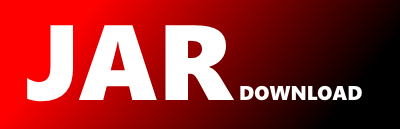
com.lx.boot.web.LXWebConfigurer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lxboot3 Show documentation
Show all versions of lxboot3 Show documentation
使用文档: https://a7fi97h1rc.feishu.cn/docx/X3LRdtLhkoXQ8hxgXDQc2CLOnEg?from=from_copylink
package com.lx.boot.web;//说明:
/**
* 创建人:游林夕/2019/5/8 17 10
*/
import com.lx.boot.OS;
import com.lx.constant.DefaultBaseConstant;
import com.lx.constant.DefaultStatusCode;
import com.lx.entity.Result;
import com.lx.util.LX;
import com.lx.util.exception.ResultServiceException;
import com.lx.util.exception.ServiceException;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import jakarta.validation.ConstraintViolationException;
import lombok.extern.slf4j.Slf4j;
import org.slf4j.MDC;
import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty;
import org.springframework.context.annotation.Configuration;
import org.springframework.validation.FieldError;
import org.springframework.validation.ObjectError;
import org.springframework.web.bind.MethodArgumentNotValidException;
import org.springframework.web.method.HandlerMethod;
import org.springframework.web.servlet.HandlerExceptionResolver;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.NoHandlerFoundException;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
import java.io.IOException;
import java.util.List;
import java.util.stream.Collectors;
@Slf4j
@Configuration
@ConditionalOnProperty(name = DefaultBaseConstant.WEB_CONFIGURER_ENABLE, havingValue = "true", matchIfMissing = true)
public class LXWebConfigurer implements WebMvcConfigurer {
//说明:判断是否是自定义业务类型异常
/**{ ylx } 2020/9/8 14:04 */
private Result serviceExceptionLog(String method, Throwable e){
do{
if (e instanceof ServiceException){
Result result;
if (e instanceof ResultServiceException){
result = Result.err(((ResultServiceException) e).getStatusCode() ,e.getMessage(),((ResultServiceException) e).getData());
}else{
result = Result.err(DefaultStatusCode.SERVICE_ERR,"网络异常,请稍后重试! "+ MDC.get("requestId"));
}
log.error("接口 ["+method+"] 业务层出错["+result.getCode()+"]=>",e);
return result;
}
}while ((e = e.getCause()) != null);
return null;
}
@Override
public void configureHandlerExceptionResolvers(List exceptionResolvers) {
exceptionResolvers.add(new HandlerExceptionResolver() {
@Override
public ModelAndView resolveException(HttpServletRequest request, HttpServletResponse response, Object handler, Exception e) {
setRequestId(request);
String method = OS.getApplicationName() + request.getRequestURI();
Result result = serviceExceptionLog(method, e);
if (result != null) {
//业务失败的异常,如“账号或密码错误”
} else if(e instanceof ConstraintViolationException){
result = Result.err(DefaultStatusCode.VIOLATION_ERR,"接口 ["+method+"] 参数验证出错:"+ e);
log.error(result.getMessage(),e);
}else if(e instanceof MethodArgumentNotValidException){
MethodArgumentNotValidException e1 = (MethodArgumentNotValidException) e;
String res = (String)e1.getBindingResult().getAllErrors().stream().map((cv) -> {
if (FieldError.class.isAssignableFrom(cv.getClass())){
FieldError err = (FieldError) cv;
return err == null ? "null" : err.getField() + ": " + cv.getDefaultMessage();
}else{
ObjectError err = cv;
return err == null ? "null" : cv.getDefaultMessage();
}
}).collect(Collectors.joining(", "));
result = Result.err(DefaultStatusCode.VIOLATION_ERR,"接口 ["+method+"] 参数验证出错:"+res);
log.error(result.getMessage(),e);
}else if(e instanceof org.springframework.web.bind.MissingServletRequestParameterException){
result = Result.err(DefaultStatusCode.MISS_ERR,"接口 ["+method+"] 缺少参数:"+ e.getMessage());
log.error(result.getMessage(),e);
}else if(e instanceof org.springframework.http.converter.HttpMessageNotReadableException){
result = Result.err(DefaultStatusCode.PARS_ERR,"接口 ["+method+"] 参数解析异常:"+ e.getMessage());
log.error(result.getMessage(),e);
}else if (e instanceof NoHandlerFoundException) {
result = Result.err(DefaultStatusCode.NO_HADNLER_ERR, "接口 [" + method + "] 不存在");
log.error(result.getMessage(),e);
}else if(e instanceof org.apache.ibatis.reflection.ReflectionException){
result = Result.err(DefaultStatusCode.NO_HADNLER_ERR, "接口 [" + method + "] 实体缺少字段!");
log.error(result.getMessage(),e);
}else if (!"dev".equals(OS.getEnv())){
result = Result.err("网络异常,请稍后重试! "+ MDC.get("requestId"));
log.error(result.getMessage(),e);
}else if(e instanceof org.springframework.dao.DataIntegrityViolationException){
result = Result.err(DefaultStatusCode.NO_HADNLER_ERR, "接口 [" + method + "] 违反约束!"+e.getCause().getMessage());
log.error(result.getMessage(),e);
}else {
String message;
if (handler instanceof HandlerMethod) {
HandlerMethod handlerMethod = (HandlerMethod) handler;
message = String.format("接口 [%s] 出现异常,方法:%s.%s,异常摘要:%s",
request.getRequestURI(),
handlerMethod.getBean().getClass().getName(),
handlerMethod.getMethod().getName(),
e.getMessage());
} else {
message = e.getMessage();
}
result = Result.err(message);
log.error(message, e);
}
responseResult(response, result);
return new ModelAndView();
}
});
}
//说明: 设置追踪码
/**{ ylx } 2021/2/3 15:37 */
private void setRequestId(HttpServletRequest request) {
try{
if(MDC.get("requestId") == null && request != null){
OS.setLogTraceId();
}
}catch (Exception e){
log.error("解析日志信息错误",e);
}
}
//说明:返回信息
/**{ ylx } 2022/3/10 11:13 */
private void responseResult(HttpServletResponse response, Result result) {
response.setCharacterEncoding("UTF-8");
response.setHeader("Content-type", "application/json;charset=UTF-8");
response.setStatus(200);
try {
response.getWriter().write(LX.toJSONString(result));
} catch (IOException ex) {
log.error(ex.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy