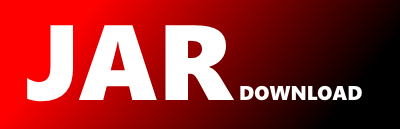
com.lx.util.XmlHelper Maven / Gradle / Ivy
package com.lx.util;
import com.lx.entity.XmlNode;
import org.w3c.dom.Document;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.xpath.XPathFactory;
import java.io.IOException;
import java.io.StringReader;
import java.util.Collection;
import java.util.List;
import java.util.Map;
/**
* xpath解析xml
*
*
* 文档地址:
* http://www.w3school.com.cn/xpath/index.asp
*
* @author L.cm
*/
class XmlHelper {
public static Map xmlToStringMap(String xml){
return new XmlNode(create(xml).getDocumentElement()).getStringMap();
}
public static Map xmlToMap(String xml){
return new XmlNode(create(xml).getDocumentElement()).getMap();
}
public static List xmlToList(String xml){
return new XmlNode(create(xml).getDocumentElement()).getList();
}
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy