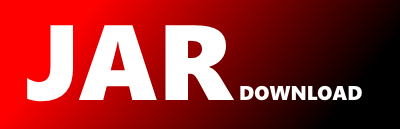
com.flowthings.client.api.FlowthingsFuture Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of flowthings-client-core Show documentation
Show all versions of flowthings-client-core Show documentation
A client library for flowthings.io realtime event processing
package com.flowthings.client.api;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import com.flowthings.client.exception.AsyncException;
import com.flowthings.client.exception.FlowthingsException;
import com.google.common.util.concurrent.Futures;
/**
* Created by matt on 7/5/16.
*/
public class FlowthingsFuture implements Future {
private final Future inner;
public FlowthingsFuture(Future inner){
this.inner = inner;
}
public static FlowthingsFuture fromResult(S result){
return new FlowthingsFuture<>(Futures.immediateFuture(result));
}
public static FlowthingsFuture fromException(FlowthingsException e){
return new FlowthingsFuture<>(Futures.immediateFailedFuture(e));
}
@Override
public boolean cancel(boolean mayInterruptIfRunning) {
return inner.cancel(mayInterruptIfRunning);
}
@Override
public boolean isCancelled() {
return inner.isCancelled();
}
@Override
public boolean isDone() {
return inner.isDone();
}
@Override
public T get() throws InterruptedException, ExecutionException {
return inner.get();
}
@Override
public T get(long timeout, TimeUnit unit) throws InterruptedException, ExecutionException, TimeoutException {
return inner.get(timeout, unit);
}
/**
* Works like "get", but returns a sane exception type
*/
public T grab() throws FlowthingsException {
try {
return this.get();
} catch (InterruptedException e) {
throw new AsyncException(e);
} catch (ExecutionException e) {
Throwable cause = e.getCause();
if (cause instanceof FlowthingsException){
throw (FlowthingsException) cause;
} else {
throw new FlowthingsException(cause);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy