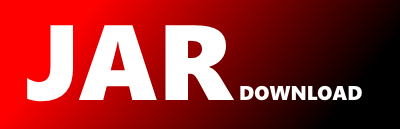
com.zznote.basecommon.common.handler.CreateAndUpdateMetaObjectHandler Maven / Gradle / Ivy
The newest version!
package com.zznote.basecommon.common.handler;
import cn.hutool.core.util.ObjectUtil;
import cn.hutool.http.HttpStatus;
import com.baomidou.mybatisplus.core.handlers.MetaObjectHandler;
import com.zznote.basecommon.common.exception.ServiceException;
import com.zznote.basecommon.common.util.LoginHelper;
import com.zznote.basecommon.dto.LoginUser;
import com.zznote.basecommon.entity.domain.BaseEntity;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.apache.ibatis.reflection.MetaObject;
import java.util.Date;
/**
* MP注入处理器
*
* @author Lion Li
* @date 2021/4/25
*/
@Slf4j
public class CreateAndUpdateMetaObjectHandler implements MetaObjectHandler {
@Override
public void insertFill(MetaObject metaObject) {
try {
if (ObjectUtil.isNotNull(metaObject) && metaObject.getOriginalObject() instanceof BaseEntity) {
BaseEntity baseEntity = (BaseEntity) metaObject.getOriginalObject();
Date current = ObjectUtil.isNotNull(baseEntity.getCreateTime())
? baseEntity.getCreateTime() : new Date();
baseEntity.setCreateTime(current);
baseEntity.setUpdateTime(current);
String username = StringUtils.isNotBlank(baseEntity.getCreateBy())
? baseEntity.getCreateBy() : getLoginUsername();
// 当前已登录 且 创建人为空 则填充
baseEntity.setCreateBy(username);
// 当前已登录 且 更新人为空 则填充
baseEntity.setUpdateBy(username);
}
} catch (Exception e) {
throw new ServiceException("自动注入异常 => " + e.getMessage(), HttpStatus.HTTP_UNAUTHORIZED);
}
}
@Override
public void updateFill(MetaObject metaObject) {
try {
if (ObjectUtil.isNotNull(metaObject) && metaObject.getOriginalObject() instanceof BaseEntity) {
BaseEntity baseEntity = (BaseEntity) metaObject.getOriginalObject();
Date current = new Date();
// 更新时间填充(不管为不为空)
baseEntity.setUpdateTime(current);
String username = getLoginUsername();
// 当前已登录 更新人填充(不管为不为空)
if (StringUtils.isNotBlank(username)) {
baseEntity.setUpdateBy(username);
}
}
} catch (Exception e) {
throw new ServiceException("自动注入异常 => " + e.getMessage(), HttpStatus.HTTP_UNAUTHORIZED);
}
}
/**
* 获取登录用户名
*/
private String getLoginUsername() {
LoginUser loginUser;
try {
loginUser = LoginHelper.getLoginUser();
} catch (Exception e) {
log.warn("自动注入警告 => 用户未登录");
return null;
}
return loginUser.getUsername();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy