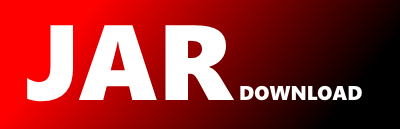
com.version1.secretsmanagement.SecretsManagement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of selenium-java-test-automation Show documentation
Show all versions of selenium-java-test-automation Show documentation
A simple Selenium framework offering externalised configuration, a good selection of libraries for supporting
test data, simple WebDriver browser binary resolution and an opinionated approach for WebDriver test design.
The newest version!
package com.version1.secretsmanagement;
import com.azure.core.util.polling.SyncPoller;
import com.azure.identity.DefaultAzureCredentialBuilder;
import com.azure.security.keyvault.secrets.SecretClient;
import com.azure.security.keyvault.secrets.SecretClientBuilder;
import com.azure.security.keyvault.secrets.models.DeletedSecret;
import com.azure.security.keyvault.secrets.models.KeyVaultSecret;
/**
* SecretsManagement class providing support methods to access the secrets from Azure key vault
* Use this set access and delete the secrets from the Azure key vault
*/
public class SecretsManagement {
/**
* Authenticated the connection to the Azure Key Vault using KeyVault URL
* The authentication is done using Default credentials builder method
* @param keyVaultName used in the Azure portal
* @return SecretClient synchronous methods to manage secrets in the Azure Key Vault
*/
public static SecretClient secretBuilder(String keyVaultName) throws InterruptedException, IllegalArgumentException {
String keyVaultUri = "https://" + keyVaultName + ".vault.azure.net";
SecretClient secretClient = new SecretClientBuilder()
.vaultUrl(keyVaultUri)
.credential(new DefaultAzureCredentialBuilder().build())
.buildClient();
return secretClient;
}
/**
* Sets the secret in the Azure key vault
* @param keyVaultName used in the Azure portal
* @param secretName, Name to be assigned to the new secret
* @param secretValue, value of the secret
*/
public static void setSecret(String keyVaultName,String secretName,String secretValue) throws InterruptedException, IllegalArgumentException {
secretBuilder(keyVaultName).setSecret(new KeyVaultSecret(secretName, secretValue));
}
/**
* Gets the secret from Azure key vault
* @param keyVaultName used in the Azure portal
* @param secretName, Name of the secret to be fetched from the Azure key vault
* @return retrieved secret
*/
public static String getSecret(String keyVaultName,String secretName) throws InterruptedException, IllegalArgumentException {
KeyVaultSecret retrievedSecret = secretBuilder(keyVaultName).getSecret(secretName);
return retrievedSecret.getValue();
}
/**
* Deletes the secret from Azure key vault
* @param keyVaultName used in the Azure portal
* @param secretName, Name of the secret to be deleted from the Azure key vault
*/
public static void deleteSecret(String keyVaultName,String secretName) throws InterruptedException, IllegalArgumentException {
SyncPoller deletionPoller = secretBuilder(keyVaultName).beginDeleteSecret(secretName);
deletionPoller.waitForCompletion();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy