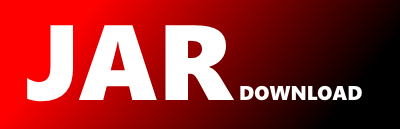
commonMain.com.g985892345.provider.manager.KtProviderManager.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of provider-manager-jvm Show documentation
Show all versions of provider-manager-jvm Show documentation
the service provider manager of KtProvider
package com.g985892345.provider.manager
import com.g985892345.provider.api.init.IKtProviderDelegate
import com.g985892345.provider.api.init.wrapper.ImplProviderWrapper
import com.g985892345.provider.api.init.wrapper.KClassProviderWrapper
import kotlin.reflect.KClass
/**
* Service provider manager
*
* @author 985892345
* 2023/6/13 22:05
*/
object KtProviderManager {
/**
* Return the implementation class that is only set with the corresponding [name].
* @throws IllegalArgumentException when [name] is an empty string.
*/
fun getImplOrNull(name: String): T? = getImplOrNull(null, name)
/**
* Return the implementation class that is only set with the corresponding [name].
* @throws NullPointerException when it does not exist.
* @throws IllegalArgumentException when [name] is an empty string.
*/
fun getImplOrThrow(name: String): T = getImplOrNull(name)!!
/**
* Return the implementation class that is only set with the corresponding [clazz].
*/
fun getImplOrNull(clazz: KClass): T? = getImplOrNull(clazz, "")
/**
* Return the implementation class that is only set with the corresponding [clazz].
* @throws NullPointerException when it does not exist.
*/
fun getImplOrThrow(clazz: KClass): T = getImplOrNull(clazz)!!
/**
* Return the implementation class that is set with the corresponding [clazz] and [name].
* @throws IllegalArgumentException when [clazz] is null and [name] is an empty string.
*/
fun getImplOrNull(clazz: KClass?, name: String): T? = getImplOrNullInternal(clazz, name)
/**
* Return the implementation class that is set with the corresponding [clazz] and [name].
* @throws NullPointerException when it does not exist.
* @throws IllegalArgumentException when [clazz] is null and [name] is an empty string.
*/
fun getImplOrThrow(clazz: KClass?, name: String): T = getImplOrNull(clazz, name)!!
/**
* Retrieve all implementation classes from @ImplProvider annotations where the clazz parameter is [clazz].
*/
@Suppress("UNCHECKED_CAST")
fun getAllImpl(clazz: KClass?): Map> {
return IKtProviderDelegate.ImplProviderMap[clazz ?: Nothing::class]
?.mapValues { it.value as ImplProviderWrapper }
?: emptyMap()
}
/**
* Return the KClass of the implementation class that is only set with the corresponding [name].
* @throws IllegalArgumentException when [name] is an empty string.
*/
fun getKClassOrNull(name: String): KClass? = getKClassOrNull(null, name)
/**
* Return the KClass of the implementation class that is only set with the corresponding [name].
* @throws NullPointerException when it does not exist.
* @throws IllegalArgumentException when [name] is an empty string.
*/
fun getKClassOrThrow(name: String): KClass = getKClassOrNull(name)!!
/**
* Return the KClass of the implementation class that is only set with the corresponding [clazz].
*/
fun getKClassOrNull(clazz: KClass): KClass? = getKClassOrNull(clazz, "")
/**
* Return the KClass of the implementation class that is only set with the corresponding [clazz].
* @throws NullPointerException when it does not exist.
*/
fun getKClassOrThrow(clazz: KClass): KClass? = getKClassOrNull(clazz)!!
/**
* 返回 [clazz] 和 [name] 对应的 KClass
* @throws IllegalArgumentException class 为 null 并且 name 为空串时抛出非法参数错误
*/
fun getKClassOrNull(clazz: KClass?, name: String): KClass? = getKClassOrNullInternal(clazz, name)
/**
* Return the KClass of the implementation class that is set with the corresponding [clazz] and [name].
* @throws NullPointerException when it does not exist.
*/
fun getKClassOrThrow(clazz: KClass?, name: String): KClass = getKClassOrNull(clazz, name)!!
/**
* Retrieve all implementation classes from @KClassProvider annotations where the clazz parameter is [clazz].
*/
@Suppress("UNCHECKED_CAST")
fun getAllKClass(clazz: KClass?): Map> {
return IKtProviderDelegate.KClassProviderMap[clazz ?: Nothing::class]
?.mapValues { it.value as KClassProviderWrapper }
?: emptyMap()
}
@Suppress("UNCHECKED_CAST")
private fun getImplOrNullInternal(
clazz: KClass<*>?,
name: String,
): T? {
if (clazz == null && name.isEmpty()) {
throw IllegalArgumentException("Either [clazz] or [name] must be included!")
}
return IKtProviderDelegate.ImplProviderMap[clazz]?.get(name)?.get() as T?
}
@Suppress("UNCHECKED_CAST")
private fun getKClassOrNullInternal(
clazz: KClass<*>?,
name: String,
): KClass? {
if (clazz == null && name.isEmpty()) {
throw IllegalArgumentException("Either [clazz] or [name] must be included!")
}
return IKtProviderDelegate.KClassProviderMap[clazz]?.get(name)?.get() as KClass?
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy