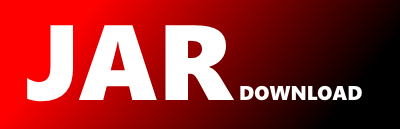
com.lx.util.OtherUtil Maven / Gradle / Ivy
package com.lx.util;
import com.lx.entity.Var;
import com.lx.util.exception.ResultServiceException;
import java.io.*;
import java.net.Inet4Address;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.net.URL;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.util.*;
class OtherUtil {
//说明: 字符串是否为空白
/** @author ylx 2022/12/21 15:30 */
public static boolean isBlank(String s){
return s == null || "".equals(s);
}
/**为空则返回true,不否则返回false*/
public static boolean isEmpty(Object s){
if (s == null){
return true;
}else if (s instanceof Map){
return ((Map)s).size()==0;
}else if(s instanceof Collection){
return ((Collection)s).size()==0;
}else if(s instanceof Object []){
return ((Object[])s).length==0;
}else if(s instanceof int []){
return ((int[])s).length==0;
}else if(s instanceof long []){
return ((long[])s).length==0;
}
return "".equals(s);
}
/**对象不为null*/
public static boolean isNotEmpty(Object s){return !isEmpty(s);}
/** map中是否包含所有指定的值 */
public static boolean isNotEmptyMap(Map map,String keys){
if (isNotEmpty(map) && isNotEmpty(keys)){
for (String object : keys.split(",")) {
if(isEmpty(map.get(object.trim()))){
return false;
}
}
return true;
}
return false;
}
/**抛异常*/
public static T exMsg(boolean boo,String msg){
if (boo){
throw new ResultServiceException(msg);
}
return null;
}
public static T exMsg(boolean boo,String msg, int errCode){
if (boo){
throw new ResultServiceException(msg, errCode);
}
return null;
}
/**判断对象为空时抛异常*/
public static void exObj(Object s){exObj(s,"值不能为空!");}
/** 判断对象为空时抛异常*/
public static void exObj(Object s,String exMsg){
if(isEmpty(s)){
LX.exMsg(exMsg);
}
}
/**判断map中key对应的value值是否为空*/
public static void exMap(Map s , String keys){
exObj(s);
exObj(keys);
StringBuilder sb = new StringBuilder();
for (String object : keys.split(",")) {
if(isEmpty(s.get(object.trim()))){
sb.append("没有获取到:"+object);
}
}
if (sb.length()>0){
LX.exMsg(sb.toString());
}
}
/** 判断实体里的字段是否为空 */
public static void exEntity(Object obj,String keys){
exObj(obj,"实体不能为空");
exMap(LX.toMap(obj),keys);
}
/** 上次生成ID的时间截 */
private static long LAST_TIME_STAMP = -1L;
/** 上一次的毫秒内序列值 */
private static long LAST_SEQ = 0L;
private static Random random = new Random();
public synchronized static long snowid(){
long now = System.currentTimeMillis();
LX.exMsg(now < LAST_TIME_STAMP, "系统时间错误! 拒绝生成雪花ID");
if (now == LAST_TIME_STAMP) {
LAST_SEQ = (LAST_SEQ + 1) & 4194303;
if (LAST_SEQ == 0){
while (now <= LAST_TIME_STAMP) {
now = System.currentTimeMillis();
}
}
} else {
LAST_SEQ = random.nextInt(2000000);
}
//上次生成ID的时间截
LAST_TIME_STAMP = now;
return (now*1000000L)+(LAST_SEQ % 1000000L);
}
/**左对齐*/
public static String left(String str,int i,char c){
if(str.length()>=i){
return str.substring(0,i);
}
StringBuilder sb = new StringBuilder(str);
for (int j=0;j=i){
return str.substring(str.length()-i);
}
StringBuilder sb = new StringBuilder();
for (int j=0;j loadProperties(String fileName){
ResourceBundle prb = ResourceBundle.getBundle(fileName);
Map pp = new HashMap<>();
for (String key : prb.keySet()){
pp.put(key , prb.getString(key));
}
return pp;
}
/**获取网络上的properties文件*/
public static Map getNetProperties(String urlPath){
Properties pro = new Properties();
try {
pro.load(new URL(urlPath).openConnection().getInputStream());
} catch (Exception e) {
LX.exMsg(e);
}
return LX.toMap(pro);
}
/*睡眠*/
public static void sleep(long time){
try {
Thread.sleep(time);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
LX.exMsg(e.getMessage());
}
}
private final static String LOCAL_IP = "127.0.0.1";
public static String getIp(){
String localHostAddress = LOCAL_IP;
try {
Enumeration allNetInterfaces = NetworkInterface.getNetworkInterfaces();
while(allNetInterfaces.hasMoreElements()){
NetworkInterface networkInterface = allNetInterfaces.nextElement();
Enumeration address = networkInterface.getInetAddresses();
while(address.hasMoreElements()){
InetAddress inetAddress = address.nextElement();
if(inetAddress != null
&& inetAddress instanceof Inet4Address
&& !LOCAL_IP.equals(inetAddress.getHostAddress())){
localHostAddress = inetAddress.getHostAddress();
}
}
}
}catch (Exception e){
}
return localHostAddress;
}
//说明: InputStream -> byte[]
/**{ ylx } 2021/6/16 21:30 */
public static byte[] toByteArray(InputStream input){
try {
try(ByteArrayOutputStream output = new ByteArrayOutputStream()){
byte[] buffer = new byte[4096];
int n = -1;
while (-1 != (n = input.read(buffer))) {
output.write(buffer, 0, n);
}
return output.toByteArray();
}
} catch (IOException e) {
return LX.exMsg(e.getMessage());
}
}
//说明:inputStream转String
/**{ ylx } 2022/4/2 23:47 */
public static String streamToString(InputStream inputStream , Charset charset){
if (inputStream == null){
return null;
}
if (charset == null){
charset = StandardCharsets.UTF_8;
}
try(BufferedReader br = new BufferedReader(new InputStreamReader(inputStream, charset))) {
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line);
}
return sb.toString();
}catch (Exception e){
LX.exMsg(e);
}
return "";
}
public static void inputStreamToOutputStream(InputStream inputStream , OutputStream outputStream ){
try {
byte[] buffer = new byte[4096];
int n = -1;
while (-1 != (n = inputStream.read(buffer))) {
outputStream.write(buffer, 0, n);
}
outputStream.flush();
} catch (IOException e) {
LX.exMsg(e.getMessage());
}
}
public static String webservice(String asmxUrl, Map params) {
String form = LX.createLinkString(params);
return LX.doPost(asmxUrl,form, new Var("Content-Type", "application/x-www-form-urlencoded"));
}
public static String webservice(String wsdlUrl,String method, String nameSpace ,Map params){
StringBuilder sb = new StringBuilder();
sb.append("");
params.forEach((k,v)->{
sb.append("<").append(k).append(">").append(v).append("").append(k).append(">");
});
sb.append(" ");
System.out.println(sb);
return LX.doPost(wsdlUrl,sb.toString(),new Var("Content-Type","Content-Type: text/xml; charset=utf-8"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy