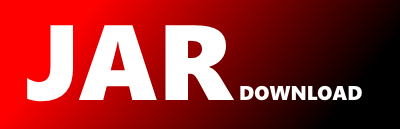
com.flowthings.client.api.DomainObjectApi Maven / Gradle / Ivy
package com.flowthings.client.api;
import java.util.List;
import com.flowthings.client.QueryOptions;
/**
* Methods to create {@link Request} objects, based on actions which the user
* might want to perform
*
* @author matt
*/
public class DomainObjectApi {
protected Class clazz;
public DomainObjectApi(Class clazz) {
this.clazz = clazz;
}
/**
* Retrieve a single object by ID
*
* @param id
* - the object's ID
* @return - A request object, to pass to an API
*/
public Request get(String id) {
return Request.createObjectRequest(clazz, Request.Action.GET).id(id);
}
/**
* Find multiple objects using search parameters
*
* @param queryOptions
* - search parameters
* @return - A request object, to pass to an API
*/
public Request> find(QueryOptions queryOptions) {
return Request.createListRequest(clazz, Request.Action.FIND).params(queryOptions);
}
/**
* Create a new object
*
* @param t
* - the object to create
* @return - A request object, to pass to an API
*/
public Request create(T t) {
return Request.createObjectRequest(clazz, Request.Action.CREATE).body(t);
}
/**
* Delete an object by ID
*
* @param id
* - the object's ID
* @return - A request object, to pass to an API
*/
public Request delete(String id) {
return Request.createObjectRequest(clazz, Request.Action.DELETE).id(id);
}
/**
* Find multiple objects using search parameters
*
* @return - A request object, to pass to an API
*/
public Request> find() {
return find(new QueryOptions());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy