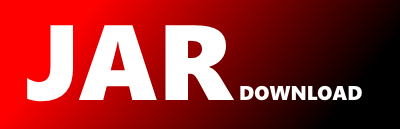
io.getstream.cloud.CloudUser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stream-java Show documentation
Show all versions of stream-java Show documentation
Stream Feeds Java Client for backend and android integrations
package io.getstream.cloud;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Preconditions.checkNotNull;
import static io.getstream.core.utils.Serialization.deserialize;
import static io.getstream.core.utils.Serialization.deserializeError;
import io.getstream.core.exceptions.StreamException;
import io.getstream.core.models.Data;
import io.getstream.core.models.ProfileData;
import java.io.IOException;
import java8.util.concurrent.CompletableFuture;
import java8.util.concurrent.CompletionException;
public final class CloudUser {
private final CloudClient client;
private final String id;
public CloudUser(CloudClient client, String id) {
checkNotNull(client, "Client can't be null");
checkNotNull(id, "User ID can't be null");
checkArgument(!id.isEmpty(), "User ID can't be empty");
this.client = client;
this.id = id;
}
public String getID() {
return id;
}
public CompletableFuture get() throws StreamException {
return client
.getUser(id)
.thenApply(
response -> {
try {
return deserialize(response, Data.class);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public CompletableFuture delete() throws StreamException {
return client
.deleteUser(id)
.thenApply(
response -> {
try {
return deserializeError(response);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public CompletableFuture getOrCreate() throws StreamException {
return getOrCreate(new Data());
}
public CompletableFuture getOrCreate(Data data) throws StreamException {
return client
.getOrCreateUser(id, data)
.thenApply(
response -> {
try {
return deserialize(response, Data.class);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public CompletableFuture create(Data data) throws StreamException {
return client
.createUser(id, data)
.thenApply(
response -> {
try {
return deserialize(response, Data.class);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public CompletableFuture create() throws StreamException {
return create(new Data());
}
public CompletableFuture update(Data data) throws StreamException {
return client
.updateUser(id, data)
.thenApply(
response -> {
try {
return deserialize(response, Data.class);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
public CompletableFuture profile() throws StreamException {
return client
.userProfile(id)
.thenApply(
response -> {
try {
return deserialize(response, ProfileData.class);
} catch (StreamException | IOException e) {
throw new CompletionException(e);
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy