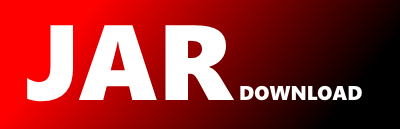
io.fluxcapacitor.javaclient.modeling.DefaultHandlerRepository Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Default Java client library for interfacing with Flux Capacitor.
/*
* Copyright (c) Flux Capacitor IP B.V. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.fluxcapacitor.javaclient.modeling;
import io.fluxcapacitor.common.api.search.Constraint;
import io.fluxcapacitor.common.api.search.constraints.MatchConstraint;
import io.fluxcapacitor.common.reflection.ReflectionUtils;
import io.fluxcapacitor.javaclient.FluxCapacitor;
import io.fluxcapacitor.javaclient.common.ClientUtils;
import io.fluxcapacitor.javaclient.common.Entry;
import io.fluxcapacitor.javaclient.persisting.search.DocumentStore;
import io.fluxcapacitor.javaclient.tracking.handling.Stateful;
import lombok.SneakyThrows;
import lombok.extern.slf4j.Slf4j;
import java.time.Instant;
import java.time.temporal.TemporalAccessor;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.function.Function;
import static io.fluxcapacitor.javaclient.common.ClientUtils.memoize;
@Slf4j
public class DefaultHandlerRepository implements HandlerRepository {
public static Function, HandlerRepository> repositorySupplier(DocumentStore documentStore) {
return memoize(type -> new DefaultHandlerRepository(
documentStore, ClientUtils.getSearchParameters(type).getCollection(),
type, ReflectionUtils.getTypeAnnotation(type, Stateful.class)));
}
private final DocumentStore documentStore;
private final String collection;
private final Class> type;
private final Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy