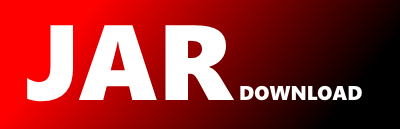
com.version1.DataBaseManagement.DatabaseHelper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenium-java-test-automation Show documentation
Show all versions of selenium-java-test-automation Show documentation
A simple Selenium framework offering externalised configuration, a good selection of libraries for supporting
test data, simple WebDriver browser binary resolution and an opinionated approach for WebDriver test design.
The newest version!
package com.version1.DataBaseManagement;
import org.apache.commons.dbutils.DbUtils;
import org.apache.commons.dbutils.QueryRunner;
import org.apache.commons.dbutils.handlers.MapListHandler;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.fasterxml.jackson.databind.JsonNode;
import com.version1.webdriver.configuration.TestConfigHelper;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
/**
* Database helper utility class providing support methods for DataBase connection
* and executing the queries
* JDBC configuration is fetched from config.json
*/
public class DatabaseHelper {
private static final Logger LOG = LoggerFactory.getLogger(DatabaseHelper.class);
private static final String jdbcUrl;
private static final String jdbcDriver;
private static final String jdbcUser;
private static final String jdbcPwd;
protected static Connection conn = null;
public static Map DBConfig = new HashMap<>();
static {
Iterator> templateConfigs = TestConfigHelper.get()
.getTemplateConfigs("Cucumber")
.fields();
while (templateConfigs.hasNext()) {
HashMap.Entry entry = templateConfigs.next();
DBConfig.put(entry.getKey(), entry.getValue().asText());
}
jdbcUrl = DBConfig.get("jdbcUrl").toString();
jdbcDriver = DBConfig.get("jdbcDriver").toString();
jdbcUser = DBConfig.get("jdbcUser").toString();
jdbcPwd = DBConfig.get("jdbcPwd").toString();
conn=setUpConnection();
}
/**
* Steps up the connection to the database using the jdbc configurations
*/
protected static Connection setUpConnection() {
try {
DbUtils.loadDriver(jdbcDriver);
return DriverManager.getConnection(jdbcUrl, jdbcUser, jdbcPwd);
} catch (SQLException se) {
LOG.info(se.getMessage());
} finally {
DbUtils.closeQuietly(conn);
}
return conn;
}
/**
* Executed the Query to the database
* @param sqlQuery to be executed in the database
* @return List of values returned by the executed Query
*/
public List executeQuery(String sqlQuery) throws SQLException {
return getQueryRunner().query(conn, sqlQuery, new MapListHandler());
}
/**
* Creates the instance of the QuerryRunner class
* QuerryRunner class executes SQL queries with pluggable strategies for handling ResultSets
* @return QueryRunner the instance of the QueryRunner class
*/
protected QueryRunner getQueryRunner() {
return new QueryRunner();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy