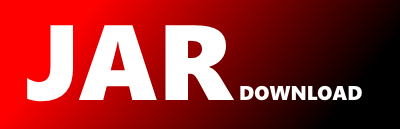
io.getstream.client.NotificationFeed Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stream-java Show documentation
Show all versions of stream-java Show documentation
Stream Feeds Java Client for backend and android integrations
package io.getstream.client;
import static io.getstream.core.utils.Serialization.*;
import com.fasterxml.jackson.core.type.TypeReference;
import io.getstream.core.exceptions.StreamException;
import io.getstream.core.models.*;
import io.getstream.core.options.*;
import io.getstream.core.utils.DefaultOptions;
import java.io.IOException;
import java8.util.concurrent.CompletableFuture;
import java8.util.concurrent.CompletionException;
public final class NotificationFeed extends Feed {
NotificationFeed(Client client, FeedID id) {
super(client, id);
}
public CompletableFuture> getActivities()
throws StreamException {
return getActivities(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getActivities(Limit limit)
throws StreamException {
return getActivities(
limit,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getActivities(Offset offset)
throws StreamException {
return getActivities(
DefaultOptions.DEFAULT_LIMIT,
offset,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getActivities(Filter filter)
throws StreamException {
return getActivities(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
filter,
DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getActivities(
ActivityMarker marker) throws StreamException {
return getActivities(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
marker);
}
public CompletableFuture> getActivities(
Limit limit, Offset offset) throws StreamException {
return getActivities(
limit, offset, DefaultOptions.DEFAULT_FILTER, DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getActivities(
Limit limit, Filter filter) throws StreamException {
return getActivities(
limit, DefaultOptions.DEFAULT_OFFSET, filter, DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getActivities(
Limit limit, ActivityMarker marker) throws StreamException {
return getActivities(
limit, DefaultOptions.DEFAULT_OFFSET, DefaultOptions.DEFAULT_FILTER, marker);
}
public CompletableFuture> getActivities(
Filter filter, ActivityMarker marker) throws StreamException {
return getActivities(
DefaultOptions.DEFAULT_LIMIT, DefaultOptions.DEFAULT_OFFSET, filter, marker);
}
public CompletableFuture> getActivities(
Offset offset, ActivityMarker marker) throws StreamException {
return getActivities(
DefaultOptions.DEFAULT_LIMIT, offset, DefaultOptions.DEFAULT_FILTER, marker);
}
public CompletableFuture> getActivities(
Limit limit, Filter filter, ActivityMarker marker) throws StreamException {
return getActivities(limit, DefaultOptions.DEFAULT_OFFSET, filter, marker);
}
public CompletableFuture> getActivities(
Limit limit, Offset offset, ActivityMarker marker) throws StreamException {
return getActivities(limit, offset, DefaultOptions.DEFAULT_FILTER, marker);
}
CompletableFuture> getActivities(
Limit limit, Offset offset, Filter filter, ActivityMarker marker) throws StreamException {
return getClient()
.getActivities(getID(), limit, offset, filter, marker)
.thenApply(
response -> {
try {
return deserialize(
response, new TypeReference>() {});
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public CompletableFuture> getCustomActivities(Class type)
throws StreamException {
return getCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getCustomActivities(
Class type, Limit limit) throws StreamException {
return getCustomActivities(
type,
limit,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getCustomActivities(
Class type, Offset offset) throws StreamException {
return getCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
offset,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getCustomActivities(
Class type, Filter filter) throws StreamException {
return getCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
filter,
DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getCustomActivities(
Class type, ActivityMarker marker) throws StreamException {
return getCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
marker);
}
public CompletableFuture> getCustomActivities(
Class type, Limit limit, Offset offset) throws StreamException {
return getCustomActivities(
type, limit, offset, DefaultOptions.DEFAULT_FILTER, DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getCustomActivities(
Class type, Limit limit, Filter filter) throws StreamException {
return getCustomActivities(
type, limit, DefaultOptions.DEFAULT_OFFSET, filter, DefaultOptions.DEFAULT_MARKER);
}
public CompletableFuture> getCustomActivities(
Class type, Limit limit, ActivityMarker marker) throws StreamException {
return getCustomActivities(
type, limit, DefaultOptions.DEFAULT_OFFSET, DefaultOptions.DEFAULT_FILTER, marker);
}
public CompletableFuture> getCustomActivities(
Class type, Filter filter, ActivityMarker marker) throws StreamException {
return getCustomActivities(
type, DefaultOptions.DEFAULT_LIMIT, DefaultOptions.DEFAULT_OFFSET, filter, marker);
}
public CompletableFuture> getCustomActivities(
Class type, Offset offset, ActivityMarker marker) throws StreamException {
return getCustomActivities(
type, DefaultOptions.DEFAULT_LIMIT, offset, DefaultOptions.DEFAULT_FILTER, marker);
}
public CompletableFuture> getCustomActivities(
Class type, Limit limit, Filter filter, ActivityMarker marker) throws StreamException {
return getCustomActivities(type, limit, DefaultOptions.DEFAULT_OFFSET, filter, marker);
}
public CompletableFuture> getCustomActivities(
Class type, Limit limit, Offset offset, ActivityMarker marker) throws StreamException {
return getCustomActivities(type, limit, offset, DefaultOptions.DEFAULT_FILTER, marker);
}
CompletableFuture> getCustomActivities(
Class type, Limit limit, Offset offset, Filter filter, ActivityMarker marker)
throws StreamException {
return getClient()
.getActivities(getID(), limit, offset, filter, marker)
.thenApply(
response -> {
try {
return deserialize(response, new TypeReference>() {});
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public CompletableFuture> getEnrichedActivities()
throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
Limit limit) throws StreamException {
return getEnrichedActivities(
limit,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
EnrichmentFlags flags) throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
flags);
}
public CompletableFuture> getEnrichedActivities(
Offset offset) throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT,
offset,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
Filter filter) throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
filter,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
ActivityMarker marker) throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
Limit limit, EnrichmentFlags flags) throws StreamException {
return getEnrichedActivities(
limit,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
flags);
}
public CompletableFuture> getEnrichedActivities(
Limit limit, Offset offset) throws StreamException {
return getEnrichedActivities(
limit,
offset,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
Limit limit, Filter filter) throws StreamException {
return getEnrichedActivities(
limit,
DefaultOptions.DEFAULT_OFFSET,
filter,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
Limit limit, ActivityMarker marker) throws StreamException {
return getEnrichedActivities(
limit,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
Offset offset, EnrichmentFlags flags) throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT,
offset,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
flags);
}
public CompletableFuture> getEnrichedActivities(
Filter filter, EnrichmentFlags flags) throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
filter,
DefaultOptions.DEFAULT_MARKER,
flags);
}
public CompletableFuture> getEnrichedActivities(
ActivityMarker marker, EnrichmentFlags flags) throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
marker,
flags);
}
public CompletableFuture> getEnrichedActivities(
Filter filter, ActivityMarker marker) throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
filter,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
Offset offset, ActivityMarker marker) throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT,
offset,
DefaultOptions.DEFAULT_FILTER,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
Limit limit, Offset offset, EnrichmentFlags flags) throws StreamException {
return getEnrichedActivities(
limit, offset, DefaultOptions.DEFAULT_FILTER, DefaultOptions.DEFAULT_MARKER, flags);
}
public CompletableFuture> getEnrichedActivities(
Limit limit, Filter filter, EnrichmentFlags flags) throws StreamException {
return getEnrichedActivities(
limit, DefaultOptions.DEFAULT_OFFSET, filter, DefaultOptions.DEFAULT_MARKER, flags);
}
public CompletableFuture> getEnrichedActivities(
Limit limit, ActivityMarker marker, EnrichmentFlags flags) throws StreamException {
return getEnrichedActivities(
limit, DefaultOptions.DEFAULT_OFFSET, DefaultOptions.DEFAULT_FILTER, marker, flags);
}
public CompletableFuture> getEnrichedActivities(
Limit limit, Filter filter, ActivityMarker marker) throws StreamException {
return getEnrichedActivities(
limit,
DefaultOptions.DEFAULT_OFFSET,
filter,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
Limit limit, Offset offset, ActivityMarker marker) throws StreamException {
return getEnrichedActivities(
limit,
offset,
DefaultOptions.DEFAULT_FILTER,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedActivities(
Filter filter, ActivityMarker marker, EnrichmentFlags flags) throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT, DefaultOptions.DEFAULT_OFFSET, filter, marker, flags);
}
public CompletableFuture> getEnrichedActivities(
Offset offset, ActivityMarker marker, EnrichmentFlags flags) throws StreamException {
return getEnrichedActivities(
DefaultOptions.DEFAULT_LIMIT, offset, DefaultOptions.DEFAULT_FILTER, marker, flags);
}
public CompletableFuture> getEnrichedActivities(
Limit limit, Filter filter, ActivityMarker marker, EnrichmentFlags flags)
throws StreamException {
return getEnrichedActivities(limit, DefaultOptions.DEFAULT_OFFSET, filter, marker, flags);
}
public CompletableFuture> getEnrichedActivities(
Limit limit, Offset offset, ActivityMarker marker, EnrichmentFlags flags)
throws StreamException {
return getEnrichedActivities(limit, offset, DefaultOptions.DEFAULT_FILTER, marker, flags);
}
CompletableFuture> getEnrichedActivities(
Limit limit, Offset offset, Filter filter, ActivityMarker marker, EnrichmentFlags flags)
throws StreamException {
return getClient()
.getEnrichedActivities(getID(), limit, offset, filter, marker, flags)
.thenApply(
response -> {
try {
return deserialize(
response, new TypeReference>() {});
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public CompletableFuture> getEnrichedCustomActivities(
Class type) throws StreamException {
return getEnrichedCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit) throws StreamException {
return getEnrichedCustomActivities(
type,
limit,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, EnrichmentFlags flags) throws StreamException {
return getEnrichedCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
flags);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Offset offset) throws StreamException {
return getEnrichedCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
offset,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Filter filter) throws StreamException {
return getEnrichedCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
filter,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, ActivityMarker marker) throws StreamException {
return getEnrichedCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit, EnrichmentFlags flags) throws StreamException {
return getEnrichedCustomActivities(
type,
limit,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
flags);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit, Offset offset) throws StreamException {
return getEnrichedCustomActivities(
type,
limit,
offset,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit, Filter filter) throws StreamException {
return getEnrichedCustomActivities(
type,
limit,
DefaultOptions.DEFAULT_OFFSET,
filter,
DefaultOptions.DEFAULT_MARKER,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit, ActivityMarker marker) throws StreamException {
return getEnrichedCustomActivities(
type,
limit,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Offset offset, EnrichmentFlags flags) throws StreamException {
return getEnrichedCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
offset,
DefaultOptions.DEFAULT_FILTER,
DefaultOptions.DEFAULT_MARKER,
flags);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Filter filter, EnrichmentFlags flags) throws StreamException {
return getEnrichedCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
filter,
DefaultOptions.DEFAULT_MARKER,
flags);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, ActivityMarker marker, EnrichmentFlags flags) throws StreamException {
return getEnrichedCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
DefaultOptions.DEFAULT_FILTER,
marker,
flags);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Filter filter, ActivityMarker marker) throws StreamException {
return getEnrichedCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
filter,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Offset offset, ActivityMarker marker) throws StreamException {
return getEnrichedCustomActivities(
type,
DefaultOptions.DEFAULT_LIMIT,
offset,
DefaultOptions.DEFAULT_FILTER,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit, Offset offset, EnrichmentFlags flags) throws StreamException {
return getEnrichedCustomActivities(
type, limit, offset, DefaultOptions.DEFAULT_FILTER, DefaultOptions.DEFAULT_MARKER, flags);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit, Filter filter, EnrichmentFlags flags) throws StreamException {
return getEnrichedCustomActivities(
type, limit, DefaultOptions.DEFAULT_OFFSET, filter, DefaultOptions.DEFAULT_MARKER, flags);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit, ActivityMarker marker, EnrichmentFlags flags)
throws StreamException {
return getEnrichedCustomActivities(
type, limit, DefaultOptions.DEFAULT_OFFSET, DefaultOptions.DEFAULT_FILTER, marker, flags);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit, Filter filter, ActivityMarker marker) throws StreamException {
return getEnrichedCustomActivities(
type,
limit,
DefaultOptions.DEFAULT_OFFSET,
filter,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit, Offset offset, ActivityMarker marker) throws StreamException {
return getEnrichedCustomActivities(
type,
limit,
offset,
DefaultOptions.DEFAULT_FILTER,
marker,
DefaultOptions.DEFAULT_ENRICHMENT_FLAGS);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Filter filter, ActivityMarker marker, EnrichmentFlags flags)
throws StreamException {
return getEnrichedCustomActivities(
type, DefaultOptions.DEFAULT_LIMIT, DefaultOptions.DEFAULT_OFFSET, filter, marker, flags);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Offset offset, ActivityMarker marker, EnrichmentFlags flags)
throws StreamException {
return getEnrichedCustomActivities(
type, DefaultOptions.DEFAULT_LIMIT, offset, DefaultOptions.DEFAULT_FILTER, marker, flags);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit, Filter filter, ActivityMarker marker, EnrichmentFlags flags)
throws StreamException {
return getEnrichedCustomActivities(
type, limit, DefaultOptions.DEFAULT_OFFSET, filter, marker, flags);
}
public CompletableFuture> getEnrichedCustomActivities(
Class type, Limit limit, Offset offset, ActivityMarker marker, EnrichmentFlags flags)
throws StreamException {
return getEnrichedCustomActivities(
type, limit, offset, DefaultOptions.DEFAULT_FILTER, marker, flags);
}
CompletableFuture> getEnrichedCustomActivities(
Class type,
Limit limit,
Offset offset,
Filter filter,
ActivityMarker marker,
EnrichmentFlags flags)
throws StreamException {
return getClient()
.getEnrichedActivities(getID(), limit, offset, filter, marker, flags)
.thenApply(
response -> {
try {
return deserialize(response, new TypeReference>() {});
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy