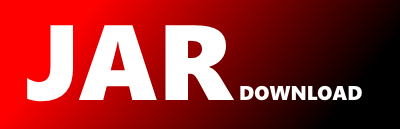
org.ndx.agile.architecture.gitlab.GitLabTicket Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gitlab-scm-handler Show documentation
Show all versions of gitlab-scm-handler Show documentation
Allow gitlab infos to be included in project documentation
The newest version!
package org.ndx.agile.architecture.gitlab;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import org.gitlab4j.api.models.Discussion;
import org.gitlab4j.api.models.Issue;
import org.ndx.agile.architecture.base.enhancers.tickets.Comment;
import org.ndx.agile.architecture.base.enhancers.tickets.Ticket;
import org.ndx.agile.architecture.base.enhancers.tickets.TicketStatus;
public class GitLabTicket implements Ticket {
private Issue source;
private List discussion;
public GitLabTicket(Issue issue, List list) {
this.source = issue;
this.discussion = list;
}
@Override
public String getUrl() {
return source.getWebUrl();
}
@Override
public String getId() {
return source.getExternalId();
}
@Override
public String getTitle() {
return source.getTitle();
}
@Override
public String getText() {
return source.getDescription();
}
@Override
public TicketStatus getStatus() {
switch(source.getState()) {
case OPENED:
case REOPENED:
return TicketStatus.OPEN;
case CLOSED:
return TicketStatus.CLOSED;
default:
throw new GitLabHandlerException(String.format("How can an issue %s be in state %s?", source.getWebUrl(), source.getState()));
}
}
@Override
public Date getLastDate() {
switch (getStatus()) {
case OPEN:
return source.getCreatedAt();
case CLOSED:
return source.getClosedAt();
default:
throw new GitLabHandlerException(String.format("How can an issue %s be in state %s?", source.getWebUrl(), source.getState()));
}
}
@Override
public Optional getDateOf(TicketStatus changeTo) {
switch (changeTo) {
case OPEN:
return Optional.ofNullable(source.getCreatedAt());
case CLOSED:
return Optional.ofNullable(source.getClosedAt());
default:
throw new GitLabHandlerException(String.format("How can an issue %s be in state %s?", source.getWebUrl(), source.getState()));
}
}
@Override
public Collection getComments() {
return discussion.stream()
.flatMap(discussion -> discussion.getNotes().stream())
.map(note -> new GitLabComment(note))
.collect(Collectors.toList());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy