRuleContext {
public TablerowContext tablerow() {
return getRuleContext(TablerowContext.class,0);
}
public TableheadingContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_tableheading; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterTableheading(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitTableheading(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitTableheading(this);
else return visitor.visitChildren(this);
}
}
public final TableheadingContext tableheading() throws RecognitionException {
TableheadingContext _localctx = new TableheadingContext(_ctx, getState());
enterRule(_localctx, 24, RULE_tableheading);
try {
enterOuterAlt(_localctx, 1);
{
setState(126);
tablerow();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TablerowContext extends ParserRuleContext {
public TerminalNode NEWLINE() { return getToken(MarkdownParser.NEWLINE, 0); }
public TerminalNode EOF() { return getToken(MarkdownParser.EOF, 0); }
public List cell() {
return getRuleContexts(CellContext.class);
}
public CellContext cell(int i) {
return getRuleContext(CellContext.class,i);
}
public TerminalNode PIPE() { return getToken(MarkdownParser.PIPE, 0); }
public TablerowContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_tablerow; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterTablerow(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitTablerow(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitTablerow(this);
else return visitor.visitChildren(this);
}
}
public final TablerowContext tablerow() throws RecognitionException {
TablerowContext _localctx = new TablerowContext(_ctx, getState());
enterRule(_localctx, 26, RULE_tablerow);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(129);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(128);
cell();
}
}
setState(131);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==CELLTEXT );
setState(134);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PIPE) {
{
setState(133);
match(PIPE);
}
}
setState(136);
_la = _input.LA(1);
if ( !(_la==EOF || _la==NEWLINE) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class CellContext extends ParserRuleContext {
public TerminalNode CELLTEXT() { return getToken(MarkdownParser.CELLTEXT, 0); }
public CellContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_cell; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterCell(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitCell(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitCell(this);
else return visitor.visitChildren(this);
}
}
public final CellContext cell() throws RecognitionException {
CellContext _localctx = new CellContext(_ctx, getState());
enterRule(_localctx, 28, RULE_cell);
try {
enterOuterAlt(_localctx, 1);
{
setState(138);
match(CELLTEXT);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static class TabledelimiterrowContext extends ParserRuleContext {
public TerminalNode NEWLINE() { return getToken(MarkdownParser.NEWLINE, 0); }
public List TABLEDELIMINATORCELL() { return getTokens(MarkdownParser.TABLEDELIMINATORCELL); }
public TerminalNode TABLEDELIMINATORCELL(int i) {
return getToken(MarkdownParser.TABLEDELIMINATORCELL, i);
}
public TerminalNode PIPE() { return getToken(MarkdownParser.PIPE, 0); }
public TabledelimiterrowContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_tabledelimiterrow; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).enterTabledelimiterrow(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof MarkdownListener ) ((MarkdownListener)listener).exitTabledelimiterrow(this);
}
@Override
public T accept(ParseTreeVisitor extends T> visitor) {
if ( visitor instanceof MarkdownVisitor ) return ((MarkdownVisitor extends T>)visitor).visitTabledelimiterrow(this);
else return visitor.visitChildren(this);
}
}
public final TabledelimiterrowContext tabledelimiterrow() throws RecognitionException {
TabledelimiterrowContext _localctx = new TabledelimiterrowContext(_ctx, getState());
enterRule(_localctx, 30, RULE_tabledelimiterrow);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(141);
_errHandler.sync(this);
_la = _input.LA(1);
do {
{
{
setState(140);
match(TABLEDELIMINATORCELL);
}
}
setState(143);
_errHandler.sync(this);
_la = _input.LA(1);
} while ( _la==TABLEDELIMINATORCELL );
setState(146);
_errHandler.sync(this);
_la = _input.LA(1);
if (_la==PIPE) {
{
setState(145);
match(PIPE);
}
}
setState(148);
match(NEWLINE);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public static final String _serializedATN =
"\3\u608b\ua72a\u8133\ub9ed\u417c\u3be7\u7786\u5964\3\20\u0099\4\2\t\2"+
"\4\3\t\3\4\4\t\4\4\5\t\5\4\6\t\6\4\7\t\7\4\b\t\b\4\t\t\t\4\n\t\n\4\13"+
"\t\13\4\f\t\f\4\r\t\r\4\16\t\16\4\17\t\17\4\20\t\20\4\21\t\21\3\2\6\2"+
"$\n\2\r\2\16\2%\3\2\3\2\3\3\3\3\3\3\3\3\3\3\3\3\3\3\5\3\61\n\3\3\4\7\4"+
"\64\n\4\f\4\16\4\67\13\4\3\4\3\4\3\4\3\5\7\5=\n\5\f\5\16\5@\13\5\3\5\6"+
"\5C\n\5\r\5\16\5D\3\6\3\6\3\6\3\7\7\7K\n\7\f\7\16\7N\13\7\3\7\6\7Q\n\7"+
"\r\7\16\7R\3\b\3\b\3\b\3\t\7\tY\n\t\f\t\16\t\\\13\t\3\t\6\t_\n\t\r\t\16"+
"\t`\3\n\3\n\3\n\3\13\3\13\5\13h\n\13\3\13\3\13\6\13l\n\13\r\13\16\13m"+
"\3\13\3\13\3\f\3\f\3\r\7\ru\n\r\f\r\16\rx\13\r\3\r\3\r\3\r\6\r}\n\r\r"+
"\r\16\r~\3\16\3\16\3\17\6\17\u0084\n\17\r\17\16\17\u0085\3\17\5\17\u0089"+
"\n\17\3\17\3\17\3\20\3\20\3\21\6\21\u0090\n\21\r\21\16\21\u0091\3\21\5"+
"\21\u0095\n\21\3\21\3\21\3\21\2\2\22\2\4\6\b\n\f\16\20\22\24\26\30\32"+
"\34\36 \2\3\3\3\13\13\2\u009e\2#\3\2\2\2\4\60\3\2\2\2\6\65\3\2\2\2\b>"+
"\3\2\2\2\nF\3\2\2\2\fL\3\2\2\2\16T\3\2\2\2\20Z\3\2\2\2\22b\3\2\2\2\24"+
"e\3\2\2\2\26q\3\2\2\2\30v\3\2\2\2\32\u0080\3\2\2\2\34\u0083\3\2\2\2\36"+
"\u008c\3\2\2\2 \u008f\3\2\2\2\"$\5\4\3\2#\"\3\2\2\2$%\3\2\2\2%#\3\2\2"+
"\2%&\3\2\2\2&\'\3\2\2\2\'(\7\2\2\3(\3\3\2\2\2)\61\5\6\4\2*\61\5\b\5\2"+
"+\61\5\f\7\2,\61\5\20\t\2-\61\5\24\13\2.\61\5\30\r\2/\61\7\13\2\2\60)"+
"\3\2\2\2\60*\3\2\2\2\60+\3\2\2\2\60,\3\2\2\2\60-\3\2\2\2\60.\3\2\2\2\60"+
"/\3\2\2\2\61\5\3\2\2\2\62\64\7\13\2\2\63\62\3\2\2\2\64\67\3\2\2\2\65\63"+
"\3\2\2\2\65\66\3\2\2\2\668\3\2\2\2\67\65\3\2\2\289\7\4\2\29:\7\13\2\2"+
":\7\3\2\2\2;=\7\13\2\2<;\3\2\2\2=@\3\2\2\2><\3\2\2\2>?\3\2\2\2?B\3\2\2"+
"\2@>\3\2\2\2AC\5\n\6\2BA\3\2\2\2CD\3\2\2\2DB\3\2\2\2DE\3\2\2\2E\t\3\2"+
"\2\2FG\7\7\2\2GH\t\2\2\2H\13\3\2\2\2IK\7\13\2\2JI\3\2\2\2KN\3\2\2\2LJ"+
"\3\2\2\2LM\3\2\2\2MP\3\2\2\2NL\3\2\2\2OQ\5\16\b\2PO\3\2\2\2QR\3\2\2\2"+
"RP\3\2\2\2RS\3\2\2\2S\r\3\2\2\2TU\7\6\2\2UV\t\2\2\2V\17\3\2\2\2WY\7\13"+
"\2\2XW\3\2\2\2Y\\\3\2\2\2ZX\3\2\2\2Z[\3\2\2\2[^\3\2\2\2\\Z\3\2\2\2]_\5"+
"\22\n\2^]\3\2\2\2_`\3\2\2\2`^\3\2\2\2`a\3\2\2\2a\21\3\2\2\2bc\7\5\2\2"+
"cd\t\2\2\2d\23\3\2\2\2eg\7\t\2\2fh\5\26\f\2gf\3\2\2\2gh\3\2\2\2hi\3\2"+
"\2\2ik\7\13\2\2jl\5\n\6\2kj\3\2\2\2lm\3\2\2\2mk\3\2\2\2mn\3\2\2\2no\3"+
"\2\2\2op\7\t\2\2p\25\3\2\2\2qr\7\7\2\2r\27\3\2\2\2su\7\13\2\2ts\3\2\2"+
"\2ux\3\2\2\2vt\3\2\2\2vw\3\2\2\2wy\3\2\2\2xv\3\2\2\2yz\5\32\16\2z|\5 "+
"\21\2{}\5\34\17\2|{\3\2\2\2}~\3\2\2\2~|\3\2\2\2~\177\3\2\2\2\177\31\3"+
"\2\2\2\u0080\u0081\5\34\17\2\u0081\33\3\2\2\2\u0082\u0084\5\36\20\2\u0083"+
"\u0082\3\2\2\2\u0084\u0085\3\2\2\2\u0085\u0083\3\2\2\2\u0085\u0086\3\2"+
"\2\2\u0086\u0088\3\2\2\2\u0087\u0089\7\20\2\2\u0088\u0087\3\2\2\2\u0088"+
"\u0089\3\2\2\2\u0089\u008a\3\2\2\2\u008a\u008b\t\2\2\2\u008b\35\3\2\2"+
"\2\u008c\u008d\7\f\2\2\u008d\37\3\2\2\2\u008e\u0090\7\b\2\2\u008f\u008e"+
"\3\2\2\2\u0090\u0091\3\2\2\2\u0091\u008f\3\2\2\2\u0091\u0092\3\2\2\2\u0092"+
"\u0094\3\2\2\2\u0093\u0095\7\20\2\2\u0094\u0093\3\2\2\2\u0094\u0095\3"+
"\2\2\2\u0095\u0096\3\2\2\2\u0096\u0097\7\13\2\2\u0097!\3\2\2\2\23%\60"+
"\65>DLRZ`gmv~\u0085\u0088\u0091\u0094";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy