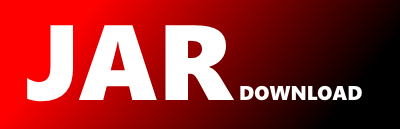
io.fusionauth.domain.UserRegistration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fusionauth-java-client Show documentation
Show all versions of fusionauth-java-client Show documentation
The Java Client library provides a native Java binding to the FusionAuth REST API.
/*
* Copyright (c) 2018-2020, FusionAuth, All Rights Reserved
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific
* language governing permissions and limitations under the License.
*/
package io.fusionauth.domain;
import java.time.ZoneId;
import java.time.ZonedDateTime;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Objects;
import java.util.SortedSet;
import java.util.TreeSet;
import java.util.UUID;
import com.inversoft.json.ToString;
import io.fusionauth.domain.internal._InternalJSONColumn;
import io.fusionauth.domain.internal.annotation.InternalJSONColumn;
import io.fusionauth.domain.util.Normalizer;
import static io.fusionauth.domain.util.Normalizer.trim;
import static io.fusionauth.domain.util.Normalizer.trimToNull;
/**
* User registration information for a single application.
*
* @author Brian Pontarelli
*/
public class UserRegistration implements Buildable, _InternalJSONColumn {
public final Map data;
@InternalJSONColumn
public final List preferredLanguages = new ArrayList<>();
/**
* @deprecated tokens are now stored in the Identity Provider Link. See the /api/identity-provider/link API.
*/
@Deprecated
@InternalJSONColumn
public final Map tokens;
public UUID applicationId;
public String authenticationToken;
public UUID cleanSpeakId;
public UUID id;
public ZonedDateTime insertInstant;
public ZonedDateTime lastLoginInstant;
public ZonedDateTime lastUpdateInstant;
public SortedSet roles = new TreeSet<>();
public ZoneId timezone;
public String username;
public ContentStatus usernameStatus;
public boolean verified;
public UserRegistration() {
this.data = new LinkedHashMap<>();
this.tokens = new LinkedHashMap<>();
}
public UserRegistration(Map mockData) {
this.data = mockData;
this.tokens = new LinkedHashMap<>();
}
public UserRegistration(UserRegistration userRegistration) {
this.applicationId = userRegistration.applicationId;
this.authenticationToken = userRegistration.authenticationToken;
this.cleanSpeakId = userRegistration.cleanSpeakId;
this.id = userRegistration.id;
this.insertInstant = userRegistration.insertInstant;
this.lastLoginInstant = userRegistration.lastLoginInstant;
this.lastUpdateInstant = userRegistration.lastUpdateInstant;
this.preferredLanguages.addAll(userRegistration.preferredLanguages);
this.roles.addAll(userRegistration.roles);
this.timezone = userRegistration.timezone;
this.username = userRegistration.username;
this.usernameStatus = userRegistration.usernameStatus;
this.verified = userRegistration.verified;
this.data = new LinkedHashMap<>();
if (userRegistration.data != null) {
this.data.putAll(userRegistration.data);
}
this.tokens = new LinkedHashMap<>();
if (userRegistration.tokens != null) {
this.tokens.putAll(userRegistration.tokens);
}
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof UserRegistration)) {
return false;
}
UserRegistration that = (UserRegistration) o;
return verified == that.verified &&
Objects.equals(data, that.data) &&
Objects.equals(preferredLanguages, that.preferredLanguages) &&
Objects.equals(tokens, that.tokens) &&
Objects.equals(applicationId, that.applicationId) &&
Objects.equals(authenticationToken, that.authenticationToken) &&
Objects.equals(cleanSpeakId, that.cleanSpeakId) &&
Objects.equals(id, that.id) &&
Objects.equals(insertInstant, that.insertInstant) &&
Objects.equals(lastUpdateInstant, that.lastUpdateInstant) &&
Objects.equals(lastLoginInstant, that.lastLoginInstant) &&
Objects.equals(roles, that.roles) &&
Objects.equals(timezone, that.timezone) &&
Objects.equals(username, that.username) &&
usernameStatus == that.usernameStatus;
}
/**
* Return true if user registration data is provided.
*
* @return true if user data exists.
*/
public boolean hasRegistrationData() {
return !data.isEmpty();
}
@Override
public int hashCode() {
return Objects.hash(data, preferredLanguages, tokens, applicationId, authenticationToken, cleanSpeakId, id, insertInstant, lastUpdateInstant, lastLoginInstant, roles, timezone, username, usernameStatus, verified);
}
public void normalize() {
authenticationToken = trimToNull(authenticationToken);
preferredLanguages.removeIf(Objects::isNull);
Normalizer.deDuplicate(preferredLanguages);
username = trim(username);
Normalizer.removeEmpty(data);
}
public String toString() {
return ToString.toString(this);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy