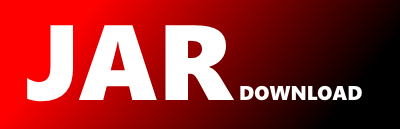
com.anlavn.ai.YourGPT Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AL-Library_VN Show documentation
Show all versions of AL-Library_VN Show documentation
Java library for many thing wonderful
package com.anlavn.ai;
// Make By Bình An || AnLaVN || KatoVN
import com.anlavn.file.Log;
import com.anlavn.file.Raw;
import com.anlavn.file.Zip;
import com.anlavn.net.DocNet;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**Lớp YourGPT hỗ trợ chạy mô hình ngôn ngữ lớn bằng cách sử dụng các tệp nhị phân dựng sẵn của llama.cpp.
* @author AnLaVN
*/
public class YourGPT implements AutoCloseable {
private static Process YGPTProcess = null;
private static String MODEL_FILE = null;
private static final String
LLAMA_PATH = "YGPT_LLaMa",
MODEL_PATH = "YGPT_Model",
MODEL_DEFAULT = "https://huggingface.co/TheBloke/Mistral-7B-OpenOrca-GGUF/resolve/main/mistral-7b-openorca.Q4_K_M.gguf";
/**Đọc thêm các tham số*/
public static Map params = new HashMap<>();
/**Tìm thêm bản dựng sẵn*/
public enum LlaMa { AVX, AVX2, AVX512, noAVX, cuBLAS11, cuBLAS12, CLBlast, OpenBLAS }
@Override public void close() throws Exception { destroy(); }
private static String getLatestLLaMa(LlaMa llama) {
String llamaFile = switch (llama) {
case AVX -> "llama-VERSION-bin-win-avx-x64.zip";
case AVX2 -> "llama-VERSION-bin-win-avx2-x64.zip";
case AVX512 -> "llama-VERSION-bin-win-avx512-x64.zip";
case noAVX -> "llama-VERSION-bin-win-noavx-x64.zip";
case cuBLAS11 -> "llama-VERSION-bin-win-cublas-cu11.7.1-x64.zip";
case cuBLAS12 -> "llama-VERSION-bin-win-cublas-cu12.2.0-x64.zip";
case CLBlast -> "llama-VERSION-bin-win-clblast-x64.zip";
case OpenBLAS -> "llama-VERSION-bin-win-openblas-x64.zip";
};
try {
String json = new DocNet("https://api.github.com/repos/ggerganov/llama.cpp/releases/latest").readAllLine();
int ind1 = json.indexOf("html_url"), ind2 = json.indexOf(",", ind1);
json = json.substring(ind1, ind2);
ind1 = json.lastIndexOf("/") + 1;
ind2 = json.length() - 1;
json = json.substring(ind1, ind2);
return ("https://github.com/ggerganov/llama.cpp/releases/download/VERSION/" + llamaFile).replaceAll("VERSION", json);
} catch (IOException e) {
Log.add("!!! Error try to init YourGPT: Can not get latest LLaMa file. !!!\n\t\tError code: " + e.toString());
throw new RuntimeException(e);
}
}
private static void setFile(String filePath, String module) {
boolean isNet = filePath.startsWith("http");
String fileName = filePath.substring(filePath.lastIndexOf("/") + 1),
fileLoca = module + "/" + fileName;
boolean isZip = fileName.endsWith(".zip"),
isExi = new File(fileLoca).exists();
MODEL_FILE = fileLoca;
if (!new File(module).exists()) new File(module).mkdir();
if (isNet && !isExi) {
try {
Log.add("Try to init YourGPT: Download from \"" + filePath + "\" to module " + module);
new DocNet(filePath).saveAs(fileLoca);
if (!isZip) return;
} catch (IOException e) {
Log.add("!!! Error try to init YourGPT: Can not init module " + module + ". Please check your connecttion. !!!\n\t\tError code: " + e.toString());
throw new RuntimeException(e);
}
}
if (isZip && !new File(module + "/main.exe").exists()) Zip.Extract(isNet ? fileLoca : filePath, module);
if (!isZip && !isExi) new Raw(filePath).copyTo(fileLoca, 26214400);
}
/**Sử dụng phương thức này để tải YGPT bằng cách sử dụng llama.cpp mới nhất (bản dựng cuBLAS12) và chạy mô hình ngôn ngữ lớn "mistral-7b-openorca.Q4_K_M.gguf".
* @see YourGPT#loadModule(com.anlavn.ai.YourGPT.LlaMa)
* @see YourGPT#loadModule(com.anlavn.ai.YourGPT.LlaMa, java.lang.String)
* @see YourGPT#loadModule(java.lang.String, java.lang.String)
*/
public static void loadModule() {
loadModule(LlaMa.cuBLAS12, MODEL_DEFAULT);
}
/**Sử dụng phương thức này để tải YGPT bằng cách sử dụng llama.cpp mới nhất và chạy mô hình ngôn ngữ lớn "mistral-7b-openorca.Q4_K_M.gguf".
* @param llama bản dựng mới nhất của llama.cpp
* @see YourGPT#loadModule()
* @see YourGPT#loadModule(com.anlavn.ai.YourGPT.LlaMa, java.lang.String)
* @see YourGPT#loadModule(java.lang.String, java.lang.String)
*/
public static void loadModule(LlaMa llama) {
loadModule(getLatestLLaMa(llama), MODEL_DEFAULT);
}
/**Sử dụng phương thức này để tải YGPT bằng cách sử dụng llama.cpp mới nhất và chạy mô hình ngôn ngữ lớn của bạn.
* @param llama bản dựng mới nhất của llama.cpp
* @param model là vị trí cụ thể của mô hình ngôn ngữ được sử dụng để chạy, nó có thể là đường dẫn cục bộ trong máy tính hoặc địa chỉ liên kết của tệp.
* Khám phá thêm tại HuggingFace
* Ví dụ:
*
"C:/Users/AnLaVN/mistral-7b-openorca.Q4_K_M.gguf" cho vị trí tập tin hoặc,
*
"https://huggingface.co/TheBloke/Mistral-7B-OpenOrca-GGUF/resolve/main/mistral-7b-openorca.Q4_K_M.gguf"
* @see YourGPT#loadModule()
* @see YourGPT#loadModule(com.anlavn.ai.YourGPT.LlaMa)
* @see YourGPT#loadModule(java.lang.String, java.lang.String)
*/
public static void loadModule(LlaMa llama, String model) {
loadModule(getLatestLLaMa(llama), model);
}
/**Sử dụng phương thức này để tải YGPT bằng cách sử dụng llama.cpp và chạy mô hình ngôn ngữ lớn của bạn.
* @param llama là vị trí cụ thể của llama.cpp dùng để chạy mô hình ngôn ngữ, nó có thể là đường dẫn cục bộ trong máy tính hoặc địa chỉ liên kết của zip.
* Khám phá thêm tại llama.cpp
* Ví dụ:
*
"C:/Users/AnLaVN/llama-b1621-bin-win-cublas-cu12.2.0-x64.zip" cho vị trí tập tin hoặc,
*
"https://github.com/ggerganov/llama.cpp/releases/download/b1621/llama-b1621-bin-win-cublas-cu12.2.0-x64.zip"
* @param model là vị trí cụ thể của mô hình ngôn ngữ được sử dụng để chạy, nó có thể là đường dẫn cục bộ trong máy tính hoặc địa chỉ liên kết của tệp.
* Khám phá thêm tại HuggingFace
* Ví dụ:
*
"C:/Users/AnLaVN/mistral-7b-openorca.Q4_K_M.gguf" cho vị trí tập tin hoặc,
*
"https://huggingface.co/TheBloke/Mistral-7B-OpenOrca-GGUF/resolve/main/mistral-7b-openorca.Q4_K_M.gguf"
* @see YourGPT#loadModule()
* @see YourGPT#loadModule(com.anlavn.ai.YourGPT.LlaMa)
* @see YourGPT#loadModule(com.anlavn.ai.YourGPT.LlaMa, java.lang.String)
*/
public static void loadModule(String llama, String model) {
setFile(llama, LLAMA_PATH);
setFile(model, MODEL_PATH);
}
/**Sử dụng phương pháp này để khởi động máy chủ llama.cpp với các tham số trước đó.
* Tự động tải mô-đun YGPT theo mặc định nếu chưa được tải.
*/
public static void start() {
if (YGPTProcess != null) {
Log.add("!!! Error try to start YourGPT: YourGPT already start before. !!!");
return;
}
if (MODEL_FILE == null) {
Log.add("YourGPT - Module has not been loaded. Try to load module by default.");
loadModule();
}
List commands = new ArrayList<>();
commands.add(LLAMA_PATH + "/server");
params.put("-m", "../" + MODEL_FILE);
for (Map.Entry entry : params.entrySet()) {
commands.add(entry.getKey());
commands.add(entry.getValue());
}
String[] command = commands.toArray(String[]::new);
new Thread() { @Override public void run() {
try {
Log.add("YourGPT - Start process with command:\n\t\t" + String.join(" ", command));
ProcessBuilder pb = new ProcessBuilder(command);
pb.directory(new File(LLAMA_PATH));
pb.redirectErrorStream(true);
YGPTProcess = pb.start();
BufferedReader reader = new BufferedReader(new InputStreamReader(YGPTProcess.getInputStream()));
int result = reader.read();
while (result != -1) {
System.out.print((char) result);
result = reader.read();
}
} catch (IOException e) {
Log.add("!!! Error try to start YourGPT: Error code: " + e.toString());
throw new RuntimeException(e);
}
}}.start();
}
/**Sử dụng phương thức này để xoá thư mục module YGPT nếu có.*/
public static void clean() {
Log.add("YourGPT - Clean YGPT module folder if exists.");
new File(LLAMA_PATH).deleteOnExit();
new File(MODEL_PATH).deleteOnExit();
}
/**Sử dụng phương thức này để buộc hủy tiến trình YGPT.*/
public static void destroy() {
if (YGPTProcess != null) {
Log.add("YourGPT - Forcibly destroy process.");
YGPTProcess.destroyForcibly();
YGPTProcess = null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy