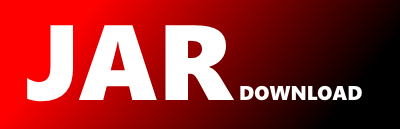
io.geewit.data.jpa.envers.repository.EnversRevisionMetadata Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gw-data-jpa-envers-repository Show documentation
Show all versions of gw-data-jpa-envers-repository Show documentation
A Java Utils Libraray By Geewit
The newest version!
package io.geewit.data.jpa.envers.repository;
import io.geewit.data.jpa.envers.EnversRevisionEntity;
import org.hibernate.envers.DefaultRevisionEntity;
import org.hibernate.envers.RevisionNumber;
import org.hibernate.envers.RevisionTimestamp;
import org.springframework.data.history.RevisionMetadata;
import org.springframework.data.util.AnnotationDetectionFieldCallback;
import org.springframework.data.util.Lazy;
import org.springframework.util.Assert;
import org.springframework.util.ReflectionUtils;
import java.io.Serializable;
import java.lang.annotation.Annotation;
import java.time.Instant;
import java.time.LocalDateTime;
import java.time.ZoneOffset;
import java.util.Date;
import java.util.Optional;
/**
* {@link RevisionMetadata} working with a {@link DefaultRevisionEntity}.
*
* @author Oliver Gierke
* @author Philip Huegelmeyer
* @author geewit
*/
public class EnversRevisionMetadata implements RevisionMetadata {
private final EnversRevisionEntity entity;
private final Lazy> revisionNumber;
private final Lazy> revisionDate;
/**
* Creates a new {@link EnversRevisionMetadata}.
*
* @param entity must not be {@literal null}.
*/
public EnversRevisionMetadata(EnversRevisionEntity entity) {
Assert.notNull(entity, "[Assertion failed] - DefaultRevisionEntity argument must be null");
this.entity = entity;
this.revisionNumber = detectAnnotation(entity, RevisionNumber.class);
this.revisionDate = detectAnnotation(entity, RevisionTimestamp.class);
}
/**
* (non-Javadoc)
* @see org.springframework.data.history.RevisionMetadata#getRevisionNumber()
*/
@Override
public Optional getRevisionNumber() {
return revisionNumber.get();
}
@Override
public Optional getRevisionInstant() {
return revisionDate.get().map(EnversRevisionMetadata::convertToInstant);
}
public O getOperatorId() {
return entity.getOperatorId();
}
public String getOperatorName() {
return entity.getOperatorName();
}
/**
* (non-Javadoc)
* @see org.springframework.data.history.RevisionMetadata#getDelegate()
*/
@SuppressWarnings("unchecked")
@Override
public T getDelegate() {
return (T) entity;
}
private static Lazy> detectAnnotation(Object entity, Class extends Annotation> annotationType) {
return Lazy.of(() -> {
AnnotationDetectionFieldCallback callback = new AnnotationDetectionFieldCallback(annotationType);
ReflectionUtils.doWithFields(entity.getClass(), callback);
return Optional.ofNullable(callback.getValue(entity));
});
}
private static Instant convertToInstant(Object timestamp) {
if (timestamp instanceof Instant) {
return (Instant) timestamp;
}
if (timestamp instanceof LocalDateTime) {
return ((LocalDateTime) timestamp).atZone(ZoneOffset.systemDefault()).toInstant();
}
if (timestamp instanceof Long) {
return Instant.ofEpochMilli((Long) timestamp);
}
if (timestamp instanceof Date) {
return ((Date) timestamp).toInstant();
}
throw new IllegalArgumentException(String.format("Can't convert %s to Instant!", timestamp));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy