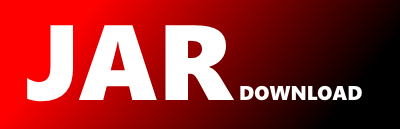
anlavn.ui.Mode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AL-Library_EN Show documentation
Show all versions of AL-Library_EN Show documentation
Java library for many thing wonderful
The newest version!
package anlavn.ui;
// Make By Bình An || AnLaVN || KatoVN
import java.awt.*;
import javax.swing.*;
import static javax.swing.JOptionPane.*;
/**The Mode class supports change mode from light to dark, component from nimbus to windows look and feel.
* @author AnLaVN - https://github.com/AnLaVN
*/
public class Mode {
private static boolean MODE = false;
private static final LookAndFeel PRE_LF = UIManager.getLookAndFeel();
private static final String SYS_LF = UIManager.getSystemLookAndFeelClassName();
private static Color TextDarkMode = Color.decode("#F0F0F0"), TextLightMode = Color.decode("#2C3338");
private static Color BackDarkMode = Color.decode("#363B41"), BackLightMode = Color.decode("#FFFFFF");
/**Use this method to get current Mode.
* @return true
if Dark Mode, false
if Light Mode.
*/
public static boolean getMode() { return MODE; }
/**Use this method to set Mode for UI.
* @param MODE true
is Dark Mode, false
is Light Mode.
*/
public static void setMode(boolean MODE) {
Mode.MODE = MODE;
}
/**Use this method to get Text color at current Mode.
* @return Text color at current Mode.
*/
public static Color getTextColor() { return MODE ? TextDarkMode : TextLightMode; }
/**Use this method to get text color at light Mode.
* @return Text Color at light Mode.
*/
public static Color getTextLightColor() { return TextLightMode; }
/**Use this method to get text color at dark Mode.
* @return Text Color at dark Mode.
*/
public static Color getTextDarkColor() { return TextDarkMode; }
/**Use this method to get background color at current Mode.
* @return Background color at current Mode.
*/
public static Color getBackColor() { return MODE ? BackDarkMode : BackLightMode; }
/**Use this method to get background color at light Mode.
* @return Background Color at light Mode.
*/
public static Color getBackLightColor() { return BackLightMode; }
/**Use this method to get background color at dark Mode.
* @return Background Color at dark Mode.
*/
public static Color getBackDarkColor() { return BackDarkMode; }
/**Use this method to custom Color of Text in 2 case Dark and Light.
* @param TextDarkMode is Color of Text if The Mode is Dark, should be light color.
* @param TextLightMode is Color of Text if The Mode is Light, should be dark color.
*/
public static void setTextColor(Color TextDarkMode, Color TextLightMode){
Mode.TextDarkMode = TextDarkMode;
Mode.TextLightMode = TextLightMode;
}
/**Use this method to custom Color of Background in 2 case Dark and Light.
* @param BackDarkMode is Color of Background if The Mode is Dark, should be dark color.
* @param BackLightMode is Color of Background if The Mode is Light, should be light color.
*/
public static void setBackColor(Color BackDarkMode, Color BackLightMode){
Mode.BackDarkMode = BackDarkMode;
Mode.BackLightMode = BackLightMode;
}
/**Use this method to change Mode for Components.
* @param components is list of variable Component need change Mode.
*/
public static void setModeComponent(Component... components){
for(Component component : components) setModeComponent(component);
}
/**Use this method to change Mode for a Component.
* @param component is a variable Component need change Mode.
*/
public static void setModeComponent(Component component){
JComponent j = null;
if(component instanceof JPanel pan) {j = pan;}
if(component instanceof JLabel lbl) {j = lbl;}
if(component instanceof JRadioButton rdo) {j = rdo;}
if(component instanceof JCheckBox chk) {j = chk;}
if(component instanceof JTextField txt) {j = txt;}
if(component instanceof JTextPane tpa) {j = tpa;}
if(component instanceof JTextArea tar) {j = tar;}
if(component instanceof JTable tbl) {j = tbl;}
if(component instanceof JButton btn) {j = btn;}
if(component instanceof JScrollBar scb) {j = scb;}
if(j != null){
if(!(j instanceof JScrollBar)){
j.setForeground ( MODE ? TextDarkMode : TextLightMode );}
j.setBackground ( MODE ? BackDarkMode : BackLightMode );
}
}
/**Use this method to change File Chooser into Windows Look and Feel.
* @return a JFileChooser
was change Look and Feel.
*/
public static JFileChooser WFileChooser(){
JFileChooser chooser = null;
try {
UIManager.setLookAndFeel(SYS_LF);
chooser = new JFileChooser();
UIManager.setLookAndFeel(PRE_LF);
} catch (IllegalAccessException | UnsupportedLookAndFeelException | InstantiationException | ClassNotFoundException e) {
System.out.println("\n!!! Error trying to change Look and Feel of File Chooser. !!!\n\tError code: " + e.toString());}
return chooser;
}
/**Use this method to change Check Box into Windows Look and Feel.
* @return a JCheckBox
was change Look and Feel.
*/
public static JCheckBox WCheckBox(){
JCheckBox checkbox = null;
try {
UIManager.setLookAndFeel(SYS_LF);
checkbox = new JCheckBox();
UIManager.setLookAndFeel(PRE_LF);}
catch (IllegalAccessException | UnsupportedLookAndFeelException | InstantiationException | ClassNotFoundException e) {
System.out.println("\n!!! Error trying to change Look and Feel of Check Box. !!!\n\tError code: " + e.toString());}
return checkbox;
}
/**Use this method to change Combo Box into Windows Look and Feel.
* @return a JComboBox
was change Look and Feel.
*/
public static JComboBox WComboBox(){
JComboBox combobox = null;
try {
UIManager.setLookAndFeel(SYS_LF);
combobox = new JComboBox();
UIManager.setLookAndFeel(PRE_LF);}
catch (IllegalAccessException | UnsupportedLookAndFeelException | InstantiationException | ClassNotFoundException e) {
System.out.println("\n!!! Error trying to change Look and Feel of Combo Box. !!!\n\tError code: " + e.toString());}
return combobox;
}
/**Use this method to brings up a dialog displaying a message.
* @param parentComponent the Frame
in which the dialog is displayed, if null, or has no Frame
, a default Frame
is used.
* @param message the Object
to display.
*/
public static void WMessage(Component parentComponent, Object message){
WMessage(parentComponent, message, "Message Dialog", INFORMATION_MESSAGE);
}
/**Use this method to brings up a dialog displaying a message, with all parameters.
* @param parentComponent the Frame
in which the dialog is displayed, if null, or has no Frame
, a default Frame
is used.
* @param message the Object
to display.
* @param title the title String
for the dialog.
* @param messageType the type of message to be displayed:
* ERROR_MESSAGE
, INFORMATION_MESSAGE
, WARNING_MESSAGE
, QUESTION_MESSAGE
, or PLAIN_MESSAGE
.
*/
public static void WMessage(Component parentComponent, Object message, String title, int messageType){
WMessage(parentComponent, message, title, messageType, null);
}
/**Use this method to brings up a dialog displaying a message, with all parameters.
* @param parentComponent the Frame
in which the dialog is displayed, if null, or has no Frame
, a default Frame
is used.
* @param message the Object
to display.
* @param title the title String
for the dialog.
* @param messageType the type of message to be displayed:
* ERROR_MESSAGE
, INFORMATION_MESSAGE
, WARNING_MESSAGE
, QUESTION_MESSAGE
, or PLAIN_MESSAGE
.
* @param icon an icon to display in the dialog that helps the user identify the kind of message that is being displayed.
*/
public static void WMessage(Component parentComponent, Object message, String title, int messageType, Icon icon){
try {
UIManager.setLookAndFeel(SYS_LF);
JOptionPane.showMessageDialog(parentComponent, message, title, messageType, icon);
UIManager.setLookAndFeel(PRE_LF);
} catch (IllegalAccessException | UnsupportedLookAndFeelException | InstantiationException | ClassNotFoundException e) {
System.out.println("\n!!! Error trying to change Look and Feel of MessageDialog. !!!\n\tError code: " + e.toString());}
}
/**Use this method to brings up a Confirm dialog with the options "Yes, No and Cancel".
* @param parentComponent the Frame
in which the dialog is displayed.
* @param message the Object
to display.
* @return an integer indicating the option selected by the user.
*/
public static int WConfirm(Component parentComponent, Object message){
return WConfirm(parentComponent, message, "Confirm Dialog", YES_NO_CANCEL_OPTION);
}
/**Use this method to brings up a Confirm dialog, where the number of choices is determined by the optionType parameter.
* @param parentComponent the Frame
in which the dialog is displayed.
* @param message the Object
to display.
* @param title The title String
for the dialog.
* @param optionType an int
designating the options available on the dialog:
* YES_NO_OPTION
, YES_NO_CANCEL_OPTION
, or OK_CANCEL_OPTION
.
* @return an integer indicating the option selected by the user.
*/
public static int WConfirm(Component parentComponent, Object message, String title, int optionType){
return WConfirm(parentComponent, message, title, optionType, QUESTION_MESSAGE);
}
/**Use this method to brings up a Confirm dialog, with all parameters and default icon.
* @param parentComponent the Frame
in which the dialog is displayed.
* @param message the Object
to display.
* @param title The title String for the dialog.
* @param optionType an int
designating the options available on the dialog:
* YES_NO_OPTION
, YES_NO_CANCEL_OPTION
, or OK_CANCEL_OPTION
.
* @param messageType the type of message to be displayed:
* ERROR_MESSAGE
, INFORMATION_MESSAGE
, WARNING_MESSAGE
, QUESTION_MESSAGE
, or PLAIN_MESSAGE
.
* @return an integer indicating the option selected by the user.
*/
public static int WConfirm(Component parentComponent, Object message, String title, int optionType, int messageType){
return WConfirm(parentComponent, message, title, optionType, messageType, null);
}
/**Use this method to brings up a Confirm dialog, with all parameters.
* @param parentComponent the Frame
in which the dialog is displayed.
* @param message the Object
to display.
* @param title The title String for the dialog.
* @param optionType an int
designating the options available on the dialog:
* YES_NO_OPTION
, YES_NO_CANCEL_OPTION
, or OK_CANCEL_OPTION
.
* @param messageType the type of message to be displayed:
* ERROR_MESSAGE
, INFORMATION_MESSAGE
, WARNING_MESSAGE
, QUESTION_MESSAGE
, or PLAIN_MESSAGE
.
* @param icon the icon to display in the dialog.
* @return an integer indicating the option selected by the user.
*/
public static int WConfirm(Component parentComponent, Object message, String title, int optionType, int messageType, Icon icon) {
try {
UIManager.setLookAndFeel(SYS_LF);
int ConfirmDialog = JOptionPane.showConfirmDialog(parentComponent, message, title, optionType, messageType, icon);
UIManager.setLookAndFeel(PRE_LF);
return ConfirmDialog;
} catch (IllegalAccessException | UnsupportedLookAndFeelException | InstantiationException | ClassNotFoundException e) {
System.out.println("\n!!! Error trying to change Look and Feel of ConfirmDialog. !!!\n\tError code: " + e.toString());
throw new RuntimeException(e);}
}
/**Use this method to brings up a Input dialog, shows a question message
.
* @param message the Object
to display.
* @return a user's input.
*/
public static String WInput(Object message){
return WInput(null, message);
}
/**Use this method to brings up a Input dialog, displayed on top of the Component
.
* @param parentComponent the parent Component
for the dialog.
* @param message the Object
to display.
* @return a user's input.
*/
public static String WInput(Component parentComponent, Object message){
return WInput(parentComponent, message,"Input Dialog" , QUESTION_MESSAGE);
}
/**Use this method to brings up a Input dialog, have tittle
and messageType
.
* @param parentComponent Component
chứa hộp thoại.
* @param message the Object
to display.
* @param title the String
to display in the dialog title bar.
* @param messageType the type of message that is to be displayed:
* ERROR_MESSAGE
, INFORMATION_MESSAGE
, WARNING_MESSAGE
, QUESTION_MESSAGE
, or PLAIN_MESSAGE
.
* @return a user's input.
*/
public static String WInput(Component parentComponent, Object message, String title, int messageType){
try {
UIManager.setLookAndFeel(SYS_LF);
String InputDialog = JOptionPane.showInputDialog(parentComponent, message, title, messageType);
UIManager.setLookAndFeel(PRE_LF);
return InputDialog;
} catch (IllegalAccessException | UnsupportedLookAndFeelException | InstantiationException | ClassNotFoundException e) {
System.out.println("\n!!! Error trying to change Look and Feel of InputDialog. !!!\n\tError code: " + e.toString());
throw new RuntimeException(e);}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy