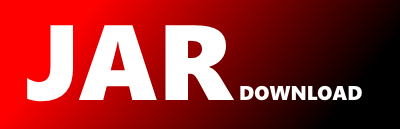
com.version1.screenshots.CaptureScreenshots Maven / Gradle / Ivy
package com.version1.screenshots;
import java.io.File;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Map;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.support.events.EventFiringWebDriver;
import org.slf4j.Logger;
import org.testng.ITestResult;
import org.testng.TestListenerAdapter;
import cucumber.api.Scenario;
/**
* Captures the Screenshots whenever there is any test failure
* Consists of two different implementations methods for cucumber and testng.
*/
public class CaptureScreenshots extends TestListenerAdapter {
public static File screenshotFile=null;
public static String targetLocation = null;
public static String reportsPath = null;
public static String testClassName = null;
public static File targetFile = null;
/**
* Capture screenshot whenever there is failure of a scenerio in cucumber
* @param scenario for inspecting results
* @param webDriver used to execute the testcases
* @param LOG for logging through concrete implementations
* @param testId of the execution generated randomly using base encoding
*/
public static void cucumberScreenshots(Scenario scenario,EventFiringWebDriver webDriver,Logger LOG,String testId){
Map screenShots = ScreenshotsHelper.getScreenShotsForCurrentTest();
for (Map.Entry screenShot : screenShots.entrySet()) {
scenario.write(screenShot.getKey());
scenario.embed((byte[]) screenShot.getValue(), "image/png");
}
ScreenshotsHelper.tidyUpAfterTestRun();
if (scenario.isFailed()) {
scenario.write(webDriver.getCurrentUrl());
byte[] screenShot = ((TakesScreenshot) webDriver).getScreenshotAs(OutputType.BYTES);
scenario.embed(screenShot, "image/png");
}
}
/**
* Capture screenshot whenever there is failure of a test method in testng
* @param result of type ITestResult for inspecting results
* @param webDriver used to execute the testcases
* @param folderPath for storing the screenshot
*/
public static void testNGScreenshots(ITestResult result,EventFiringWebDriver webDriver,String folderPath) {
Calendar calendar = Calendar.getInstance();
testClassName = result.getTestClass().getRealClass().getSimpleName();
SimpleDateFormat formater = new SimpleDateFormat("dd_MM_yyyy_hh_mm_ss");
String timeStamp = formater.format(calendar.getTime());
String testMethodName = result.getName().toString().trim();
String screenShotName = testMethodName + timeStamp + ".png";
String fileSeperator = System.getProperty("file.separator");
reportsPath = System.getProperty("user.dir") + folderPath + "screenshots";
screenshotFile = ((TakesScreenshot) webDriver).getScreenshotAs(OutputType.FILE);
try {
targetLocation = reportsPath + fileSeperator + testClassName + fileSeperator + screenShotName;
targetFile = new File(targetLocation);
FileUtils.copyFile(screenshotFile, targetFile);
} catch (IOException e) {
e.printStackTrace();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy