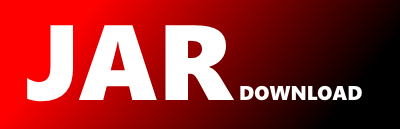
com.lx.boot.log.ESUtil Maven / Gradle / Ivy
package com.lx.boot.log;
import com.lx.boot.es.ElasticLowerClient;
import com.lx.boot.log.entity.EsInfo;
import com.lx.boot.log.entity.RunLogMessage;
import lombok.extern.slf4j.Slf4j;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
import java.util.function.Supplier;
@Slf4j
public class ESUtil {
private final ThreadPoolExecutor threadPoolExecutor = new ThreadPoolExecutor(4,8,10, TimeUnit.SECONDS, new ArrayBlockingQueue(5000), new ThreadPoolExecutor.DiscardOldestPolicy());
private final LinkedBlockingQueue QUEUES = new LinkedBlockingQueue<>();
private Supplier esInfoSupplier;
public ESUtil(Supplier esInfoSupplier){
this.esInfoSupplier = esInfoSupplier;
this.start();
}
//说明:添加新日志进入队列
/**{ ylx } 2020/6/24 10:24 */
public void offer(RunLogMessage msg){
threadPoolExecutor.execute(new Runnable() {
@Override
public void run() {
try {
if (QUEUES.size()>9000){
QUEUES.poll();
}
QUEUES.offer(msg);
}catch (Exception e){
}
}
});
}
private void start(){
threadPoolExecutor.execute(new Runnable() {
@Override
public void run() {
List ls = null;
while (true){
try {
ls = new ArrayList<>(500);
for (int j =0; j<500; j++){
RunLogMessage msg = QUEUES.poll(5,TimeUnit.SECONDS);
if (msg == null){
break;
}
ls.add(msg);
}
if (ls.size()>0){
EsInfo esInfo = esInfoSupplier.get();
if (esInfo != null){
ElasticLowerClient.getInstance(esInfo.getEsHosts(),esInfo.getUsername(),esInfo.getPassword()).insertList(ls,esInfo.getIndex());
}
}
}catch (Exception e){
log.info("上传日志失败!",e);
}
}
}
});
}
private static void sleep(long t){
try {
Thread.sleep(t);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy