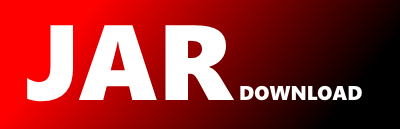
com.zznote.basecommon.service.impl.TPostServiceImpl Maven / Gradle / Ivy
The newest version!
package com.zznote.basecommon.service.impl;
import cn.hutool.core.util.ObjectUtil;
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.zznote.basecommon.common.constant.UserConstants;
import com.zznote.basecommon.common.exception.ServiceException;
import com.zznote.basecommon.common.page.PageInfo;
import com.zznote.basecommon.common.page.PageQuery;
import com.zznote.basecommon.dao.TPostDao;
import com.zznote.basecommon.dao.TUserPostDao;
import com.zznote.basecommon.entity.system.TPost;
import com.zznote.basecommon.entity.system.TUserPost;
import com.zznote.basecommon.service.TPostService;
import lombok.RequiredArgsConstructor;
import org.apache.commons.lang3.StringUtils;
import org.springframework.stereotype.Service;
import java.util.Arrays;
import java.util.List;
@Service("tPostService")
@RequiredArgsConstructor
public class TPostServiceImpl extends ServiceImpl implements TPostService {
private final TUserPostDao userPostMapper;
/**
* 根据用户ID获取岗位选择框列表
*
* @param userId 用户ID
* @return 选中岗位ID列表
*/
@Override
public List selectPostListByUserId(Long userId) {
return baseMapper.selectPostListByUserId(userId);
}
@Override
public PageInfo selectPagePostList(TPost post, PageQuery pageQuery) {
LambdaQueryWrapper lqw = new LambdaQueryWrapper()
.like(StringUtils.isNotBlank(post.getPostCode()), TPost::getPostCode, post.getPostCode())
.eq(StringUtils.isNotBlank(post.getStatus()), TPost::getStatus, post.getStatus())
.like(StringUtils.isNotBlank(post.getPostName()), TPost::getPostName, post.getPostName());
Page page = baseMapper.selectPage(pageQuery.build(), lqw);
return PageInfo.build(page);
}
/**
* 查询岗位信息集合
*
* @param post 岗位信息
* @return 岗位信息集合
*/
@Override
public List selectPostList(TPost post) {
return baseMapper.selectList(new LambdaQueryWrapper()
.like(StringUtils.isNotBlank(post.getPostCode()), TPost::getPostCode, post.getPostCode())
.eq(StringUtils.isNotBlank(post.getStatus()), TPost::getStatus, post.getStatus())
.like(StringUtils.isNotBlank(post.getPostName()), TPost::getPostName, post.getPostName()));
}
/**
* 校验岗位名称是否唯一
*
* @param post 岗位信息
* @return 结果
*/
@Override
public String checkPostNameUnique(TPost post) {
boolean exist = baseMapper.exists(new LambdaQueryWrapper()
.eq(TPost::getPostName, post.getPostName())
.ne(ObjectUtil.isNotNull(post.getPostId()), TPost::getPostId, post.getPostId()));
if (exist) {
return UserConstants.NOT_UNIQUE;
}
return UserConstants.UNIQUE;
}
/**
* 校验岗位编码是否唯一
*
* @param post 岗位信息
* @return 结果
*/
@Override
public String checkPostCodeUnique(TPost post) {
boolean exist = baseMapper.exists(new LambdaQueryWrapper()
.eq(TPost::getPostCode, post.getPostCode())
.ne(ObjectUtil.isNotNull(post.getPostId()), TPost::getPostId, post.getPostId()));
if (exist) {
return UserConstants.NOT_UNIQUE;
}
return UserConstants.UNIQUE;
}
/**
* 批量删除岗位信息
*
* @param postIds 需要删除的岗位ID
* @return 结果
*/
@Override
public int deletePostByIds(Long[] postIds) {
for (Long postId : postIds) {
TPost post = getById(postId);
if (countUserPostById(postId) > 0) {
throw new ServiceException(String.format("%1$s已分配,不能删除", post.getPostName()));
}
}
return baseMapper.deleteBatchIds(Arrays.asList(postIds));
}
/**
* 通过岗位ID查询岗位使用数量
*
* @param postId 岗位ID
* @return 结果
*/
// @Override
public long countUserPostById(Long postId) {
return userPostMapper.selectCount(new LambdaQueryWrapper().eq(TUserPost::getPostId, postId));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy