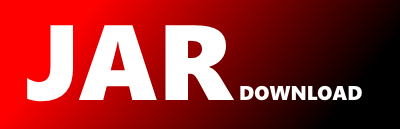
io.getstream.cloud.CloudFeed Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stream-java Show documentation
Show all versions of stream-java Show documentation
Stream Feeds Java Client for backend and android integrations
package io.getstream.cloud;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Preconditions.checkNotNull;
import static io.getstream.core.utils.Serialization.*;
import com.google.common.collect.Iterables;
import io.getstream.core.exceptions.StreamException;
import io.getstream.core.faye.subscription.ChannelSubscription;
import io.getstream.core.http.Response;
import io.getstream.core.models.Activity;
import io.getstream.core.models.FeedID;
import io.getstream.core.models.FollowRelation;
import io.getstream.core.options.CustomQueryParameter;
import io.getstream.core.options.Limit;
import io.getstream.core.options.Offset;
import io.getstream.core.options.RequestOption;
import io.getstream.core.utils.DefaultOptions;
import io.getstream.core.utils.Streams;
import java.io.IOException;
import java.lang.reflect.ParameterizedType;
import java.util.List;
import java8.util.J8Arrays;
import java8.util.concurrent.CompletableFuture;
import java8.util.concurrent.CompletionException;
public class CloudFeed {
private final CloudClient client;
private final FeedID id;
private final FeedSubscriber subscriber;
CloudFeed(CloudClient client, FeedID id) {
checkNotNull(client, "Can't create feed w/o a client");
checkNotNull(id, "Can't create feed w/o an ID");
this.client = client;
this.id = id;
this.subscriber = null;
}
CloudFeed(CloudClient client, FeedID id, FeedSubscriber subscriber) {
checkNotNull(client, "Can't create feed w/o a client");
checkNotNull(id, "Can't create feed w/o an ID");
this.client = client;
this.id = id;
this.subscriber = subscriber;
}
protected final CloudClient getClient() {
return client;
}
public final CompletableFuture subscribe(
RealtimeMessageCallback messageCallback) {
checkNotNull(subscriber, "A subscriber must be provided in order to start listening to a feed");
return subscriber.subscribe(id, messageCallback);
}
public final FeedID getID() {
return id;
}
public final String getSlug() {
return id.getSlug();
}
public final String getUserID() {
return id.getUserID();
}
public final CompletableFuture addActivity(Activity activity) throws StreamException {
return getClient()
.addActivity(id, activity)
.thenApply(
response -> {
try {
return deserialize(response, Activity.class);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public final CompletableFuture addCustomActivity(T activity) throws StreamException {
return getClient()
.addActivity(id, Activity.builder().fromCustomActivity(activity).build())
.thenApply(
response -> {
try {
return deserialize(response, (Class) activity.getClass());
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public final CompletableFuture> addActivities(Iterable activities)
throws StreamException {
return addActivities(Iterables.toArray(activities, Activity.class));
}
public final CompletableFuture> addCustomActivities(Iterable activities)
throws StreamException {
final Activity[] custom =
Streams.stream(activities)
.map(activity -> Activity.builder().fromCustomActivity(activity).build())
.toArray(Activity[]::new);
return getClient()
.addActivities(id, custom)
.thenApply(
(Response response) -> {
try {
Class element =
(Class)
((ParameterizedType) getClass().getGenericSuperclass())
.getActualTypeArguments()[0];
return deserializeContainer(response, "activities", element);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public final CompletableFuture> addActivities(Activity... activities)
throws StreamException {
return getClient()
.addActivities(id, activities)
.thenApply(
(Response response) -> {
try {
return deserializeContainer(response, "activities", Activity.class);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public final CompletableFuture> addCustomActivities(T... activities)
throws StreamException {
final Activity[] custom =
J8Arrays.stream(activities)
.map(activity -> Activity.builder().fromCustomActivity(activity).build())
.toArray(Activity[]::new);
return getClient()
.addActivities(id, custom)
.thenApply(
(Response response) -> {
try {
Class element = (Class) activities.getClass().getComponentType();
return deserializeContainer(response, "activities", element);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public final CompletableFuture removeActivityByID(String id) throws StreamException {
return client
.removeActivityByID(this.id, id)
.thenApply(
(Response response) -> {
try {
return deserializeError(response);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public final CompletableFuture removeActivityByForeignID(String foreignID)
throws StreamException {
return client
.removeActivityByForeignID(id, foreignID)
.thenApply(
(Response response) -> {
try {
return deserializeError(response);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public final CompletableFuture follow(CloudFlatFeed feed) throws StreamException {
return follow(feed, DefaultOptions.DEFAULT_ACTIVITY_COPY_LIMIT);
}
public final CompletableFuture follow(CloudFlatFeed feed, int activityCopyLimit)
throws StreamException {
checkArgument(
activityCopyLimit <= DefaultOptions.MAX_ACTIVITY_COPY_LIMIT,
String.format(
"Activity copy limit should be less then %d", DefaultOptions.MAX_ACTIVITY_COPY_LIMIT));
return client
.follow(id, feed.getID(), activityCopyLimit)
.thenApply(
(Response response) -> {
try {
return deserializeError(response);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public final CompletableFuture> getFollowers(Iterable feedIDs)
throws StreamException {
return getFollowers(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
Iterables.toArray(feedIDs, FeedID.class));
}
public final CompletableFuture> getFollowers(FeedID... feedIDs)
throws StreamException {
return getFollowers(DefaultOptions.DEFAULT_LIMIT, DefaultOptions.DEFAULT_OFFSET, feedIDs);
}
public final CompletableFuture> getFollowers(
Limit limit, Iterable feedIDs) throws StreamException {
return getFollowers(
limit, DefaultOptions.DEFAULT_OFFSET, Iterables.toArray(feedIDs, FeedID.class));
}
public final CompletableFuture> getFollowers(Limit limit, FeedID... feedIDs)
throws StreamException {
return getFollowers(limit, DefaultOptions.DEFAULT_OFFSET, feedIDs);
}
public final CompletableFuture> getFollowers(
Offset offset, Iterable feedIDs) throws StreamException {
return getFollowers(
DefaultOptions.DEFAULT_LIMIT, offset, Iterables.toArray(feedIDs, FeedID.class));
}
public final CompletableFuture> getFollowers(
Offset offset, FeedID... feedIDs) throws StreamException {
return getFollowers(DefaultOptions.DEFAULT_LIMIT, offset, feedIDs);
}
public final CompletableFuture> getFollowers(
Limit limit, Offset offset, Iterable feedIDs) throws StreamException {
return getFollowers(limit, offset, Iterables.toArray(feedIDs, FeedID.class));
}
public final CompletableFuture> getFollowers(
Limit limit, Offset offset, FeedID... feeds) throws StreamException {
checkNotNull(feeds, "No feed ids to filter on");
final String[] feedIDs = J8Arrays.stream(feeds).map(id -> id.toString()).toArray(String[]::new);
final RequestOption[] options =
feedIDs.length == 0
? new RequestOption[] {limit, offset}
: new RequestOption[] {
limit, offset, new CustomQueryParameter("filter", String.join(",", feedIDs))
};
return client
.getFollowers(id, options)
.thenApply(
(Response response) -> {
try {
return deserializeContainer(response, FollowRelation.class);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public final CompletableFuture> getFollowed(Iterable feedIDs)
throws StreamException {
return getFollowed(
DefaultOptions.DEFAULT_LIMIT,
DefaultOptions.DEFAULT_OFFSET,
Iterables.toArray(feedIDs, FeedID.class));
}
public final CompletableFuture> getFollowed(FeedID... feedIDs)
throws StreamException {
return getFollowed(DefaultOptions.DEFAULT_LIMIT, DefaultOptions.DEFAULT_OFFSET, feedIDs);
}
public final CompletableFuture> getFollowed(
Limit limit, Iterable feedIDs) throws StreamException {
return getFollowed(
limit, DefaultOptions.DEFAULT_OFFSET, Iterables.toArray(feedIDs, FeedID.class));
}
public final CompletableFuture> getFollowed(Limit limit, FeedID... feedIDs)
throws StreamException {
return getFollowed(limit, DefaultOptions.DEFAULT_OFFSET, feedIDs);
}
public final CompletableFuture> getFollowed(
Offset offset, Iterable feedIDs) throws StreamException {
return getFollowed(
DefaultOptions.DEFAULT_LIMIT, offset, Iterables.toArray(feedIDs, FeedID.class));
}
public final CompletableFuture> getFollowed(Offset offset, FeedID... feedIDs)
throws StreamException {
return getFollowed(DefaultOptions.DEFAULT_LIMIT, offset, feedIDs);
}
public final CompletableFuture> getFollowed(
Limit limit, Offset offset, Iterable feedIDs) throws StreamException {
return getFollowed(limit, offset, Iterables.toArray(feedIDs, FeedID.class));
}
public final CompletableFuture> getFollowed(
Limit limit, Offset offset, FeedID... feeds) throws StreamException {
checkNotNull(feeds, "No feed ids to filter on");
final String[] feedIDs = J8Arrays.stream(feeds).map(id -> id.toString()).toArray(String[]::new);
final RequestOption[] options =
feedIDs.length == 0
? new RequestOption[] {limit, offset}
: new RequestOption[] {
limit, offset, new CustomQueryParameter("filter", String.join(",", feedIDs))
};
return client
.getFollowed(id, options)
.thenApply(
(Response response) -> {
try {
return deserializeContainer(response, FollowRelation.class);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public final CompletableFuture unfollow(CloudFlatFeed feed) throws StreamException {
return unfollow(feed, io.getstream.core.KeepHistory.NO);
}
public final CompletableFuture unfollow(
CloudFlatFeed feed, io.getstream.core.KeepHistory keepHistory) throws StreamException {
return client
.unfollow(id, feed.getID(), new io.getstream.core.options.KeepHistory(keepHistory))
.thenApply(
(Response response) -> {
try {
return deserializeError(response);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy