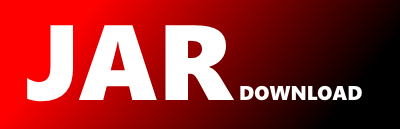
io.gdcc.xoai.services.impl.AbstractMetadataSearcher Maven / Gradle / Ivy
/*
* The contents of this file are subject to the license and copyright
* detailed in the LICENSE and NOTICE files at the root of the source
* tree and available online at
*
* http://www.dspace.org/license/
*/
package io.gdcc.xoai.services.impl;
import io.gdcc.xoai.model.xoai.Element;
import io.gdcc.xoai.model.xoai.XOAIMetadata;
import io.gdcc.xoai.services.api.MetadataSearch;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public abstract class AbstractMetadataSearcher implements MetadataSearch {
protected static final String DEFAULT_FIELD = "value";
protected Map> index = new HashMap<>();
protected AbstractMetadataSearcher(XOAIMetadata metadata) {
for (Element element : metadata.getElements()) {
consume(new ArrayList<>(), element);
}
}
@Override
public T findOne(String xoaiPath) {
List elements = index.get(xoaiPath);
if (elements != null && !elements.isEmpty()) return elements.get(0);
return null;
}
@Override
public List findAll(String xoaiPath) {
return index.get(xoaiPath);
}
@Override
public Map> index() {
return index;
}
protected void init(XOAIMetadata metadata) {
for (Element element : metadata.getElements()) {
consume(new ArrayList<>(), element);
}
}
protected abstract void consume(List newNames, Element element);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy