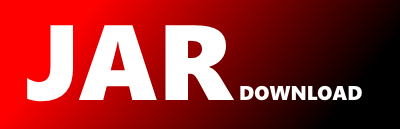
io.ghostwriter.GhostWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ghostwriter-api-java Show documentation
Show all versions of ghostwriter-api-java Show documentation
API for GhostWriter management and modules
package io.ghostwriter;
import java.util.ServiceLoader;
/**
* @author ghostwriter.io
*/
public enum GhostWriter {
INSTANCE;
private io.ghostwriter.TracerProvider extends Tracer> tracerProvider;
GhostWriter() {
tracerProvider = initialize();
}
public static void setTracerProvider(TracerProvider extends Tracer> tp) {
if (tp == null) {
throw new NullPointerException("Must provide a valid instance!");
}
Tracer tracer = tp.getTracer();
INSTANCE.tracerProvider = tp;
System.out.println("GWRT: Initialized with Tracer implementation: " + tracer.getClass().getCanonicalName());
}
public static void entering(Object source, String method, Object... params) {
Tracer tracer = INSTANCE.tracerProvider.getTracer();
tracer.entering(source, method, params);
}
public static void exiting(Object source, String method, Object retVal) {
Tracer tracer = INSTANCE.tracerProvider.getTracer();
tracer.exiting(source, method, retVal);
}
public static void exiting(Object source, String method) {
Tracer tracer = INSTANCE.tracerProvider.getTracer();
tracer.exiting(source, method);
}
public static void valueChange(Object source, String method, String variable, Object value) {
Tracer tracer = INSTANCE.tracerProvider.getTracer();
tracer.valueChange(source, method, variable, value);
}
public static void onError(Object source, String method, Throwable error) {
Tracer tracer = INSTANCE.tracerProvider.getTracer();
tracer.onError(source, method, error);
}
@SuppressWarnings({"rawtypes"})
private TracerProvider> initialize() {
TracerProvider foundProv = null;
ServiceLoader serviceLoader = ServiceLoader.load(TracerProvider.class);
for (TracerProvider tracerProvider : serviceLoader) {
Tracer tracer = tracerProvider.getTracer();
if (foundProv == null
&& !(tracerProvider instanceof NoopTracerProvider)
&& tracer != null) {
foundProv = tracerProvider;
// FIXME (snorbi07): this should be enabled using a DEBUG environmental variable or something
System.out.println("GWRT: Initialized with Tracer implementation: " + tracer.getClass().getCanonicalName());
} else if (tracer != null) {
// FIXME (snorbi07): this should be enabled using a DEBUG environmental variable or something
System.out.println("GWRT: Found other tracer implementation: "
+ tracer.getClass().getCanonicalName());
}
}
if (foundProv != null) {
return foundProv;
}
System.out.println("GWRT: Using the default no-op implementation!");
return new NoopTracerProvider();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy