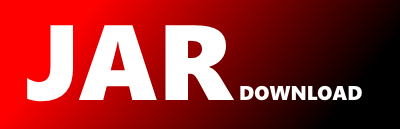
io.gitee.kingdonwang.tool.common.convertor.AbstractConvertor Maven / Gradle / Ivy
The newest version!
package io.gitee.kingdonwang.tool.common.convertor;
import java.util.List;
import java.util.stream.Collectors;
/**
* 转换器
*
* @author kingdonwang
* @since 2022-10-20 16:09:00
*/
public abstract class AbstractConvertor {
/**
* 转换
*
* @param source 源
* @return 目标
*/
public T convert(S source) {
if (null == source) {
return null;
}
return doConvert(source);
}
/**
* 逆向转换
*
* @param target 目标
* @return 源
*/
public S reverseConvert(T target) {
if (null == target) {
return null;
}
return doReverseConvert(target);
}
/**
* 转换数组
*
* @param sourceList 源数组
* @return 目标数组
*/
public List convertList(List sourceList) {
if (null == sourceList) {
return null;
}
return sourceList.stream()
.map(this::convert)
.collect(Collectors.toList());
}
/**
* 逆向转换数组
*
* @param targetList 目标数组
* @return 源数组
*/
public List reverseConvertList(List targetList) {
if (null == targetList) {
return null;
}
return targetList.stream()
.map(this::reverseConvert)
.collect(Collectors.toList());
}
/**
* 转换核心逻辑
*
* @param source 源
* @return 目标
*/
protected abstract T doConvert(S source);
/**
* 逆向转换核心逻辑
*
* @param target 目标
* @return 源
*/
protected abstract S doReverseConvert(T target);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy