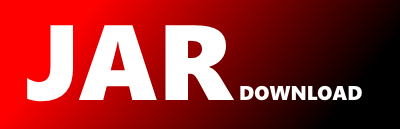
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceCallbackHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of powerauth-java-client-axis Show documentation
Show all versions of powerauth-java-client-axis Show documentation
PowerAuth Server SOAP Service Client - Axis
/**
* PowerAuthPortV3ServiceCallbackHandler.java
*
* This file was auto-generated from WSDL
* by the Apache Axis2 version: 1.7.9 Built on : Nov 16, 2018 (12:05:37 GMT)
*/
package io.getlime.powerauth.soap.v3;
/**
* PowerAuthPortV3ServiceCallbackHandler Callback class, Users can extend this class and implement
* their own receiveResult and receiveError methods.
*/
public abstract class PowerAuthPortV3ServiceCallbackHandler {
protected Object clientData;
/**
* User can pass in any object that needs to be accessed once the NonBlocking
* Web service call is finished and appropriate method of this CallBack is called.
* @param clientData Object mechanism by which the user can pass in user data
* that will be avilable at the time this callback is called.
*/
public PowerAuthPortV3ServiceCallbackHandler(Object clientData) {
this.clientData = clientData;
}
/**
* Please use this constructor if you don't want to set any clientData
*/
public PowerAuthPortV3ServiceCallbackHandler() {
this.clientData = null;
}
/**
* Get the client data
*/
public Object getClientData() {
return clientData;
}
/**
* auto generated Axis2 call back method for createApplication method
* override this method for handling normal response from createApplication operation
*/
public void receiveResultcreateApplication(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.CreateApplicationResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from createApplication operation
*/
public void receiveErrorcreateApplication(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for unblockActivation method
* override this method for handling normal response from unblockActivation operation
*/
public void receiveResultunblockActivation(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.UnblockActivationResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from unblockActivation operation
*/
public void receiveErrorunblockActivation(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for validateToken method
* override this method for handling normal response from validateToken operation
*/
public void receiveResultvalidateToken(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.ValidateTokenResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from validateToken operation
*/
public void receiveErrorvalidateToken(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for getEciesDecryptor method
* override this method for handling normal response from getEciesDecryptor operation
*/
public void receiveResultgetEciesDecryptor(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.GetEciesDecryptorResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from getEciesDecryptor operation
*/
public void receiveErrorgetEciesDecryptor(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for updateActivationOtp method
* override this method for handling normal response from updateActivationOtp operation
*/
public void receiveResultupdateActivationOtp(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.UpdateActivationOtpResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from updateActivationOtp operation
*/
public void receiveErrorupdateActivationOtp(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for createRecoveryCode method
* override this method for handling normal response from createRecoveryCode operation
*/
public void receiveResultcreateRecoveryCode(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.CreateRecoveryCodeResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from createRecoveryCode operation
*/
public void receiveErrorcreateRecoveryCode(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for lookupApplicationByAppKey method
* override this method for handling normal response from lookupApplicationByAppKey operation
*/
public void receiveResultlookupApplicationByAppKey(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.LookupApplicationByAppKeyResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from lookupApplicationByAppKey operation
*/
public void receiveErrorlookupApplicationByAppKey(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for lookupActivations method
* override this method for handling normal response from lookupActivations operation
*/
public void receiveResultlookupActivations(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.LookupActivationsResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from lookupActivations operation
*/
public void receiveErrorlookupActivations(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for verifyOfflineSignature method
* override this method for handling normal response from verifyOfflineSignature operation
*/
public void receiveResultverifyOfflineSignature(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.VerifyOfflineSignatureResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from verifyOfflineSignature operation
*/
public void receiveErrorverifyOfflineSignature(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for revokeRecoveryCodes method
* override this method for handling normal response from revokeRecoveryCodes operation
*/
public void receiveResultrevokeRecoveryCodes(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.RevokeRecoveryCodesResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from revokeRecoveryCodes operation
*/
public void receiveErrorrevokeRecoveryCodes(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for getErrorCodeList method
* override this method for handling normal response from getErrorCodeList operation
*/
public void receiveResultgetErrorCodeList(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.GetErrorCodeListResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from getErrorCodeList operation
*/
public void receiveErrorgetErrorCodeList(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for verifyECDSASignature method
* override this method for handling normal response from verifyECDSASignature operation
*/
public void receiveResultverifyECDSASignature(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.VerifyECDSASignatureResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from verifyECDSASignature operation
*/
public void receiveErrorverifyECDSASignature(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for activationHistory method
* override this method for handling normal response from activationHistory operation
*/
public void receiveResultactivationHistory(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.ActivationHistoryResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from activationHistory operation
*/
public void receiveErroractivationHistory(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for getApplicationList method
* override this method for handling normal response from getApplicationList operation
*/
public void receiveResultgetApplicationList(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.GetApplicationListResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from getApplicationList operation
*/
public void receiveErrorgetApplicationList(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for verifySignature method
* override this method for handling normal response from verifySignature operation
*/
public void receiveResultverifySignature(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.VerifySignatureResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from verifySignature operation
*/
public void receiveErrorverifySignature(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for getApplicationDetail method
* override this method for handling normal response from getApplicationDetail operation
*/
public void receiveResultgetApplicationDetail(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.GetApplicationDetailResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from getApplicationDetail operation
*/
public void receiveErrorgetApplicationDetail(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for getSystemStatus method
* override this method for handling normal response from getSystemStatus operation
*/
public void receiveResultgetSystemStatus(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.GetSystemStatusResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from getSystemStatus operation
*/
public void receiveErrorgetSystemStatus(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for createIntegration method
* override this method for handling normal response from createIntegration operation
*/
public void receiveResultcreateIntegration(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.CreateIntegrationResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from createIntegration operation
*/
public void receiveErrorcreateIntegration(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for getCallbackUrlList method
* override this method for handling normal response from getCallbackUrlList operation
*/
public void receiveResultgetCallbackUrlList(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.GetCallbackUrlListResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from getCallbackUrlList operation
*/
public void receiveErrorgetCallbackUrlList(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for createPersonalizedOfflineSignaturePayload method
* override this method for handling normal response from createPersonalizedOfflineSignaturePayload operation
*/
public void receiveResultcreatePersonalizedOfflineSignaturePayload(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.CreatePersonalizedOfflineSignaturePayloadResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from createPersonalizedOfflineSignaturePayload operation
*/
public void receiveErrorcreatePersonalizedOfflineSignaturePayload(
java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for createToken method
* override this method for handling normal response from createToken operation
*/
public void receiveResultcreateToken(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.CreateTokenResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from createToken operation
*/
public void receiveErrorcreateToken(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for blockActivation method
* override this method for handling normal response from blockActivation operation
*/
public void receiveResultblockActivation(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.BlockActivationResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from blockActivation operation
*/
public void receiveErrorblockActivation(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for removeCallbackUrl method
* override this method for handling normal response from removeCallbackUrl operation
*/
public void receiveResultremoveCallbackUrl(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.RemoveCallbackUrlResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from removeCallbackUrl operation
*/
public void receiveErrorremoveCallbackUrl(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for prepareActivation method
* override this method for handling normal response from prepareActivation operation
*/
public void receiveResultprepareActivation(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.PrepareActivationResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from prepareActivation operation
*/
public void receiveErrorprepareActivation(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for updateStatusForActivations method
* override this method for handling normal response from updateStatusForActivations operation
*/
public void receiveResultupdateStatusForActivations(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.UpdateStatusForActivationsResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from updateStatusForActivations operation
*/
public void receiveErrorupdateStatusForActivations(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for commitUpgrade method
* override this method for handling normal response from commitUpgrade operation
*/
public void receiveResultcommitUpgrade(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.CommitUpgradeResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from commitUpgrade operation
*/
public void receiveErrorcommitUpgrade(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for confirmRecoveryCode method
* override this method for handling normal response from confirmRecoveryCode operation
*/
public void receiveResultconfirmRecoveryCode(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.ConfirmRecoveryCodeResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from confirmRecoveryCode operation
*/
public void receiveErrorconfirmRecoveryCode(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for supportApplicationVersion method
* override this method for handling normal response from supportApplicationVersion operation
*/
public void receiveResultsupportApplicationVersion(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.SupportApplicationVersionResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from supportApplicationVersion operation
*/
public void receiveErrorsupportApplicationVersion(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for commitActivation method
* override this method for handling normal response from commitActivation operation
*/
public void receiveResultcommitActivation(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.CommitActivationResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from commitActivation operation
*/
public void receiveErrorcommitActivation(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for removeIntegration method
* override this method for handling normal response from removeIntegration operation
*/
public void receiveResultremoveIntegration(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.RemoveIntegrationResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from removeIntegration operation
*/
public void receiveErrorremoveIntegration(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for getRecoveryConfig method
* override this method for handling normal response from getRecoveryConfig operation
*/
public void receiveResultgetRecoveryConfig(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.GetRecoveryConfigResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from getRecoveryConfig operation
*/
public void receiveErrorgetRecoveryConfig(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for lookupRecoveryCodes method
* override this method for handling normal response from lookupRecoveryCodes operation
*/
public void receiveResultlookupRecoveryCodes(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.LookupRecoveryCodesResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from lookupRecoveryCodes operation
*/
public void receiveErrorlookupRecoveryCodes(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for getActivationStatus method
* override this method for handling normal response from getActivationStatus operation
*/
public void receiveResultgetActivationStatus(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.GetActivationStatusResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from getActivationStatus operation
*/
public void receiveErrorgetActivationStatus(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for unsupportApplicationVersion method
* override this method for handling normal response from unsupportApplicationVersion operation
*/
public void receiveResultunsupportApplicationVersion(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.UnsupportApplicationVersionResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from unsupportApplicationVersion operation
*/
public void receiveErrorunsupportApplicationVersion(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for recoveryCodeActivation method
* override this method for handling normal response from recoveryCodeActivation operation
*/
public void receiveResultrecoveryCodeActivation(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.RecoveryCodeActivationResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from recoveryCodeActivation operation
*/
public void receiveErrorrecoveryCodeActivation(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for createCallbackUrl method
* override this method for handling normal response from createCallbackUrl operation
*/
public void receiveResultcreateCallbackUrl(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.CreateCallbackUrlResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from createCallbackUrl operation
*/
public void receiveErrorcreateCallbackUrl(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for removeActivation method
* override this method for handling normal response from removeActivation operation
*/
public void receiveResultremoveActivation(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.RemoveActivationResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from removeActivation operation
*/
public void receiveErrorremoveActivation(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for vaultUnlock method
* override this method for handling normal response from vaultUnlock operation
*/
public void receiveResultvaultUnlock(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.VaultUnlockResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from vaultUnlock operation
*/
public void receiveErrorvaultUnlock(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for startUpgrade method
* override this method for handling normal response from startUpgrade operation
*/
public void receiveResultstartUpgrade(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.StartUpgradeResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from startUpgrade operation
*/
public void receiveErrorstartUpgrade(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for removeToken method
* override this method for handling normal response from removeToken operation
*/
public void receiveResultremoveToken(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.RemoveTokenResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from removeToken operation
*/
public void receiveErrorremoveToken(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for updateRecoveryConfig method
* override this method for handling normal response from updateRecoveryConfig operation
*/
public void receiveResultupdateRecoveryConfig(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.UpdateRecoveryConfigResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from updateRecoveryConfig operation
*/
public void receiveErrorupdateRecoveryConfig(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for createNonPersonalizedOfflineSignaturePayload method
* override this method for handling normal response from createNonPersonalizedOfflineSignaturePayload operation
*/
public void receiveResultcreateNonPersonalizedOfflineSignaturePayload(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.CreateNonPersonalizedOfflineSignaturePayloadResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from createNonPersonalizedOfflineSignaturePayload operation
*/
public void receiveErrorcreateNonPersonalizedOfflineSignaturePayload(
java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for getIntegrationList method
* override this method for handling normal response from getIntegrationList operation
*/
public void receiveResultgetIntegrationList(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.GetIntegrationListResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from getIntegrationList operation
*/
public void receiveErrorgetIntegrationList(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for initActivation method
* override this method for handling normal response from initActivation operation
*/
public void receiveResultinitActivation(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.InitActivationResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from initActivation operation
*/
public void receiveErrorinitActivation(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for signatureAudit method
* override this method for handling normal response from signatureAudit operation
*/
public void receiveResultsignatureAudit(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.SignatureAuditResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from signatureAudit operation
*/
public void receiveErrorsignatureAudit(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for createActivation method
* override this method for handling normal response from createActivation operation
*/
public void receiveResultcreateActivation(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.CreateActivationResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from createActivation operation
*/
public void receiveErrorcreateActivation(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for createApplicationVersion method
* override this method for handling normal response from createApplicationVersion operation
*/
public void receiveResultcreateApplicationVersion(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.CreateApplicationVersionResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from createApplicationVersion operation
*/
public void receiveErrorcreateApplicationVersion(java.lang.Exception e) {
}
/**
* auto generated Axis2 call back method for getActivationListForUser method
* override this method for handling normal response from getActivationListForUser operation
*/
public void receiveResultgetActivationListForUser(
io.getlime.powerauth.soap.v3.PowerAuthPortV3ServiceStub.GetActivationListForUserResponse result) {
}
/**
* auto generated Axis2 Error handler
* override this method for handling error response from getActivationListForUser operation
*/
public void receiveErrorgetActivationListForUser(java.lang.Exception e) {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy