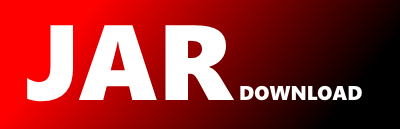
io.getstream.client.BatchClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stream-java Show documentation
Show all versions of stream-java Show documentation
Stream Feeds Java Client for backend and android integrations
package io.getstream.client;
import static io.getstream.core.utils.Auth.*;
import com.google.common.collect.Iterables;
import io.getstream.core.KeepHistory;
import io.getstream.core.StreamBatch;
import io.getstream.core.exceptions.StreamException;
import io.getstream.core.http.Token;
import io.getstream.core.models.*;
import io.getstream.core.options.EnrichmentFlags;
import io.getstream.core.utils.DefaultOptions;
import java.util.List;
import java8.util.J8Arrays;
import java8.util.concurrent.CompletableFuture;
public final class BatchClient {
private final String secret;
private final StreamBatch batch;
BatchClient(String secret, StreamBatch batch) {
this.secret = secret;
this.batch = batch;
}
public CompletableFuture addToMany(Activity activity, FeedID... feeds)
throws StreamException {
final Token token = buildFeedToken(secret, TokenAction.WRITE);
return batch.addToMany(token, activity, feeds);
}
public CompletableFuture followMany(int activityCopyLimit, FollowRelation... follows)
throws StreamException {
final Token token = buildFollowToken(secret, TokenAction.WRITE);
return batch.followMany(token, activityCopyLimit, follows);
}
public CompletableFuture followMany(int activityCopyLimit, Iterable follows)
throws StreamException {
return followMany(activityCopyLimit, Iterables.toArray(follows, FollowRelation.class));
}
public CompletableFuture followMany(FollowRelation... follows) throws StreamException {
return followMany(DefaultOptions.DEFAULT_ACTIVITY_COPY_LIMIT, follows);
}
public CompletableFuture followMany(Iterable follows)
throws StreamException {
return followMany(Iterables.toArray(follows, FollowRelation.class));
}
public CompletableFuture unfollowMany(FollowRelation... follows) throws StreamException {
final Token token = buildFollowToken(secret, TokenAction.WRITE);
final UnfollowOperation[] ops =
J8Arrays.stream(follows)
.map(follow -> new UnfollowOperation(follow, io.getstream.core.KeepHistory.YES))
.toArray(UnfollowOperation[]::new);
return batch.unfollowMany(token, ops);
}
public CompletableFuture unfollowMany(KeepHistory keepHistory, FollowRelation... follows)
throws StreamException {
final Token token = buildFollowToken(secret, TokenAction.WRITE);
final UnfollowOperation[] ops =
J8Arrays.stream(follows)
.map(follow -> new UnfollowOperation(follow, keepHistory))
.toArray(UnfollowOperation[]::new);
return batch.unfollowMany(token, ops);
}
public CompletableFuture unfollowMany(UnfollowOperation... unfollows)
throws StreamException {
final Token token = buildFollowToken(secret, TokenAction.WRITE);
return batch.unfollowMany(token, unfollows);
}
public CompletableFuture> getActivitiesByID(Iterable activityIDs)
throws StreamException {
return getActivitiesByID(Iterables.toArray(activityIDs, String.class));
}
public CompletableFuture> getActivitiesByID(String... activityIDs)
throws StreamException {
final Token token = buildActivityToken(secret, TokenAction.READ);
return batch.getActivitiesByID(token, activityIDs);
}
public CompletableFuture> getEnrichedActivitiesByID(
Iterable activityIDs) throws StreamException {
return getEnrichedActivitiesByID(Iterables.toArray(activityIDs, String.class));
}
public CompletableFuture> getEnrichedActivitiesByID(String... activityIDs)
throws StreamException {
return getEnrichedActivitiesByID(DefaultOptions.DEFAULT_ENRICHMENT_FLAGS, activityIDs);
}
public CompletableFuture> getEnrichedActivitiesByID(
EnrichmentFlags flags, String... activityIDs) throws StreamException {
final Token token = buildActivityToken(secret, TokenAction.READ);
return batch.getEnrichedActivitiesByID(token, flags, activityIDs);
}
public CompletableFuture> getActivitiesByForeignID(
Iterable activityIDTimePairs) throws StreamException {
return getActivitiesByForeignID(
Iterables.toArray(activityIDTimePairs, ForeignIDTimePair.class));
}
public CompletableFuture> getActivitiesByForeignID(
ForeignIDTimePair... activityIDTimePairs) throws StreamException {
final Token token = buildActivityToken(secret, TokenAction.READ);
return batch.getActivitiesByForeignID(token, activityIDTimePairs);
}
public CompletableFuture> getEnrichedActivitiesByForeignID(
Iterable activityIDTimePairs) throws StreamException {
return getEnrichedActivitiesByForeignID(
Iterables.toArray(activityIDTimePairs, ForeignIDTimePair.class));
}
public CompletableFuture> getEnrichedActivitiesByForeignID(
ForeignIDTimePair... activityIDTimePairs) throws StreamException {
final Token token = buildActivityToken(secret, TokenAction.READ);
return batch.getEnrichedActivitiesByForeignID(token, activityIDTimePairs);
}
public CompletableFuture updateActivities(Iterable activities)
throws StreamException {
return updateActivities(Iterables.toArray(activities, Activity.class));
}
public CompletableFuture updateActivities(Activity... activities) throws StreamException {
final Token token = buildActivityToken(secret, TokenAction.WRITE);
return batch.updateActivities(token, activities);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy