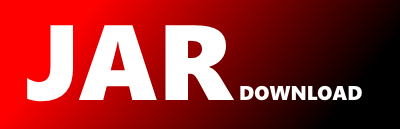
ru.foodtechlab.lib.auth.service.domain.preference.entity.ServicePreferenceEntity Maven / Gradle / Ivy
package ru.foodtechlab.lib.auth.service.domain.preference.entity;
import com.rcore.domain.commons.entity.BaseEntity;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.EqualsAndHashCode;
import lombok.NoArgsConstructor;
import ru.foodtechlab.lib.auth.service.domain.auth.entity.AuthSessionEntity;
import ru.foodtechlab.lib.auth.service.domain.confirmationCode.entity.ConfirmationCodeDestinationType;
import ru.foodtechlab.lib.auth.service.domain.confirmationCode.entity.ConfirmationCodeEntity;
import java.util.Optional;
@NoArgsConstructor
@AllArgsConstructor
@Data
@EqualsAndHashCode(callSuper = true)
public class ServicePreferenceEntity extends BaseEntity {
private String defaultRoleCode;
private String defaultIsoTwoLetterCountryCode;
private String defaultPersonalConfirmationCode;
private String generalConfirmationCode;
private ConfirmationCodeEntity.Type defaultConfirmationCodeType;
private ConfirmationCodeDayAttemptLimit confirmationCodeDayAttemptLimits = new ConfirmationCodeDayAttemptLimit();
private AuthSessionTypeAttemptLimits deviceIdDayAttemptLimits = new AuthSessionTypeAttemptLimits();
private AuthSessionTypeAttemptLimits ipV4HourAttemptLimits = new AuthSessionTypeAttemptLimits();
private AuthSessionWithConfirmationTTLs authSessionWithConfirmationTTLs = new AuthSessionWithConfirmationTTLs();
private ConfirmationCodeMessageBodies confirmationCodeMessageBodies = new ConfirmationCodeMessageBodies();
private AttemptLimitsByLoginType authSessionLoginConfirmAttemptsLimits = new AttemptLimitsByLoginType();
private ConfirmationCodeMessageWebhookProviders defaultConfirmationCodeMessageWebhookProviders = new ConfirmationCodeMessageWebhookProviders();
private DefaultConfirmationCodeMessageBodies defaultConfirmationCodeMessageBodies = new DefaultConfirmationCodeMessageBodies();
private AttemptLimitsByLoginType passwordValidationHourAttemptLimits = new AttemptLimitsByLoginType();
public Long getPasswordValidationHourAttemptLimitsByLoginType(AuthSessionEntity.LoginType loginType) {
if (loginType.equals(AuthSessionEntity.LoginType.EMAIL))
return passwordValidationHourAttemptLimits.getEmailLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.USERNAME))
return passwordValidationHourAttemptLimits.getUsernameLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.PHONE_NUMBER))
return passwordValidationHourAttemptLimits.getPhoneNumberLimit();
return 0L;
}
public Long getTTLForAuthSessionWithConfirmation(ConfirmationCodeEntity.Type type) {
if (type.equals(ConfirmationCodeEntity.Type.PERSONAL))
return this.authSessionWithConfirmationTTLs.getAuthSessionWithPersonalConfirmationCodeTTL();
else if (type.equals(ConfirmationCodeEntity.Type.ONE_TIME))
return this.authSessionWithConfirmationTTLs.getAuthSessionWithOneTimeConfirmationCodeTTL();
else if (type.equals(ConfirmationCodeEntity.Type.GENERAL))
return this.authSessionWithConfirmationTTLs.getAuthSessionWithGeneralConfirmationCodeTTL();
return 0L;
}
public Long getDeviceTokenLimit(AuthSessionEntity.Type type, AuthSessionEntity.LoginType loginType) {
if (this.deviceIdDayAttemptLimits == null) {
this.deviceIdDayAttemptLimits = new AuthSessionTypeAttemptLimits();
}
if (type.equals(AuthSessionEntity.Type.SINGLE_FACTOR_TWO_STEPS_WITH_CONFIRMATION)) {
if (loginType.equals(AuthSessionEntity.LoginType.EMAIL))
return this.deviceIdDayAttemptLimits.getSingleFactorWithConfirmationAuthSessionLimits().getEmailLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.PHONE_NUMBER))
return this.deviceIdDayAttemptLimits.getSingleFactorWithConfirmationAuthSessionLimits().getPhoneNumberLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.USERNAME))
return this.deviceIdDayAttemptLimits.getSingleFactorWithConfirmationAuthSessionLimits().getUsernameLimit();
} else {
if (loginType.equals(AuthSessionEntity.LoginType.EMAIL)) {
return this.deviceIdDayAttemptLimits.getSingleFactorWithPasswordAuthSessionLimits().getEmailLimit();
} else if (loginType.equals(AuthSessionEntity.LoginType.PHONE_NUMBER)) {
return this.deviceIdDayAttemptLimits.getSingleFactorWithPasswordAuthSessionLimits().getPhoneNumberLimit();
} else if (loginType.equals(AuthSessionEntity.LoginType.USERNAME)) {
return this.deviceIdDayAttemptLimits.getSingleFactorWithPasswordAuthSessionLimits().getUsernameLimit();
}
}
return 0L;
}
public Long getIpLimit(AuthSessionEntity.Type type, AuthSessionEntity.LoginType loginType) {
if (type.equals(AuthSessionEntity.Type.SINGLE_FACTOR_TWO_STEPS_WITH_CONFIRMATION)) {
if (loginType.equals(AuthSessionEntity.LoginType.EMAIL))
return this.ipV4HourAttemptLimits.getSingleFactorWithConfirmationAuthSessionLimits().getEmailLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.PHONE_NUMBER))
return this.ipV4HourAttemptLimits.getSingleFactorWithConfirmationAuthSessionLimits().getPhoneNumberLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.USERNAME))
return this.ipV4HourAttemptLimits.getSingleFactorWithConfirmationAuthSessionLimits().getUsernameLimit();
} else {
if (loginType.equals(AuthSessionEntity.LoginType.EMAIL))
return this.ipV4HourAttemptLimits.getSingleFactorWithPasswordAuthSessionLimits().getEmailLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.PHONE_NUMBER))
return this.ipV4HourAttemptLimits.getSingleFactorWithPasswordAuthSessionLimits().getPhoneNumberLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.USERNAME))
return this.ipV4HourAttemptLimits.getSingleFactorWithPasswordAuthSessionLimits().getUsernameLimit();
}
return 0L;
}
public Long getConfirmationCodeLimit(ConfirmationCodeEntity.Type confirmationCodeType, AuthSessionEntity.LoginType loginType) {
if (confirmationCodeType.equals(ConfirmationCodeEntity.Type.PERSONAL)) {
if (loginType.equals(AuthSessionEntity.LoginType.EMAIL))
return this.confirmationCodeDayAttemptLimits.getPersonalConfirmationCode().getEmailLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.PHONE_NUMBER))
return this.confirmationCodeDayAttemptLimits.getPersonalConfirmationCode().getPhoneNumberLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.USERNAME))
return this.confirmationCodeDayAttemptLimits.getPersonalConfirmationCode().getUsernameLimit();
} else if (confirmationCodeType.equals(ConfirmationCodeEntity.Type.ONE_TIME)) {
if (loginType.equals(AuthSessionEntity.LoginType.EMAIL))
return this.confirmationCodeDayAttemptLimits.getOneTimeConfirmationCode().getEmailLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.PHONE_NUMBER))
return this.confirmationCodeDayAttemptLimits.getOneTimeConfirmationCode().getPhoneNumberLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.USERNAME))
return this.confirmationCodeDayAttemptLimits.getOneTimeConfirmationCode().getUsernameLimit();
} else {
if (loginType.equals(AuthSessionEntity.LoginType.EMAIL))
return this.confirmationCodeDayAttemptLimits.getGeneralConfirmationCode().getEmailLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.PHONE_NUMBER))
return this.confirmationCodeDayAttemptLimits.getGeneralConfirmationCode().getPhoneNumberLimit();
else if (loginType.equals(AuthSessionEntity.LoginType.USERNAME))
return this.confirmationCodeDayAttemptLimits.getGeneralConfirmationCode().getUsernameLimit();
}
return 0L;
}
public Optional getWebhookProviderByType(ConfirmationCodeDestinationType confirmationCodeDestinationType) {
if (confirmationCodeDestinationType.equals(ConfirmationCodeDestinationType.EMAIL))
return Optional.of(this.defaultConfirmationCodeMessageWebhookProviders.getEmailWebhookProvider());
if (confirmationCodeDestinationType.equals(ConfirmationCodeDestinationType.PHONE_NUMBER))
return Optional.of(this.defaultConfirmationCodeMessageWebhookProviders.getSmsWebhookProvider());
return Optional.empty();
}
public Long getAuthSessionLoginConfirmAttemptsLimitsByLoginType(AuthSessionEntity.LoginType loginType) {
if (loginType.equals(AuthSessionEntity.LoginType.EMAIL))
return this.authSessionLoginConfirmAttemptsLimits.getEmailLimit();
if (loginType.equals(AuthSessionEntity.LoginType.USERNAME))
return this.authSessionLoginConfirmAttemptsLimits.getUsernameLimit();
if (loginType.equals(AuthSessionEntity.LoginType.PHONE_NUMBER))
return this.authSessionLoginConfirmAttemptsLimits.getPhoneNumberLimit();
return 0L;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy