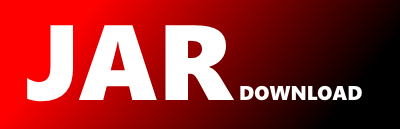
org.crazycake.shiro.serializer.ObjectSerializer Maven / Gradle / Ivy
package org.crazycake.shiro.serializer;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.io.Serializable;
import org.crazycake.shiro.exception.SerializationException;
public class ObjectSerializer implements RedisSerializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy