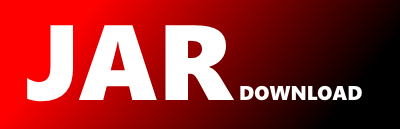
jvmMain.com.iprd.report.Transformation.kt Maven / Gradle / Ivy
/* IPRD Group 2022 */
package com.iprd.report
import org.hl7.fhir.r4.model.Base
import org.hl7.fhir.r4.model.DateTimeType
import org.hl7.fhir.r4.model.DateType
import org.hl7.fhir.r4.model.DecimalType
import org.hl7.fhir.r4.model.IntegerType
import java.time.Instant
class Transformation {
fun computeGestationalAge(lmp: List): String {
// https://www.slhd.nsw.gov.au/rpa/neonatal/temp/wheel.htm
// Gestational age is calculated by finding the difference of lmp date and todays date in epoch seconds and converting those seconds to weeks
val gestationalAge = (Instant.now().epochSecond - lmp.first().value.toInstant().epochSecond) / (3600 * 24 * 7)
return gestationalAge.toString()
}
fun verifyGreaterThanEqualToAndLessThanEqualToOnFhirPathValue(birthDate: List, additionalArgument: List): String {
val transformList = listOf(">=", "<=")
val finalResult: MutableList = mutableListOf()
birthDate.forEach { item ->
val differenceInSeconds = FhirPathEvaluator.getSecondsWithCurrentTime(item.value)
transformList.map { t ->
when (t) {
">=" -> differenceInSeconds >= additionalArgument.first().value.toInt()
"<=" -> differenceInSeconds <= additionalArgument[1].value.toInt()
else -> false
}
}.all {
it
}.let { result ->
if (result)
finalResult.add(item)
}
}
return finalResult.size.toString()
}
fun verifyGreaterThanEqualToOnFhirPathValue(birthDate: List, additionalArgument: List): String {
val finalResult: MutableList = mutableListOf()
birthDate.forEach {
val differenceInSeconds = FhirPathEvaluator.getSecondsWithCurrentTime(it.value)
if (differenceInSeconds >= additionalArgument.first().value.toInt()) {
finalResult.add(it)
}
}
return finalResult.size.toString()
}
fun verifyGreaterThanEqualToOnFhirPathValueForDateTimeType(birthDate: List, additionalArgument: List): String {
val finalResult: MutableList = mutableListOf()
birthDate.forEach {
val differenceInSeconds = FhirPathEvaluator.getSecondsWithCurrentTime(it.value)
if (differenceInSeconds >= additionalArgument.first().value.toInt()) {
finalResult.add(it)
}
}
return finalResult.size.toString()
}
fun verifyGreaterThanEqualToAndLessThanOnFhirPathValueForDateTimeType(birthDate: List, additionalArgument: List): String {
val transformList = listOf(">=", "<")
val finalResult: MutableList = mutableListOf()
birthDate.forEach { item ->
val differenceInSeconds = FhirPathEvaluator.getSecondsWithCurrentTime(item.value)
transformList.map { t ->
when (t) {
">=" -> differenceInSeconds >= additionalArgument.first().value.toInt()
"<" -> differenceInSeconds <= additionalArgument[1].value.toInt()
else -> false
}
}.all {
it
}.let { result ->
if (result)
finalResult.add(item)
}
}
return finalResult.size.toString()
}
fun transformTwoArgumentsForTest(firstArg: DecimalType, secondArg: DecimalType): String {
val firstArgDoubleValue = firstArg.value
val secondArgDoubleValue = secondArg.value
return (firstArgDoubleValue!! + secondArgDoubleValue!!).toString()
}
fun transformTwoArgumentsForTestWithDifferentArgType(firstArg: DecimalType, secondArg: IntegerType): String {
val decimalValue = DecimalType(secondArg.value.toString())
return (firstArg.value + decimalValue.value).toString()
}
fun processFhirPath(parameterList: List , transform: String, additionalArgument: List ?): String {
val method = this::class.members.single { it.name == transform }
return if (additionalArgument != null) {
method.call(this, parameterList, additionalArgument).toString()
} else {
method.call(this, parameterList).toString()
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy