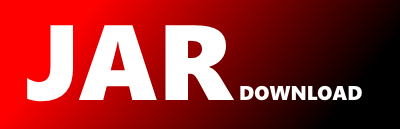
com.lx.util.LX Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lxboot3 Show documentation
Show all versions of lxboot3 Show documentation
使用文档: https://a7fi97h1rc.feishu.cn/docx/X3LRdtLhkoXQ8hxgXDQc2CLOnEg?from=from_copylink
package com.lx.util;//说明:
import com.lx.annotation.Note;
import com.lx.entity.Var;
import com.lx.entity.XmlNode;
import com.lx.util.exception.ResultServiceException;
import java.io.*;
import java.math.BigDecimal;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
import java.time.temporal.ChronoUnit;
import java.util.*;
import java.util.function.Function;
import java.util.function.Supplier;
/**
* 创建人:游林夕/2019/3/27 14 53
*/
public final class LX {
public static final String UTF_8 = "UTF-8";
public enum RequestMethod{POST,GET,PUT,DELETE}
public final static String LOCALHOST_IP = OtherUtil.getIp();
private LX(){}
@Note("Map List Set Object[] size=0 或者 字符串==空串 或者 为 null")
public static boolean isEmpty(Object obj){return OtherUtil.isEmpty(obj);}
public static boolean isNotEmpty(Object s){return !isEmpty(s);}
@Note("字符串是空白")
public static boolean isEmpty(String s){return OtherUtil.isBlank(s);}
@Note("字符串不为空白")
public static boolean isNotEmpty(String s){return !OtherUtil.isBlank(s);}
@Note("抛异常")
public static T exMsg(String msg){throw new ResultServiceException(msg);}
public static T exMsg(Throwable e){throw new RuntimeException(e);}
@Note("抛特殊异常-ResultServiceException")
public static T exMsg(String msg, int errCode){throw new ResultServiceException(msg,errCode);}
@Note("boo为true时抛异常")
public static T exMsg(boolean boo,String msg){return OtherUtil.exMsg(boo,msg);}
@Note("boo为true抛特殊异常-ResultServiceException")
public static T exMsg(boolean boo,String msg, int errCode){return OtherUtil.exMsg(boo,msg,errCode);}
@Note("判断对象为空时抛异常, 报错固定:值不能为空")
public static void exObj(Object s){exObj(s,"值不能为空!");}
@Note("判断对象为空时抛异常")
public static void exObj(Object s,String exMsg){if(isEmpty(s)){exMsg(exMsg);}}
@Note("获取随机UUID, i指定长度1-36位")
public static String uuid(){return UUID.randomUUID().toString();}
public static String uuid(int i){return uuid().substring(0, i<1||i>36 ? 36 : i);}
@Note("获取随机UUID 不带-, i指定长度1-32位")
public static String uuid32(){return UUID.randomUUID().toString().replace("-","");}
public static String uuid32(int i){return uuid32().substring(0, i<1||i>32 ? 32 : i);}
@Note("获取雪花id")
public static long snowid(){return OtherUtil.snowid();}
@Note("将对象转为Map")
public static T toMap(Class t,Object obj) {return CollectionUtil.toMap(t,obj);}
public static Var toMap(Object obj) {return CollectionUtil.toMap(Var.class,obj);}
@Note("将对象转为其他对象")
public static T toObj(Class t,Object obj){return CollectionUtil.toObj(t,obj);}
@Note("将对象转为List")
public static List toList(Object obj) { return CollectionUtil.toList(obj);}
public static List toList(Class t,Object obj){return CollectionUtil.toList(t, obj);}
@Note("将对象转为json串")
public static String toJSONString(Object obj) { return CollectionUtil.toJSONString(obj);}
public static String toFormatJson(Object obj){return CollectionUtil.toFormatJson(obj);}
@Note("调用Http请求, timeoutMillisecond:超时时间(毫秒), exMsgByFailure: 报错是否抛异常,charset: 字符集")
public static String doPost(String url,String body){return LXHttp.doPost(url,body,null);}
public static String doPost(String url,String body,Map headers){return LXHttp.doPost(url,body,headers);}
public static String doPost(String url,String body,Map headers, int timeoutMillisecond, boolean exMsgByFailure,Charset charset){return LXHttp.doPost(url,body,headers,timeoutMillisecond,exMsgByFailure,charset);}
public static String doGet(String url) {return LXHttp.doGet(url,null);}
public static String doGet(String url,Map headers) {return LXHttp.doGet(url,headers);}
public static String doGet(String url,Map headers, int timeoutMillisecond, boolean exMsgByFailure,Charset charset) {return LXHttp.doGet(url,headers,timeoutMillisecond,exMsgByFailure,charset);}
public static String doPut(String url, String body, Map headers, int millisecond, boolean exMsgByFailure) {return LXHttp.doPut(url, body, headers,millisecond, exMsgByFailure);}
public static String doDelete(String url,Map headers, int timeoutMillisecond, boolean exMsgByFailure){return LXHttp.doDelete(url, headers,timeoutMillisecond, exMsgByFailure);}
public static InputStream doGetStream(String url, Map headers, int timeoutMillisecond, boolean exMsgByFailure,Charset charset) {return LXHttp.doGetStream(url,headers,timeoutMillisecond,exMsgByFailure,charset);}
public static InputStream doPostInputStream(String url, String body, Map headers, int timeoutMillisecond,boolean exMsgByFailure){return LXHttp.doPostInputStream(url,body,headers,timeoutMillisecond, exMsgByFailure);}
public static InputStream httpInputStream(String url, RequestMethod method, InputStream body, Map headers, int timeoutMillisecond, boolean exMsgByFailure) {return LXHttp.httpInputStream(url,method,body,headers,timeoutMillisecond,exMsgByFailure);}
@Note("调用webservice接口 一般为C#form表单提交形式 asmxUrl:以asmx结尾拼接/方法名 例:http://ip:prot/xx.asmx/methodName ;params:表单参数")
public static String webservice(String asmxUrl ,Map params){return OtherUtil.webservice(asmxUrl,params);}
@Note("调用webservice接口 一般为java SOAP接口XML, wsdlUrl:wsdl地址, method:方法名, targetNamespace:命名空间 params:参数")
public static String webservice(String wsdlUrl ,String method ,String targetNamespace,Map params){return OtherUtil.webservice(wsdlUrl, method, targetNamespace , params);}
@Note("对字符串进行base64编解码")
public static String encode(String str){return SignUtil.base64Encode(str.getBytes(StandardCharsets.UTF_8));}
public static String decode(String str){return new String(SignUtil.base64Decode(str),StandardCharsets.UTF_8);}
@Note("对byte[]进行base64编解码")
public static String base64Encode(byte[] data){return SignUtil.base64Encode(data);}
public static byte[] base64EncodeToByteArray(byte[] data){return SignUtil.base64EncodeToByteArray(data);}
public static byte[] base64Decode(byte[] data){return SignUtil.base64Decode(data);}
public static byte[] base64Decode(String str){return SignUtil.base64Decode(str);}
@Note("对字符串进行md5")
public static String md5(String string){return SignUtil.md5(string);}
public static String md5(String string, Charset charset){return SignUtil.md5(string,charset);}
@Note("对byte[]进行md5")
public static String md5(byte[] data){return SignUtil.md5(data);}
@Note("拼接签名字符串,params:待拼接数据 排除字段为sign,拼接符为&,值不进行urlEncode, 参数为空时跳过拼接")
public static String createLinkString(final Map params) {return SignUtil.createLinkString(params,"sign" ,"&", false, true);}
@Note("拼接签名字符串,params:待拼接数据, exclude:排除字段,connStr:拼接符为&, encode:值进行urlEncode, skipEmpty:参数为空时跳过拼接")
public static String createLinkString(final Map params,String exclude, String connStr, boolean encode, boolean skipEmpty) {return SignUtil.createLinkString(params,exclude, connStr, encode , skipEmpty);}
@Note("RSA私钥签名,参数使用LX.createLinkString进行拼接")
public static String rsaSign(final Map params, String privateStr){return SignUtil.sign(params,privateStr);}
@Note("RSA私钥签名")
public static String rsaEncrypt(String data, String privateStr) {return SignUtil.encryptByPrivateKey(data, privateStr);}
@Note("RSA公钥验证签名,参数使用LX.createLinkString进行拼接")
public static boolean rsaVerify(Map params,String sign, String publicKey){return SignUtil.checkByPublicKey(createLinkString(params), sign, publicKey);}
public static boolean rsaVerify(String data,String sign, String publicKey){return SignUtil.checkByPublicKey(data, sign, publicKey);}
@Note("DES 加解密 C# 与java 加密 将byte[]转16进制字符串 参考:https://blog.csdn.net/weixin_43996276/article/details/121085081")
public static String desEncrypt(String data, String key) {return SignUtil.desEncrypt(data,key);}
public static String desDecrypt(String data,String key) {return SignUtil.desDecrypt(data,key);}
public static byte[] desEncrypt(byte[] data, String key, String ivParameter, String fillingAlgorithm, Charset charset) {return SignUtil.desEncrypt(data,key,ivParameter,fillingAlgorithm,charset);}
public static byte[] desDecrypt(byte[] data, String key, String ivParameter, String fillingAlgorithm, Charset charset) {return SignUtil.desDecrypt(data,key,ivParameter,fillingAlgorithm,charset);}
@Note("hmacSHA")
public enum HmacSha {
HmacSHA1,HmacSHA256,HmacSHA512;
@Note("key 密钥 content 待签名加密的数据")
public byte[] encode(byte[] key,byte[]...content){return SignUtil.hmacSHA(name(),key,content);}
public String encode(String key,String content){return toHexString(SignUtil.hmacSHA(name(),key.getBytes(StandardCharsets.UTF_8),content.getBytes(StandardCharsets.UTF_8)));}
}
@Note("byte[]转16进制字符串")
public static String toHexString(byte[] data) {return SignUtil.toHexString(data);}
@Note("16进制字符串转byte[]")
public static byte[] convertHexString(String data) {return SignUtil.convertHexString(data);}
@Note("对字符串进行urlEncode编码")
public static String urlEncode(String src) {return SignUtil.urlEncode(src);}
@Note("对字符串进行urlDecode编码")
public static String urlDecode(String src) {return SignUtil.urlDecode(src);}
@Note("转xml")
public static String toXml(Map params, String label, boolean skipEmpty) {return XmlHelper.toXml(null, params,label , skipEmpty);}
public static String toXml(Collection params, String label, String childLabel, boolean skipEmpty) {return XmlHelper.toXml(null, params,label,childLabel,skipEmpty);}
@Note("xml转map")
public static Map xmlToStringMap(String xml){return XmlHelper.xmlToStringMap(xml);}
public static Map xmlToMap(String xmlStr) {return XmlHelper.xmlToMap(xmlStr);}
public static List xmlToList(String xml){return XmlHelper.xmlToList(xml);}
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy